汽车租赁系统(初识面向对象):
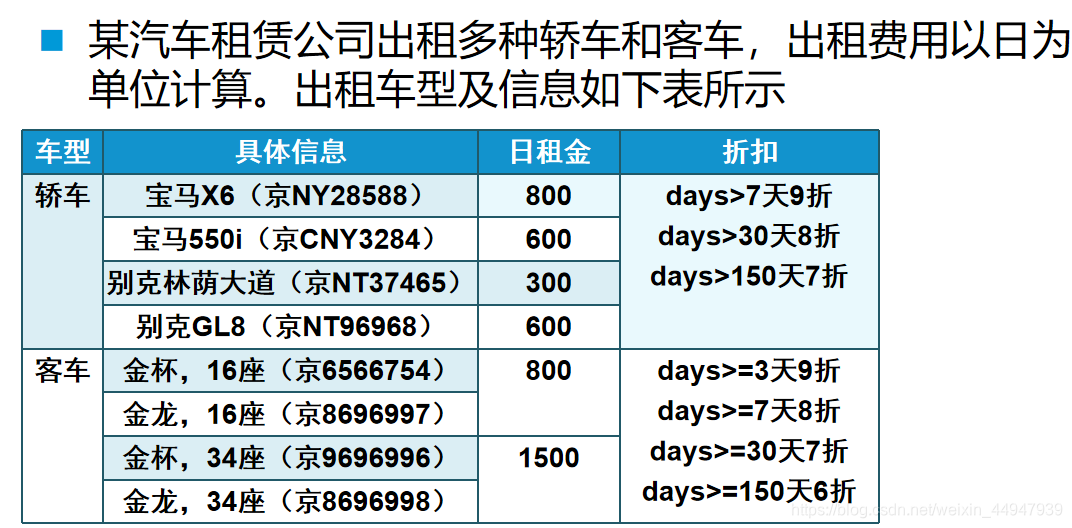
1.父类:
package project.cartwo;
public class Car {
public int serial;
public String brand;
public String plate;
public double addr;
public int seating;
public int type;
public int status;
public Car(){
}
public Car(String brand, double addr, String plate,int type,int status,int serial) {
this.brand = brand;
this.plate = plate;
this.addr = addr;
this.type=type;
this.status=status;
this.serial=serial;
}
public Car(String brand, String plate, double addr, int seating,int type,int status,int serial) {
this.brand = brand;
this.plate = plate;
this.addr = addr;
this.seating = seating;
this.type=type;
this.status=status;
this.serial=serial;
}
public double discount(double day,int addr){
return 0.0;
}
}
2.子类继承,重写方法
package project.cartwo;
public class PassengerCar extends Car{
public PassengerCar(String brand, String plate, double addr,int seating,int type,int status,int serial) {
super( brand, plate, addr,seating,type ,status,serial);
}
public PassengerCar(){
}
@Override
public double discount(double day, int addr) {
double money;
if (day >= 3 && day <7) {
money = addr * 0.9 * day;
} else if (day >=7 && day <30) {
money = addr * 0.8 * day;
} else if (day >= 30&&day<150) {
money= addr * 0.7 * day;
} else if(day>150){
money = addr * 0.6 * day;
}else {
money=addr*day;
}
return money;
}
}
====================================================
package project.cartwo;
public class Limousine extends Car {
public Limousine(String brand, String plate, double addr ,int type,int status,int serial) {
super( brand, addr, plate, type ,status,serial);
}
public Limousine(){
super();
}
@Override
public double discount(double day, int addr) {
double money;
if (day > 7 && day <= 30) {
money = addr * 0.9 * day;
} else if (day > 30 && day <= 150) {
money =addr * 0.8 * day;
} else if (day >= 150) {
money = addr * 0.7 * day;
} else {
money=addr*day;
}
return money;
}
}
3.静态方法:
package project.cartwo;
import java.util.Scanner;
public class Comments {
public static boolean juge=true;
public static Car garage[]=new Car[100];
public static void creat() {
Limousine L1 = new Limousine( "宝马X6", "京NY28588", 800, 0 ,0,1);
Limousine L2 = new Limousine( "宝马550i", "京CNY3284", 600, 0 ,0,2);
Limousine L3 = new Limousine( "别克林荫", "京NT37465", 300, 0 ,0,3);
Limousine L4 = new Limousine( "别克GL8", "京NT96968", 600, 0 ,0,4);
PassengerCar p1 = new PassengerCar( "金龙", "京8696997", 1500, 16, 1, 0, 1 );
PassengerCar p2 = new PassengerCar( "金杯", "京9696996", 1500, 34, 1,0,2);
PassengerCar p3 = new PassengerCar( "金龙", "京8696998", 1500, 34, 1 ,0,3);
garage[0] = L1;
garage[1] = L2;
garage[2] = L3;
garage[3] = L4;
garage[4] = p1;
garage[5] = p2;
garage[6] = p3;
}
========================================
public static void usedSelect(){
boolean j = Comments.juge;
