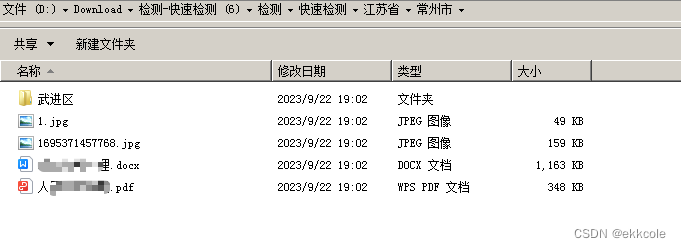
1、测试类
import lombok.Data;
@Data
public class ZipVo {
private String pathName;
private String data;
private String type;
private String suffix;
}
2、控制器
import com.ekkcole.utils.Func;
import org.apache.logging.log4j.util.Base64Util;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import javax.servlet.http.HttpServletResponse;
import java.io.*;
import java.net.HttpURLConnection;
import java.net.URL;
import java.util.*;
import java.util.stream.Collectors;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
@RestController
public class TestZip {
@GetMapping("/generateFileGroupDownZip")
public void generateFileGroupDownZip(HttpServletResponse response) {
List<ZipVo> zipVoList = new ArrayList<>();
ZipVo bean = new ZipVo();
bean.setData("http://localhost:8999/machine/upload/image/20230922/29909950475117.docx");
bean.setPathName("/常州/武进/");
bean.setType(".jpg");
zipVoList.add(bean);
ZipVo bean1 = new ZipVo();
bean1.setData("http://localhost:8999/machine/upload/image/20230922/30035456012377.zip");
bean1.setPathName("/常州/武进/湖塘/");
bean1.setType(".jpg");
zipVoList.add(bean1);
ZipVo bean2 = new ZipVo();
bean2.setData("http://localhost:8999/machine/upload/image/20230922/30895400564085.docx");
bean2.setPathName("/徐州/睢宁/庆安/");
bean2.setType(".jpg");
zipVoList.add(bean2);
String zipFileName = "测试" + ".zip";
ZipOutputStream zipStream = null;
BufferedInputStream bufferStream = null;
File zipFile = new File(zipFileName);
try {
zipStream = new ZipOutputStream(new FileOutputStream(zipFile));
Map<String, List<ZipVo>> collect = zipVoList.stream().collect(Collectors.groupingBy(ZipVo::getPathName));
for (Map.Entry<String, List<ZipVo>> zipMap : collect.entrySet()) {
String key = zipMap.getKey();
List<ZipVo> zipMapValueList = zipMap.getValue();
if (zipMapValueList.size() > 0) {
for (ZipVo zipVo : zipMapValueList) {
String attachUrl = zipVo.getData();
String suffix = zipVo.getType();
InputStream input = getInputStream(attachUrl);
ZipEntry zipEntry = new ZipEntry("\\" + key + "\\" + System.currentTimeMillis() + suffix);
zipStream.putNextEntry(zipEntry);
bufferStream = new BufferedInputStream(input, 1024 * 10);
int read = 0;
byte[] buf = new byte[1024 * 10];
while ((read = bufferStream.read(buf, 0, 1024 * 10)) != -1) {
zipStream.write(buf, 0, read);
}
}
}
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (null != bufferStream) {
bufferStream.close();
}
if (null != zipStream) {
zipStream.flush();
zipStream.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
if (zipFile.exists()) {
groupDownZip(response, zipFileName);
zipFile.delete();
}
}
private void groupDownZip(HttpServletResponse response, String filename) {
if (filename != null) {
FileInputStream inputStream = null;
BufferedInputStream bufferedInputStream = null;
OutputStream outputStream = null;
try {
File file = new File(filename);
if (file.exists()) {
response.setHeader("Content-Type", "application/octet-stream");
response.setHeader("Content-Disposition", "attachment;filename=" + new String(filename.getBytes(), "ISO-8859-1"));
inputStream = new FileInputStream(file);
bufferedInputStream = new BufferedInputStream(inputStream);
outputStream = response.getOutputStream();
byte[] buffer = new byte[1024];
int lenth = 0;
while ((lenth = bufferedInputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, lenth);
}
} else {
String error = Base64Util.encode("文件内容为空");
}
} catch (IOException ex) {
ex.printStackTrace();
} finally {
try {
if (inputStream != null) {
inputStream.close();
}
if (bufferedInputStream != null) {
bufferedInputStream.close();
}
if (outputStream != null) {
outputStream.flush();
outputStream.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
private InputStream getInputStream(String attachUrl) {
URL url = null;
InputStream is = null;
ByteArrayOutputStream outStream = null;
HttpURLConnection httpUrl = null;
try {
if (Func.isNotEmpty(attachUrl)) {
String[] args = attachUrl.split("/");
String name1 = args[args.length - 1];
String name2 = java.net.URLEncoder.encode(name1, "utf-8");
String newUrl = attachUrl.replace(name1, name2);
url = new URL(newUrl);
httpUrl = (HttpURLConnection) url.openConnection();
httpUrl.setConnectTimeout(900);
httpUrl.setReadTimeout(900);
httpUrl.connect();
is = httpUrl.getInputStream();
return is;
}
} catch (Exception e) {
}
return null;
}
}