第一种,java代码递归获取
package com.lg.sgg.product.entity;
import com.baomidou.mybatisplus.annotation.TableField;
import com.baomidou.mybatisplus.annotation.TableId;
import com.baomidou.mybatisplus.annotation.TableName;
import java.io.Serializable;
import java.util.Date;
import java.util.List;
import lombok.Data;
@Data
@TableName("pms_category")
public class PmsCategoryEntity implements Serializable {
private static final long serialVersionUID = 1L;
@TableId
private Long catId;
private String name;
private Long parentCid;
private Integer catLevel;
private Integer showStatus;
private Integer sort;
private String icon;
private String productUnit;
private Integer productCount;
@TableField(exist = false)
List<PmsCategoryEntity> children;
}
Create Table
CREATE TABLE `pms_category` (
`cat_id` bigint(20) NOT NULL AUTO_INCREMENT COMMENT '分类id',
`name` char(50) DEFAULT NULL COMMENT '分类名称',
`parent_cid` bigint(20) DEFAULT NULL COMMENT '父分类id',
`cat_level` int(11) DEFAULT NULL COMMENT '层级',
`show_status` tinyint(4) DEFAULT NULL COMMENT '是否显示[0-不显示,1显示]',
`sort` int(11) DEFAULT NULL COMMENT '排序',
`icon` char(255) DEFAULT NULL COMMENT '图标地址',
`product_unit` char(50) DEFAULT NULL COMMENT '计量单位',
`product_count` int(11) DEFAULT NULL COMMENT '商品数量',
PRIMARY KEY (`cat_id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4 COMMENT='商品三级分类'
package com.lg.sgg.product.service.impl;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper;
import com.baomidou.mybatisplus.core.metadata.IPage;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.lg.common.utils.PageUtils;
import com.lg.common.utils.Query;
import com.lg.sgg.product.dao.PmsCategoryDao;
import com.lg.sgg.product.entity.PmsCategoryEntity;
import com.lg.sgg.product.service.PmsCategoryService;
@Service("pmsCategoryService")
public class PmsCategoryServiceImpl extends ServiceImpl<PmsCategoryDao, PmsCategoryEntity> implements PmsCategoryService {
@Override
public List<PmsCategoryEntity> queryCategoryListTree() {
List<PmsCategoryEntity> pmsCategoryEntities = this.baseMapper.selectList(new QueryWrapper<>());
List<PmsCategoryEntity> entities = pmsCategoryEntities.stream().filter((categoryEntity) -> {
return categoryEntity.getParentCid().equals(0L);
}).map((categoryEntity) -> {
categoryEntity.setChildren(getChildren(categoryEntity,pmsCategoryEntities));
