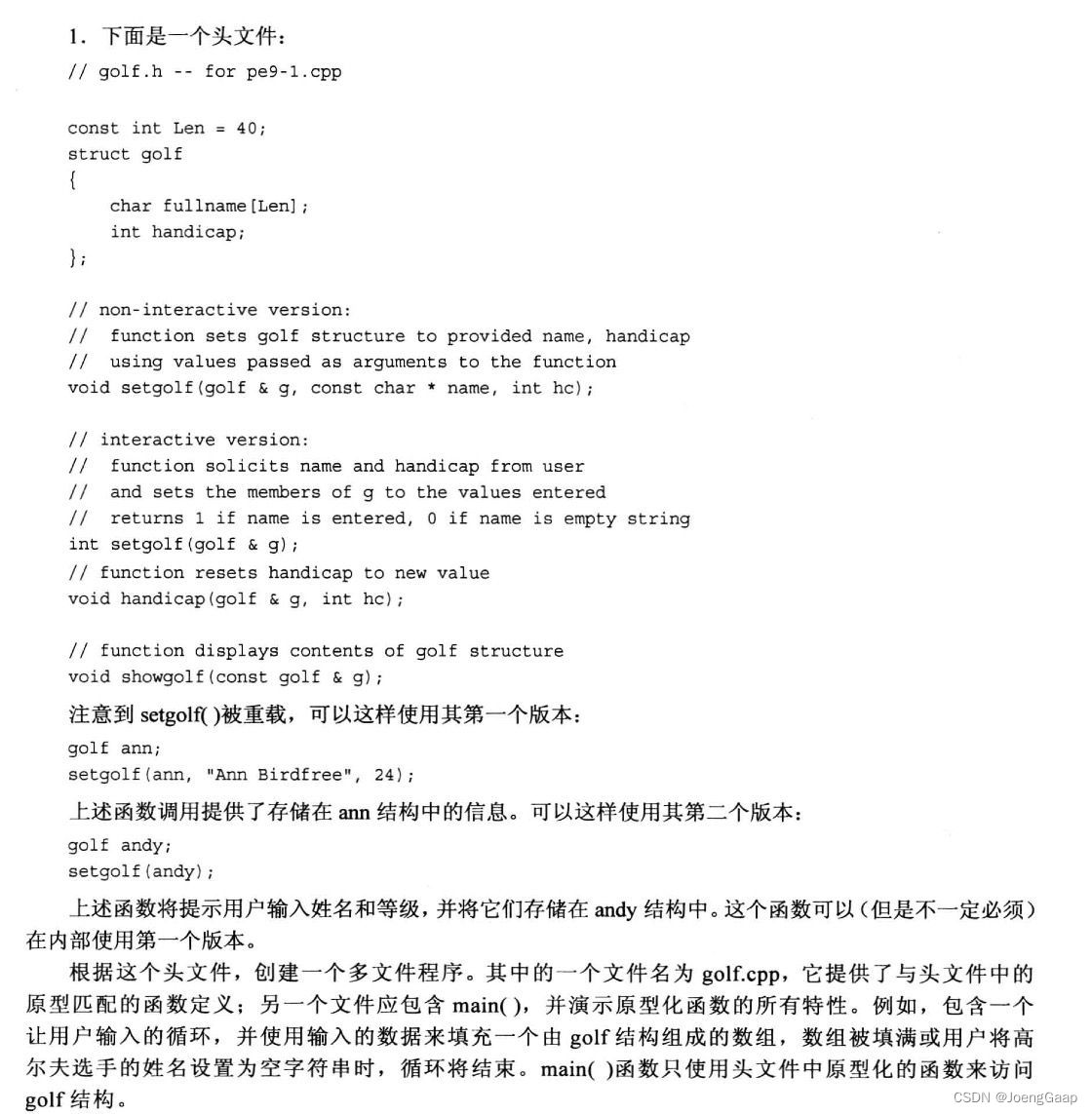
#ifndef GOLF_H_
#define GOLF_H_
const int Len = 40;
struct golf
{
char fullname[Len];
int handicap;
};
void setgolf(golf& g, const char* name, int hc);
int setgolf(golf& g);
void handicap(golf& g, int hc);
void showgolf(const golf& g);
#endif
#include "golf.h"
#include<cstring>
#include<iostream>
void setgolf(golf& g, const char* name, int hc)
{
strncpy(g.fullname, name, Len);
g.fullname[Len - 1] = '\0';
g.handicap = hc;
}
int setgolf(golf& g)
{
using namespace std;
cout << "Please enter the fullname:";
cin.getline(g.fullname, Len);
if (0 == strcmp(g.fullname, "\0"))
{
return 0;
}
cout << "Please enter the handicap: ";
while (!(cin >> g.handicap))
{
cin.clear();
while (cin.get() != '\n')
continue;
cout << "Please enter an number: ";
}
cin.get();
return 1;
}
void handicap(golf& g, int hc)
{
g.handicap = hc;
}
void showgolf(const golf& g)
{
using namespace std;
cout << "fullname:" << g.fullname<<endl;
cout << "handicap:" << g.handicap;
}
#include <iostream>
#include "golf.h"
int main()
{
using namespace std;
int i;
int sum = 0;
golf andy[Len];
setgolf(andy[0], "Ann Birdfree", 24);
cout << "Starting output:" << endl;
showgolf(andy[0]);
handicap(andy[0], 666);
cout << "Changing handicap:" << endl;
showgolf(andy[0]);
cout.put('\n');
for (i = 0; i < Len; i++)
{
cout << "Please enter andy #" << i + 1 << ": " << endl;
if (0 == setgolf(andy[i]))
