订单结束之后,我会发送mq消息到小程序后端得微服务,你在微服务中处理调用停车场接口。
有几点要注意:
1.最好发送http前记录一笔减免记录。
2.因为调用公网的http接口可能会存在网络问题,延迟啊超时啊,导致事物时间拉长,所以你看下要不要将减免记录新增和
停车场http接口调用事物分开处理
3.然后减免记录估计会有失败重试的功能,你看下可能会加定时器
一。保证接收到停车场的减免记录会有记录。不要因为特殊异常或者停车场接口问题 导致这笔减免丢失
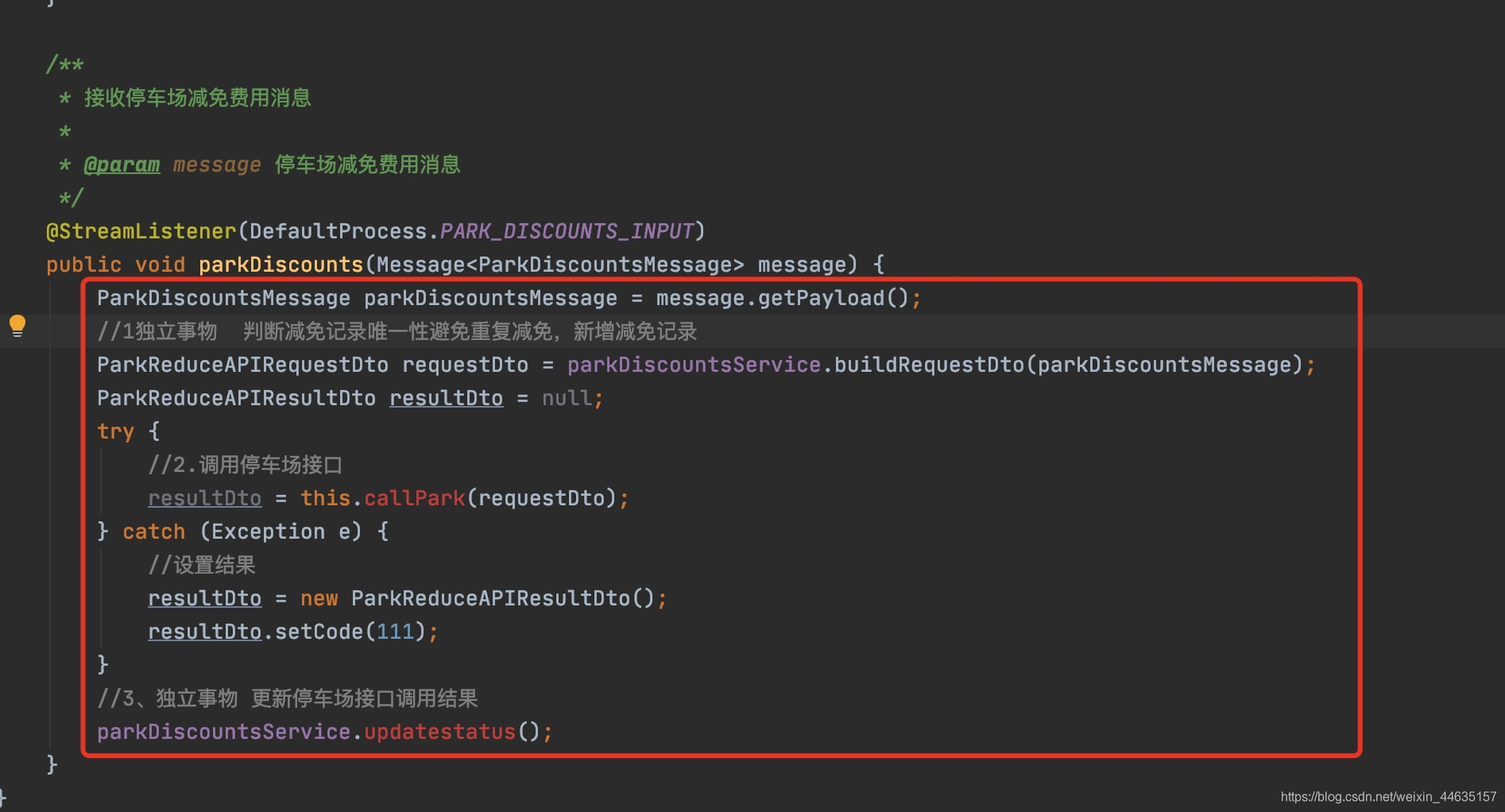
二。独立service出来做定时服务
package com.nebula.microservice.appbackend.parkdiscounts.service;
import com.nebula.microservice.appbackend.parkdiscounts.domain.OperationReductionRecord;
import com.nebula.microservice.appbackend.parkdiscounts.web.dto.*;
import com.nebula.microservice.stype.SParkReductionRecord;
import com.querydsl.core.types.Expression;
import com.querydsl.core.types.Predicate;
import com.querydsl.core.types.Projections;
import com.querydsl.sql.SQLQueryFactory;
import org.springframework.scheduling.annotation.Scheduled;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
import org.springframework.util.CollectionUtils;
import java.util.*;
@Service
public class ParkDiscountsCronService {
private final SQLQueryFactory sqlQueryFactory;
private final ParkDiscountsService parkDiscountsService;;
public ParkDiscountsCronService(SQLQueryFactory sqlQueryFactory,ParkDiscountsService parkDiscountsService) {
this.sqlQueryFactory = sqlQueryFactory;
this.parkDiscountsService = parkDiscountsService;
}
@Scheduled(cron = "0 0 23 * * ?")
@Transactional
public void recall () {
List<ParkReductionRecordDto> parkReductionRecordDtoList = this.getParkReductionRecordDto();
if (!CollectionUtils.isEmpty(parkReductionRecordDtoList)){
for (ParkReductionRecordDto recordDto: parkReductionRecordDtoList){
if (!recordDto.getCode().equals(Integer.parseInt("10000")) && !recordDto.getCode().equals(Integer.parseInt("10002")) && !recordDto.getCode().equals(Integer.parseInt("10003")) && !recordDto.getCode().equals(Integer.parseInt("10006")) && !recordDto.getCode().equals(Integer.parseInt("10007"))){
ParkReduceAPIRequestDto requestDto = new ParkReduceAPIRequestDto();
if (recordDto.getPlateNo() != null) requestDto.setPlateNo(recordDto.getPlateNo());
if (recordDto.getParkCode() != null) requestDto.setMerchId(recordDto.getParkCode());
if (recordDto.getReductionTime() != null) requestDto.setDuration(recordDto.getReductionTime());
