基于百度地图API实现"网易出行&Travel Planing"
作者:黑衣侠客
一、前言
这次项目主要是因为软件工程老师布置的作业,实现一个名为Travel Planing的课程设计,基于具体要求,我决定基于之前项目的基础上,用Android实现一个可互通网络的地图APP,目前这个项目还没有完全成熟,只是有一个大体的功能框架,实现了部分功能,日后我会继续完善Travel Planing的功能,完善博客内容。
使用工具:Androidstudio
使用网络服务器:leancloud、百度地图
视图展示:
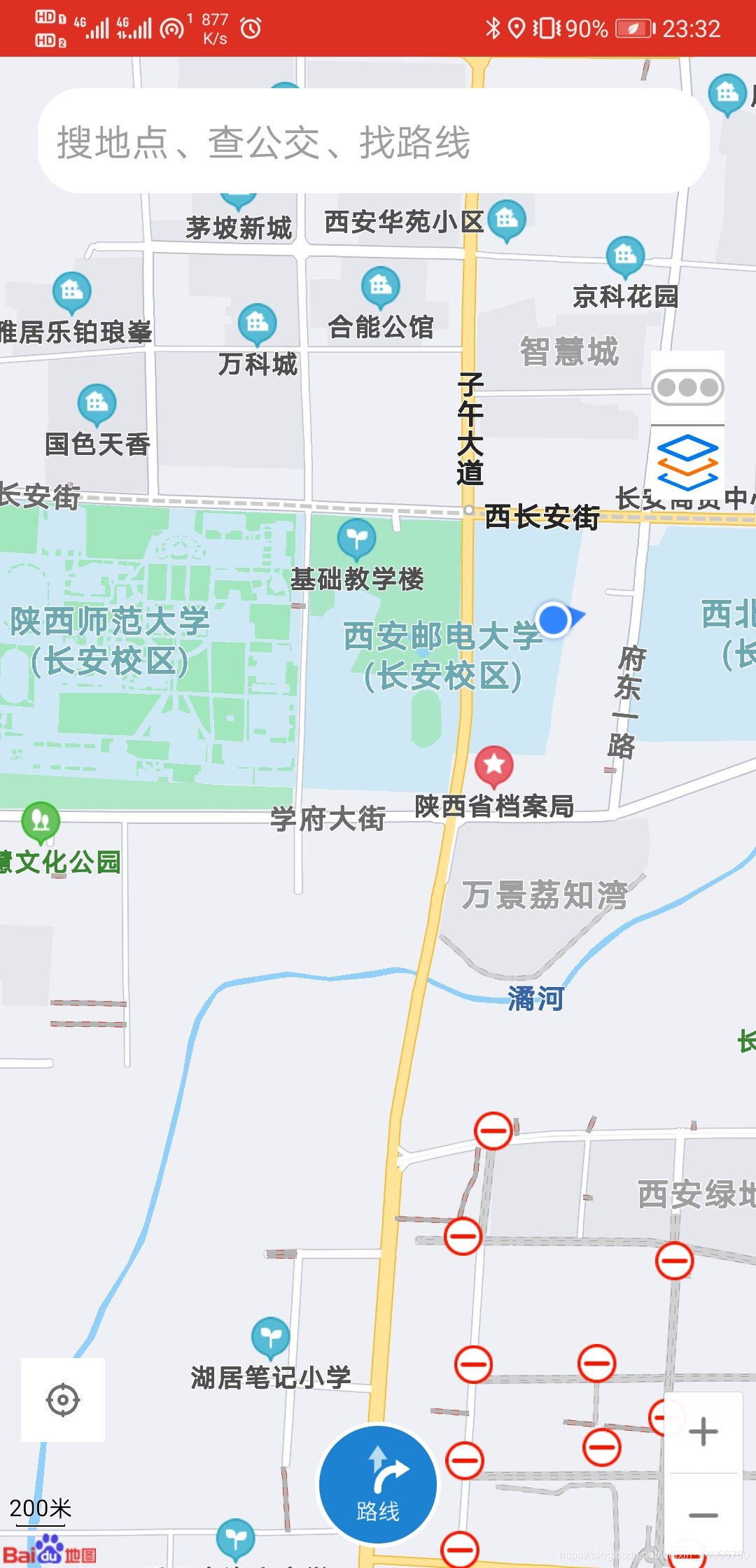
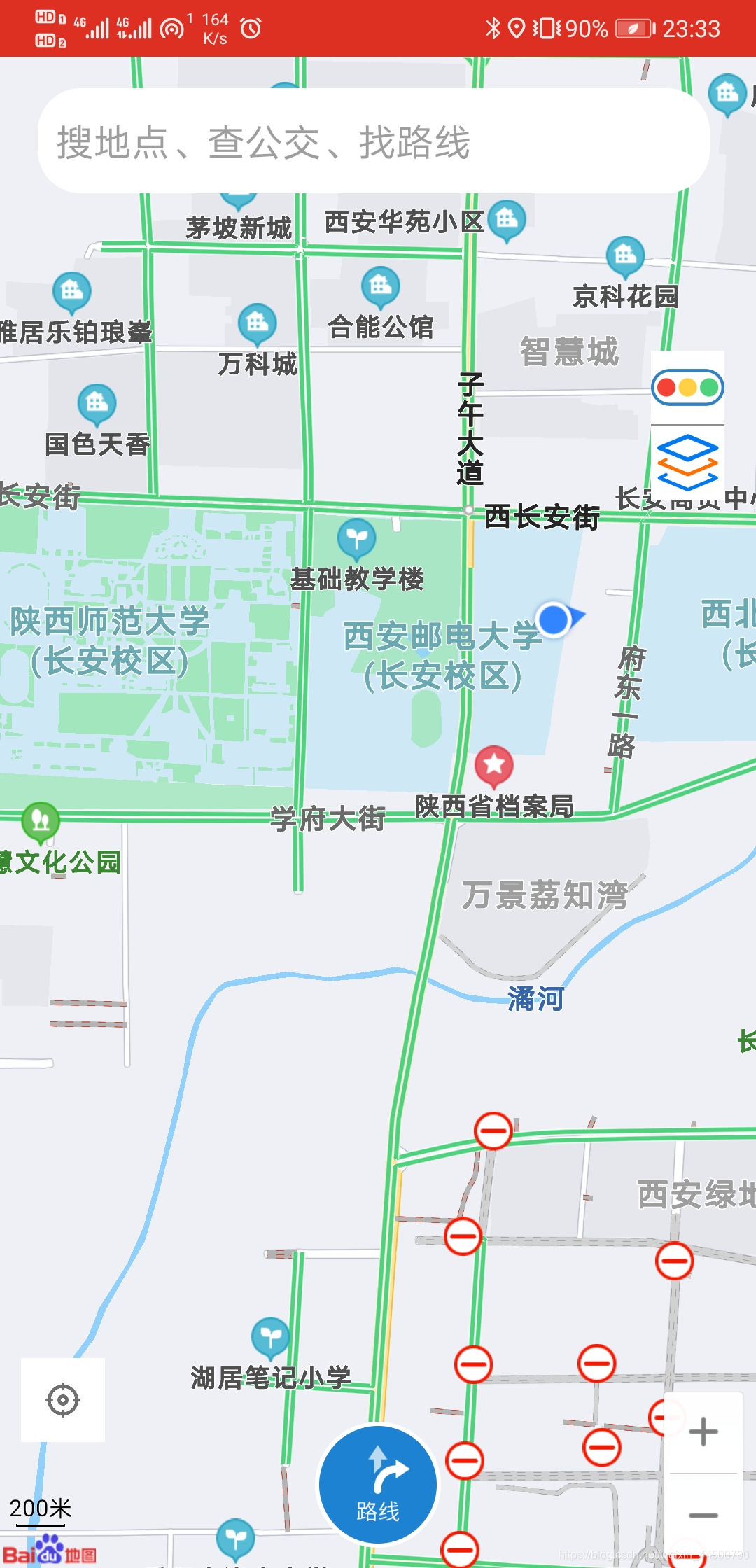
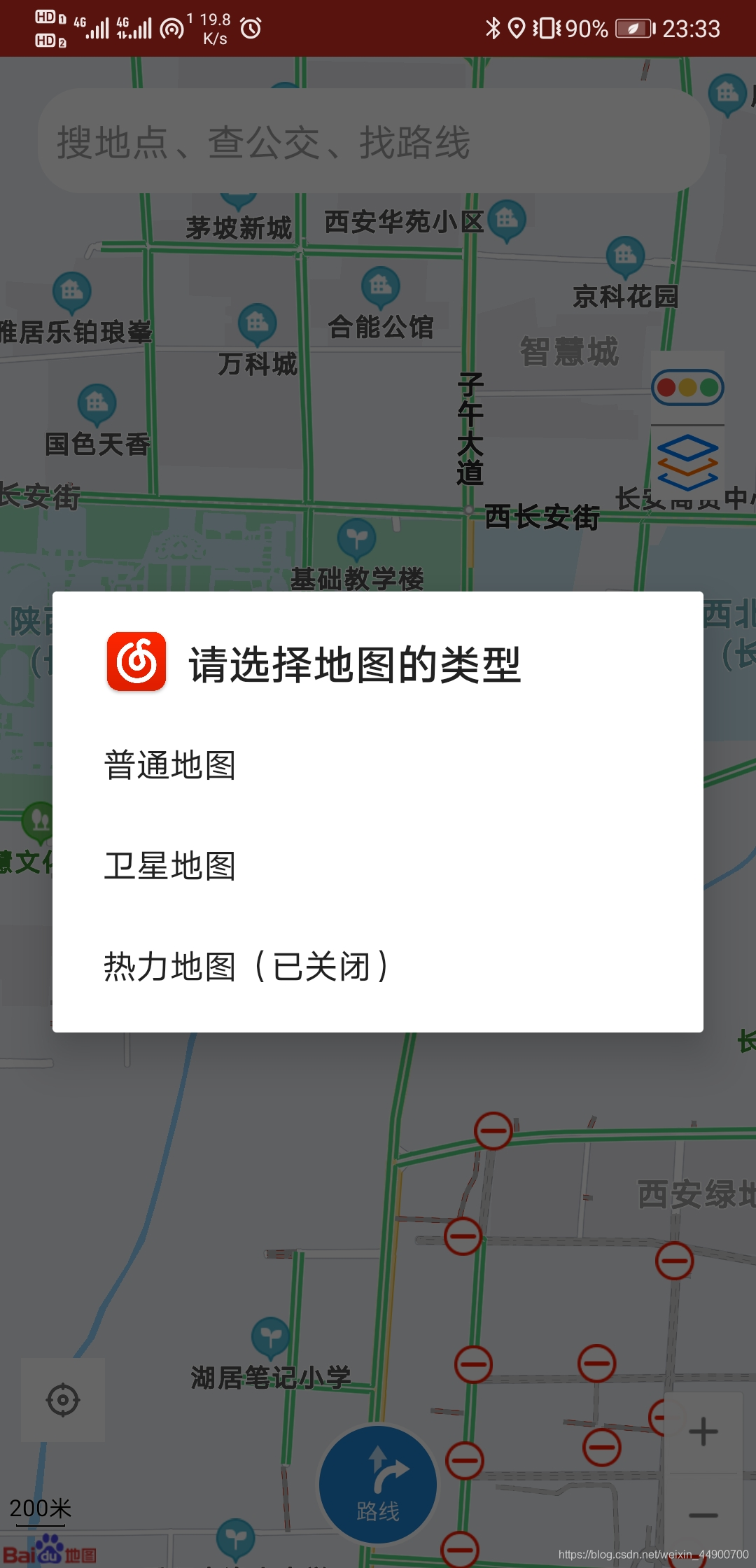
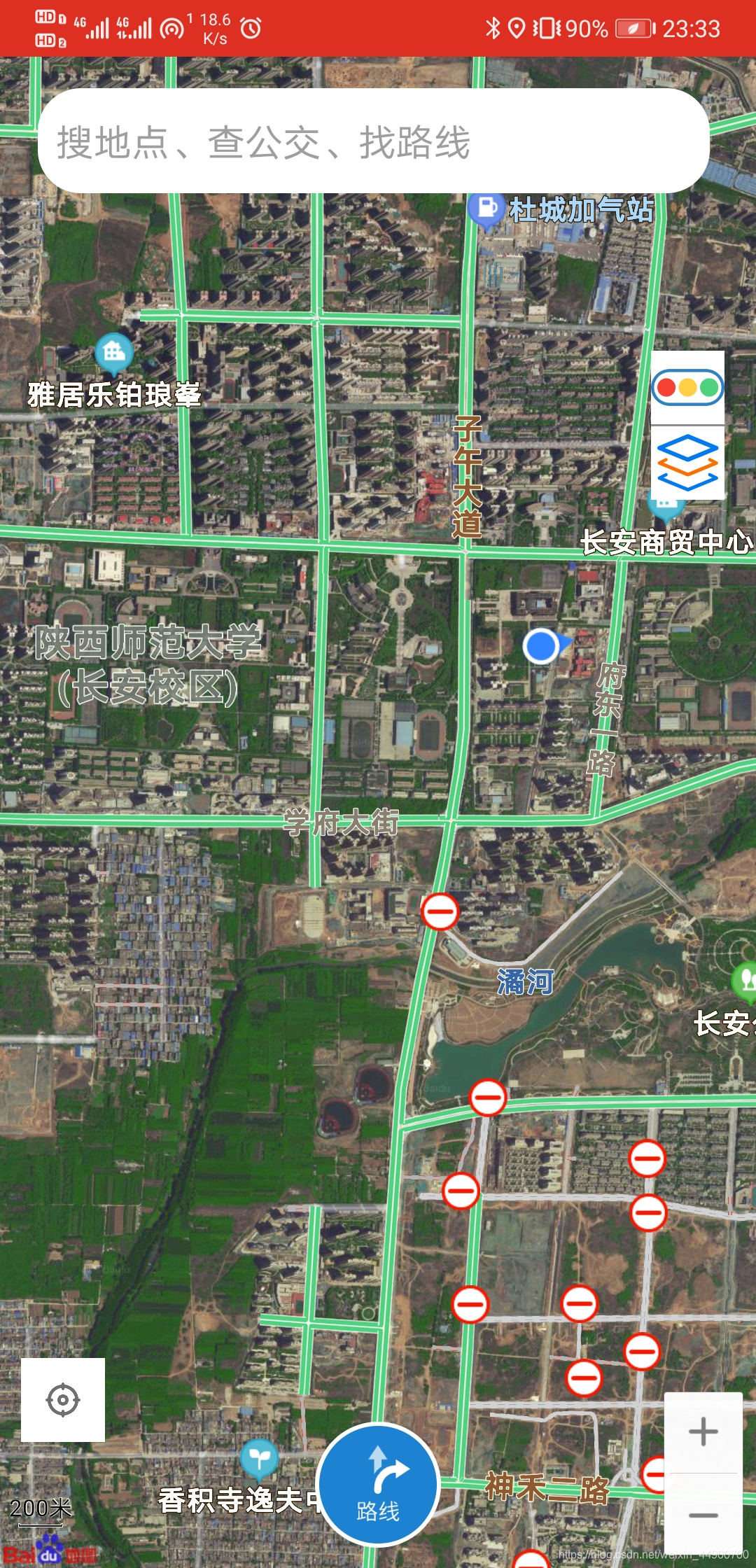
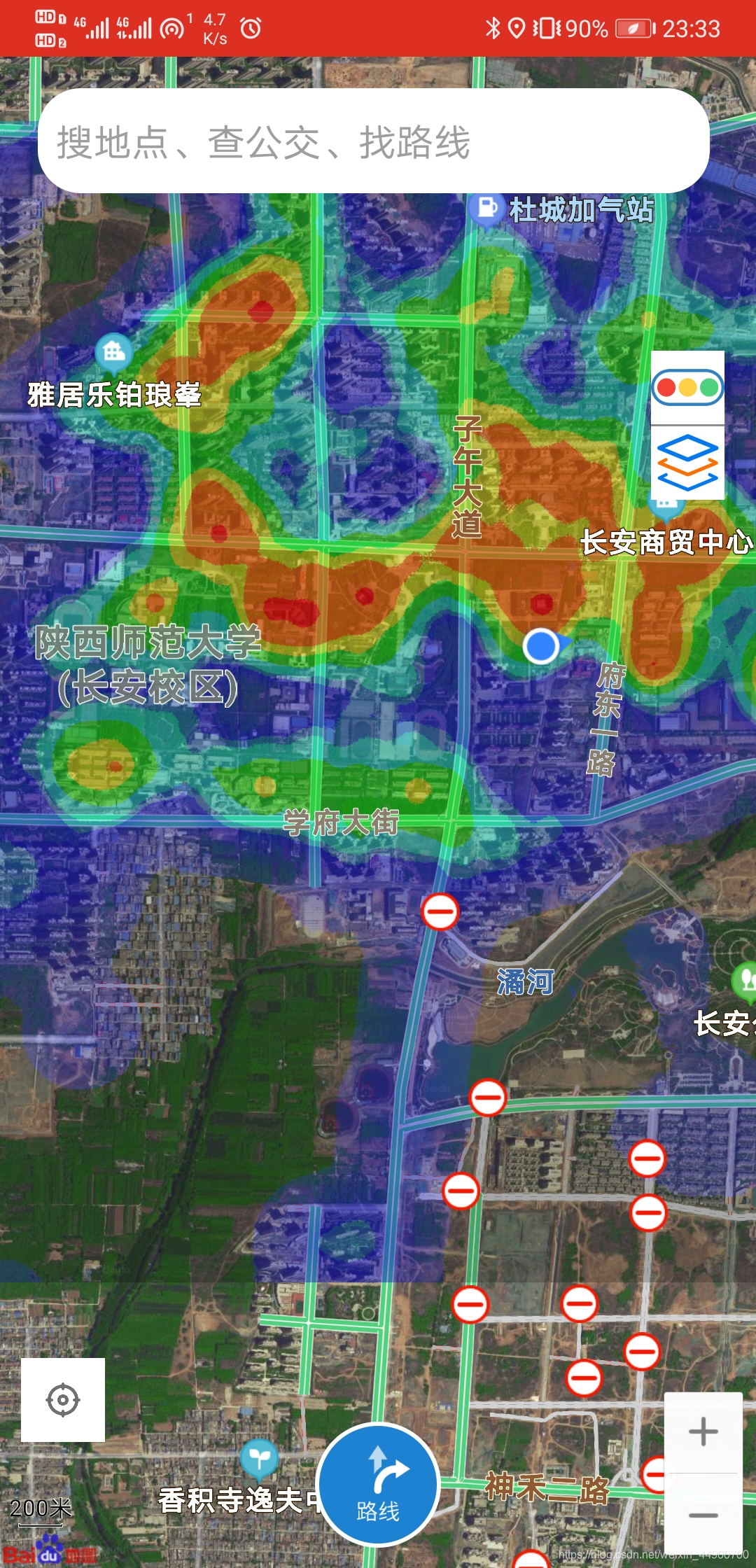
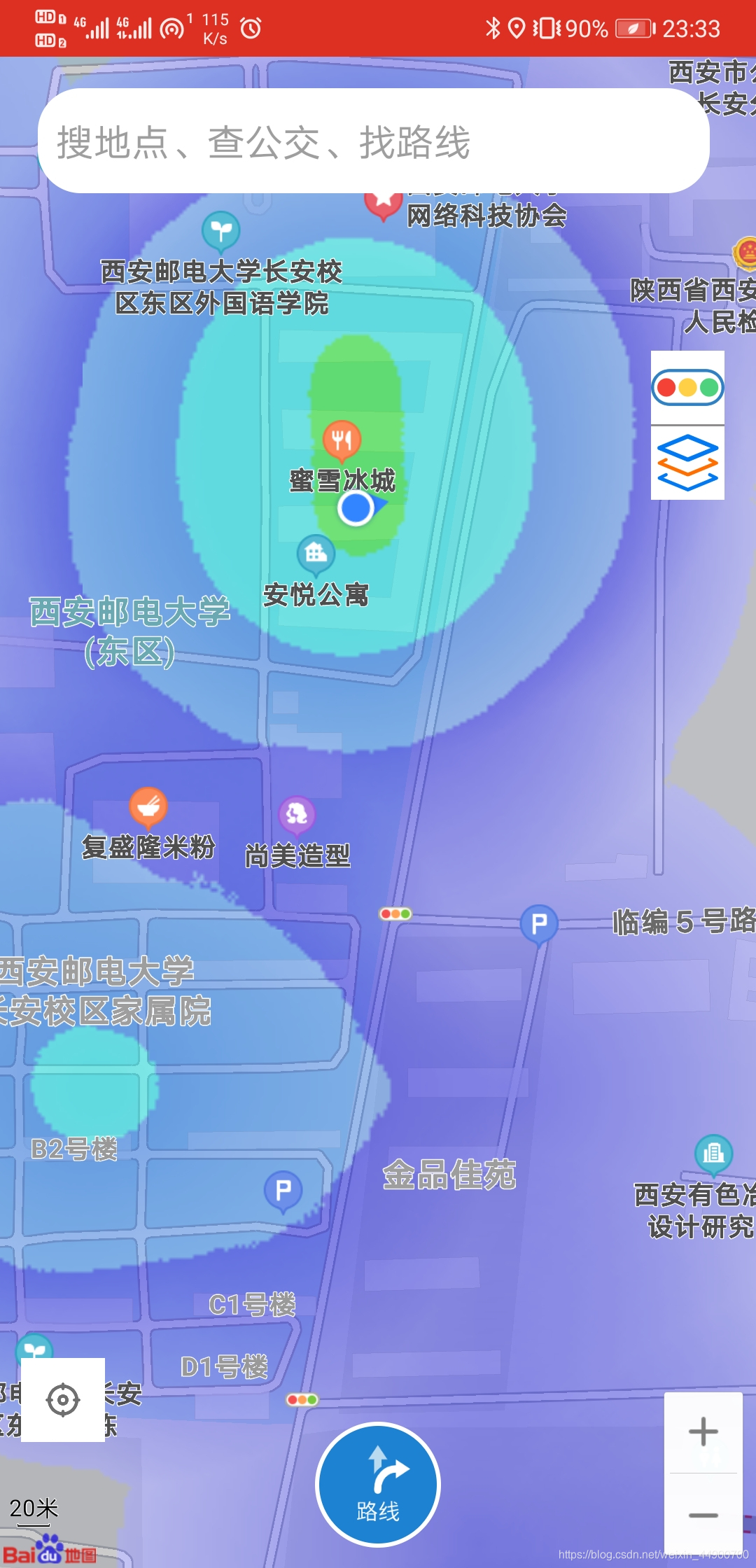
二、具体流程设计图
1. 注册登录流程图:
2. 具体功能流程图
3.添加依赖并导入百度API
- 首先,在build.gradle(:app)中添加依赖
implementation 'com.android.support:design:29.1.1'
implementation 'com.android.support:support-v4:29.1.1'
implementation 'cn.leancloud.android:avoscloud-sdk:v4.6.4'
implementation 'cn.leancloud.android:avoscloud-statistics:v4.6.4'
implementation 'cn.leancloud.android:avoscloud-feedback:v4.6.4@aar'
implementation 'cn.leancloud.android:avoscloud-sns:v4.6.4@aar'
implementation 'cn.leancloud.android:qq-sdk:1.6.1-leancloud'
implementation 'cn.leancloud.android:avoscloud-search:v4.6.4@aar'
implementation 'com.android.support:recyclerview-v7:29.1.1'
androidTestImplementation 'androidx.test:runner:1.2.0'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.2.0'
implementation 'de.hdodenhof:circleimageview:3.0.1'
implementation 'com.android.support:design:29.0.0'
4. 功能代码:
MainActivity
package com.example.trip_project;
import android.Manifest;
import android.app.AlertDialog;
import android.app.SearchManager;
import android.content.DialogInterface;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.os.Build;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.view.View;
import android.view.ViewGroup;
import android.view.Window;
import android.widget.BaseAdapter;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import androidx.core.content.ContextCompat;
import com.baidu.location.BDLocation;
import com.baidu.location.BDLocationListener;
import com.baidu.location.LocationClient;
import com.baidu.location.LocationClientOption;
import com.baidu.mapapi.SDKInitializer;
import com.baidu.mapapi.map.BaiduMap;
import com.baidu.mapapi.map.MapStatusUpdate;
import com.baidu.mapapi.map.MapStatusUpdateFactory;
import com.baidu.mapapi.map.MapView;
import com.baidu.mapapi.map.MyLocationData;
import com.baidu.mapapi.model.LatLng;
import org.apache.http.client.ClientProtocolException;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import de.hdodenhof.circleimageview.CircleImageView;
public class MainActivity extends AppCompatActivity {
private ImageView mapRoad;
private ImageView Road_tuceng;
private EditText searchEdit;
private List<SearchInfo> searchInfoLists;
private double bdLons;
private double bdLats;
private MapView mapView = null;
public LocationClient mLocationClient;
private BaiduMap myBaiduMap = null;
private TextView positionText;
private String[] types = {"普通地图", "卫星地图", "热力地图(已关闭)"};
private BaiduMap baiduMap;
private boolean isFirstLocate = true;
private CircleImageView route_Button; // 路线导航键
private ImageView MyLocation;
private String uid;
@Override
protected void onPause() {
super.onPause();
mapView.onPause();
}
@Override
protected void onPostResume() {
super.onPostResume();
mapView.onResume();
}
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
if (Build.VERSION.SDK_INT >= 21) {
Window window = getWindow();
window.setStatusBarColor(getResources().getColor(R.color.colorHead));
}
getSupportActionBar().hide();
onSearchRequested(); //显示搜索悬浮框
mapView = findViewById(R.id.bmapView);
mapRoad = findViewById(R.id.road_condition);
Road_tuceng = findViewById(R.id.road_tuceng);
searchEdit = findViewById(R.id.findmap);
myBaiduMap = mapView.getMap();
/*MapStatusUpdateFactory factory = MapStatusUpdateFactory.zoomTo(15.0f);
myBaiduMap.animateMapStatus(factory);*/
mLocationClient = new LocationClient(getApplicationContext());
mLocationClient.registerLocationListener(new MyLocationListener());
