python sklearn one-hot
"""
数据预处理 独热编码
"""
import numpy as np
import sklearn.preprocessing as sp
samples = np.array([
[1, 3, 2],
[7, 5, 4],
[1, 8, 6],
[7, 3, 9]
])
ohe = sp.OneHotEncoder(sparse=False, dtype="int32")
result = ohe.fit_transform(samples)
print(result)
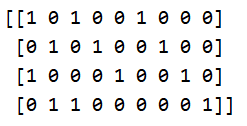
python sklearn 标签编码
"""
标签编码器
"""
import numpy as np
import sklearn.preprocessing as sp
raw_samples = np.array(["audi", "ford", "audi", "toyota",
"ford", "bmw", "ford", "redflag", "audi"])
print(raw_samples)
lbe = sp.LabelEncoder()
result = lbe.fit_transform(raw_samples)
print("-----编码后\n", result)
test = [0, 0, 1, 1, 4]
print("-----编码反推\n", lbe.inverse_transform(test))
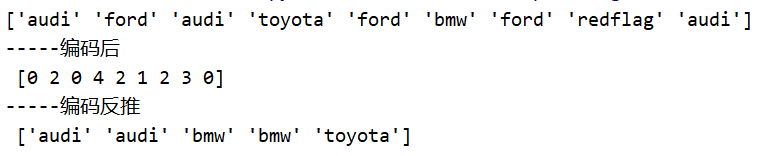