来源:juejin.cn/post/7263760831052906552
前言
正文
实现更多的接口
重写 equals 和 hashcode 方法
增加配置项和参数
增加监听回调
构建通用工具类
添加新的异常类型
实现更多设计模式
扩展注释和文档
结语
如果按代码量算工资,也许应该这样写!
前言
假如有一天我们要按代码量来算工资,那怎样才能写出一手漂亮的代码,同时兼顾代码行数和实际意义呢?
要在增加代码量的同时提高代码质量和可维护性,能否做到呢?
答案当然是可以,这可难不倒我们这种摸鱼高手。
耐心看完,你一定有所收获。
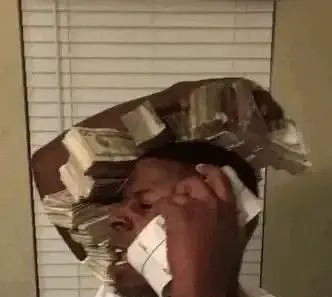
正文
实现更多的接口
给每一个方法都实现各种“无关痛痒”的接口,比如Serializable
、Cloneable
等,真正做到不影响使用的同时增加了相当数量的代码。
为了这些代码量,其中带来的性能损耗当然是可以忽略的。
public class ExampleClass implements Serializable, Comparable<ExampleClass>, Cloneable, AutoCloseable {
@Override
public int compareTo(ExampleClass other) {
// 比较逻辑
return 0;
}
// 实现 Serializable 接口的方法
private void writeObject(ObjectOutputStream out) throws IOException {
// 序列化逻辑
}
private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException {
// 反序列化逻辑
}
// 实现 Cloneable 接口的方法
@Override
public ExampleClass clone() throws CloneNotSupportedException {
// 复制对象逻辑
return (ExampleClass) super.clone();
}
// 实现 AutoCloseable 接口的方法
@Override
public void close() throws Exception {
// 关闭资源逻辑
}
}
除了示例中的Serializable
、 Comparable
、 Cloneable
、 AutoCloseable
,还有Iterable
重写 equals 和 hashcode 方法
重写 equals
和 hashCode
方法绝对是上上策,不仅增加了代码量,还为了让对象在相等性判断和散列存储时能更完美的工作,确保代码在处理对象相等性时更准确、更符合业务逻辑。
public class ExampleClass {
private String name;
private int age;
// 重写 equals 方法
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || getClass() != obj.getClass()) {
return false;
}
ExampleClass other = (ExampleClass) obj;
return this.age == other.age && Objects.equals(this.name, other.name);
}
// 重写 hashCode 方法
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
增加配置项和参数
不要管能不能用上,梭哈就完了,问就是为了健壮性和拓展性。
public class AppConfig {
private int maxConnections;
private String serverUrl;
private boolean enableFeatureX;
// 新增配置项
private String emailTemplate;
private int maxRetries;
private boolean enableFeatureY;
// 写上构造函数和getter/setter
}
增加监听回调
给业务代码增加监听回调,比如执行前、执行中、执行后等各种Event,这里举个完整的例子。
比如创建个 EventListener
,负责监听特定类型的事件,事件源则是产生事件的对象。通过EventListener
在代码中增加执行前、执行中和执行后的事件。
首先,我们定义一个简单的事件类 Event
:
public class Event {
private String name;
public Event(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
然后,我们定义一个监听器接口 EventListener
:
public interface EventListener {
void onEventStart(Event event);
void onEventInProgress(Event event);
void onEventEnd(Event event);
}
接下来,我们定义一个事件源类 EventSource
,在执行某个业务方法时,触发事件通知:
public class EventSource {
private List<EventListener> listeners = new ArrayList<>();
public void addEventListener(EventListener listener) {
listeners.add(listener);
}
public void removeEventListener(EventListener listener) {
listeners.remove(listener);
}
public void businessMethod() {
Event event = new Event("BusinessEvent");
// 通知监听器:执行前事件
for (EventListener listener : listeners) {
listener.onEventStart(event);
}
// 模拟执行业务逻辑
System.out.println("Executing business method...");
// 通知监听器:执行中事件
for (EventListener listener : listeners) {
listener.onEventInProgress(event);
}
// 模拟执行业务逻辑
System.out.println("Continuing business method...");
// 通知监听器:执行后事件
for (EventListener listener : listeners) {
listener.onEventEnd(event);
}
}
}
现在,我们可以实现具体的监听器类,比如 BusinessEventListener
,并在其中定义事件处理逻辑:
public class BusinessEventListener implements EventListener {
@Override
public void onEventStart(Event event) {
System.out.println("Event Start: " + event.getName());
}
@Override
public void onEventInProgress(Event event) {
System.out.println("Event In Progress: " + event.getName());
}
@Override
public void onEventEnd(Event event) {
System.out.println("Event End: " + event.getName());
}
}
最后,我们写个main函数来演示监听事件:
public class Main {
public static void main(String[] args) {
EventSource eventSource = new EventSource();
eventSource.addEventListener(new BusinessEventListener());
// 执行业务代码,并触发事件通知
eventSource.businessMethod();
// 移除监听器
eventSource.removeEventListener(businessEventListener);
}
}
如此这般那般,代码量猛增,还顺带实现了业务代码的流程监听。当然这只是最简陋的实现,真实环境肯定要比这个复杂的多。
构建通用工具类
同样的,甭管用不用的上,定义更多的方法,都是为了健壮性。
比如下面这个StringUtils,可以从ApacheCommons、SpringBoot的StringUtil或HuTool的StrUtil中拷贝更多的代码过来,美其名曰内部工具类。
public class StringUtils {