SDKInitializer.initialize(getApplicationContext());
positionText = findViewById(R.id.position_text_view);
mapView = findViewById(R.id.bmapView);
route_Button = findViewById(R.id.icon_route); //声明路线按键
MyLocation = findViewById(R.id.my_location);
baiduMap = mapView.getMap();
baiduMap.setMyLocationEnabled(true);
List<String> permissionList = new ArrayList<>();
if (ContextCompat.checkSelfPermission(MainActivity.this, Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
permissionList.add(Manifest.permission.ACCESS_FINE_LOCATION);
}
if (ContextCompat.checkSelfPermission(MainActivity.this, Manifest.permission.READ_PHONE_STATE) != PackageManager.PERMISSION_GRANTED) {
permissionList.add(Manifest.permission.READ_PHONE_STATE);
}
if (ContextCompat.checkSelfPermission(MainActivity.this, Manifest.permission.WRITE_EXTERNAL_STORAGE) != PackageManager.PERMISSION_GRANTED) {
permissionList.add(Manifest.permission.WRITE_EXTERNAL_STORAGE);
}
if (!permissionList.isEmpty()) {
String[] permissions = permissionList.toArray(new String[permissionList.size()]);
ActivityCompat.requestPermissions(MainActivity.this, permissions, 1);
} else {
requestLocation();
}
Intent intent = getIntent();
if (Intent.ACTION_SEARCH.equals(intent.getAction())) {
String query = intent.getStringExtra(SearchManager.QUERY);
//Log.v("name : "," " + query);
Toast.makeText(this, query, Toast.LENGTH_SHORT).show();
}
/*route_Button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//Intent intent = new Intent(MainActivity.this,find.class);
//startActivity(intent);
//selectMapType();
//myBaiduMap.setMapType(BaiduMap.MAP_TYPE_NORMAL);
}
});*/
MyLocation.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
getMyLatestLocation(bdLats, bdLons);
}
});
Road_tuceng.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
selectMapType();
}
});
mapRoad.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
switchRoadCondition();
}
});
initSearchDestination();
}
private void navigateTo(BDLocation location) {
if (isFirstLocate) {
LatLng ll = new LatLng(location.getLatitude(), location.getLongitude());
MapStatusUpdate update = MapStatusUpdateFactory.newLatLng(ll);
baiduMap.animateMapStatus(update);
update = MapStatusUpdateFactory.zoomTo(16f);
baiduMap.animateMapStatus(update);
isFirstLocate = false;
}
MyLocationData.Builder locationBuilder = new MyLocationData.Builder();
double x = location.getLongitude();
double y = location.getLatitude();
double z = Math.sqrt(x * x + y * y) + 0.00002 * Math.sin(y * Math.PI);
double temp = Math.atan2(y, x) + 0.000003 * Math.cos(x * Math.PI);
double bdLon = z * Math.cos(temp) + 0.0065;
double bdLat = z * Math.sin(temp) + 0.006;
locationBuilder.latitude(bdLat);
locationBuilder.longitude(bdLon);
MyLocationData locationData = locationBuilder.build();
baiduMap.setMyLocationData(locationData);
}
private void requestLocation() {
initLocation();
mLocationClient.start();
}
private void initLocation() {
LocationClientOption option = new LocationClientOption();
option.setScanSpan(3000);
option.setIsNeedAddress(true);
option.setLocationMode(LocationClientOption.LocationMode.Device_Sensors);
mLocationClient.setLocOption(option);
}
@Override
protected void onDestroy() {
super.onDestroy();
mLocationClient.stop();
mapView.onDestroy();
baiduMap.setMyLocationEnabled(false);
}
@Override
public void onRequestPermissionsResult(int requestCode, @NonNull String[] permissions, @NonNull int[] grantResults) {
switch (requestCode) {
case 1:
if (grantResults.length > 0) {
for (int result : grantResults) {
if (result != PackageManager.PERMISSION_GRANTED) {
Toast.makeText(this, "必须同意所有权限才能使用本程序", Toast.LENGTH_SHORT).show();
finish();
return;
}
}
requestLocation();
} else {
Toast.makeText(this, "发生未知错误", Toast.LENGTH_SHORT).show();
finish();
}
break;
default:
}
}
public class MyLocationListener implements BDLocationListener {
@Override
public void onReceiveLocation(BDLocation bdLocation) {
StringBuilder currentPosition = new StringBuilder();
