依赖下载
<!--七牛云图片上传-->
<dependency>
<groupId>com.qiniu</groupId>
<artifactId>qiniu-java-sdk</artifactId>
<version>[7.2.0, 7.2.99]</version>
</dependency>
<!-- 七牛云sdk相关依赖-->
<dependency>
<groupId>com.squareup.okhttp3</groupId>
<artifactId>okhttp</artifactId>
<version>3.14.2</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.google.code.gson</groupId>
<artifactId>gson</artifactId>
<version>2.8.5</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>com.qiniu</groupId>
<artifactId>happy-dns-java</artifactId>
<version>0.1.6</version>
<scope>test</scope>
</dependency>
代码整合
七牛云相关属性配置,直接写在application.yml
中
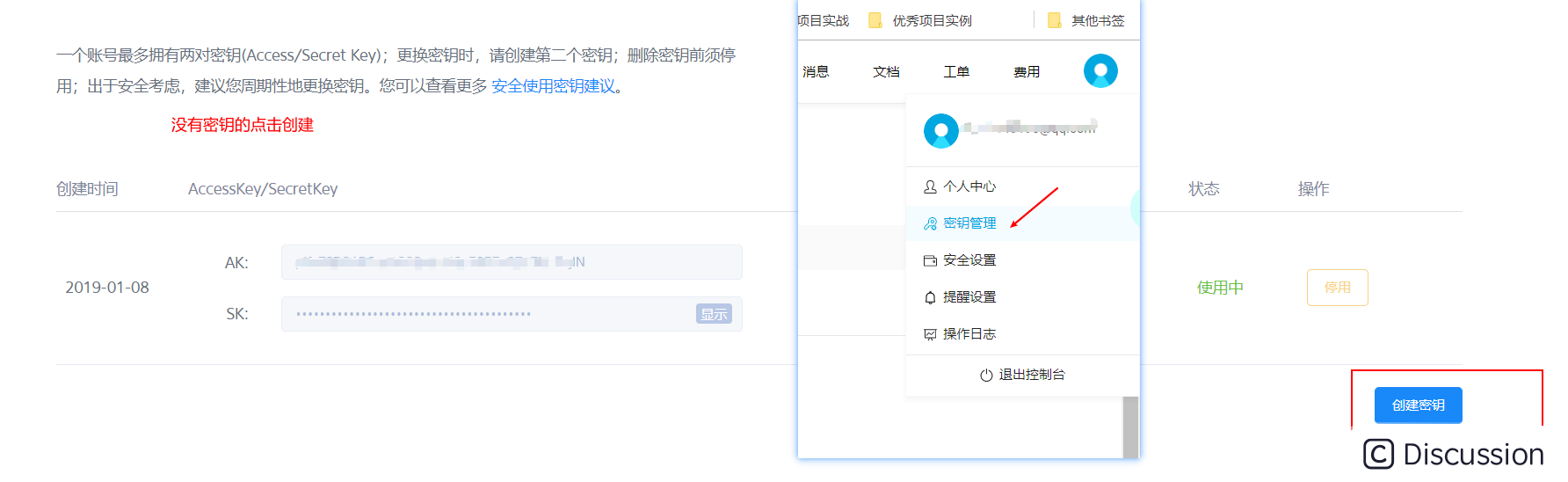
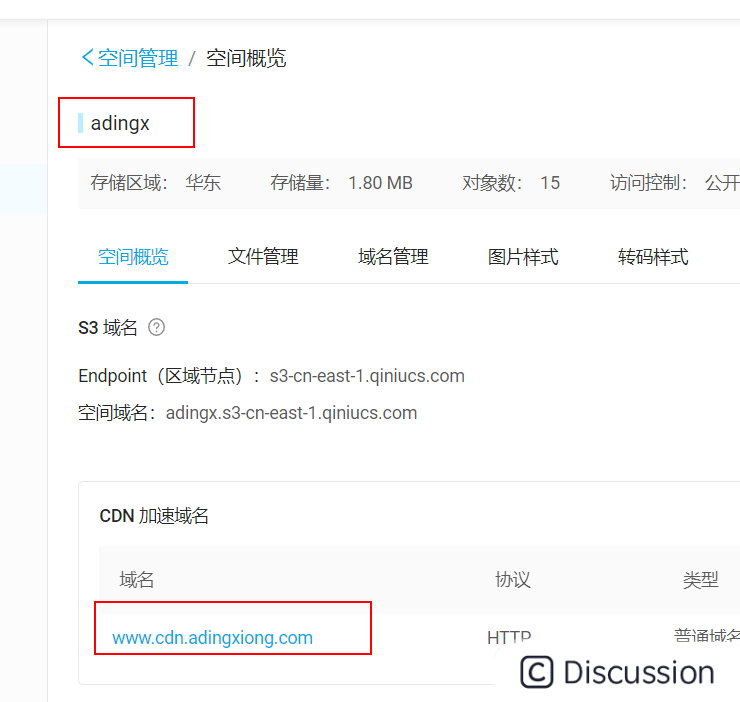
qiniu:
accesskey: xxxxxxxxxxxxxxxx
secretkey: xxxxxxxxxxxxxxxx
#空间名
bucket: admin
# 加速域名
domain: cdn.nihao.com
package com.adingxiong.pm.controller;
import com.adingxiong.pm.entity.vo.ImageListVo;
import com.adingxiong.pm.model.Result;
import com.adingxiong.pm.model.RetCode;
import com.adingxiong.pm.util.UploadUtils;
import com.google.gson.Gson;
import com.qiniu.common.QiniuException;
import com.qiniu.http.Response;
import com.qiniu.storage.BucketManager;
import com.qiniu.storage.Configuration;
import com.qiniu.storage.Region;
import com.qiniu.storage.UploadManager;
import com.qiniu.storage.model.BatchStatus;
import com.qiniu.storage.model.DefaultPutRet;
import com.qiniu.storage.model.FileInfo;
import com.qiniu.util.Auth;
import io.swagger.annotations.Api;
import io.swagger.annotations.ApiImplicitParam;
import io.swagger.annotations.ApiImplicitParams;
import io.swagger.annotations.ApiOperation;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletRequest;
import java.io.File;
import java.io.IOException;
import java.text.SimpleDateFormat;
import java.util.*;
import java.util.stream.Collectors;
/**
* 七牛云图片上传
*/
@RestController
@RequestMapping("/img")
@Api(tags = "资源管理")
public class ImageUploadController {
@Autowired
private QiniuConfiguration config;
private String accesskey = config.getAccesskey();
private String secretKey = config.getSecretKey();
private String bucket = config.getBucket();
private String bucket = config.getBucket();
@ApiOperation(value = "获取图片上传的Token")
@RequestMapping(value = "/getUploadToken", method = RequestMethod.GET)
public Result qiniuToken() {
try {
//验证七牛云身份是否认证通过
Auth auth = Auth.create(accesskey, secretKey);
//生成上传凭证
String token = auth.uploadToken(bucket);
Map<String, String> map = new HashMap<>();
map.put("upToken", token);
map.put("domain", domain);
String randomFile = UUID.randomUUID().toString();
map.put("imgUrl", randomFile);
return new Result<>().setCode(RetCode.SUCCESS).setMsg("获取token成功").setData(map);
} catch (Exception e) {
return new Result().setMsg(e.toString()).setCode(RetCode.FAIL).setMsg("获取token失败");
}
}
@ApiOperation(value = "上传资源至指定七牛云服务器")