if (recordDto.getReductionOrderNo() != null) requestDto.setBizld(recordDto.getReductionOrderNo());
if (recordDto.getStartChargingTime() != null) requestDto.setStartChargingTime(recordDto.getStartChargingTime());
if (recordDto.getStopChargingTime() != null) requestDto.setStopChargingTime(recordDto.getStopChargingTime());
if (recordDto.getSign() != null) requestDto.setSign(recordDto.getSign());
ParkReduceAPIResultDto resultDto = parkDiscountsService.callPark(requestDto);
if (resultDto != null){
Integer code = Integer.parseInt(resultDto.get("code").toString());
String msg = resultDto.get("msg").toString();
String data = resultDto.get("data").toString();
SParkReductionRecord sParkReductionRecord = SParkReductionRecord.parkReductionRecord;
sqlQueryFactory.update(sParkReductionRecord)
.set(sParkReductionRecord.prrCode,code)
.set(sParkReductionRecord.prrMsg,msg)
.set(sParkReductionRecord.prrData,data)
.where(sParkReductionRecord.prrParkId.eq(recordDto.getId()))
.execute();
}
}
}
}
}
private List<ParkReductionRecordDto> getParkReductionRecordDto() {
SParkReductionRecord sParkReductionRecord = SParkReductionRecord.parkReductionRecord;
Map<String, Expression<?>> resultMap = new HashMap<>();
resultMap.put("id", sParkReductionRecord.prrParkId);
resultMap.put("stationCode", sParkReductionRecord.prrStationCode);
resultMap.put("stationName", sParkReductionRecord.prrStationName);
resultMap.put("parkName", sParkReductionRecord.prrParkName);
resultMap.put("plateNo", sParkReductionRecord.prrPlateNo);
resultMap.put("reductionOrderNo", sParkReductionRecord.prrReductionOrderNo);
resultMap.put("startChargingTime", sParkReductionRecord.prrStartChargingTime.stringValue());
resultMap.put("stopChargingTime", sParkReductionRecord.prrStopChargingTime.stringValue());
resultMap.put("reductionTime", sParkReductionRecord.prrReductionTime);
resultMap.put("code", sParkReductionRecord.prrCode);
resultMap.put("parkCode", sParkReductionRecord.prrParkCode);
resultMap.put("sign", sParkReductionRecord.prrSign);
List<Predicate> predicateList = new ArrayList<>();
List<ParkReductionRecordDto> parkReductionRecordList = sqlQueryFactory
.select(Projections.bean(ParkReductionRecordDto.class, resultMap))
.from(sParkReductionRecord)
