python之Pandas
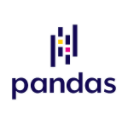
"""
Created on Wed Dec 22 20:40:41 2021
@author: 阿磊很努力
summary:Pandas study
"""
import pandas as pd
df = pd.read_csv(r'C:\Users\Lenlovo\Desktop\pandas\pokemon_data.csv')
b=df.head(3)
c=df.tail(3)
print(df.head(3))
print(df.tail(3))
df_xlsx = pd.read_excel('pokemon_data.xlsx')
e = df_xlsx.head(3)
print(e)
df_txt = pd.read_csv('pokemon_data.txt',delimiter='\t')
print(df_txt.head(3))
print(df.columns)
print(df['Name'])
print(df.Name)
print(df['Name'][0:5])
print(df.head(3))
print(df.iloc[1])
print(df.iloc[1:5])
print(df.iloc[3,1])
for index, row in df.iterrows():
print(index)
print(row)
df.loc[df['Type 1'] == 'Fire']
df.describe()
df.sort_values('Name')
df.sort_values('Name',ascending=False)
df.sort_values(['Type 1','Speed'],ascending=[1,0])
df['Total'] = df['HP']+df['Attack']+df['Defense']+df['Sp. Atk']+df['Sp. Def']+df['Speed']
df.head(5)
df = df.drop(columns=['Total'])
df.head(5)
df['Total'] = df.iloc[:,4:10].sum(axis=1)
cols = list(df.columns)
df = df[cols[0:4] + [cols[-1]]+cols[4:12]]
df.to_csv(r'C:\Users\Lenlovo\Desktop\pandas\modified.csv',index = False)
df.to_excel(r'C:\Users\Lenlovo\Desktop\pandas\modified.xlsx',index = False)
df.to_csv(r'C:\Users\Lenlovo\Desktop\pandas\modified.txt',index = False,sep='\t')
df.loc[df['Type 1'] == 'Grass']
df.loc[(df['Type 1'] == 'Grass')& (df['Type 2'] == 'Poison')]
new_df = df.loc[(df['Type 1']=='Grass') & (df['Type 2']=='Poison') & (df['HP']>70)]
new_df.to_csv(r'C:\Users\Lenlovo\Desktop\pandas\Filtered.csv',index = False)
new_df = new_df.reset_index()
new_df = new_df.reset_index(drop = True)
new_df = new_df.reset_index(drop = True,inplace = True)
df.loc[df['Name'].str.contains('Mega')]
df.loc[~df['Name'].str.contains('Mega')]
import re
df.loc[df['Type 1'].str.contains('fire|grass',flags = re.I,regex = True)]
df.loc[df['Name'].str.contains('^pi[a-z]',flags = re.I,regex = True)]
df.loc[df['Type 1']=='Fire','Type 1']='Flamer'
df.loc[df['Type 1']=='Fire','Legendary']='True'
df.loc[df['Total']>500,['Generation','Legendary']]=['Test 1','Test 2']
df.groupby(['Type 1']).mean()
df.groupby(['Type 1']).mean().sort_values('HP',ascending = False)
df.groupby(['Type 1']).sum()
df.groupby(['Type 1']).count()
df['count'] = 1
df.groupby(['Type 1']).count()['count']
df.groupby(['Type 1','Type 2']).count()['count']
df = pd.read_csv('modified.csv')
for df in pd.read_csv('modified.csv',chunksize = 5):
print('chunk DF')
print(df)
new_df = pd.DataFrame(columns = df.columns)
for df in pd.read_csv('modified.csv',chunksize = 5):
results = df.groupby(['Type 1']).count()
new_df=pd.concat([new_df,results])