1、简介
2、示例
2.1 引入依赖
<dependency>
<groupId>com.github.librepdf</groupId>
<artifactId>openpdf</artifactId>
<version>1.3.34</version>
</dependency>
<dependency>
<groupId>com.github.librepdf</groupId>
<artifactId>openpdf-fonts-extra</artifactId>
<version>1.3.34</version>
</dependency>
2.2 代码
2.2.1 主程序
String filePath = "/Users/xingyu/Documents/tmp/a.pdf";
FileOutputStream fos = new FileOutputStream(filePath);
PdfWriter pdfWriter = null;
Document document = null;
try {
document = new Document();
document.setPageSize(PageSize.A4);
pdfWriter = PdfWriter.getInstance(document, fos);
document.open();
Image rightTopIcon = getRightTopIcon("4");
document.add(rightTopIcon);
Paragraph titleParagraph = createParagraph("新模版002", FONT_TITLE, Element.ALIGN_CENTER, 0);
document.add(titleParagraph);
ProcessPdfVO processPdf = new ProcessPdfVO();
processPdf.setCompanyName("xxx公司");
processPdf.setCreateTime("2024-05-27");
processPdf.setProcessId("202405270004");
processPdf.setPrintDateTime("2024-05-28 17:25:09");
processPdf.setPrinter("张三");
PdfPTable firstRow = createFirstRow(processPdf);
document.add(firstRow);
PdfPTable mainTable = createMainTable();
PdfPCell cell = new PdfPCell(createParagraph("审批流程", FONT_MAIN_TABLE, Element.ALIGN_CENTER, 0));
cell.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
cell.setVerticalAlignment(PdfPCell.ALIGN_MIDDLE);
mainTable.addCell(cell);
PdfPTable subTable = new PdfPTable(2);
subTable.setWidths(new float[]{30,70});
subTable.setWidthPercentage(100);
PdfPCell subCell1 = new PdfPCell(createParagraph("subCell1", FONT_MAIN_TABLE, Element.ALIGN_CENTER, 0));
subCell1.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
subCell1.setVerticalAlignment(PdfPCell.ALIGN_MIDDLE);
subCell1.setBorder(Cell.BOX);
subTable.addCell(subCell1);
PdfPCell subCell2 = new PdfPCell(createParagraph("subCell2", FONT_MAIN_TABLE, Element.ALIGN_CENTER, 0));
subCell2.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
subCell2.setBorder(Cell.BOX);
subTable.addCell(subCell2);
PdfPCell subCell3 = new PdfPCell(createParagraph("subCell3", FONT_MAIN_TABLE, Element.ALIGN_CENTER, 0));
subCell3.setBorder(Cell.BOX);
subCell3.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
subTable.addCell(subCell3);
PdfPCell subCell4 = new PdfPCell(createParagraph("subCell4", FONT_MAIN_TABLE, Element.ALIGN_CENTER, 0));
subCell4.setBorder(Cell.BOX);
subCell4.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
subTable.addCell(subCell4);
subTable.setComplete(true);
PdfPCell subCell5 = new PdfPCell();
subCell5.setBorder(Cell.BOX);
subCell5.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
subCell5.setPadding(0);
subCell5.addElement(subTable);
mainTable.addCell(subCell5);
mainTable.addCell(new PdfPCell(createParagraph("审批流程1", FONT_MAIN_TABLE, Element.ALIGN_CENTER, 0)));
mainTable.addCell(new PdfPCell(createParagraph("审批流程2", FONT_MAIN_TABLE, Element.ALIGN_CENTER, 0)));