{
break;
}
++sum;
}
if (sum > 0)
{
cout << "Ending output:" << endl;
for (i = 0; i < sum; i++)
{
showgolf(andy[i]);
}
}
cout << "Bye." << endl;
return 0;
}

#include<iostream>
#include<string>
using namespace std;
void strcount(string &str);
int main()
{
string input;
cout << "Enter a line:\n";
while (getline(cin, input), input != "")
{
strcount(input);
cout << "Enter next line (empty line to quit):\n";
}
cout << "Bye\n";
return 0;
}
void strcount(string& str)
{
using namespace std;
static int total = 0;
int count = 0;
cout << "\"" << str << "\"contains";
count = str.size();
total += count;
cout << count << "characters\n";
cout << total << "characters total\n";
}
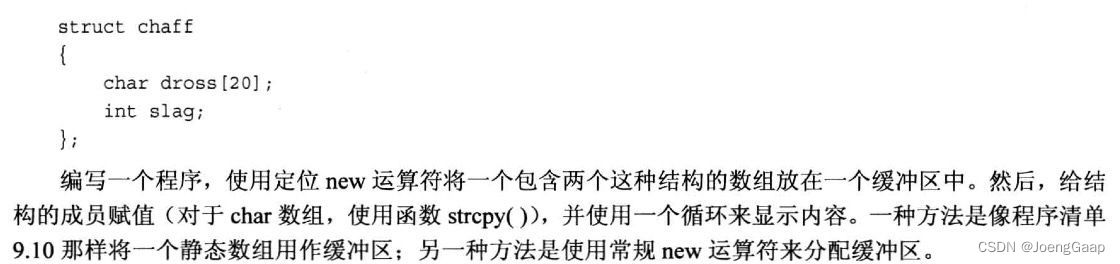
#include<iostream>
#include<new>
#include<cstring>
using namespace std;
const int BUF = 512;
const int N = 5;
char buffer[BUF];
struct chaff
{
char dross[20];
int slag;
};
int main()
{
char temp[20];
chaff* pd1;
pd1 = new(buffer) chaff[2];
for (int i = 0; i < 2; i++)
{
cout << "Enter dross:";
cin.getline(temp,20);
strcpy_s(pd1[i].dross, temp);
cout << "Enter slag:";
while (!(cin >> pd1[i].slag))
{
cin.clear();
while (cin.get() != '\n')
continue;
cout << "Please enter a number: ";
}
cin.get();
}
for (int i = 0; i < 2; i++)
{
cout << "adress:" << &pd1[i] << endl;
cout << "dross:" << pd1[i].dross << endl;
cout << "slag:" << pd1[i].slag << endl;
}
}
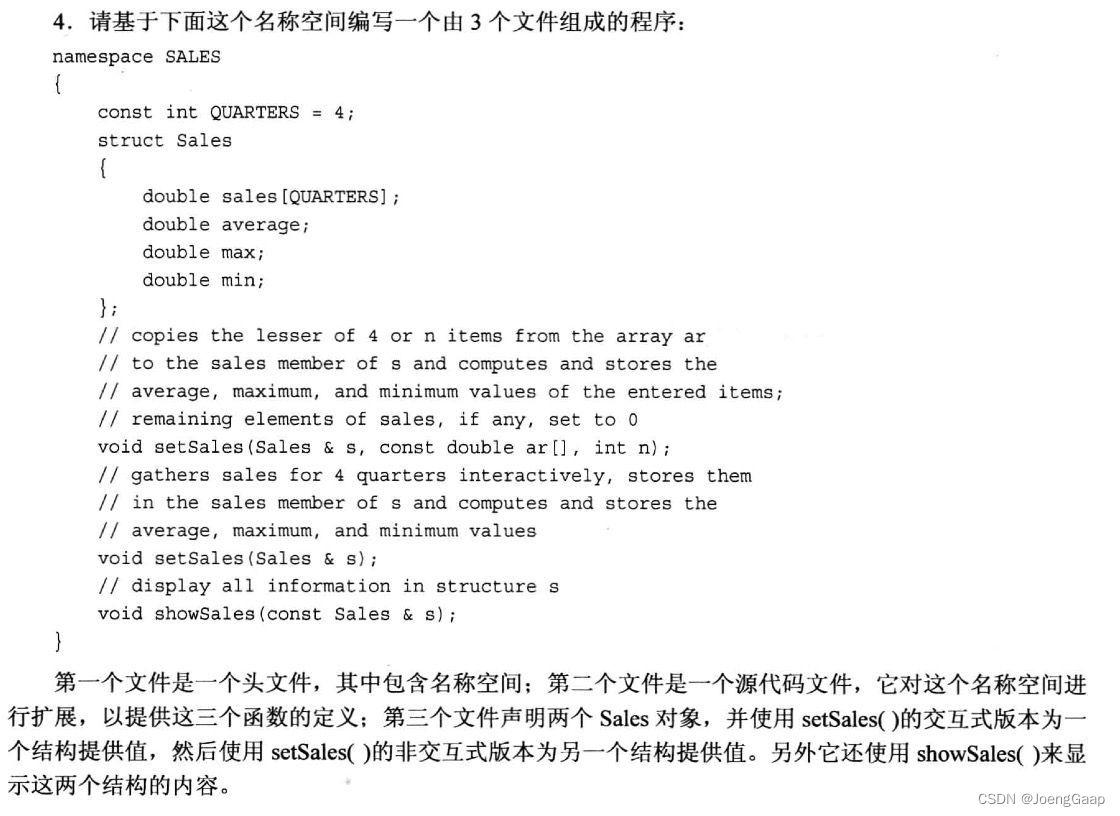