double x = bdLocation.getLongitude();
double y = bdLocation.getLatitude();
double z = Math.sqrt(x * x + y * y) + 0.00002 * Math.sin(y * Math.PI);
double temp = Math.atan2(y, x) + 0.000003 * Math.cos(x * Math.PI);
bdLons = z * Math.cos(temp) + 0.0065;
bdLats = z * Math.sin(temp) + 0.006;
currentPosition.append("纬度:").append(bdLats).append("\n");
currentPosition.append("经度:").append(bdLons).append("\n");
currentPosition.append("国家:").append(bdLocation.getCountry()).append("\n");
currentPosition.append("省份:").append(bdLocation.getProvince()).append("\n");
currentPosition.append("市:").append(bdLocation.getCity()).append("\n");
currentPosition.append("区:").append(bdLocation.getDistrict()).append("\n");
currentPosition.append("街道:").append(bdLocation.getStreet()).append("\n");
currentPosition.append("定位方式:");
if (bdLocation.getLocType() == BDLocation.TypeGpsLocation) {
currentPosition.append("GPS");
} else if (bdLocation.getLocType() == BDLocation.TypeNetWorkLocation) {
currentPosition.append("网络");
}
positionText.setText(currentPosition);
if (bdLocation.getLocType() == BDLocation.TypeGpsLocation || bdLocation.getLocType() == BDLocation.TypeNetWorkLocation) {
navigateTo(bdLocation);
}
}
}
private void selectMapType() {
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
builder.setIcon(R.drawable.wangyi1)
.setTitle("请选择地图的类型")
.setItems(types, new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
String select = types[which];
if (select.equals("普通地图")) {
myBaiduMap.setMapType(BaiduMap.MAP_TYPE_NORMAL);
//Toast.makeText(MainActivity.this, "普通地图点击成功", Toast.LENGTH_SHORT).show();
} else if (select.equals("卫星地图")) {
myBaiduMap.setMapType(BaiduMap.MAP_TYPE_SATELLITE);
} else if (select.equals("热力地图(已关闭)") || select.equals("热力地图(已打开)")) {
if (myBaiduMap.isBaiduHeatMapEnabled()) {
myBaiduMap.setBaiduHeatMapEnabled(false);
Toast.makeText(MainActivity.this, "热力地图已关闭", Toast.LENGTH_SHORT).show();
types[which] = "热力地图(已关闭)";
} else {
myBaiduMap.setBaiduHeatMapEnabled(true);
Toast.makeText(MainActivity.this, "热力地图已打开", Toast.LENGTH_SHORT).show();
types[which] = "热力地图(已打开)";
}
}
}
}).show();
}
private void getMyLatestLocation(double lat, double lng) {
LatLng latLng = new LatLng(lat, lng);
MapStatusUpdate msu = MapStatusUpdateFactory.newLatLng(latLng);
myBaiduMap.animateMapStatus(msu);
}
private void switchRoadCondition() {
if (myBaiduMap.isTrafficEnabled()) {
myBaiduMap.setTrafficEnabled(false);
mapRoad.setImageResource(R.drawable.loadcondition1);
} else {
myBaiduMap.setTrafficEnabled(true);
mapRoad.setImageResource(R.drawable.loadcondition);
}
}
private void initSearchDestination(){
searchEdit = findViewById(R.id.findmap);
route_Button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//Intent intent = new Intent(MainActivity.this,find.class);
//startActivity(intent);
//selectMapType();
//myBaiduMap.setMapType(BaiduMap.MAP_TYPE_NORMAL);
searchInfoLists = new ArrayList<SearchInfo>();
getSearchDataFromNetWork();
}
});
}
private void getSearchDataFromNetWork(){
new Thread(new Runnable() {
@Override
public void run() {
try{
JSONObject jsonObject = HttpUtils.send(searchEdit.getText().toString(), null);
Message msg = new Message();
msg.obj = jsonObject;
msg.what = 0x1234;
handler.sendMessage(msg);
}catch(ClientProtocolException e){
e.printStackTrace();
}catch(IOException e){
e.printStackTrace();
}catch(JSONException e){
e.printStackTrace();
}
}
}).start();
}
private Handler handler = new Handler() {
public void handleMessage(android.os.Message msg) {
if (msg.what == 0x1234) {
JSONObject object = (JSONObject) msg.obj;
try {
JSONArray array = object.getJSONArray("results");
for (int i = 0; i < array.length(); i++) {
JSONObject jsonObject = array.getJSONObject(i);
String name = jsonObject.getString("name");
JSONObject object2 = jsonObject.getJSONObject("location");
double lat = object2.getDouble("lat");
double lng = object2.getDouble("lng");
String address = jsonObject.getString("address");
