package day10;
import java.util.Random;
import java.util.Scanner;
/**
* 用一个二维数组代表地图。二维数组中0代表空,1代表人,2代表箱子,3代表终点,
* 用随机器分别生成箱子,人,终点的坐标。
* 从键盘中输入"w","s","a","d",分别代表上下左右移动的操作,每操作一次打印一次地图。
*
*
*/
public class TXZ {
//在控制台打印地图。0-□ 代表空,1-●代 表人,2-■ 代表箱子,3-▲ 代表终点。
public static void showMap(int[][] map) {
for (int i = 0; i < map.length; i++) {
for (int j = 0; j < map[i].length; j++) {
if (map[i][j] == 0) {
System.out.print("□ ");
} else if (map[i][j] == 1) {
System.out.print("● ");
} else if (map[i][j] == 2) {
System.out.print("■ ");
} else {
System.out.print("▲ ");
}
}
System.out.println();
}
}
public static void main(String[] args) {
//初始化一张地图
Scanner sc = new Scanner(System.in);//扫描仪
Random r = new Random();//随机器
int[][] map = new int[10][10];//生成一个二维数组(地图)
int personX = r.nextInt(10);//随机生成人的横坐标
int personY = r.nextInt(10);//随机生成人的纵坐标
int chestX = r.nextInt(8) + 1;//随机生成箱子的横坐标
int chestY = r.nextInt(8) + 1;//随机生成箱子的横坐标
int targetX = r.nextInt(10);//随机生成终点的横坐标
int targetY = r.nextInt(10);//随机生成终点的横坐标
map[targetY][targetX] = 3;//将终点的纵坐标赋值为3
System.out.println("提示:w为向上移动,s为向下移动,a为向左移动,d为向右移动。请移动");
while (true) {
map[personY][personX] = 1;//将人的纵坐标赋值为1
map[chestY][chestX] = 2;//将箱子的纵坐标赋值为2
//判断箱子的坐标是否等于终点的坐标,如果满足跳出循环,游戏结束
if (map[chestY][chestX] == map[targetY][targetX]) {
map[chestY][chestX] = 3;//将箱子的值设为3
System.out.println("恭喜通关");
showMap(map);//打印地图
break;
}
showMap(map);//打印地图
System.out.println("提示:w为向上移动,s为向下移动,a为向左移动,d为向右移动。请移动");
String move = sc.next();//输入要移动的方向
// 向上一种移动的操作,其余三个方向类似。
if (move.equals("w")) {
//判断人的纵坐标是否大于0,大于零才能向上移动(防止人移动越界)
if (personY > 0) {
//判断1.人的横坐标受否等于人的纵坐标且人要在终点的下面(这个判断是不能让终点被人吃掉),如果满足不进行任何的操作
//判断2.箱子的纵坐标为0且人的横坐标和箱子的横坐标相等(防止人把箱子推出界外),如果满足不进行任何的操作
if (personX == targetX && personY == targetY + 1||chestY==0&&chestX==personX) {
} else {
//推箱子和人移动的操作
//如果箱子在人的上方,箱子纵坐标见减一
if (personX == chestX && personY == chestY + 1) {
chestY--;
}
map[personY][personX] = 0;//人的坐标归为0
personY--;//箱子纵坐标见减一
}
}
} else if (move.equals("s")) {
if (personY < 9) {
if (personX == targetX && personY == targetY - 1||chestY==9&&chestX==personX) {
} else {
if (personX == chestX && personY == chestY - 1) {
chestY++;
}
map[personY][personX] = 0;
personY++;
}
}
} else if (move.equals("a")) {
if (personX > 0) {
if (personY == targetY && personX == targetX + 1||chestX==0&&chestY==personY) {
}else {
if (personY == chestY && personX == chestX + 1) {
chestX--;
}
map[personY][personX] = 0;
personX--;
}
}
} else if (move.equals("d")) {
if (personX < 9) {
if (personY == targetY && personX == targetX - 1||chestX==9&&chestY==personY) {
}else {
if (personY == chestY && personX == chestX - 1) {
chestX++;
}
map[personY][personX] = 0;
personX++;
}
}
} else {
System.out.println("输入错误");
}
}
}
}
控制台的小游戏推箱子
最新推荐文章于 2022-03-18 19:14:26 发布
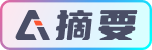