@RequestMapping("approval")
public class ApprovalManageController {
final static String ORDER_BIZ_KEY = "下订单";
final static String AWARD_BIZ_KEY = "定标";
final static String invoice_BIZ_KEY = "invoice_approval"; //开票
final static String purchase_BIZ_KEY = "purchase-payment"; //采购付款管理
/**
* 通用电气
*/
final static Long HZGE_ID = 1723L;
/**
* 梅特勒
*/
final static Long MTL_ID = 1682L;
@Lazy
@Autowired
private TaskApi taskApi;
@Lazy
@Autowired
private ProcessInstancesApi processInstancesApi;
@Lazy
@Autowired
private SessionUser sessionUser;
@Lazy
@Autowired
private IOrdersService iOrdersService;
@Lazy
@Autowired
private IQuotationService iQuotationService;
@Lazy
@Autowired
private IApproveService iApproveService;
@Autowired
private OrganizationApi organizationApi;
@Lazy
@Autowired
private InvoiceApi invoiceApi;
@Lazy
@Autowired
private SettleApi settleApi;
/**
* 审批
*/
@RequestMapping("complete")
public RestResponse<String> complete(String taskID, boolean isOk, String approvalReason,
@RequestParam("id") Long id) {
User user = sessionUser.get();
String name = user.getUsername();
// String name = "liutingting";
// String name = "gm_jhh";
String ok = "";
if (isOk) {
ok = "同意";
} else {
ok = "驳回";
}
RestResponse done = RestResponse.success("审批完成"); //定义变量
try {
// 审批后对原业务数据进行版本号更新 用于业务数据检索时自然判断是否在审批任务中,避免重复送审
TaskEntityCustom task;
//刷新页面
try {
task = taskApi.getTask(taskID); //根据任务id查询任务
} catch (Exception e) {
e.printStackTrace();
done = RestResponse.success("已经通过审批,请刷新页面!");
return done;
}
//获取实例id
String processInstanceId = task.getProcessInstanceId();
//获取实例
ProcessInstanceEntityCustom processInstance = processInstancesApi.getProcessInstance(processInstanceId);
String businessKey = processInstance.getBusinessKey();
//获取执行中的实例的参数
RestVariable variable = processInstancesApi.getVariable(task.getExecutionId(), "form");
Organization currOrg = organizationApi.get(sessionUser.getCurrentOrgID()).getData();
//财务开票审批
if (invoice_BIZ_KEY.equals(businessKey)) {
//满足任务完成动作
List<RestVariable> mapList = new ArrayList<>();
RestVariable isOkRestVariable = new RestVariable();
isOkRestVariable.setName("isOk");
isOkRestVariable.setValue(false);
mapList.add(isOkRestVariable);
RestVariable commentRestVariable = new RestVariable();
commentRestVariable.setName("approvalReason");
commentRestVariable.setValue(approvalReason);
mapList.add(commentRestVariable);
//添加意见
CommentRequest commentRequest = new CommentRequest();
commentRequest.setMessage(name + ":" + ok + approvalReason);
commentRequest.setSaveProcessInstanceId(true);
taskApi.newComment(taskID, commentRequest);
//完成审批任务
TaskActionRequest actionRequest = new TaskActionRequest();
//修改变量
List<RestVariable> list = new ArrayList<>();
Map<String, Object> result = new HashMap<>(3);
RestVariable approvalResult = new RestVariable();
//审批结果 0处理中,1以通过,2已驳回,3未提交
if (isOk) {
//通过
approvalResult.setName("approvalResult");
approvalResult.setValue("1");
variable.setScope("global");
list.add(approvalResult);
} else {
//驳回
approvalResult.setName("approvalResult");
approvalResult.setValue("2");
variable.setScope("global");
list.add(approvalResult);
}
RestVariable approvalStatus = new RestVariable();
//申请单状态 0.处理中,1已结束 2已撤回。
approvalStatus.setName("approvalStatus");
approvalStatus.setValue("1");
variable.setScope("global");
list.add(approvalStatus);
ApproveEntityCustom approveEntity = new ApproveEntityCustom();
approveEntity.setApprover(name);
approveEntity.setOpinion(approvalReason);
approveEntity.setRatify(ok);
approveEntity.setGrade("一级审批");
List<ApproveEntityCustom> approveList = new ArrayList<>();
approveList.add(approveEntity);
//将值转为字符串
ObjectMapper mapper = new ObjectMapper();
String jsonString = null;
try {
jsonString = mapper.writeValueAsString(approveList);
} catch (Exception e) {
e.printStackTrace();
}
RestVariable approvalRank = new RestVariable();
approvalRank.setName("approvalRank");
variable.setScope("global");
approvalRank.setValue(jsonString);
processInstancesApi.updateVariable(processInstanceId, "approvalResult", approvalResult);
processInstancesApi.updateVariable(processInstanceId, "approvalStatus", approvalStatus);