String streetIds = jsonObject.getString("street_id");
String uids = jsonObject.getString("uid");
SearchInfo mInfo = new SearchInfo(name, lat, lng, address, streetIds, uids);
}
} catch (JSONException e) {
e.printStackTrace();
}
}
displayInDialog();
}
private void displayInDialog(){
if(searchInfoLists != null){
AlertDialog.Builder builder = new AlertDialog.Builder(MainActivity.this);
builder.setIcon(R.drawable.wangyi1)
.setTitle("请选择你查询到的地点")
.setAdapter(new myDialogListAdapter(), new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
final SearchInfo mInfos = searchInfoLists.get(which);
uid = mInfos.getUids();
//myBaiduMap.setOnMarkerClickListener();
}
});
}
}
};
class myDialogListAdapter extends BaseAdapter{
public int getCount(){
return searchInfoLists.size();
}
public Object getItem(int position){
return getItem(position);
}
public long getItemId(int position){
return position;
}
public View getView(int position, View convertView, ViewGroup parent){
SearchInfo mSearchInfo = searchInfoLists.get(position);
View view = View.inflate(MainActivity.this , R.layout.support_simple_spinner_dropdown_item,null);
TextView desnameTv = view.findViewById(R.id.road_tuceng);
TextView addressTv = view.findViewById(R.id.my_location);
desnameTv.setText(mSearchInfo.getName());
addressTv.setText(mSearchInfo.getAddress());
return view;
}
}
}
activity_main.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/position_text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
/>
<com.baidu.mapapi.map.MapView
android:id="@+id/bmapView"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:clickable="true"
android:paddingBottom="1dp" />
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<EditText
android:id="@+id/findmap"
android:layout_width="320dp"
android:layout_height="50dp"
android:layout_marginTop="15dp"
android:background="@drawable/item_shape"
android:hint=" 搜地点、查公交、找路线"
android:theme="@style/MyEditText1"
android:layout_marginLeft="18dp"/>
</LinearLayout>
<ImageView
android:id="@+id/road_condition"
android:layout_width="35dp"
android:layout_height="35dp"
android:layout_alignParentRight="true"
android:layout_marginTop="140dp"
android:layout_marginEnd="15dp"
android:layout_marginRight="60dp"
android:layout_marginBottom="60dp"
android:background="#fff"
android:src="@drawable/loadcondition1" />
<ImageView
android:layout_width="35dp"
android:layout_height="1dp"
android:layout_alignParentRight="true"
android:layout_marginTop="175dp"
android:layout_marginEnd="15dp"
android:layout_marginRight="60dp"
android:layout_marginBottom="60dp"
android:background="#8E8C8C"/>
<ImageView
android:id="@+id/road_tuceng"
android:layout_width="35dp"
android:layout_height="35dp"
android:layout_alignParentRight="true"
android:layout_marginTop="176dp"
android:layout_marginEnd="15dp"
android:layout_marginRight="60dp"
android:layout_marginBottom="60dp"
android:background="#fff"
android:src="@drawable/tuceng1" />
<ImageView
android:id="@+id/my_location"
android:layout_width="40dp"
android:layout_height="40dp"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_marginBottom="60dp"
android:layout_marginLeft="10dp"
android:background="#FFF"
android:padding="10dp"
android:src="@drawable/main_icon_location" />
<de.hdodenhof.circleimageview.CircleImageView
xmlns:CircleimageView="http://schemas.android.com/apk/res-auto"
android:id="@+id/icon_route"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_centerHorizontal="true"
android:layout_marginTop="650dp"
android:src="@drawable/route1"
CircleimageView:civ_border_width="2dp"
CircleimageView:civ_border_color="#fff" />
</RelativeLayout>
Login.class:
package com.example.trip_project;
import android.content.Intent;
import android.os.Build;
import android.os.Bundle;
import android.view.View;
import android.view.Window;
import android.widget.Button;
import androidx.appcompat.app.AppCompatActivity;
public class Login extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
