Description:
Given the root of a binary tree with N nodes, each node in the tree has node.val coins, and there are N coins total.
In one move, we may choose two adjacent nodes and move one coin from one node to another. (The move may be from parent to child, or from child to parent.)
Return the number of moves required to make every node have exactly one coin.
Example 1:
Input: [3,0,0]
Output: 2
Explanation: From the root of the tree, we move one coin to its left child, and one coin to its right child.
Example 2:
Input: [0,3,0]
Output: 3
Explanation: From the left child of the root, we move two coins to the root [taking two moves]. Then, we move one coin from the root of the tree to the right child.
Example 3:
Input: [1,0,2]
Output: 2
Example 4:
Input: [1,0,0,null,3]
Output: 4
Note:
1. 1<= N <= 100
2. 0 <= node.val <= N**
Analysis:
从叶子结点开始自底向上分析,保证每个结点都能获得1枚硬币。
- 如果一个结点拥有的硬币数量大于1,那么它将向1它的父结点贡献
node.val - 1
枚硬币,同时move的数量增加Math.abs(node.val - 1)
。 - 如果一个结点拥有的硬币数量等于1,那么它保持不变,当然也可以理解为向它的父结点贡献
node.val - 1
枚硬币,同时move的数量增加Math.abs(node.val - 1)
。 - 如果一个结点拥有的硬币数量小于1, 那么它的父结点将向它传送
1 - node.val
枚硬币,设父结点一定可以满足子结点的要求,即使父结点会处于一种欠账(赊账、借款、贷款)状态,即parentNode.val < 0
。因为父结点为了满足子节点的要求,受到了node.val - 1
的硬币损失, 所以我们也可以反向理解为子结点向父结点传送了node.val - 1
枚硬币,同时move的数量增加Math.abs(node.val - 1)
。
即在这套解法中,子结点总是通过向父结点传送node.val - 1
枚硬币以确保自己拥有1枚硬币,同时使得move的数量增加Math.abs(node.val - 1)
。
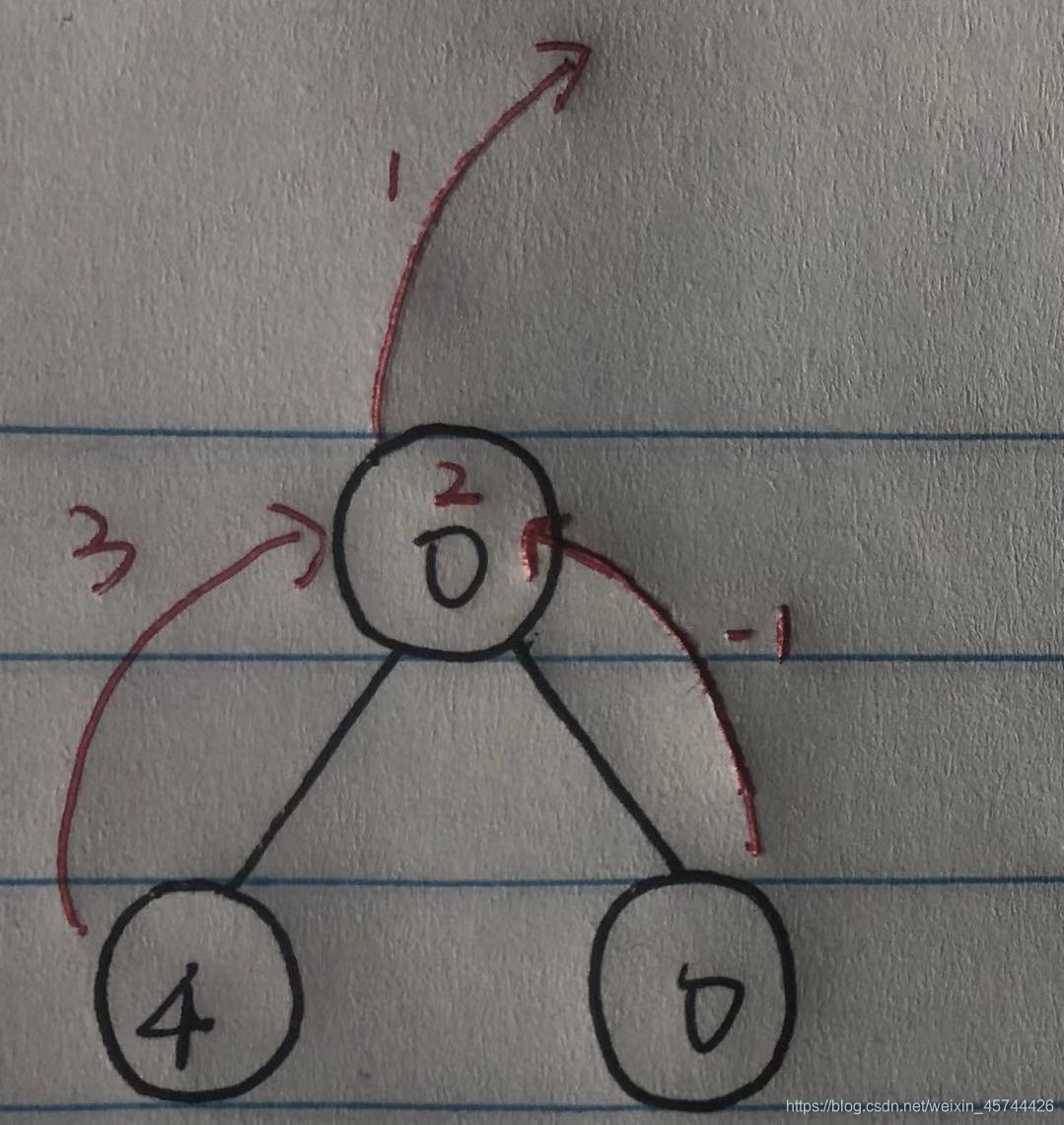
如图所示,一个结点在处理完它的两个子结点之后,拥有的硬币数量为node.val = (node.left.val - 1) + (node.right.val -1)
。同理,它将向它的父结点传送node.val - 1
枚硬币,并使得move的数量增加Math.abs(node.val - 1)
。重复这一过程,直到根结点。根结点在处理完它的子结点之后,会自动实现node.val = 1
并且不需要再做硬币移动。
以上过程可以考虑使用DFS来实现。
Solution:
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
private int move;
public int distributeCoins(TreeNode root) {
dfs(root);
return move;
}
public int dfs(TreeNode node) {
if(node == null) {
return 0;
}else{
if(node.left == null && node.right == null) {
move += Math.abs(node.val - 1);
return node.val - 1;
}else{
if(node.left != null) {
node.val += dfs(node.left);
}
if(node.right != null) {
node.val += dfs(node.right);
}
move += Math.abs(node.val - 1);
return node.val - 1;
}
}
}
}