return categoryEntity;
}).sorted((categoryEntity1, categoryEntity2) -> {
return categoryEntity1.getSort() - categoryEntity2.getSort();
}).collect(Collectors.toList());
return entities;
}
private List<PmsCategoryEntity> getChildren(PmsCategoryEntity categoryEntity, List<PmsCategoryEntity> pmsCategoryEntities) {
return pmsCategoryEntities.stream().filter((cate)->{
return cate.getParentCid().equals(categoryEntity.getCatId());
}).map((cate)->{
cate.setChildren(getChildren(cate,pmsCategoryEntities));
return cate;
}).sorted((cate1,cate2)->{
return cate1.getSort()-cate2.getSort();
}).collect(Collectors.toList());
}
}
- 效果展示
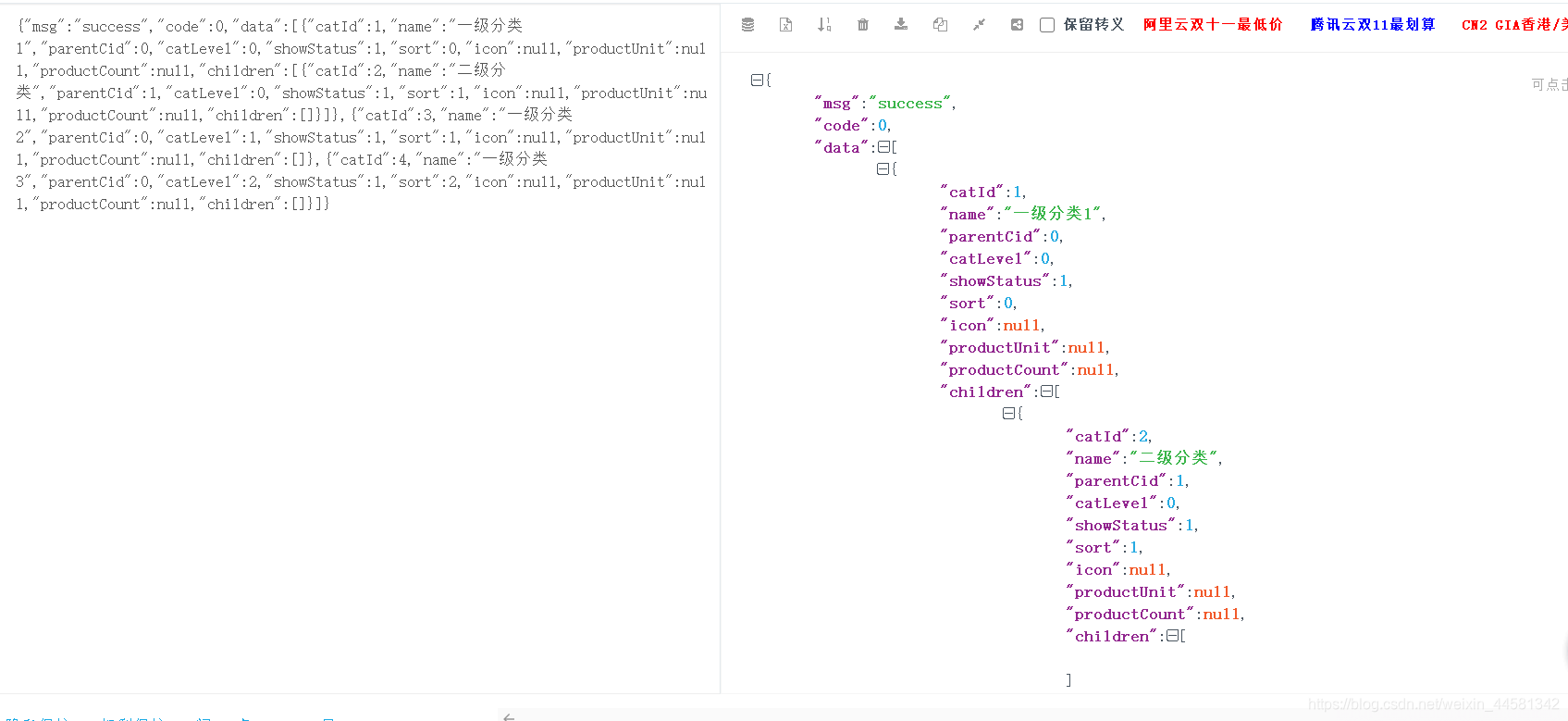
第二种,有空在写上去(mybatis递归查询语句)
@ApiModel("分组树形结构")
@Data
public class DevGroupVo {
@ApiModelProperty("id")
@NotBlank(groups = {ValidUpdateGroup.class},message = "分组id不能为空")
private String id;
@ApiModelProperty("名称")
@NotBlank(message = "分组名称不能为空")
private String name;
@ApiModelProperty("父id")
@NotBlank(message = "父id不能为空",groups = {ValidUpdateGroup.class})
@Excel(name = "父id")
private String parentId;
@ApiModelProperty("标识")
@NotBlank(message = "标识不能为空")
@Excel(name = "标识")
private String identify;
@ApiModelProperty("排序字段")
@NotNull(message = "排序字段不能为空")
@Excel(name = "排序字段")
private Integer orderNum;
private String delFlag;
private String remark;
@ApiModelProperty(value = "子级")
private List<DevGroupVo> children;
}
<resultMap type="com.xxx.devmgr.vo.DevGroupVo" id="devGroupTreeResult">
<result property="id" column="id"/>
<result property="name" column="name"/>
<result property="parentId" column="parent_id"/>
<result property="identify" column="identify"/>
<result property="orderNum" column="order_num"/>
<result property="delFlag" column="del_flag"/>
<result property="remark" column="remark"/>
<collection property="children" ofType="com.xxx.devmgr.vo.DevGroupVo" column="id"
select="getDevGroupByParentId"/>
</resultMap>
<select id="getTreeById" resultMap="devGroupTreeResult">
select id,
name,
parent_id,
identify,
order_num,
del_flag,
remark
from dev_group
where id = #{id}
and del_flag = '0'
</select>
<select id="getDevGroupByParentId" resultMap="devGroupTreeResult">
select id,
name,
parent_id,
identify,
order_num,
del_flag,
remark
from dev_group
where parent_id = #{id}
and del_flag = '0'
</select>