getSupportActionBar().hide();
if(Build.VERSION.SDK_INT >= 21){
Window window = getWindow();
window.setStatusBarColor(getResources().getColor(R.color.colorLogin));
}
Button login = findViewById(R.id.login);
login.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(Login.this,Login2.class);
startActivity(intent);
}
});
Button login2 = findViewById(R.id.login2);
login2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//Toast.makeText(Login.this, "游客登录", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(Login.this,MainActivity.class);
startActivity(intent);
}
});
}
}
activity_login.xml:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.trip_project.Login">
<ImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_alignParentStart="true"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:background="#DD2F22"
android:scaleType="centerCrop"
android:src="#E70214" />
<ImageView
android:layout_width="wrap_content"
android:layout_height="120dp"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="80dp"
android:background="#E70214"
android:src="@drawable/wangyilogin3" />
<Button
android:id="@+id/login"
android:layout_width="250dp"
android:layout_height="40dp"
android:layout_centerHorizontal="true"
android:layout_marginTop="500dp"
android:background="@drawable/item_shape"
android:text="用户登录"
android:textColor="#E70214" />
<Button
android:id="@+id/login2"
android:layout_width="250dp"
android:layout_height="40dp"
android:layout_centerHorizontal="true"
android:layout_marginTop="560dp"
android:background="@drawable/item_shapes"
android:text="游客登录"
android:textColor="#fff" />
<TextView
android:id="@+id/tv_copyright"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="20dp"
android:layout_marginTop="10dp"
android:text="已同意《用户协议》《隐私政策》《儿童隐私政策》"
android:textSize="10sp"
android:textColor="#fff"/>
</RelativeLayout>
Login2.class:
package com.example.trip_project;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Build;
import android.os.Bundle;
import android.view.View;
import android.view.Window;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import com.avos.avoscloud.AVException;
import com.avos.avoscloud.AVUser;
import com.avos.avoscloud.LogInCallback;
public class Login2 extends AppCompatActivity {
private EditText name;
private EditText password;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login2);
if(Build.VERSION.SDK_INT >= 21 ){
Window window = getWindow();
window.setStatusBarColor(getResources().getColor(R.color.colorHead));
}
getSupportActionBar().hide();
Button login = findViewById(R.id.yonghu_login);
name = findViewById(R.id.yonghuming);
password = findViewById(R.id.mima);
login.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//Toast.makeText(Login2.this, "登录成功", Toast.LENGTH_SHORT).show();
if(!name.getText().toString().equals("")&&!password.getText().toString().equals("")){
AVUser.logInInBackground(name.getText().toString(), password.getText().toString(), new LogInCallback<AVUser>() {
@Override
public void done(AVUser avUser, AVException e) {
if(e==null){
startActivity(new Intent(Login2.this,MainActivity.class));
finish();
}else{
Toast.makeText(Login2.this, "用户名或密码错误", Toast.LENGTH_SHORT).show();
}
}
});
}else{
if(name.getText().toString().equals("")&&password.getText().toString().equals("")){
Toast.makeText(Login2.this, "请输入用户名和用户密码", Toast.LENGTH_SHORT).show();
}else if(name.getText().toString().equals("")&&!password.getText().toString().equals("")){
Toast.makeText(Login2.this, "请输入用户名", Toast.LENGTH_SHORT).show();
}else if(!name.getText().toString().equals("")&&password.getText().toString().equals("")){
Toast.makeText(Login2.this, "请输入用户密码" , Toast.LENGTH_SHORT).show();
}
}
}
});
Button register = findViewById(R.id.register);
register.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
//Toast.makeText(Login2.this, "注册成功", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(Login2.this,Register.class);
startActivity(intent);
}
});
}
}
