表格子组件代码
/* eslint-disable eqeqeq */
<template>
<el-table
:data="gridData"
sum-text="年度需求用电量"
show-summary
fit
:cell-class-name="cellClass"
class="tb-edit"
width="100%"
@cell-click="cellClick"
>
<el-table-column property="periodType" label="时段/月份" width="150" fixed :sortable="false" align="center">
<template slot-scope="scope">
<div v-if="scope.row.periodType == 1" class="el-input">正常时段</div>
<div v-else-if="scope.row.periodType == 2" class="el-input">高峰时段</div>
<div v-else-if="scope.row.periodType == 3" class="el-input">低谷时段</div>
</template>
</el-table-column>
<el-table-column v-for="i in 12" :key="i" :property="cellProperty(i)" width="100" :label="i+'月'" :sortable="false" align="center">
<template slot-scope="scope">
<!-- i > nowMonth 当前月及之后的月份可编辑-->
<el-input-number
v-if="customEdit && i >= nowMonth && tabClickIndex === scope.row.index && scope.column.label === tabClickLable"
v-model="scope.row[cellProperty(i)]"
v-focus :controls="false"
placeholder="请输入内容"
@blur="inputBlur(scope)"
/>
<div v-else class="el-input">{{ scope.row[cellProperty(i)] }}</div>
</template>
</el-table-column>
<el-table-column property="annualTotal" label="累计" width="100" fixed="right" :sortable="false" align="center" />
</el-table>
</template>
js
<script>
export default {
directives: { // 局部注册
'focus': {
inserted: function(el) {
// 聚焦元素 children[0]的原因是input标签外层Vue会自动渲染上一层div
el.children[0].children[0].focus()
}
}
},
props: {
gridData: {
type: Array,
default: () => ([])
},
customEdit: { // 表格月份单元格是否可编辑
type: Boolean,
default: true
}
},
data() {
return {
nowMonth: null,
tabClickIndex: null, // 当前行index
tabClickLable: null // 点击的单元格label
}
},
mounted() {
this.getDate()
},
methods: {
cellProperty(index) {
switch (index) {
case 1 : return 'january'
case 2 : return 'february'
case 3 : return 'march'
case 4 : return 'april'
case 5 : return 'may'
case 6 : return 'june'
case 7 : return 'july'
case 8 : return 'august'
case 9 : return 'september'
case 10 : return 'october'
case 11 : return 'november'
case 12 : return 'december'
}
},
getDate() { // 获取当前月份
const now = new Date() // 当前日期
this.nowMonth = now.getMonth() + 1 // 当前月
},
inputBlur(scope) {
this.tabClickLable = ''
if (this.customEdit) { // 可编辑时进行累计计算
let count = 0
for (let i = 1; i <= 12; i++) {
const labelName = this.cellProperty(i)
count = count + Number(scope.row[labelName])
}
scope.row.annualTotal = count
}
},
cellClick(row, column, cell, event) {
if (this.customEdit) {
this.tabClickIndex = row.index
this.tabClickLable = column.label
}
},
cellClass({ row, column, rowIndex, columnIndex }) {
row.index = rowIndex
if ((columnIndex >= 1 && this.nowMonth > columnIndex) || !this.customEdit) {
return 'gray-color'
} else if (column.property === 'annualTotal') {
return 'gray-color'
}
},
}
}
style
</script>
<style lang="scss" scoped>
.tb-edit >>> .gray-color{
background: #eee;
}
.tb-edit .el-input >>> .el-input__inner , .tb-edit .el-input-number >>> .el-input__inner{
height: 23px;
line-height: 23px;
}
.tb-edit .el-input-number{
width: 100%;
line-height: 23px;
}
</style>
表格参数
参数 | 说明 | 类型 | 可选值 | 默认值 |
---|---|---|---|---|
gridData | 表格数据 | Array | – | [] |
customEdit | 表格是否可编辑 | Boolean | – | true |
组件函数说明
1.组件内方法说明,该表格没有抛出任何方法,不可在父组件使用
事件 | 说明 |
---|---|
cellProperty | 根据传值来显示1-12月份单元列显示的表头文字(label) |
getDate | 该函数主要用了获取当前月份,主要用于小于当前月份的单元格不可编辑 |
cellClick | 单元格点击触发事件 |
cellClass | 更改单元格样式 |
inputBlur | 单元格内input失焦事件,重新计算当前行的月份累加数据 |
2.element show-summary开启合计后每列默认计算,可使用它的自定义合计方法做处理
methods添加方法
getSummaries(param) {
const { columns, data } = param
const sums = []
const currentIndex = columns.length - 1
columns.forEach((column, index) => {
if (index === 0) {
sums[index] = '年度需求用电量'
return
}
const values = data.map(item => Number(item[column.property]))
if (!values.every(value => isNaN(value)) && currentIndex === index) {
sums[index] = values.reduce((prev, curr) => {
const value = Number(curr)
if (!isNaN(value)) {
return prev + curr
} else {
return prev
}
}, 0)
} else {
sums[index] = ''
}
})
return sums
}
局部自定义指令
主要是element-inout-number 自动聚焦
父组件使用
1.可编辑
效果
<template>
<el-container>
<el-main>
<tradingCapacityTable :grid-data="phaseDosage" />
</el-main>
</el-container>
</template>
<script>
import tradingCapacityTable from '@/views/pxf-service-omarketservice/components/tradingCapacity'
export default {
components: {
tradingCapacityTable
},
data() {
return {
phaseDosage: [{
'january': 0,
'february': 0,
'march': 0,
'april': 0,
'may': 0,
'june': 0,
'july': 0,
'august': 0,
'september': 0,
'october': 0,
'november': 0,
'december': 0,
'periodType': 1,
'annualTotal': 0
},
{
'january': 0,
'february': 0,
'march': 0,
'april': 0,
'may': 0,
'june': 0,
'july': 0,
'august': 0,
'september': 0,
'october': 0,
'november': 0,
'december': 0,
'periodType': 2,
'annualTotal': 0
},
{
'january': 0,
'february': 0,
'march': 0,
'april': 0,
'may': 0,
'june': 0,
'july': 0,
'august': 0,
'september': 0,
'october': 0,
'november': 0,
'december': 0,
'periodType': 3,
'annualTotal': 0
}],
}
2.不可编辑
效果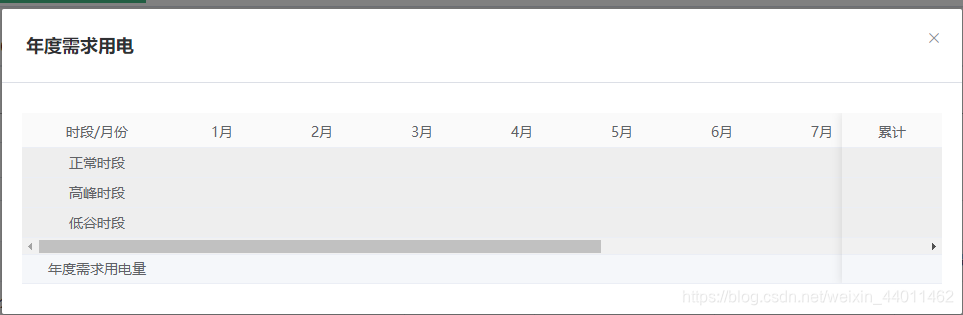
<tradingCapacityTable :grid-data="phaseDosage" :custom-edit="false" />
// 数据
phaseDosage: [{ 'periodType': 1 }, { 'periodType': 2 }, { 'periodType': 3 }],