#ifndef Name_H_
#define Name_H_
namespace SALES
{
const int QUARTERS = 4;
struct Sales
{
double sales[QUARTERS];
double average;
double max;
double min;
};
void setSales(Sales& s, const double ar[], int n);
void setSales(Sales& s);
void showSales(const Sales& s);
}
#endif
#include"Name.h"
#include<iostream>
#include<cstring>
using namespace std;
namespace SALES
{
void setSales(Sales& s, const double ar[], int n)
{
double sum = 0;
double min = ar[0];
double max = ar[0];
for (int i = 0; i < n; i++)
{
s.sales[i] = ar[i];
if (ar[i] < min)
min = ar[i];
if (ar[i] > max)
max = ar[i];
sum += ar[i];
}
s.average = sum / n;
s.max = max;
s.min = min;
}
void setSales(Sales& s)
{
double sum = 0;
double min = 100000;
double max = 0;
for (int i = 0; i < 4; i++)
{
cout << "Enter Sale:";
cin >> s.sales[i];
sum += s.sales[i];
if (s.sales[i] < min)
min = s.sales[i];
if (s.sales[i] > max)
max = s.sales[i];
}
s.average = sum / 4;
s.max = max;
s.min = min;
}
void showSales(const Sales& s)
{
for (int i = 0; i < 4; i++)
{
cout << "Sales:" << s.sales[i] << endl;
}
cout << "average:" << s.average<<endl;
cout << "min:" << s.min<<endl;
cout << "max:" << s.max << endl;
}
}
#include"Name.h"
int main()
{
SALES::Sales objects[2];
double tempt[4] = { 2.1,2.3,4.3,4 };
SALES::setSales(objects[0],tempt, 4);
SALES::showSales(objects[0]);
SALES::setSales(objects[1]);
SALES::showSales(objects[1]);
return 0;
}