利用ajax来解决验证用户名问题
目录结构
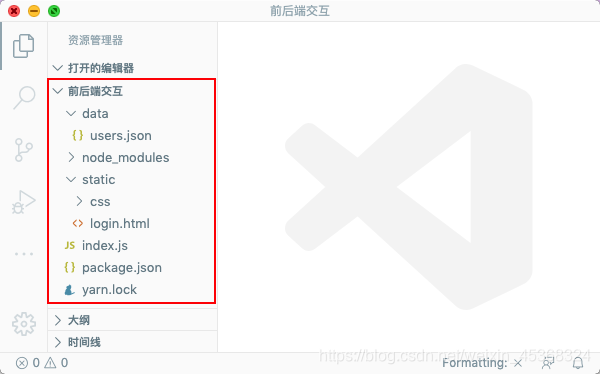
[{
"id":1,
"username":"张三",
"pwd":123
},{
"id":2,
"username":"李四",
"pwd":123
}]
const Koa = require("koa");
const Router = require("koa-router");
const koaStatic = require("koa-static");
const path = require("path");
const usersData = require("./data/users.json");
let app = new Koa();
let router = new Router();
app.use(koaStatic(path.join(__dirname, "/static")));
router.get("/", (ctx, next) => {
ctx.body = "hello";
});
router.get("/checkUserName", (ctx, next) => {
console.log(ctx.query.username);
let res = usersData.find((v) => v.username == ctx.query.username);
if (res) {
ctx.body = {
status: 1,
info: "用户名正确",
};
} else {
ctx.body = {
status: 2,
info: "用户名错误",
};
}
});
app.use(router.routes());
app.listen(3000, () => {
console.log("running...");
});
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<link rel="stylesheet" href="css/login.css" />
<title>Document</title>
</head>
<body>
<div class="loginContainer">
<h1>登录</h1>
<form action="/checkUser" method="post">
姓名:
<input class="inputStyle" type="text" name="username" />
<div class="exchange">用户名错误</div>
<br />密码:
<input class="inputStyle" type="password" name="pwd" /><br />
<input class="loginStyle" type="submit" value="登录" />
</form>
</div>
<script>
document.querySelector(".inputStyle").onblur = function () {
let xhr = new XMLHttpRequest();
xhr.open("get", `/checkUserName?username=${this.value}`, true);
xhr.onload = function () {
console.log(xhr.responseText);
let obj = JSON.parse(xhr.responseText);
document.querySelector(".exchange").innerHTML = obj.info;
if (obj.status == 1) {
document.querySelector(".exchange").style.color = "green";
} else {
document.querySelector(".exchange").style.color = "red";
}
};
xhr.send();
};
</script>
</body>
</html>
.loginContainer{
margin: 0 auto;
width: 600px;
text-align: center;
padding-top: 20px;
padding-bottom: 50px;
border: 1px solid;
}
.loginContainer input{
margin-bottom: 20px;
}
.loginStyle{
width: 160px;
height: 40px;
background: rgb(50,203,77);
color: white;
font-size: 17px;
}
.inputStyle{
width: 200px;
height: 30px;
padding: 5px;
outline: none;
}
.inputStyle:focus{
border: 1px solid rgb(50,203,77);
}
form{
position: relative;
}
.exchange{
position: absolute;
top:8px;
right: 65px;
color: red;
}