功能需求:
① 鼠标经过轮播图模块,左右键显示,离开隐藏左右键
② 点击右侧按钮一次,图片往左播放一张,以此类推,左侧按钮同理
③ 图片播放的同时,下面小圆圈模块跟随一起变化
④ 点击小圆圈,可以播放相应图片
⑤ 鼠标不经过轮播图,轮播图也会自动播放
html部分
<div class="bg">
<a href="javascript:;" class="left"><</a>
<a href="javascript:;" class="right">></a>
<ul>
<li><img src="image/清新1.png" alt=""></li>
<li><img src="image/清新2.png" alt=""></li>
<li><img src="image/清新9.jpg" alt=""></li>
<li><img src='image/清新10.jpg' alt=""></li>
</ul>
<ol class="circle">
</ol>
</div>
css部分
*{
padding: 0;
margin: 0;
}
body{
background-color: black;
}
a{
text-decoration: none;
background-color: #ccc;
width: 20px;
height: 40px;
line-height: 40px;
text-align: center;
color: white;
opacity: .7;
}
.left{
position: absolute;
top:calc(50% - 40px);
z-index: 2;
display: none;
}
.right{
position: absolute;
top:calc(50% - 40px);
right: 0;
z-index: 2;
display: none;
}
.bg{
width:300px ;
height: 550px;
background-color: aquamarine;
position: relative;
margin: 0 auto;
overflow: hidden;
}
.bg ul{
overflow: hidden;
width: 500%;
height: auto;
position: absolute;
z-index: 0;
padding-left: 0;
margin: 0;
left: calc(50% - 150px);
top: calc(50% - 250px);
}
.bg ul li{
list-style-type: none;
float: left;
}
.bg ul li img{
width: 300px;
height: 500px;
}
.circle{
background-color: pink;
list-style-type: none;
position: absolute;
bottom: 0px;
width: 100px;
height: 21px;
left: calc(50% - 50px);
display: flex;
border-radius: 10px;
}
.circle li {
border: 1px solid rgb(233, 233, 233);
height: 20px;
width: 20px;
border-radius: 10px;
margin-right:5px;
}
.circle li:last-child{
margin-right: 0;
}
.current{
background-color: white;
}
js部分
let bg = document.querySelector('.bg'); //获取背景盒子
let left = document.querySelector('.left'); //获取左边按钮
let right = document.querySelector('.right'); //获取右边按钮
let ul = bg.querySelector('ul'); //获取图片列表
let ol = bg.querySelector('ol'); //获取圆圈列表
let bgWidth = bg.offsetWidth; //获取窗口宽度
for(let i = 0 ;i<ul.children.length ;i++){ //使用循环动态生成圆圈,根据图片张数生成圆圈
let li = document.createElement('li'); //创建li标签
li.setAttribute('index',i); //使用setAttribute为li添加自定义属性 即为索引号
ol.appendChild(li); //将li标签添加至ol标签内
li.addEventListener('click',function(){ //依据循环为每个li标签添加点击事件
for(let i = 0 ;i<ol.children.length;i++){ //利用排他思想,点击前消灭所有li样式
ol.children[i].className = '';
}
this.className = 'current'; //在当前节点添加样式
let index = this.getAttribute('index',i); //获取当前index也就是索引号
num = index; //将索引号赋值给ol里面的变量
circle = index;
animate(ul,-index*bgWidth) //移动动画,ul为移动对象 -index*bgWidth 是移动距离 为索引号*窗口宽度即为图片移动距离
})
}
ol.children[0].className = 'current'; //默认第一个li的样式
bg.addEventListener('mouseenter',function(){ //当鼠标移入bg时左右con显示
left.style.display = 'block';
right.style.display = 'block';
clearInterval(timer);
timer = null;
})
bg.addEventListener('mouseleave',function(){ //当鼠标移出bg时左右con消失
left.style.display = 'none';
right.style.display = 'none';
timer = setInterval(function(){
right.click();
},2000)
})
//克隆第一张图片 将其添加至ul最后
let first = ul.children[0].cloneNode(true);
ul.appendChild(first);
let circle = 0;
let num = 0; //声明一个Num 点击依次num++ ul移动距离为num*bgWidth点击一次图片滚动一次
right.addEventListener('click',function(){ //为右按钮添加点击事件
if(num == ul.children.length-1){ //添加判断条件 如果图片滚到到最后一张时 快速将ul的left复原为0
ul.style.left = 0;
num = 0;
}
num++;
animate(ul,-num*bgWidth);
circle++;
if(circle == ol.children.length){
circle = 0;
}
circleChange();
})
left.addEventListener('click',function(){ //为左按钮添加点击事件
if(num == 0){ //添加判断条件 如果图片滚到到最后一张时 快速将ul的left复原为0
num = ul.children.length-1;
ul.style.left = -num*bgWidth + 'px';
}
num--;
animate(ul,-num*bgWidth);
circle--; //判断circle小于0的时候 小圆圈则改为第四个小圆圈
// if(circle < 0){
// circle = ol.children.length-1;
// }
circle = circle < 0? ol.children.length-1 : circle;
circleChange();
})
function circleChange(){
for(let i = 0; i<ol.children.length;i++){
ol.children[i].className = '';
}
ol.children[circle].className = 'current';
}
function animate(obj,target,callback){ //动画移动函数 obj为调用对象,target为移动距离
clearInterval(obj.timer); //当再次调用时清除之前的定时器
obj.timer = setInterval(function(){ //设置一个重复定时器
let step = (target - obj.offsetLeft) / 10 ; //
step = step > 0 ? Math.ceil(step) : Math.floor(step);
if(obj.offsetLeft == target){
clearInterval(obj.timer);
if(callback){
callback();
}
}
obj.style.left = obj.offsetLeft + step + 'px';
},15)
}
最终效果
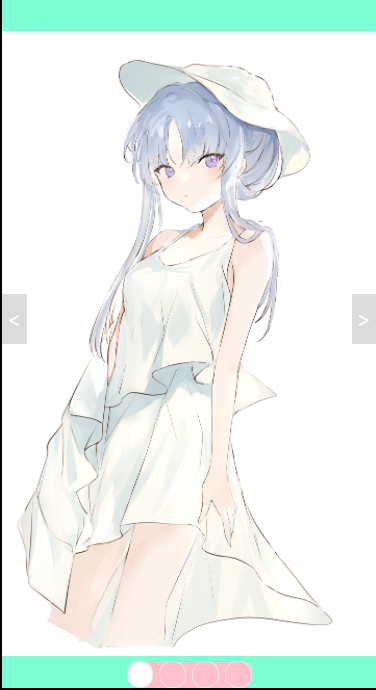