public static boolean isEmpty(String str) {
return str == null || str.trim().isEmpty();
}
public static boolean isBlank(String str) {
return str == null || str.trim().isEmpty();
}
// 新增方法:将字符串反转
public static String reverse(String str) {
if (str == null) {
return null;
}
return new StringBuilder(str).reverse().toString();
}
// 新增方法:判断字符串是否为整数
public static boolean isInteger(String str) {
try {
Integer.parseInt(str);
return true;
} catch (NumberFormatException e) {
return false;
}
}
}
添加新的异常类型
添加更多异常类型,对不同的业务抛出不同的异常,每种异常都要单独去处理
public class CustomException extends RuntimeException {
// 构造函数
public CustomException(String message) {
super(message);
}
// 新增异常类型
public static class NotFoundException extends CustomException {
public NotFoundException(String message) {
super(message);
}
}
public static class ValidationException extends CustomException {
public ValidationException(String message) {
super(message);
}
}
}
// 示例:添加不同类型的异常处理
public class ExceptionHandling {
public void process(int value) {
try {
if (value < 0) {
throw new IllegalArgumentException("Value cannot be negative");
} else if (value == 0) {
throw new ArithmeticException("Value cannot be zero");
} else {
// 正常处理逻辑
}
} catch (IllegalArgumentException e) {
// 异常处理逻辑
} catch (ArithmeticException e) {
// 异常处理逻辑
}
}
}
实现更多设计模式
在项目中运用更多设计模式,也不失为一种合理的方式,比如单例模式、工厂模式、策略模式、适配器模式等各种常用的设计模式。
比如下面这个单例,大大节省了内存空间,虽然它存在线程不安全等问题。
public class SingletonPattern {
// 单例模式
private static SingletonPattern instance;
private SingletonPattern() {
// 私有构造函数
}
public static SingletonPattern getInstance() {
if (instance == null) {
instance = new SingletonPattern();
}
return instance;
}
}
还有下面这个策略模式,能避免过多的if-else条件判断,降低代码的耦合性,代码的扩展和维护也变得更加容易。
// 策略接口
interface Strategy {
void doOperation(int num1, int num2);
}
// 具体策略实现类
class AdditionStrategy implements Strategy {
@Override
public void doOperation(int num1, int num2) {
int result = num1 + num2;
System.out.println("Addition result: " + result);
}
}
class SubtractionStrategy implements Strategy {
@Override
public void doOperation(int num1, int num2) {
int result = num1 - num2;
System.out.println("Subtraction result: " + result);
}
}
// 上下文类
class Context {
private Strategy strategy;
public Context(Strategy strategy) {
this.strategy = strategy;
}
public void executeStrategy(int num1, int num2) {
strategy.doOperation(num1, num2);
}
}
// 测试类
public class StrategyPattern {
public static void main(String[] args) {
int num1 = 10;
int num2 = 5;
// 使用加法策略
Context context = new Context(new AdditionStrategy());
context.executeStrategy(num1, num2);
// 使用减法策略
context = new Context(new SubtractionStrategy());
context.executeStrategy(num1, num2);
}
}
对比下面这段条件判断,高下立判。
public class Calculator {
public static void main(String[] args) {
int num1 = 10;
int num2 = 5;
String operation = "addition"; // 可以根据业务需求动态设置运算方式
if (operation.equals("addition")) {
int result = num1 + num2;
System.out.println("Addition result: " + result);
} else if (operation.equals("subtraction")) {
int result = num1 - num2;
System.out.println("Subtraction result: " + result);
} else if (operation.equals("multiplication")) {
int result = num1 * num2;
System.out.println("Multiplication result: " + result);
} else if (operation.equals("division")) {
int result = num1 / num2;
System.out.println("Division result: " + result);
} else {
System.out.println("Invalid operation");
}
}
}
扩展注释和文档
如果要增加代码量,写更多更全面的注释也不失为一种方式。
/**
* 这是一个示例类,用于展示增加代码数量的技巧和示例。
* 该类包含一个示例变量 value 和示例构造函数 ExampleClass(int value)。
* 通过示例方法 getValue() 和 setValue(int newValue),可以获取和设置 value 的值。
* 这些方法用于展示如何增加代码数量,但实际上并未实现实际的业务逻辑。
*/
public class ExampleClass {
// 示例变量
private int value;
/**
* 构造函数
*/
public ExampleClass(int value) {
this.value = value;
}
/**
* 获取示例变量 value 的值。
* @return 示范变量 value 的值
*/
public int getValue() {
return value;
}
/**
* 设置示例变量 value 的值。
* @param newValue 新的值,用于设置 value 的值。
*/
public void setValue(int newValue) {
this.value = newValue;
}
}
结语
哪怕是以代码量算工资,咱也得写出高质量的代码,合理合法合情的赚票子。
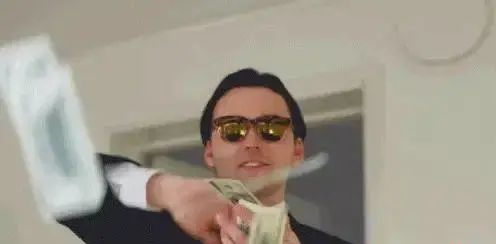
推荐阅读:
不是你需要中台,而是一名合格的架构师(附各大厂中台建设PPT)