交叉熵计算(分类)
"""
分类问题 损失函数的计算
"""
import tensorflow as tf
import numpy as np
print("普通函数 计算交叉熵", "--"*30)
a = tf.fill([4], 0.25)
b = - tf.reduce_sum(a * tf.math.log(a) / tf.math.log(2.0))
print(a.numpy(), "--->", b.numpy())
a = tf.constant([0.1, 0.1, 0.1, 0.7])
b = - tf.reduce_sum(a * tf.math.log(a) / tf.math.log(2.0))
print(a.numpy(), "--->", b.numpy())
a = tf.constant([0.01, 0.01, 0.01, 0.97])
b = - tf.reduce_sum(a * tf.math.log(a) / tf.math.log(2.0))
print(a.numpy(), "--->", b.numpy())
print("使用函数计算交叉熵")
y_true = np.array([0, 1, 0, 0])
y_predict1 = np.array([0.25, 0.25, 0.25, 0.25])
y_predict2 = np.array([0.1, 0.1, 0.7, 0.1])
y_predict3 = np.array([0.1, 0.7, 0.1, 0.1])
y_predict4 = np.array([0.01, 0.97, 0.01, 0.01])
loss1 = tf.losses.categorical_crossentropy(y_true, y_predict1)
loss2 = tf.losses.categorical_crossentropy(y_true, y_predict2)
loss3 = tf.losses.categorical_crossentropy(y_true, y_predict3)
loss4 = tf.losses.categorical_crossentropy(y_true, y_predict4)
print("y_true:", y_true)
print(y_predict1, "--->", loss1.numpy())
print(y_predict2, "--->", loss2.numpy())
print(y_predict3, "--->", loss3.numpy())
print(y_predict4, "--->", loss4.numpy())
criten = tf.losses.CategoricalCrossentropy()
loss5 = criten(y_true, y_predict3)
print("类的调用法:\n",
y_predict3, "--->", loss5.numpy())
loss6 = criten([0, 1], [0.9, 0.1])
print(loss6)
print("二分类交叉熵计算\n", tf.losses.BinaryCrossentropy()([1], [0.1]))
print(tf.losses.binary_crossentropy([1], [0.1]))
x = tf.random.normal([1, 784])
w = tf.random.normal([784, 2])
b = tf.zeros([2])
logits = x @ w + b
print("模拟真实情况-------------------------------")
print("计算结果:", logits.numpy())
prob = tf.math.softmax(logits, axis=1)
print("softmax处理后:", prob.numpy())
logits = tf.reshape(logits, shape=(logits.shape[1],))
prob = tf.reshape(prob, shape=(prob.shape[1],))
loss1 = tf.losses.categorical_crossentropy([0, 1], logits, from_logits=True)
loss2 = tf.losses.categorical_crossentropy([0, 1], prob)
print("损失结果:", loss1.numpy())
print("损失结果:", loss2.numpy())
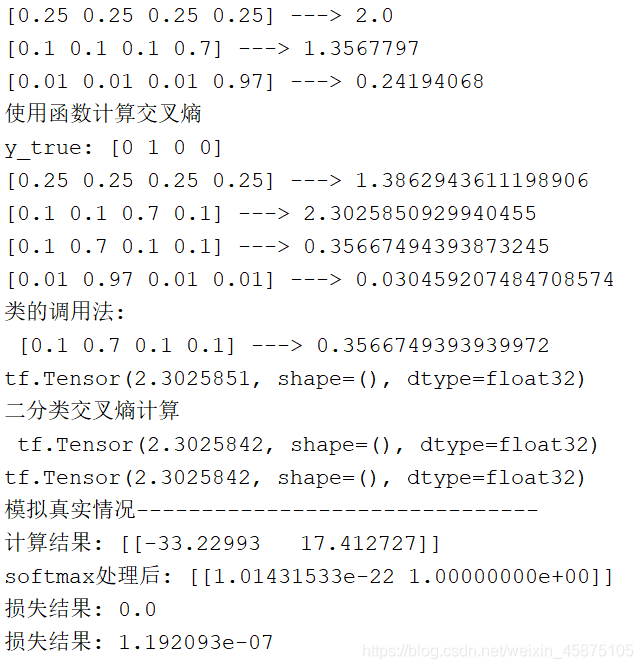
均方误差计算(回归问题)
"""
MSE 均方差损失
"""
import tensorflow as tf
y = tf.constant([1, 2, 3, 0, 2])
y = tf.one_hot(y, depth=4)
y = tf.cast(y, dtype=tf.float32)
print("真实值:\n", y.numpy())
out = tf.random.normal([5, 4])
print("out:\n", out.numpy())
loss1 = tf.reduce_mean(tf.square(y - out))
loss2 = tf.square(tf.norm(y - out)) / (5.0 * 4.0)
loss3 = tf.reduce_mean(tf.losses.MSE(y, out))
print("普通函数:", loss1.numpy())
print("范数计算:", loss2.numpy())
print("MSE函数:", loss3.numpy())
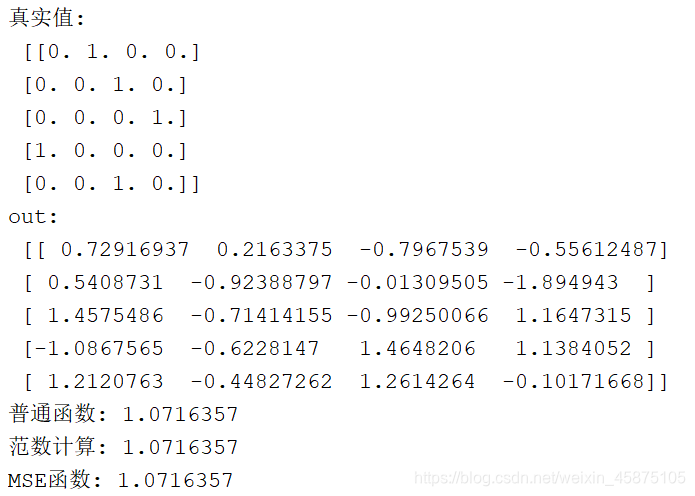