第六章:类和对象
1.类和对象的关系
js的基本类型,number,string,boolean...
我们需要描述这个客观世界,描述一个学生,学号,姓名,年龄,性别,手机号码...
我们的世界是由物质组成的,对象,就是一个真正存在的物体。一本书,一架飞机,一个人...
万事万物皆对象,世界是由对象构成的。
我们把同一种类的对象进行抽象,形成了类
类:特征和行为
学生类:
特征(属性):学号,姓名,年龄,性别,手机号码
行为(方法):上学,放学,考试...
========
类是同一种类对象的抽象,对象时类的实例
2.js如何创建类
ES5.1: function 函数式方式
function 类名(参数...){
//初始化函数...
}
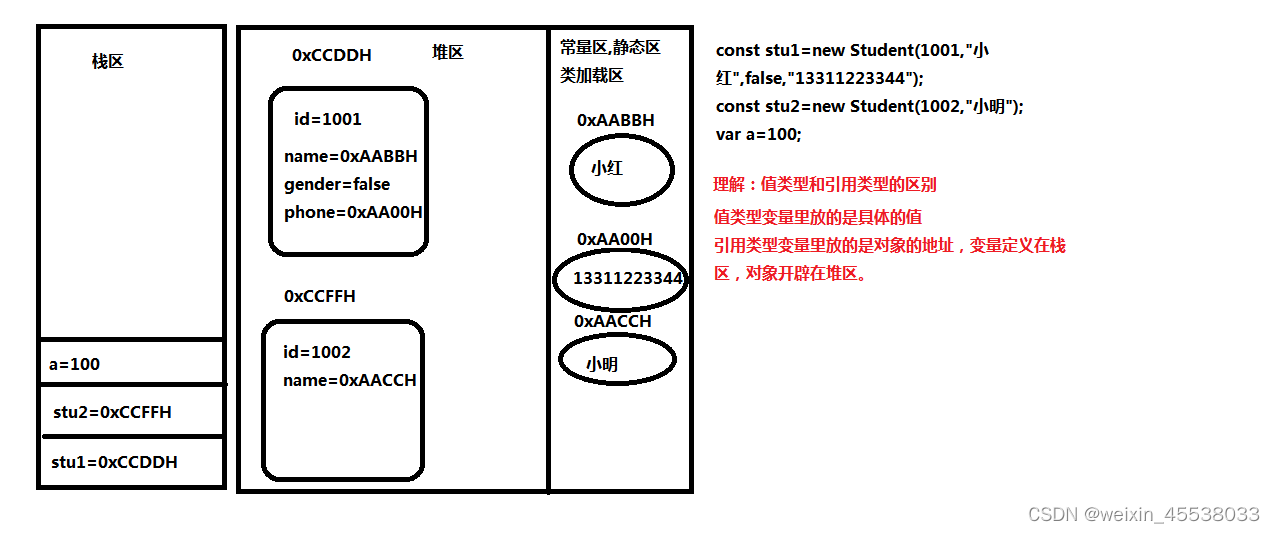
<script type="text/javascript">
function Student(id,name="",gender=false,phone=""){
this.id=id;
this.name=name;
this.gender=gender;
this.phone=phone;
this.gotoSchool=function(){
console.log(this.name+"上学去了");
}
this.goHome=function(){
console.log(this.name+"放学了");
}
}
const stu1=new Student(1001,"小红",false,"13311223344");
console.log( stu1.id,stu1.name,stu1.gender,stu1.phone);
stu1.gotoSchool();
stu1.goHome();
const stu2=new Student(1002,"小明");
console.log( stu2.id,stu2.name,stu2.gender,stu2.phone);
</script>
3.对象数组
<script type="text/javascript">
function Student(id,name="",gender=false,phone=""){
this.id=id;
this.name=name;
this.gender=gender;
this.phone=phone;
this.showme=function(){
console.log(this.id,this.name,this.gender,this.phone);
}
}
var students=new Array();
students[0]=new Student(1001,"小红",false,"13311223344");
students[1]=new Student(1002,"小明",true,"13311223366");
students.push(new Student(1003,"汤姆",true,"13811223366"));
var html="<table><tr><th>学号</th><th>姓名</th><th>性别</th><th>手机号码</th></tr>";
for (let s of students) {
html+=`
<tr>
<td>${s.id}</td>
<td>${s.name}</td>
<td>${s.gender?"帅哥":"美女"}</td>
<td>${s.phone}</td>
</tr>
`;
}
html+="</table>";
document.write(html);
</script>
4.原型对象
实例成员:是属于对象的 圆的半径
非实例成员:
===好比,手机号码,是属于对象的,但是 人的正常体温37,是属于整个人类的
圆周率
==================
js中,实例成员,都是this.xxx
==========================
什么是原型对象:prototype,js中,所有对象公有一个原型对象,原型对象时属于整个类型的
5.理解Object对象
<script type="text/javascript">
function Emp(id,name){
this.id=id;
this.name=name;
}
Emp.prototype.toString=function(){
return this.id+","+this.name;
}
const emp=new Emp(1001,"张三");
console.log(emp.toString());
console.log(emp.valueOf());
</script>
第七章:JSON对象
1.什么是JSON对象
JSON是存储和传输数据的格式
前后端分离的开发模型中,服务端常用来传输json数据给前端;
====
JSON--JavaScript Object Notation
是一种轻量级的数据交换格式,独立于语言, 自描述的,易于理解
json的语法来自于javascript对象符号语法, 格式是纯文本的
2.定义和使用
<script type="text/javascript">
const stu={
"id":1001,
"name":"小红",
"gender":false
}
console.log(stu,stu.id,stu.name,stu.gender);
const stu2={
"id":1001,
"name":"小红",
"gender":false,
"say":function(s){
console.log(this.name+"说:"+s);
}
}
stu2.say("饭吃了没");
const stu3={
id:1001,
name:"小红",
gender:false,
say:function(s){
console.log(this.name+"说:"+s);
},
address:{
city:"上海市",
area:"浦东新区",
street:"东方路123号"
}
}
console.log(stu3.address.city,stu3.address.street);
const book={};
book.id=1001;
book.title="js基础";
book.price=30;
book.show=function(){
console.log(this.id,this.title,this.price);
}
console.log(book.title);
book.show();
</script>
3.json对象数组
<script type="text/javascript">
const books=[
{id:1,title:"java基础",qty:1,price:20},
{id:2,title:"c++基础",qty:2,price:30},
{id:3,title:"html基础",qty:1,price:40},
{id:4,title:"javascript基础",qty:2,price:50},
];
function show(){
var html="<table>";
html+="<tr><td>编号</td><td>书名</td><td>数量</td><td>单价</td><td>小计</td></tr>";
for (let b of books) {
html+=`
<tr>
<td>${b.id}</td>
<td>${b.title}</td>
<td>${b.qty}</td>
<td>${b.price}</td>
<td>${b.price*b.qty}</td>
</tr>
`;
}
html+="</table>";
document.write(html);
}
show();
</script>
4.json对象和字符串互操作
<script type="text/javascript">
const stu = {
"id": 1001,
"name": "小红",
"gender": false
}
var s= JSON.stringify(stu);
console.log(s,typeof(s));
var jsonstr=`
[
{id:1,title:"java基础",qty:1,price:20},
{id:2,title:"c++基础",qty:2,price:30},
{id:3,title:"html基础",qty:1,price:40},
{id:4,title:"javascript基础",qty:2,price:50},
]
`;
console.log(jsonstr,typeof(jsonstr));
const books=eval(jsonstr);
console.log(eval("1+2+3+4"));
console.log(books.length,books);
</script>