activity_login2.xml:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.trip_project.Login2">
<ImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_alignParentStart="true"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:background="#DD2F22"
android:scaleType="centerCrop"
android:src="#E70214" />
<ImageView
android:layout_width="wrap_content"
android:layout_height="120dp"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="80dp"
android:background="#E70214"
android:src="@drawable/wangyilogin3" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center"
android:layout_marginTop="300dp">
<EditText
android:id="@+id/yonghuming"
android:layout_width="280dp"
android:layout_height="wrap_content"
android:hint="用户名/手机号/邮箱:"
android:textColorHint="#ffffff"
android:theme="@style/MyEditText"
android:textColor="#ffffff"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center"
android:layout_marginTop="350dp">
<EditText
android:id="@+id/mima"
android:layout_width="280dp"
android:layout_height="wrap_content"
android:hint="密码:"
android:textColorHint="#ffffff"
android:theme="@style/MyEditText"
android:textColor="#ffffff"/>
</LinearLayout>
<Button
android:id="@+id/yonghu_login"
android:layout_width="250dp"
android:layout_height="40dp"
android:layout_centerHorizontal="true"
android:layout_marginTop="500dp"
android:background="@drawable/item_shape"
android:text="用户登录"
android:textColor="#E70214" />
<Button
android:id="@+id/register"
android:layout_width="250dp"
android:layout_height="40dp"
android:layout_centerHorizontal="true"
android:layout_marginTop="560dp"
android:background="@drawable/item_shapes"
android:text="用户注册"
android:textColor="#fff" />
</RelativeLayout>
BaseApplication.class:
package com.example.trip_project;
import android.app.Application;
import android.content.Context;
import android.widget.Toast;
import com.avos.avoscloud.AVOSCloud;
import com.baidu.lbsapi.BMapManager;
import com.baidu.lbsapi.MKGeneralListener;
import com.baidu.mapapi.SDKInitializer;
public class BaseApplication extends Application {
private static Context mContext;
public static float sScale;
public static int sWidthDp;
public static int sWidthPix;
public BMapManager mBMapManager = null;
private static BaseApplication mInstance;
public static BaseApplication getInstance() {
return mInstance;
}
@Override
public void onCreate() {
super.onCreate();
AVOSCloud.initialize(this,"ezYoU03wEEqtam3FCFKrxGK5-gzGzoHsz","eQAigVL7kORWY6eAiGDrzlqI");
//SDKInitializer.initialize(this);
SDKInitializer.initialize(this);
initEngineManager(this);
mContext = this;
mInstance = this;
sScale = getResources().getDisplayMetrics().density;
sWidthPix = getResources().getDisplayMetrics().widthPixels;
sWidthDp = (int) (sWidthPix / sScale);
}
public void initEngineManager(Context context) {
if (mBMapManager == null) {
mBMapManager = new BMapManager(context);
}
if (!mBMapManager.init(new MyGeneralListener())) {
Toast.makeText(
BaseApplication.getInstance().getApplicationContext(),
"BMapManager!", Toast.LENGTH_LONG).show();
}
}
public static class MyGeneralListener implements MKGeneralListener {
@Override
public void onGetPermissionState(int iError) {
if (iError != 0) {
} else {
}
}
}
}
HttpUtils.class:
package com.example.trip_project;
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.NameValuePair;
import org.apache.http.client.ClientProtocolException;
import org.apache.http.client.HttpClient;
import org.apache.http.client.entity.UrlEncodedFormEntity;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.impl.client.DefaultHttpClient;
import org.apache.http.protocol.HTTP;
import org.apache.http.util.EntityUtils;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import java.util.List;
/**
* @author mikyou
* 封装get和post方式请求
* HttpClient 维护session
* 一个HttpClient对象代表一个浏览器
* 高度封装了客户端与服务端交互的,可以直接返回一个JSONObject对象
* */
public class HttpUtils {
private static JSONObject jsonObjects;
private static String entityString;
