1- 思路
- 1- 定义两个指针
- pre 指针:指向所有已经翻转的链表的尾部结点
- end 指针:指向所有待翻转结点的最后一个结点
- 2- 两个指针定位后——> 处理断链逻辑,断链需要记录 end 后的节点,便于下次翻转
- 3- 断链之后 ——> 执行递归翻转链表逻辑
2- 实现
⭐25. K 个一组翻转链表——题解思路
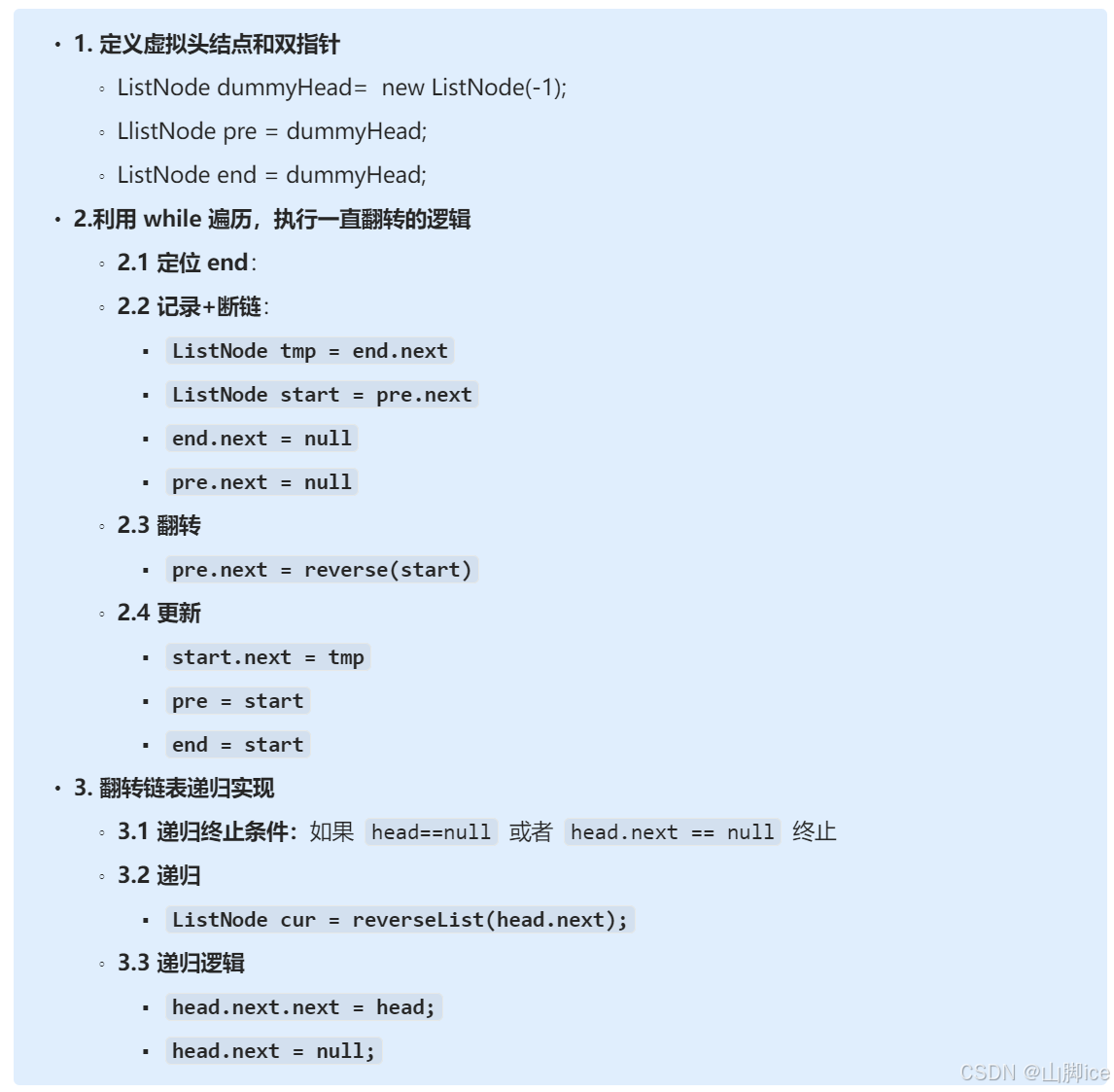
class Solution {
public ListNode reverseKGroup(ListNode head, int k) {
ListNode dummyHead = new ListNode(-1);
dummyHead.next = head;
ListNode pre = dummyHead;
ListNode end = dummyHead;
while(end.next!=null){
for(int i = 0 ;i<k && end!=null;i++){
end = end.next;
}
if(end==null){
break;
}
ListNode tmp = end.next;
ListNode start = pre.next;
end.next = null;
pre.next = null;
pre.next = reverseList(start);
start.next = tmp;
pre = start;
end = start;
}
return dummyHead.next;
}
public ListNode reverseList(ListNode head){
if(head==null || head.next == null){
return head;
}
ListNode cur = reverseList(head.next);
head.next.next = head;
head.next = null;
return cur;
}
}
3- ACM 实现
public class kreverseList {
static class ListNode{
int val;
ListNode next;
ListNode(){}
ListNode(int x){
val = x;
}
}
public static ListNode reverseKGroup(ListNode head,int k){
ListNode dummyHead = new ListNode(-1);
dummyHead.next = head;
ListNode pre = dummyHead;
ListNode end = dummyHead;
while(end.next!=null){
for(int i = 0;i<k && end!=null;i++){
end = end.next;
}
if(end==null) {
break;
}
ListNode tmp = end.next;
ListNode start = pre.next;
end.next = null;
pre.next = null;
pre.next = reverList(start);
start.next = tmp;
pre = start;
end = start;
}
return dummyHead.next;
}
public static ListNode reverList(ListNode head){
if(head==null || head.next==null){
return head;
}
ListNode cur = reverList(head.next);
head.next.next = head;
head.next = null;
return cur;
}
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
ListNode head= null;
ListNode tail = null;
System.out.println("输入链表长度");
int n = sc.nextInt();
for(int i = 0 ;i < n;i++){
ListNode newNode = new ListNode(sc.nextInt()) ;
if(head==null){
head = newNode;
tail = newNode;
}else{
tail.next = newNode;
tail = tail.next;
}
}
System.out.println("输入k");
int k = sc.nextInt();
ListNode forRes = reverseKGroup(head,k);
while(forRes!=null){
System.out.print(forRes.val+" ");
forRes = forRes.next;
}
}
}