一、构造函数
1.1特性
构造函数是特殊的成员函数,需要注意的是,构造函数的虽然名称叫构造,但是需要注意的是构造函数的主要任务并不是开空间创建对象,而是初始化对象。
1. 函数名与类名相同。2. 无返回值。3. 对象实例化时编译器自动调用对应的构造函数。4. 构造函数可以重载。
class Date
{
public :
// 1.无参构造函数
Date ()
{}
// 2.带参构造函数
Date (int year, int month , int day )
{
_year = year ;
_month = month ;
_day = day ;
}
private :
int _year ;
int _month ;
int _day ;
};
void TestDate()
{
Date d1; // 调用无参构造函数
Date d2 (2015, 1, 1); // 调用带参的构造函数
// 注意:如果通过无参构造函数创建对象时,对象后面不用跟括号,否则就成了函数声明
// 以下代码的函数:声明了d3函数,该函数无参,返回一个日期类型的对象
Date d3();
}
如果类中没有显式定义构造函数,则C++编译器会自动生成一个无参的默认构造函数,一旦用户显式定义编译器将不再生成。
class Date
{
public:
private:
int _year;
int _month;
int _day;
};
void Test()
{
// 没有定义构造函数,对象也可以创建成功,因此此处调用的是编译器生成的默认构造函数
//对_year、_month、_day赋随机值
Date d;
}
无参的构造函数和全缺省的构造函数都称为默认构造函数,并且默认构造函数只能有一个。
class Date
{
public:
Date()
{}
Date (int year = 1900, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
private :
int _year ;
int _month ;
int _day ;
};
// 以下测试函数不能通过编译
void Test()
{
Date d1; //对d1赋初始值时,不知道该用全缺省还是随机值,冲突
}
二、析构函数
2.1定义
与构造函数功能相反,析构函数不是完成对象的销毁,局部对象销毁工作是由编译器完成的。而
对象在销毁时会自动调用析构函数,完成类的一些资源清理工作。
2.2特性
1. 析构函数名是在类名前加上字符 ~。2. 无参数无返回值。3. 一个类有且只有一个析构函数。若未显式定义,系统会自动生成默认的析构函数。4. 对象生命周期结束时,C++编译系统系统自动调用析构函数。
typedef int DataType;
class SeqList
{
public :
SeqList (int capacity = 10)
{
_pData = (DataType*)malloc(capacity * sizeof(DataType));
assert(_pData);
_size = 0;
_capacity = capacity;
}
~SeqList()
{
if (_pData)
{
free(_pData ); // 释放堆上的空间
_pData = NULL; // 将指针置为空
_capacity = 0;
_size = 0;
}
}
private :
int* _pData ;
size_t _size;
size_t _capacity;
};
编译器生成的默认析构函数,对会自定类型成员调用它的析构函数。
class String
{
public:
String(const char* str = "jack")
{
_str = (char*)malloc(strlen(str) + 1);
strcpy(_str, str);
}
~String()
{
cout << "~String()" << endl;
free(_str);
}
private:
char* _str;
};
class Person
{
private:
String _name;
int _age;
};
int main()
{
Person p;
return 0;
}
三、拷贝构造函数
3.1特性
1. 拷贝构造函数是构造函数的一个重载形式。2. 拷贝构造函数的参数只有一个且必须使用引用传参,使用传值方式会引发无穷递归调用。
