# 5.1 一个简单的示例
cars = ['auti','bmw','subaru','toyota'] #bmw全大写,其他首字母大写
for car in cars:
if car == 'bmw':
print(car.upper())
else:
print(car.title())
# 5.2 条件测试
True False
## 5.2.1 检查是否相等
car = 'bmw'
car == 'bmw'
car = 'audi'
car == 'bmw'
## 5.2.2 检查是否相等时忽略大小写
car = 'Audi'
car == "audi"
car = 'Audi'
car.lower() == 'audi' #变为小写,lower不会去改变值,暂时修改
car
## 5.2.3 检查是否不相等
要判断两个值是否不相等,可结合使用惊叹号和等号!=
requested_topping = 'mushrooms'
if requested_topping != 'anchovies':
print("Hold the anchovies!")
## 5.2.4 数值比较
age = 18
age == 18
answer = 17
if answer != 42:
print("asx")
age = 19
age < 21
age = 19
age <= 21
age = 19
age > 21
## 5.2.5 检查多个条件
### 1,使用and检查多个条件
age_0 = 22
age_1 = 18
age_0 >= 21 and age_1 >=21
age_0 = 22
age_1 = 23
(age_0 >= 21) and (age_1 >=21)
### 2,使用or检查多个条件
age_0 = 22
age_1 = 18
age_0 >= 21 or age_1 >=21
age_0 = 22
age_1 = 18
age_0 = 18
age_0 >= 21 or age_1 >=21
## 5.2.6 检查特定值是否包含在列表中 in
requested_toppings = ['mushrooms','onions','pineapple']
'mushrooms' in requested_toppings
'dgd' in requested_toppings
## 5.2.7 检查特定值是否不包含在列表中 not in
banned_users = ['andrew','carolina','david']
user = 'marie'
if user not in banned_users:
print(f"{user.title()},you can post a response if you can")
## 5.2.8 布尔表达式
game_active = True
can_edit = False
# 5.3 if 语句
## 5.3.1 简单的if语句
if conditional_text:
do something
如果年龄大于等于18,打印:
You are old enough to vote!
age = 19
if age >= 18 :
print("You are old enough to vote!")
如果年龄大于等于18,打印:
You are old enough to vote!
Have you registered to vote yet?
age = 19
if age >= 18 :
print("You are old enough to vote!")
print("\nHave you registered to vote yet?")
## 5.3.2 if-else 语句
如果年龄大于等于18,打印:
You are old enough to vote!
Have you registered to vote yet?
如果年龄小于18,打印:
Sorry,you are too young to vote.
Please register to vote as soon as you turn 18!
age = 17
if age >= 18 :
print("You are old enough to vote!")
print("\nHave you registered to vote yet?")
else:
print("Sorry,you are too young to vote.\nPlease register to vote as soon as you turn 18!")
## 5.3.3 if-elif-else 结构
根据年龄段收费的游乐场:
4岁以下免费;
4~18岁收费25美元;$
18岁(含)以上收费40美元
age = 12
if age < 4:
print("You admission cost is $0.")
elif age <18:
print("You admission cost is $25.")
else:
print("You admission cost is $40.")
age = 12
if age < 4:
price = 0
elif age <18:
price = 25
else:
price=40
print(f"You admission cost is ${price}")
## 5.3.4 使用多个elif 代码块
假设前述游乐场要给老人打折,可再添加一个测试条件,判断顾客是否符合打折条件:
下面假设对于65岁(含)以上的老人,可半价(即20美元)购买门票
根据年龄段收费的游乐场:
4岁以下免费;
4~18岁收费25美元;$
18岁(含)以上收费40美元
65岁(含)以上收费20美元
age = 75
if age < 4:
price = 0
elif age <18:
price = 25
elif age <65 :
price = 40
else:
price = 20
print(f"You admission cost is ${price}")
## 5.3.5 省略else代码块
age = 75
if age < 4:
price = 0
elif age <18:
price = 25
elif age <65 :
price = 40
price = 20
print(f"You admission cost is ${price}")
## 5.3.6 测试多个条件
# 5.4 使用if语句处理列表
## 5.4.1 检查特殊元素
披萨配料:mushrooms,'gree peppers','extra cheese
使用for 语句循环告诉用户需要陆续添加到披萨的3种配料才能完成披萨制作
Adding xx配料
Adding xx配料
Adding xx配料
完成你的披萨啦!
requested_toppings = ['mushrooms','gree peppers','extra cheese']
for requested_topping in requested_toppings:
print(f"Adding {requested_topping}.")
print("\n完成你的披萨啦!")
青椒用完了,告诉用户不能添加青椒的原因
requested_toppings = ['mushrooms','gree peppers','extra cheese']
for requested_topping in requested_toppings:
if requested_topping == "gree peppers":
print("Sorry,we are out of gree peppers ! ")
else:
print(f"Adding {requested_topping}.")
print("\n完成你的披萨啦!")
## 5.4.2 确定列表不是空的
在制作披萨前检查顾客点的配料列表是否为空。
如果列表为空,就像顾客确认是否要点原味披萨
如果列表不为空,就像前面的示例那样制作披萨
requested_toppings = []
if requested_toppings: #列表为空,返回false;有一个元素返回True
for requested_topping in requested_toppings:
print(f"Adding {requested_topping}.")
print("\n完成你的披萨啦!")
else:
print("Are you sure you want a plaint pazza ?")
## 5.4.3 使用多个列表
available_topping = ['mushrooms','gree peppers','extra cheese',
'pepperoni','pineapple','extra cheese']
requested_toppings = ['mushrooms','french ','extra cheese']
for requested_topping in requested_toppings:
if requested_topping in available_topping: #循环检查点单是否都在库存里面
print(f"Adding {requested_topping}.")
else:
print(f"Sorry,we are out of {requested_topping} ! ")
print("\n完成你的披萨啦!")
# 5.5 设置if语句格式
python基础:四,if语句
于 2023-07-15 21:57:51 首次发布
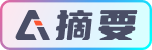