.where(predicateList.toArray(new Predicate[0])).fetch();
return parkReductionRecordList;
}
}
三.MQ的发送
package com.nebula.microservice.operationmanage.rabbitmq.provider;
import com.nebula.microservice.operationmanage.organization.web.dto.StationWeatherDTO;
import com.nebula.microservice.operationmanage.rabbitmq.DefaultProcess;
import com.nebula.module.common.message.ManualStopChargeMessage;
import com.nebula.module.common.message.RefundMessage;
import com.nebula.module.common.message.ServerUpdateMessage;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.cloud.stream.annotation.EnableBinding;
import org.springframework.integration.support.MessageBuilder;
import org.springframework.messaging.MessageChannel;
import javax.inject.Inject;
@EnableBinding(DefaultProcess.class)
public class MessageProvider {
private final MessageChannel refundChannel;
private final MessageChannel pileUpgradeChannel;
private final MessageChannel manualCloseOrderChannel;
private final MessageChannel weatherOutputChannel;
@Inject
public MessageProvider(@Qualifier(value = DefaultProcess.REFUND_OUTPUT) MessageChannel refundChannel,
@Qualifier(value = DefaultProcess.PILE_UPGRADE_OUTPUT) MessageChannel pileUpgradeChannel,
@Qualifier(value = DefaultProcess.MANUAL_CLOSE_ORDER_OUTPUT) MessageChannel manualCloseOrderChannel,
@Qualifier(value = DefaultProcess.STATION_WEATHER_OUTPUT) MessageChannel weatherOutputChannel) {
this.refundChannel = refundChannel;
this.pileUpgradeChannel = pileUpgradeChannel;
this.manualCloseOrderChannel = manualCloseOrderChannel;
this.weatherOutputChannel=weatherOutputChannel;
}
public void sendRefundMessage(RefundMessage refundMessage) {
refundChannel.send(MessageBuilder.withPayload(refundMessage).build());
}
public void sendPileUpgrade(ServerUpdateMessage serverUpdateMessage) {
pileUpgradeChannel.send(MessageBuilder.withPayload(serverUpdateMessage).build());
}
public void sendManualCloseOrder(ManualStopChargeMessage stopChargeMessage) {
manualCloseOrderChannel.send(MessageBuilder.withPayload(stopChargeMessage).build());
}
public void sendStationWeather(StationWeatherDTO weatherDTO){
weatherOutputChannel.send(MessageBuilder.withPayload(weatherDTO).build());
}
}