1. BOM概述:
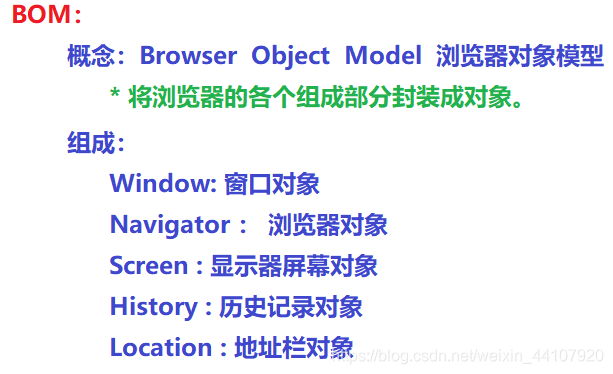
2. window_弹出方法+窗口的打开和关闭:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Window对象</title>
</head>
<body>
<input id= "openBtn" type="button" value = "打开窗口">
<input type="closeBtn" type="button" value="关闭窗口">
<script>
/*
windows 创建对象:
1.创建
2.方法
1. 与弹出窗有关的方法:
alert() 显示带有一段消息和一个确认按钮的警告键。
confirm() 显示带有一段消息以及确认按钮和取消按钮的对话框
*如果用户点击确定按钮,则方法返回true;
*如果用户点击取消按钮,则方法返回false;
prompt() 显示可提示用户输入的对话框
*返回值,获取用户输入的值。
2. 与打开关闭有关的方法
open() 打开一个新的浏览器窗口
close() 关闭浏览器窗口(默认关闭当前窗口window.close(),谁调用就关谁)
3.属性
4.特点
*Window对象不需要创建可以直接使用 window使用。 window.方法名();
*window 引用可以省略。 方法名();
*/
//方法演示一: alert()
alert("hello a");
window.alert("hello b");
//方法演示二: confirm()
var flag = confirm("您确定要退出吗?"); //会显示确认和取消
alert(flag);
if(flag){
//退出操作
alert("欢迎下次光临!");
}else{
//提示:手别抖
alert("手别抖...");
}
//方法演示三:prompt()
var s = prompt("请输入用户名");
alert(s);
//方法演示四:open()
open();
// 方法四拓展,鼠标点击一次,打开一个新窗口
var openBtn = document.getElementById("openBtn");
var newWindown;
openBtn.onclick = function(){
// 打开新窗口
newWindown = open("http://www.baidu.com");
}
//方法演示五:close()
var closenBtn = document.getElementById("closeBtn");
openBtn.onclick = function(){
// 关闭新窗口
newWindow.close();
}
</script>
</body>
</html>
3. window_定时器方法:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<script>
/*
3.与定时器有关的方法
setTimeOut() 在指定的毫秒数后调用函数或计算表达式。
* 参数有两个:
第一个参数:js代码或者方法对象 第二个参数: 毫秒值。
*返回值:唯一表示,用于取消定时器
clearTimeOut() 取消由 setTimeOut() 方法设置的 timeout。
setInterval() 按照指定的周期(以毫秒计)来调用函数或计算表达式。
clearInterval() 取消由 setInterval() 设置的 timeout.
*/
// 一次性的定时器
var aa = setTimeout("alert('boom~~~')",3000);
// 取消该定时器
clearTimeout(aa);
setTimeout("fun();",5000);
setTimeout(fun,8000);
function fun(){
alert('boom~~~');
}
// 循环定时器
var bb = setInterval(fun,2000); // 每2s boom 一次
// 取消循环定时器
clearInterval(bb);
</script>
</body>
</html>
Window属性
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<input id= "openBtn" type="button" value = "打开窗口">
<script>
/*
BOM属性:
1.获取其他BOM对象:
history
location
Navigator
Screen
*/
// 获取当前页面的历史记录 history
var h1 = window.history
var h2 = history
alert(h1);
alert(h2);
window.document.getElementById("");
document.getElementById("");
var openBtn = document.getElementById("openBtn");
alert(openBtn)
</script>
</body>
</html>
Location地址栏对象
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Location对象</title>
</head>
<body>
<input type="button" id="btn" value="刷新">
<input type="button" id = "goIt" value="访问博客">
<script>
/*
1.创建:
1.window.location
2.location
2.方法:
reload() 重新加载当前文档,刷新.
3.属性:
href : 设置或返回完整的url
*/
// reload方法:定义一个按钮,点击按钮,刷新当前页面。
// 1.获取按钮
var btn = document.getElementById("btn")
// 2.绑定单击事件
btn.onclick = function(){
// 3. 刷新页面
location.reload();
}
// 获取href
var href = location.href;
//alert(href)
// 点击按钮,去访问www.csdn.net 官网
// 1.获取按钮
var goIt = document.getElementById("goIt")
// 2. 绑定单击事件
goIt.onclick = function(){
// 3.去访问www.csdn.net 官网
location.href = "http://www.csdn.net";
}
</script>
</body>
</html>
History 历史记录对象
History 对象包含用户 (在浏览器窗口中)访问过的url
Hustory 是window对象的一部分,可通过window.history属性对其进行访问
-
创建
- window.history
- history
-
方法
- back() 加载history 列表中的前一个url
- forward() 加载history列表中的下一个url
- go() 加载history列表中的某个具体页面
-
属性
- length 返回当前窗口历史列表中的url数量
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>History对象</title>
</head>
<body>
<input type="button" id = "btn" value= "获取历史记录个数">
<a href="http://www.baidu.com">百度页面</a>
<input type="button" name="" id="forward" value="前进">
<script>
// 1.获取按钮
var btn = document.getElementById("btn")
// 2.绑定单击事件
btn.onclick = function(){
// 3. 获取当前窗口历史记录个数
var length = history.length;
alert(length);
}
// 1.获取按钮
var forward = document.getElementById("forwa")
// 2.绑定单击事件
forward.onclick = function(){
// 3. 前进
history.forward();
}
</script>
</body>
</html>