Scanner scanner = new Scanner( System.in );
System.out.println( "===========欢迎来到汽车租聘系统==============" );
Comments.creat();
while (j) {
System.out.println( "=======请选择您要选择的汽车种类:1.轿车 2.客车 3.退出" );
System.out.println( " " );
System.out.println( "请输入您要选择的汽车种类:" );
int kind = scanner.nextInt();
switch (kind) {
case 1:
Comments.selectKind( 0 );
j=false;
break;
case 2:
Comments.selectKind( 1 );
j=false;
break;
case 3:
System.out.println("欢迎您下次使用!!");
j=false;
break;
default:
System.out.println( "输入有误请重新输入" );
break;
}
}
}
===========================================
public static void show(int type) {
for (int i = 0; i <7 ; i++) {
if (garage[i].type == type && garage[i].status==0) {
if(type==0){
System.out.println( garage[i].serial + "\t\t" +garage[i].brand + "\t\t" + garage[i].plate + "\t\t" + garage[i].addr );
}else if(type==1)
System.out.println( "\t"+garage[i].serial + "\t\t"+ garage[i].brand + "\t\t" + garage[i].plate + "\t\t" + garage[i].addr + "\t\t" + garage[i].seating );
}
}
}
======================================
public static void selectLimousine(){
Scanner scanner = new Scanner( System.in );
System.out.println("请输入您要选择的车辆编号:");
int serial = scanner.nextInt();
garage[serial-1].status=1;
System.out.println("请输入您要租用的天数:");
double days = scanner.nextInt();
Limousine limousine = new Limousine();
printCar( serial-1,days );
System.out.print(limousine.discount( days, (int) garage[serial-1].addr ));
}
==================================================
public static void selectPassenger(){
Scanner scanner = new Scanner( System.in );
System.out.println("请输入您要选择的车辆编号:");
int serial = scanner.nextInt();
garage[serial+3].status=1;
System.out.println("请输入您要租用的天数:");
double days = scanner.nextInt();
PassengerCar passengerCar = new PassengerCar();
printCar( serial+3,days );
System.out.print(passengerCar.discount( days, (int) garage[serial+3].addr ));
}
============================================
public static void printCar(int V,double day){
System.out.println( "您选购的轿车订单如下:" );
System.out.println( "品牌: " + " 车牌号:" );
System.out.println( garage[V].brand + "\t\t" + garage[V].plate+"\t\t"
);
System.out.println( "总价格:");
}
==============================================
public static void selectKind(int type){
Scanner scanner = new Scanner( System.in );
while (juge) {
System.out.println( "=====汽车种类如下=====:" );
System.out.println( "编号: " + "品牌: " + " 车牌号:" + " 日租价格:" );
Comments.show( type );
if (type == 0) {
Comments.selectLimousine();
} else {
selectPassenger();
}
System.out.println( "=====>是否要继续选购:1.是 2.否" );
int i = scanner.nextInt();
if (i == 1) {
juge = true;
} else if (i == 2) {
juge = false;
System.out.println( "欢迎下次使用!!" );
}
}
}
}
4.系统入口:
package project.Text;
import project.cartwo.Comments;
public class StartText {
public static void main(String[] args) {
Comments.usedSelect();
}
}