private static HttpResponse res=null;
private static String APPKEY="vC9Iool2QwGnmvrDrZWqkdGCa6S6nWKj";//APPKEY
private static String outputForm="json";//返回网络数据的格式
private static String Head="http://api.map.baidu.com/place/v2/search?ak=";//URL接口地址首部
private static String IP=null;
private static int pageSize=20;//一次返回数据总条数
private static String mcode="EA:A0:5E:3E:8A:55:D4:01:9C:6A:27:FF:2D:80:54:B0:46:CB:2E:F5;com.example.trip_project";//mcode参数
private static HttpClient clients=new DefaultHttpClient();
@SuppressWarnings("unused")
public static JSONObject send(String destination, List<NameValuePair> params) throws ClientProtocolException, IOException, JSONException {
if (params==null) {//表示发送get方式请求,否则就发送Post发送请求(因为get方式不需要params)
IP=Head+APPKEY+"&output="+outputForm+"&query="+destination+"&page_size="+pageSize+"&page_num=0&scope=1®ion=全国&mcode="+mcode;
System.out.println("IP_Address:--------->"+IP);
HttpGet get=new HttpGet(IP);
res=clients.execute(get);
}else{
HttpPost post=new HttpPost(IP);
post.setEntity(new UrlEncodedFormEntity(params, HTTP.UTF_8));
res=clients.execute(post);
}
if (res.getStatusLine().getStatusCode()==200) {
HttpEntity entity=res.getEntity();
entityString= EntityUtils.toString(entity,HTTP.UTF_8);
System.out.println("httpUtils--------------->"+entityString);
jsonObjects=new JSONObject(entityString);
}
return jsonObjects;
}
public static String getEntityString() {
return entityString;
}
public static void setEntityString(String entityString) {
HttpUtils.entityString = entityString;
}
}
Register.class:
package com.example.trip_project;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Build;
import android.os.Bundle;
import android.view.View;
import android.view.Window;
import android.widget.Button;
import android.widget.EditText;
import android.widget.Toast;
import com.avos.avoscloud.AVException;
import com.avos.avoscloud.AVUser;
import com.avos.avoscloud.SignUpCallback;
public class Register extends AppCompatActivity implements View.OnClickListener{
private Button register1,cancel;
private EditText name,email,password,confirmation;
@Override
protected void onCreate(Bundle savedInstanceState){
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_register);
getSupportActionBar().hide();
if(Build.VERSION.SDK_INT >= 21){
Window window = getWindow();
window.setStatusBarColor(getResources().getColor(R.color.colorLogin));
}
register1 = findViewById(R.id.user_register);
cancel = findViewById(R.id.cancel);
name = findViewById(R.id.registername);
email = findViewById(R.id.registeremail);
password = findViewById(R.id.registerpassword);
confirmation = findViewById(R.id.confirmation);
register1.setOnClickListener(this);
cancel.setOnClickListener(this);
}
@Override
public void onClick(View v) {
switch(v.getId()){
case R.id.user_register:
String str1 = password.getText().toString();
String str2 = confirmation.getText().toString();
String str3 = name.getText().toString();
String str4 = email.getText().toString();
if(str1.equals("")&&str2.equals("")&&str3.equals("")&&str4.equals("")){
Toast.makeText(this, "请输入用户信息", Toast.LENGTH_SHORT).show();
}else if(str1.equals("")||str2.equals("")||str3.equals("")||str4.equals("")){
if(str3.equals("")){
Toast.makeText(this, "请输入用户名", Toast.LENGTH_SHORT).show();
}
else if(str4.equals("")){
Toast.makeText(this, "请输入邮箱地址", Toast.LENGTH_SHORT).show();
}
else if(str1.equals("")){
Toast.makeText(this, "请输入密码", Toast.LENGTH_SHORT).show();
}
else if(str2.equals("")){
Toast.makeText(this, "请确认密码", Toast.LENGTH_SHORT).show();
}
}else if (str1.equals(str2)&&!str1.equals("")&&!str3.equals("")&&!str4.equals("")){
//Toast.makeText(this, "注册成功", Toast.LENGTH_SHORT).show();
AVUser user = new AVUser();
user.setUsername(name.getText().toString());
user.setEmail(email.getText().toString());
user.setPassword(password.getText().toString());
user.signUpInBackground(new SignUpCallback() {
@Override
public void done(AVException e) {
if(e==null){