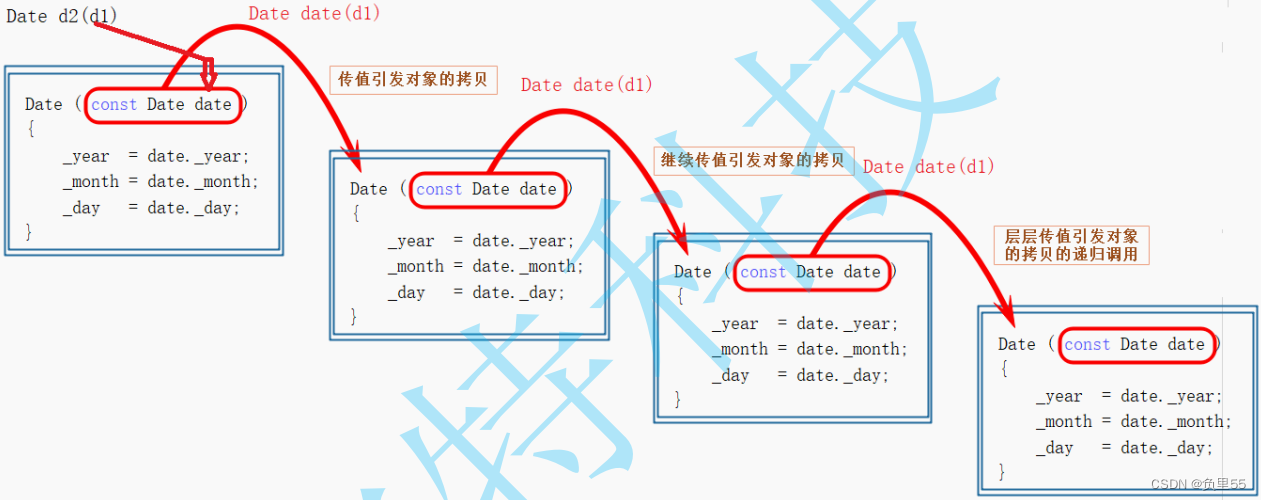
class Date
{
public:
Date(int year = 1900, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
Date(const Date& d)
{
_year = d._year;
_month = d._month;
_day = d._day;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1;
Date d2(d1); //d2是d1的拷贝
return 0;
}
3.2
若未显示定义,系统生成默认的拷贝构造函数。 默认的拷贝构造函数对象按内存存储按字节序完成拷贝,这种拷贝我们叫做浅拷贝,或者值拷贝。
class Date
{
public:
Date(int year = 1900, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1;
// 这里d2调用的默认拷贝构造完成拷贝,d2和d1的值也是一样的。
//浅拷贝,只是将d2的指向了d1所在的位置,&d1=&d2
Date d2(d1); //等价于Date d2 = d1;
return 0;
}
四、赋值运算符重载
4.1定义
C++为了增强代码的可读性引入了运算符重载,运算符重载是具有特殊函数名的函数,也具有其返回值类型,函数名字以及参数列表,其返回值类型与参数列表与普通的函数类似。
函数名字为:关键字operator后面接需要重载的运算符符号。函数原型:返回值类型 operator操作符(参数列表)运算符重载是针对自定义类型,对于内置类型,编译器可以直接对他们做等于、大于小于等运算;对于自定义类型,需要利用运算符重载。
4.2特性
1.不能通过连接其他符号来创建新的操作符:比如operator@;2.重载操作符必须有一个类类型或者枚举类型的操作数;3.用于内置类型的操作符,其含义不能改变,例如:内置的整型+,不 能改变其含义;4.作为类成员的重载函数时,其形参看起来比操作数数目少1成员函数的操作符有一个默认的形参this,限定为第一个形参;5. .* 、:: 、sizeof 、?: 、. 注意以上5个运算符不能重载。这个经常在笔试选择题中出现。
4.3 operator放全局和放入类中
// 全局的operator==,但要注意private
class Date
{
public:
Date(int year = 1900, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
private:
int _year;
int _month;
int _day;
};
// 这里会发现运算符重载成全局的就需要成员变量是共有的,无法访问private中的
bool operator==(const Date& d1, const Date& d2)
{
return d1._year == d2._year;
&& d1._month == d2._month
&& d1._day == d2._day;
}
void Test ()
{
Date d1(2018, 9, 26);
Date d2(2018, 9, 27);
cout<<(d1 == d2)<<endl; //->operator==(d1,d2)
}
//operator==放入类中
class Date
{
public:
Date(int year = 1900, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
// bool operator==(Date* this, const Date& d2)
// 这里需要注意的是,左操作数是this指向的调用函数的对象
bool operator==(const Date& d2)
{
return _year == d2._year
&& _month == d2._month
&& _day == d2._day;
}
private:
int _year;
int _month;
int _day;
};
void Test ()
{
Date d1(2018, 9, 26);
Date d2(2018, 9, 27);
cout<<(d1 == d2)<<endl; //->d1.operator==(&d1,d2)->d1.operator==(d2)