@RequestMapping(value = "/uploadResource", method = RequestMethod.POST)
public Result uploadResource(@RequestParam("file") MultipartFile file) {
//构造一个带指定 Region 对象的配置类
Configuration cfg = new Configuration(Region.region0());
//...其他参数参考类注释
UploadManager uploadManager = new UploadManager(cfg);
//如果是Windows情况下,格式是 D:\\qiniu\\test.png
//String localFilePath = "D:\\qiniu\\4.png";
//默认不指定key的情况下,以文件内容的hash值作为文件名
String key = UUID.randomUUID().toString().replaceAll("-", "");
Auth auth = Auth.create(accesskey, secretKey);
String upToken = auth.uploadToken(bucket);
try {
byte[] byteInputStream = file.getBytes();
Response response = uploadManager.put(byteInputStream, key, upToken);
//解析上传成功的结果
DefaultPutRet putRet = new Gson().fromJson(response.bodyString(), DefaultPutRet.class);
return new Result<>().setCode(RetCode.SUCCESS).setMsg("资源上传成功").setData(putRet);
} catch (QiniuException ex) {
Response r = ex.response;
return new Result<>().setCode(RetCode.FAIL).setMsg(r.toString());
} catch (IOException e) {
e.printStackTrace();
return new Result<>().setCode(RetCode.FAIL).setMsg(e.toString());
}
}
@ApiOperation(value = "获取七牛云空间中的列表信息")
@RequestMapping(value = "/getQiniuList", method = RequestMethod.GET)
public Result getQiniuList() {
//构造一个带指定 Region 对象的配置类
Configuration cfg = new Configuration(Region.region0());
//...其他参数参考类注释
Auth auth = Auth.create(accesskey, secretKey);
BucketManager bucketManager = new BucketManager(auth, cfg);
//文件名前缀
String prefix = "";
//每次迭代的长度限制,最大1000,推荐值 1000
int limit = 1000;
//指定目录分隔符,列出所有公共前缀(模拟列出目录效果)。缺省值为空字符串
String delimiter = "";
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
//列举空间文件列表
BucketManager.FileListIterator fileListIterator = bucketManager.createFileListIterator(bucket, prefix, limit, delimiter);
List<ImageListVo> list = new ArrayList<>();
while (fileListIterator.hasNext()) {
//处理获取的file list结果
FileInfo[] items = fileListIterator.next();
for(FileInfo info :items){
ImageListVo vo = new ImageListVo();
vo.setEndUser(info.endUser);
vo.setFsize(info.fsize);
vo.setHash(info.hash);
vo.setMineType(info.mimeType);
vo.setKey(info.key);
String time = info.putTime + "";
time = time.substring(0,13);
vo.setPutTime(sdf.format(new Date(Long.parseLong(time))));
list.add(vo);
}
}
return new Result<>().setCode(RetCode.SUCCESS).setMsg("获取资源列表成功").setData(list);
}
@ApiOperation(value = "批量删除文件(key,type)")
@ApiImplicitParams({
@ApiImplicitParam(name = "keys", value = "需要删除的所有keys", paramType = "query", dataType = "Array")
})
@RequestMapping(value = "/deletImg", method = RequestMethod.GET)
public Result deletImg(String[] keys) {
//构造一个带指定 Region 对象的配置类
Configuration cfg = new Configuration(Region.region0());
//...其他参数参考类注释
Auth auth = Auth.create(accesskey, secretKey);
BucketManager bucketManager = new BucketManager(auth, cfg);
try {
BucketManager.BatchOperations batchOperations = new BucketManager.BatchOperations();
