"""多进程使用消息队列 将任务放入/取出队列时不等待,但队列已满/为空时,会报错,所以要使用try: finnaly"""
import multiprocessing
from multiprocessing import Process, Queue
import time
import os
def inputQ(queue):
"""加入队列"""
# queue.put(str(os.getpid()) + " -- " + str(time.time())) # 当队列已满时,会阻塞,等待
try:
queue.put_nowait(str(os.getpid()) + " -- " + str(time.time()))
print("用户 %d 进来要抽奖了 ,添加到队列" % os.getpid())
except Exception as e:
print("队列已满, %d" % os.getpid(), e)
def outputQ(queue):
"""从队列内取出数据"""
try:
data = queue.get_nowait() # 当队列内无数据时,不会阻塞,会报错
except Exception as e:
print("队列已空", e)
with open("data.txt", "r") as f:
num = int(f.read())
if num > 0:
num_end = num
num_end -= 1
with open("data.txt", "w") as f:
f.write(str(num_end))
print(str(os.getpid()) + "用户 '%s' 已抽奖,抽奖前库存:%d,抽奖后库存:%d" %(data, num, num_end))
else:
print(str(os.getpid()) + "无库存了")
def run_test(q):
"""模拟有10个线程往队列内插入数据,有100个线程从队列内取数据"""
for i in range(10):
p = Process(target=inputQ, args=(q,))
p.start()
record_in.append(p)
for i in range(100): # 抽奖用户数比奖品数量多, 这种情况如果使用get_nowait()不会阻塞
p = Process(target=outputQ, args=(q,))
p.start()
record_out.append(p)
if __name__ == '__main__':
multiprocessing.freeze_support()
record_in = []
record_out = []
q = Queue(2) # 设置队列长度
run_test(q)
Python 进程 进程池 非阻塞
最新推荐文章于 2024-10-29 11:13:58 发布
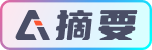