}
4.4大于运算符重载
class Date
{
public:
Date(int year, int month, int day)
{
_year = year;
_month = month;
_day = day;
}
//大于
bool operator>(const Date& d2)
{
if (_year > d2._year)
return true;
else if (_year == d2._year && _month > d2._month)
return true;
else if (_year == d2._year && _month == d2._month && _day > d2._day)
return true;
else
return false;
}
private:
int _year;
int _month;
int _day;
};
int main()
{
Date d1(2015, 1, 2);
Date d2(2015, 1, 1);
cout << (d1 > d2) << endl;
return 0;
}
详见运算符实现一个完善日期类 C++:运算符重载实现一个完善日期类-CSDN博客
五、const成员
5.1 对象调用成员函数
将const修饰的类成员函数称之为const成员函数,const修饰类成员函数,实际修饰该成员函数隐含的this指针,表明在该成员函数中不能对类的任何成员进行修改。
class Date
{
public:
Date(int year = 0, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
void print()
{
cout<<_year<<"-"<<_month<<"-"<<_day<<endl;
}
private:
int _year;
int _month;
int _day;
};
void f(const Date& d)
{
d.print();
}
int main()
{
Date d1(2018, 9, 27);
f(d1); //报错
return 0;
}
&d的类型是const Date*,传到Print函数里的是Date*。
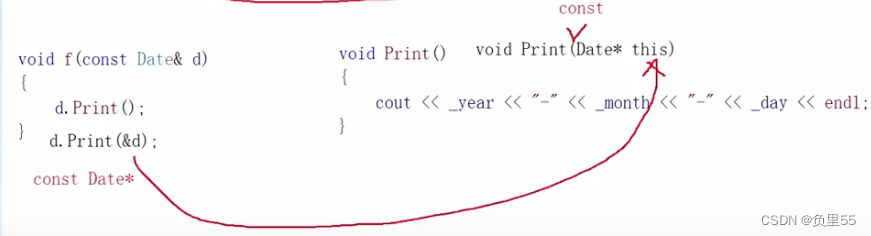
所以要在Print函数里的是Date*的前面加const,但this是默认不写的,又该如何处理?
将const放入括号外面。
p1和p2的写法没有区别。
5.2 成员函数调用成员函数
class Date
{
public:
Date(int year = 0, int month = 1, int day = 1)
{
_year = year;
_month = month;
_day = day;
}
void f1() //void f1(Date* this)
{
f2(); //可以 this->f2(this)
}
void f2() const
{}
//
void f3()
{}
void f4() const
{
f3(); //不可以
}
private:
int _year;
int _month;
int _day;
};
总结:
1. const对象可以调用非const成员函数吗? 不行2. 非const对象可以调用const成员函数吗? 可以3. const成员函数内可以调用其它的非const成员函数吗?不行4. 非const成员函数内可以调用其它的const成员函数吗?可以
六、取地址及const取地址操作符重载
class Date
{
public :
Date* operator&() //Date*
{
cout<<"operator&()"<<endl;
return this ;
}
const Date* operator&()const //cosnt Date*,与上面的构成函数重载
{
cout<<"operator&() const"<<endl;
return this ;
}
private :
int _year ;
int _month ;
int _day ;
};
int main()
{
Date d1;
Date d2;
const Date d3;
cout<<&d1<<endl;
cout<<&d2<<endl;
cout<<&d3<<endl;
}