IO
import java.io.File;
import java.io.IOException;
import java.io.ObjectInputStream.GetField;
public class ioDemo {
public static void main(String[] args) throws IOException {
File f=new File("d:\\b.txt");
File f1=new File("a.txt");
System.out.println(f1.createNewFile());
File f2=new File("b");
System.out.println(f2.mkdir());
File f3=new File("c\\d\\e");
System.out.println(f3.mkdirs());
System.out.println(f3.delete());
String name=f1.getName();
long length=f1.length();
boolean hidden=f1.isHidden();
System.out.println("文件名称:"+name);
System.out.println("文件长度:"+length);
System.out.println("该文件是隐藏文件?"+hidden);
}
}
- 使用FileOutPutStream类向文件word.txt写入信息,然后通过FileInputStream类将文件中的数据读取到控制台
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.FilePermission;
public class Filetest {
public static void main(String[] args) {
File file=new File("word.txt");
try {
FileOutputStream out =new FileOutputStream(file);
byte buy[]="我有一只小毛驴,从来也不骑".getBytes();
out.write(buy);
out.close();
} catch (Exception e) {
e.printStackTrace();
}
try {
FileInputStream in = new FileInputStream(file);
byte byt[] = new byte[1024];
int len = in.read(byt);
System.out.println("文件中的信息是:" + new String(byt, 0, len));
in.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}
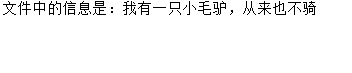
- FileReader和FileWriter类
(由于汉字在文件中占两个字符,如果使用字节流读取不好可能会出现乱码现象,此时采用字符流Reader或Writer即可避免这种情况)
import java.awt.*;
import java.awt.event.*;
import java.io.*;
import javax.swing.*;
public class ftest extends JFrame {
private JScrollPane scrollPane;
private static final long serialVersionUID = 1L;
private JPanel jContentPane = null;
private JTextArea jTextArea = null;
private JPanel controlPanel = null;
private JButton openButton = null;
private JButton closeButton = null;
private JTextArea getJTextArea() {
if (jTextArea == null) {
jTextArea = new JTextArea();
}
return jTextArea;
}
private JPanel getControlPanel() {
if (controlPanel == null) {
FlowLayout flowLayout = new FlowLayout();
flowLayout.setVgap(1);
controlPanel = new JPanel();
controlPanel.setLayout(flowLayout);
controlPanel.add(getOpenButton(), null);
controlPanel.add(getCloseButton(), null);
}
return controlPanel;
}
private JButton getOpenButton() {
if (openButton == null) {
openButton = new JButton();
openButton.setText("写入文件");
openButton
.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(ActionEvent e) {
File file = new File("word.txt");
try {
FileWriter out = new FileWriter(file);
String s = jTextArea.getText();
out.write(s);
out.close();
} catch (Exception e1) {
e1.printStackTrace();
}
}
});
}
return openButton;
}
private JButton getCloseButton() {
if (closeButton == null) {
closeButton = new JButton();
closeButton.setText("读取文件");
closeButton
.addActionListener(new java.awt.event.ActionListener() {
public void actionPerformed(ActionEvent e) {
File file = new File("word.txt");
try {
FileReader in = new FileReader(file);
char byt[] = new char[1024];
int len = in.read(byt);
jTextArea.setText(new String(byt, 0, len));
in.close();
} catch (Exception e1) {
e1.printStackTrace();
}
}
});
}
return closeButton;
}
public ftest() {
super();
initialize();
}
private void initialize() {
this.setSize(300, 200);
this.setContentPane(getJContentPane());
this.setTitle("JFrame");
}
private JPanel getJContentPane() {
if (jContentPane == null) {
jContentPane = new JPanel();
jContentPane.setLayout(new BorderLayout());
jContentPane.add(getScrollPane(), BorderLayout.CENTER);
jContentPane.add(getControlPanel(), BorderLayout.SOUTH);
}
return jContentPane;
}
public static void main(String[] args) {
ftest thisClass = new ftest();
thisClass.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
thisClass.setVisible(true);
}
protected JScrollPane getScrollPane() {
if (scrollPane == null) {
scrollPane = new JScrollPane();
scrollPane.setViewportView(getJTextArea());
}
return scrollPane;
}
}
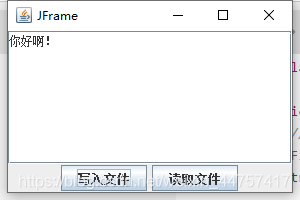
- 带缓存的输入输出流
- BufferedInputStream与BufferedOutputStream类
- BufferedReader与BufferedWriter
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
public class t1 {
public static void main(String[] args) {
String content[]= {"好久不见","最近好吗","常联系"};
File file=new File("1.txt");
try {
FileWriter fw=new FileWriter(file);
BufferedWriter bufw=new BufferedWriter(fw);
for(int k=0;k<content.length;k++){
bufw.write(content[k]);
bufw.newLine();
}
bufw.close();
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
FileReader fr=new FileReader(file);
BufferedReader bufr=new BufferedReader(fr);
String s=null;
int i=0;
while((s=bufr.readLine())!=null) {
i++;
System.out.println("第"+i+"行"+s);
}
bufr.close();
fr.close();
} catch (Exception e) {
e.printStackTrace();
}
}
}

import java.io.DataInputStream;
import java.io.DataOutput;
import java.io.DataOutputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
public class e1 {
public static void main(String[] args) {
try {
FileOutputStream fs=new FileOutputStream("work.txt");
DataOutputStream ds=new DataOutputStream(fs);
ds.writeUTF("使用writeUTF()方法写入数据:");
ds.writeChars("使用writeChars写入数据:");
ds.writeBytes("使用writeBytes写入数据:");
ds.close();
FileInputStream fis=new FileInputStream("work.txt");
DataInputStream dis=new DataInputStream(fis);
System.out.println(dis.readUTF());
} catch (Exception e) {
e.printStackTrace();
}
}
}
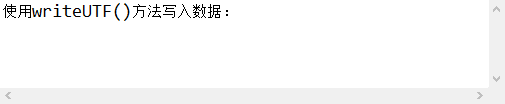