数据结构(严蔚敏)算法2.2
-----------------------------------------------------------------------------------
Problem:已知线性表LA和LB中的数据归并为一个新的线性表LC,且LC中的数据元素仍按值非递减有序排列。例如,设
LA=(3,5,8,11)
LB=(2,6,8,9,11,15,20)
则LC=(2,3,5,6,8,8,9,11,11,15,20)
-----------------------------------------------------------------------------------
#include <iostream>
#include <malloc.h>
using namespace std;
struct List
{
int* sta;
int lenth;
int loclen;
};
void InitList(List* Ls,int lenth);
void InputList(List* Ls);
int LenthList(List* Ls);
void GetElem(List* Ls,int i,int *e);
bool ListInsert(List* Ls,int pos,int e);
bool IsFull(List* Ls);
void MergeList(List* La,List* Lb,List* Lc);
void ShowList(const List* Ls);
void SortList(List* Ls);
int main()
{
List la,lb;
int alen,blen;
cout<<"请输入初始化表a,表b的长度"<<endl;
cin>>alen>>blen;
cout<<"请初始化表a的数值"<<endl;
InitList(&la,alen);
InputList(&la);
SortList(&la);
cout<<"请初始化表b的数值"<<endl;
InitList(&lb,blen);
InputList(&lb);
SortList(&lb);
cout<<"当前表a的数值为:"<<endl;
ShowList(&la);
cout<<"当前表b的数值为:"<<endl;
ShowList(&lb);
List Lc;
MergeList(&la,&lb,& Lc);
cout<<"当前表c的数值为:"<<endl;
ShowList(&Lc);
return 0;
}
void InitList(List* Ls,int lenth)
{
Ls->sta=(int *)malloc(sizeof(int)*lenth);
if(!Ls->sta)
{
cout<<"内存分配失败!!!"<<endl;
exit;
}
Ls->loclen=0;
Ls->lenth=lenth;
}
void InputList(List* Ls)
{
cout<<"请输入表中元素"<<endl;
for(int i=0;i<Ls->lenth;i++)
cin>>Ls->sta[i];
Ls->loclen=Ls->lenth;
}
int LenthList(List* Ls)
{
return Ls->lenth;
}
void GetElem(List* Ls,int i,int *e)
{
*e=Ls->sta[--i];
}
bool ListInsert(List* Ls,int pos,int e)
{
pos--;
if(pos<0||pos>Ls->loclen) return false;
else
{
if(!IsFull(Ls))
{
int* p=Ls->sta;
for(int i=Ls->loclen-1;i>=pos;i++)
{
Ls->sta[i+1]=Ls->sta[i];
}
++Ls->loclen;
Ls->sta[pos]=e;
}
}
}
bool IsFull(List* Ls)
{
if(Ls->lenth==Ls->loclen) return true;
return false;
}
void MergeList(List* La,List* Lb,List* Lc)
{
int i=1,j=1,k=0;
int La_len=LenthList(La),Lb_len=LenthList(Lb);
InitList(Lc,La_len+Lb_len);
int a,b;
while((i<=La_len)&&(j<=Lb_len))
{
GetElem(La,i,&a); GetElem(Lb,j,&b);
if(a<=b){ListInsert(Lc,++k,a);++i;}
else{ListInsert(Lc,++k,b);j++;}
}
while(i<=La_len)
{
GetElem(La,i++,&a);ListInsert(Lc,++k,a);
}
while(j<=Lb_len)
{
GetElem(Lb,j++,&b);ListInsert(Lc,++k,b);
}
}
void ShowList(const List* Ls)
{
int*p=Ls->sta,*pe=&Ls->sta[Ls->loclen-1],i=0;
for(p;p<=pe;p++,i++)
cout<<*p<<" ";
cout<<endl;
}
void SortList(List* Ls)
{
int i,j;
for(i=0;i<Ls->lenth-1;i++)
for(j=i+1;j<Ls->lenth;j++)
{
if(Ls->sta[i]>Ls->sta[j])
{
int temp=Ls->sta[i];
Ls->sta[i]=Ls->sta[j];
Ls->sta[j]=temp;
}
}
}
结果:
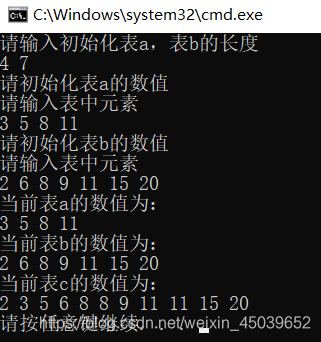