系统操作日志
自定义注解类MyLog
package com.pm.common.annotation;
import java.lang.annotation.*;
@Target(ElementType.METHOD)
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface MyLog {
String value() default "";
}
Controller @MyLog(value=“添加加减分项”)
@MyLog(value="添加加减分项")
定义SysLogAspectController
package com.pm.common.aspect;
import java.lang.reflect.Method;
import javax.servlet.http.HttpServletRequest;
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.AfterReturning;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
import org.aspectj.lang.reflect.MethodSignature;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.alibaba.fastjson.JSON;
import com.pm.common.annotation.MyLog;
import com.pm.entity.po.security.OperatingLog;
import com.pm.entity.po.security.User;
import com.pm.service.security.OperatingLogService;
import com.pm.utils.HttpContextUtils;
import com.pm.utils.IPUtils;
@Aspect
@Component
public class SysLogAspect {
@Autowired
private OperatingLogService operatingLogService;
@Pointcut("@annotation(com.pm.common.annotation.MyLog)")
public void logPoinCut() {
}
@AfterReturning("logPoinCut()")
public void saveSysLog(JoinPoint joinPoint) {
OperatingLog sysLog = new OperatingLog();
MethodSignature signature = (MethodSignature) joinPoint.getSignature();
Method method = signature.getMethod();
MyLog myLog = method.getAnnotation(MyLog.class);
if (myLog != null) {
String value = myLog.value();
sysLog.setOperation(value);
}
String className = joinPoint.getTarget().getClass().getName();
String methodName = method.getName();
sysLog.setMethod(className + "." + methodName);
Object[] args = joinPoint.getArgs();
String params = JSON.toJSONString(args);
sysLog.setParams(params);
HttpServletRequest request = HttpContextUtils.getHttpServletRequest();
User user = null;
try {
user = (User) request.getAttribute("CurrentUser");
} catch (Exception e) {
user = new User(String.valueOf(args[0]), String.valueOf(args[1]));
}
if(user==null) {
user = new User(String.valueOf(args[0]), String.valueOf(args[1]));
}
sysLog.setUsername(user.getUsername());
sysLog.setIp(IPUtils.getIpAddr(request));
operatingLogService.add(sysLog);
}
}
定义Service
package com.pm.service.security;
import com.pm.entity.po.security.OperatingLog;
import com.pm.service.BaseService;
public interface OperatingLogService extends BaseService<OperatingLog>{
}
定义Impl
package com.pm.service.security.impl;
import javax.annotation.Resource;
import org.springframework.stereotype.Service;
import com.pm.entity.po.security.OperatingLog;
import com.pm.mapper.security.OperatingLogMapper;
import com.pm.service.impl.BaseServiceImpl;
import com.pm.service.security.OperatingLogService;
@Service
public class OperatingLogServiceImpl extends BaseServiceImpl<OperatingLog> implements OperatingLogService {
@Resource
public void setBasemapper(OperatingLogMapper operatingLogMapper) {
super.setBaseMapper(operatingLogMapper);
}
}
定义Mapper
定义Mapper.xml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.pm.mapper.security.OperatingLogMapper">
<resultMap id="BaseResultMap" type="com.pm.entity.po.security.OperatingLog">
<id column="id" jdbcType="INTEGER" property="id" />
<result column="username" jdbcType="VARCHAR" property="username" />
<result column="operation" jdbcType="VARCHAR" property="operation" />
<result column="method" jdbcType="VARCHAR" property="method" />
<result column="params" jdbcType="VARCHAR" property="params" />
<result column="ip" jdbcType="VARCHAR" property="ip" />
<result column="model_value" jdbcType="TIMESTAMP" property="modelValue" />
<result column="create_date" jdbcType="TIMESTAMP" property="createDate" />
</resultMap>
<sql id="queryLog">
SELECT g.*
FROM
operating_log AS g
</sql>
<insert id="add" parameterType="com.pm.entity.po.security.Group">
INSERT INTO operating_log
(username,
operation,
method,
params,
ip,
model_value
)
VALUES(
#{username},
#{operation},
#{method},
#{params},
#{ip},
#{modelValue})
</insert>
<select id="loadById" resultMap="BaseResultMap">
<include refid="queryLog" />
WHERE
g.id = #{id}
</select>
<select id="getList" resultMap="BaseResultMap">
<include refid="queryLog" />
where 1=1
<if test="username!=null and username!=''">
and g.username=#{username}
</if>
<if test="operation!=null and operation!=''">
and g.opration=#{operation}
</if>
</select>
<select id="load" resultType="com.pm.entity.po.security.Group">
<include refid="queryLog" />
WHERE
g.id = #{id}
</select>
</mapper>
MySql数据库
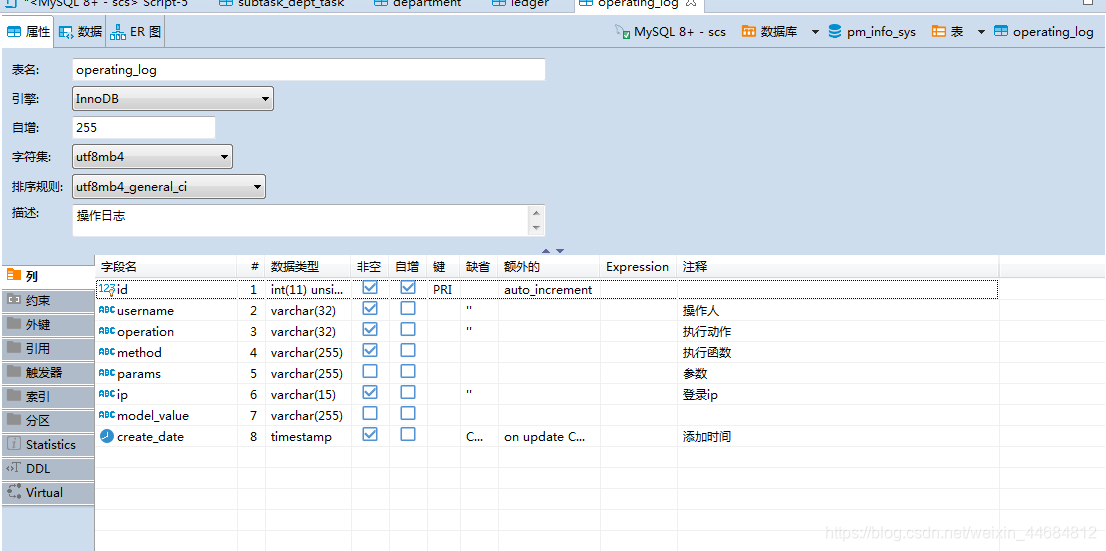