startActivity(new Intent(Register.this,Login2.class));
finish();
}else{
Toast.makeText(Register.this, "邮箱规格不符", Toast.LENGTH_SHORT).show();
}
}
});
}else{
Toast.makeText(this, "两次密码输入不一致,请重新输入", Toast.LENGTH_SHORT).show();
}
break;
case R.id.cancel:
finish();
break;
}
}
}
activity_register.xml:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.trip_project.Register">
<ImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_alignParentStart="true"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:background="#DD2F22"
android:scaleType="centerCrop"
android:src="#E70214" />
<ImageView
android:layout_width="wrap_content"
android:layout_height="120dp"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="80dp"
android:background="#E70214"
android:src="@drawable/wangyilogin3" />
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center"
android:layout_marginTop="230dp">
<EditText
android:id="@+id/registername"
android:layout_width="250dp"
android:layout_height="wrap_content"
android:hint="用户名:"
android:textColorHint="#ffffff"
android:theme="@style/MyEditText"
android:textColor="#ffffff"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center"
android:layout_marginTop="290dp">
<EditText
android:id="@+id/registeremail"
android:layout_width="250dp"
android:layout_height="wrap_content"
android:hint="邮箱:"
android:textColorHint="#ffffff"
android:theme="@style/MyEditText"
android:textColor="#ffffff"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center"
android:layout_marginTop="350dp">
<EditText
android:id="@+id/registerpassword"
android:layout_width="250dp"
android:layout_height="wrap_content"
android:hint="密码:"
android:textColorHint="#ffffff"
android:theme="@style/MyEditText"
android:textColor="#ffffff"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:gravity="center"
android:layout_marginTop="410dp">
<EditText
android:id="@+id/confirmation"
android:layout_width="250dp"
android:layout_height="wrap_content"
android:hint="确认密码:"
android:textColorHint="#ffffff"
android:theme="@style/MyEditText"
android:textColor="#ffffff"/>
</LinearLayout>
<Button
android:id="@+id/user_register"
android:layout_width="250dp"
android:layout_height="40dp"
android:layout_centerHorizontal="true"
android:layout_marginTop="500dp"
android:background="@drawable/item_shape"
android:text="注册账户"
android:textColor="#E70214" />
<Button
android:id="@+id/cancel"
android:layout_width="250dp"
android:layout_height="40dp"
android:layout_centerHorizontal="true"
android:layout_marginTop="560dp"
android:background="@drawable/item_shapes"
android:text="取消"
android:textColor="#fff" />
</RelativeLayout>
activity_splash.xml:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.trip_project.SplashActivity">
<ImageView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_alignParentStart="true"
android:layout_alignParentLeft="true"
android:layout_alignParentTop="true"
android:background="#DD2F22"
android:scaleType="centerCrop"
android:src="#DD2F22" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="130dp"
android:text="Travel Planing"
android:textColor="#fff"
android:textSize="40dp"
android:textStyle="italic" />
<LinearLayout
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:layout_above="@+id/tv_copyright"
android:layout_centerHorizontal="true">
<ImageView
android:id="@+id/music"
android:layout_width="35dp"
android:layout_height="35dp"
android:layout_marginRight="-1dp"
android:layout_toLeftOf="@+id/textView"
android:src="@drawable/wangyi1" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@+id/tv_copyright"
android:layout_centerHorizontal="true"
android:src="#DD2F22"
android:text="网易出行"
android:textSize="20dp"
android:textColor="#fff"/>
</LinearLayout>
<TextView
android:id="@+id/tv_copyright"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_centerHorizontal="true"
android:layout_marginBottom="20dp"
android:layout_marginTop="10dp"
android:text="Copyright 2021 Ixuea. All Rights Reserved 黑衣侠客" />
</RelativeLayout>