batchOperations.addDeleteOp(bucket, keys);
Response response = bucketManager.batch(batchOperations);
BatchStatus[] batchStatusList = response.jsonToObject(BatchStatus[].class);
for (int i = 0; i < keys.length; i++) {
BatchStatus status = batchStatusList[i];
String key = keys[i];
System.out.print(key + "\t");
if (status.code != 200) {
return new Result<>().setCode(RetCode.FAIL).setMsg(status.data.error);
}
}
return new Result<>().setCode(RetCode.SUCCESS).setMsg("删除成功");
} catch (QiniuException ex) {
return new Result<>().setCode(RetCode.INTERNAL_SERVER_ERROR).setMsg(ex.response.toString());
}
}
@ApiOperation(value = "文件重命名")
@ApiImplicitParams({
@ApiImplicitParam(name = "key", value = "文件key", paramType = "query", dataType = "String"),
@ApiImplicitParam(name = "newName", value = "新的文件名", paramType = "query", dataType = "Array"),
})
@RequestMapping(value = "/rename", method = RequestMethod.GET)
public Result rename(String[] keyList, String newName) {
Configuration cfg = new Configuration(Region.region0());
//...其他参数参考类注释
Auth auth = Auth.create(accesskey, secretKey);
BucketManager bucketManager = new BucketManager(auth, cfg);
try {
BucketManager.BatchOperations batchOperations = new BucketManager.BatchOperations();
for (String key : keyList) {
batchOperations.addMoveOp(bucket, key, bucket, newName);
}
Response response = bucketManager.batch(batchOperations);
BatchStatus[] batchStatusList = response.jsonToObject(BatchStatus[].class);
for (int i = 0; i < keyList.length; i++) {
BatchStatus status = batchStatusList[i];
String key = keyList[i];
if (status.code != 200) {
return new Result<>().setCode(RetCode.FAIL).setMsg(status.data.error);
}
}
return new Result<>().setCode(RetCode.SUCCESS).setMsg("修改完成");
} catch (QiniuException ex) {
return new Result<>().setCode(RetCode.INTERNAL_SERVER_ERROR).setMsg(ex.response.toString());
}
}
@Value(value = "${file.location.path}")
private String savePath;
@ApiOperation(value = "本地资源上传")
@RequestMapping(value = "/uploadlocal", method = RequestMethod.POST)
public Result uploadImg(@RequestParam MultipartFile file, HttpServletRequest request) throws IOException {
if (file != null) {
double size = file.getSize();
byte[] sizebyte = file.getBytes();
String filename = file.getOriginalFilename();
String type = filename.indexOf(".") != -1 ? filename.substring(filename.lastIndexOf(".") + 1, filename.length()) : null;
if (type != null) {
if ("GIF".equals(type.toUpperCase()) || "PNG".equals(type.toUpperCase()) || "JPG".equals(type.toUpperCase()) || "JPEG".equals(type.toUpperCase())) {
String url = UploadUtils.upload(file, "/upload", savePath);
return new Result<>().setCode(RetCode.SUCCESS).setMsg("上传成功").setData(url);
} else {
return new Result<>().setCode(RetCode.FAIL).setMsg("类型不支持");
}
}
}
return new Result<>().setCode(RetCode.FAIL).setMsg("上传文件为空");
}
}