processInstancesApi.updateVariable(processInstanceId, "approvalRank", approvalRank);
//添加审批人变量
List<RestVariable> li = new ArrayList<>();
RestVariable approverVariable = new RestVariable();
approverVariable.setName(name + "Variable");
approverVariable.setValue(name);
li.add(approverVariable);
taskApi.createTaskVariable(taskID, li);
//添加审批人审批结果变量
List<RestVariable> listRest = new ArrayList<>();
RestVariable approverResultVariable = new RestVariable();
approverResultVariable.setName(name + "ResultVariable");
if (isOk) {
approverResultVariable.setValue("同意");
} else {
approverResultVariable.setValue("驳回");
}
listRest.add(approverResultVariable);
taskApi.createTaskVariable(taskID, listRest);
//添加审批时间
SimpleDateFormat simpleDateFormat = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
List<RestVariable> da = new ArrayList<>();
RestVariable dateVariable = new RestVariable();
dateVariable.setName(name + "dateVariable");
dateVariable.setValue(simpleDateFormat.format(new Date()));
da.add(dateVariable);
taskApi.createTaskVariable(taskID, da);
//添加任务等级名称
List<RestVariable> grade = new ArrayList<>();
RestVariable gradeVariable = new RestVariable();
gradeVariable.setName(name + "gradeVariable");
gradeVariable.setValue(task.getName());
grade.add(gradeVariable);
taskApi.createTaskVariable(taskID, grade);
//意见和完成动作添加进变量
actionRequest.setVariables(mapList); // 审批人,意见
actionRequest.setAction("complete"); //设置动作完成
taskApi.executeTaskAction(taskID, actionRequest);
//修改开票状态 待审批为待开票
InvoiceStatus status;
if (!isOk) {
status = InvoiceStatus.Returned;
} else {
status = InvoiceStatus.DrawerConfirming;
}
InvoiceApprove approve = InvoiceApprove.builder()
.ids(Collections.singletonList(Long.valueOf(id.toString())))
.approvalReason(null == approvalReason ? "" : approvalReason)
.status(status).build();
try {
invoiceApi.approve(approve);
} catch (Exception e) {
GomroExceptionUtils.error(e);
done = RestResponse.success("任务完成失败:" + e.getMessage());
}
}//采购付款管理审批
else if (purchase_BIZ_KEY.equals(businessKey)) {
//满足任务完成动作
List<RestVariable> mapList = new ArrayList<>();
RestVariable isOkRestVariable = new RestVariable();
isOkRestVariable.setName("isOk");
isOkRestVariable.setValue(isOk);
mapList.add(isOkRestVariable);
RestVariable commentRestVariable = new RestVariable();
commentRestVariable.setName("approvalReason");
commentRestVariable.setValue(approvalReason);
mapList.add(commentRestVariable);
//完成审批任务
TaskActionRequest actionRequest = new TaskActionRequest();
//一级审批
// if (name.equals("liutingting")) {
if (name.equals("liutingting")) {
//修改变量
/* List<RestVariable> list = new ArrayList<>();
Map<String, Object> result = new HashMap<>(3);
RestVariable approvalResult = new RestVariable();
*/
List<RestVariable> list = new ArrayList<>();
Map<String, Object> result = new HashMap<>(3);
RestVariable approvalResult = new RestVariable();
//审批结果 0处理中,1以通过,2已驳回,3未提交
RestVariable approvalStatus = new RestVariable();
//申请单状态 0.处理中,1已结束 2已撤回。
if (isOk) {
approvalResult.setName("approvalResult");
approvalResult.setValue("0");
//申请单状态
approvalStatus.setName("approvalStatus");
approvalStatus.setValue("0");
} else {
//驳回
approvalResult.setName("approvalResult");
approvalResult.setValue("2");
//申请单状态
approvalStatus.setName("approvalStatus");
approvalStatus.setValue("1");
}
processInstancesApi.updateVariable(processInstanceId, "approvalResult", approvalResult);
processInstancesApi.updateVariable(processInstanceId, "approvalStatus", approvalStatus);
//修改一级审批内容
ApproveEntityCustom approveEntity = new ApproveEntityCustom();
approveEntity.setApprover(name);
approveEntity.setOpinion(approvalReason);
approveEntity.setRatify(ok);
approveEntity.setGrade("一级审批");
List<ApproveEntityCustom> approveList = new ArrayList<>();
approveList.add(approveEntity);
//将值转为字符串
ObjectMapper mapper = new ObjectMapper();
String jsonString = null;
try {
jsonString = mapper.writeValueAsString(approveList);
} catch (Exception e) {
e.printStackTrace();
}
RestVariable approvalRank = new RestVariable(</
flowable 方法记录
最新推荐文章于 2024-08-21 08:16:57 发布
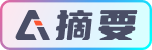