mainTable.addCell(new PdfPCell(createParagraph("审批流程3", FONT_MAIN_TABLE, Element.ALIGN_CENTER, 0)));
mainTable.addCell(subCell5);
mainTable.setComplete(true);
document.add(mainTable);
PdfPTable lastTable = createLastTable(processPdf);
document.add(lastTable);
} finally {
IoUtil.close(document);
IoUtil.close(pdfWriter);
IoUtil.close(fos);
}
2.2.2 表格
private static PdfPTable createMainTable() {
PdfPTable table = new PdfPTable(2);
float[] width = getUnitValues(table.getNumberOfColumns(), new float[]{30f, 70f});
table.setWidths(width);
return table;
}
private static float[] getUnitValues(int columnNum, float[] percents) {
float[] unitValues = new float[columnNum];
for (int i = 0; i < columnNum; i++) {
float percentValue;
if (Objects.nonNull(percents) && columnNum == percents.length) {
percentValue = percents[i];
} else {
percentValue = BigDecimal.valueOf(100)
.divide(BigDecimal.valueOf(columnNum), 10, RoundingMode.HALF_UP)
.floatValue();
}
unitValues[i] = percentValue;
}
return unitValues;
}
2.2.3 单元格
private static PdfPCell createCell(Integer horizontalAlignment, Integer verticalAlignment, Integer border,
Color borderColor) {
PdfPCell cell = new PdfPCell();
if (Objects.nonNull(horizontalAlignment)) {
cell.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
}
if (Objects.nonNull(verticalAlignment)) {
cell.setVerticalAlignment(PdfPCell.ALIGN_MIDDLE);
}
if (Objects.nonNull(border)) {
cell.setBorder(border);
}
if (Objects.nonNull(borderColor)) {
cell.setBorderColor(borderColor);
}
return cell;
}
2.2.4 创建单元格
private static PdfPCell createCell(Integer horizontalAlignment, Integer verticalAlignment, Integer border,
Color borderColor) {
PdfPCell cell = new PdfPCell();
if (Objects.nonNull(horizontalAlignment)) {
cell.setHorizontalAlignment(PdfPCell.ALIGN_CENTER);
}
if (Objects.nonNull(verticalAlignment)) {
cell.setVerticalAlignment(PdfPCell.ALIGN_MIDDLE);
}
if (Objects.nonNull(border)) {
cell.setBorder(border);
}
if (Objects.nonNull(borderColor)) {
cell.setBorderColor(borderColor);
}
return cell;
}
2.2.5 图片
private static Image getRightTopIcon(String processStatus) {
Image image = null;
ProcessStatusEnum processStatusEnum = ProcessStatusEnum.getEnum(processStatus);
if (Objects.isNull(processStatusEnum)) {
return image;
}
if (StringUtils.isBlank(processStatusEnum.getIcon())) {
return image;
}
String iconPath = String.format(Locale.ROOT, "%s%s%s", "images", File.separator, processStatusEnum.getIcon());
InputStream is = null;
ByteArrayOutputStream bos = null;
try {
ClassPathResource classPathResource = new ClassPathResource(iconPath);
is = classPathResource.getInputStream();
bos = new ByteArrayOutputStream();
IoUtil.copy(is, bos);
is.close();
image = Image.getInstance(bos.toByteArray());
image.scalePercent(50);
image.setAbsolutePosition(500,700);
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
IoUtil.close(is);
IoUtil.close(bos);
}
return image;
}
2.2.6 预览图
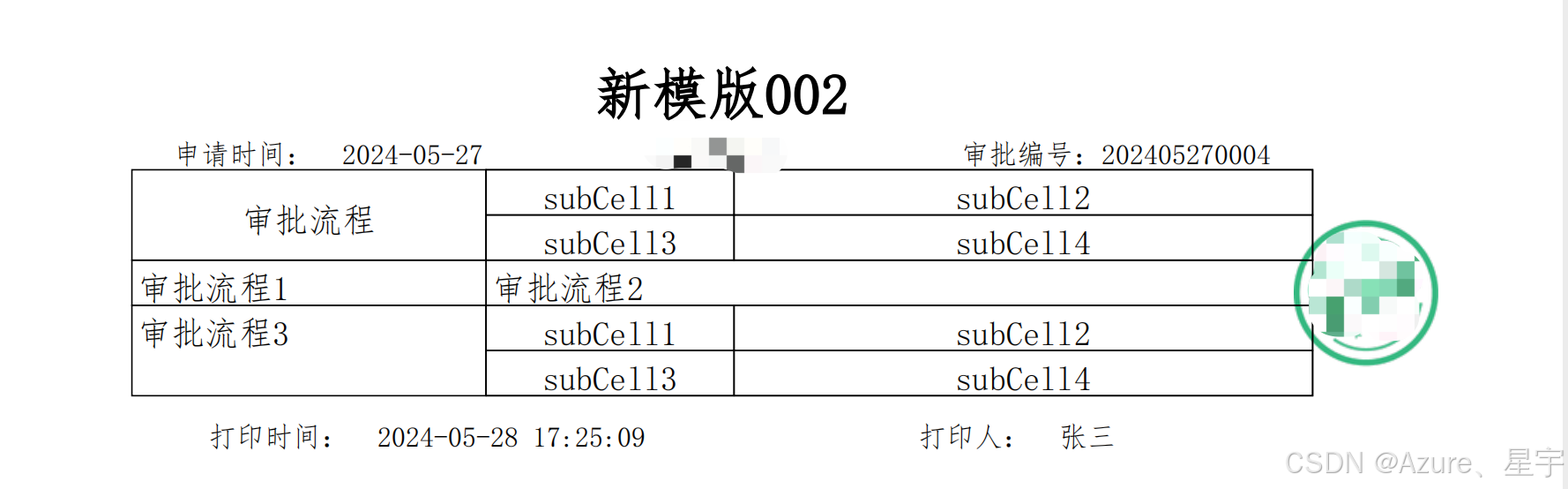