一、相同的树:
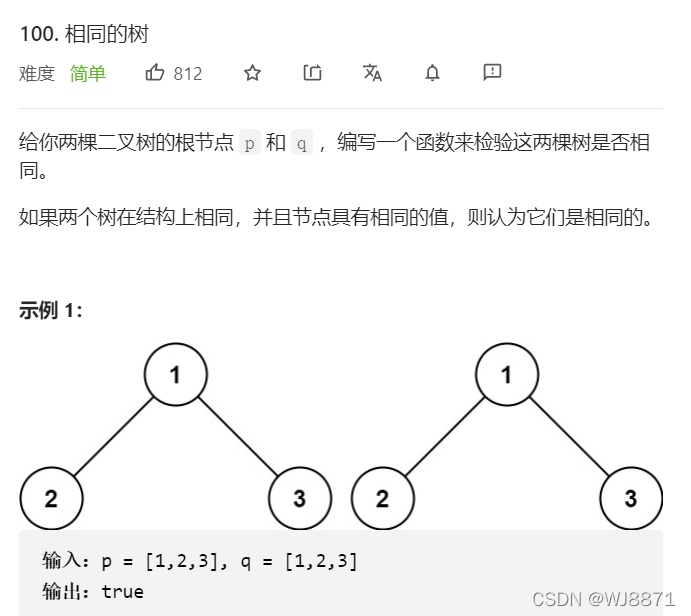
bool isSameTree(struct TreeNode* p, struct TreeNode* q){
if(p==NULL&&q==NULL)
{
return true;
}
if(p==NULL||q==NULL)
{
return false;
}
return p->val==q->val && isSameTree(p->left,q->left) && isSameTree(p->right,q->right);
}
二、对称二叉树:
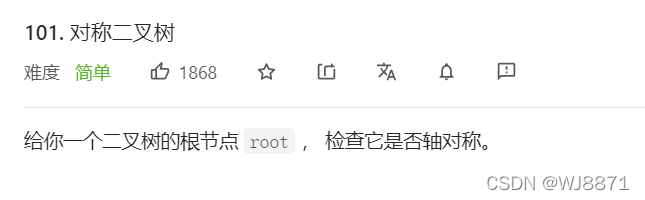
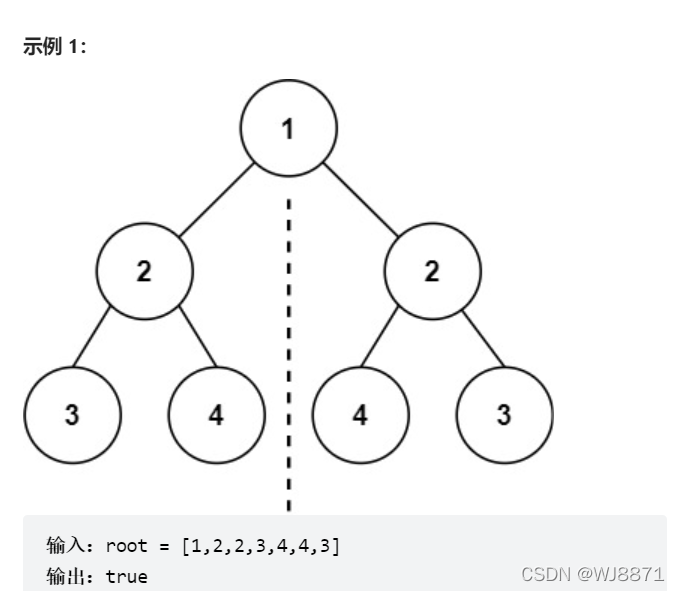
bool _isSymmertric(struct TreeNode*p,struct TreeNode*q)
{
if(p==NULL&&q==NULL)
{
return true;
}
if(p==NULL||q==NULL)
{
return false;
}
return p->val==q->val &&_isSymmertric(p->left,q->right) && _isSymmertric(p->right,q->left);
}
bool isSymmetric(struct TreeNode* root){
if(root==NULL)
{
return true;
}
//主要是检测root的左右子树
return _isSymmertric(root->left,root->right);
}
三、二叉树前序遍历:
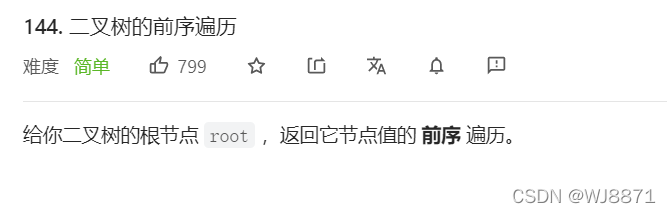
int getTreeSize(struct TreeNode*root)
{
if(root==NULL)
{
return 0;
}
return getTreeSize(root->left)+getTreeSize(root->right)+1;
}
void _preorderTraversal(struct TreeNode*root,int array[],int *index)
{
if(NULL==root)
{
return;
}
array[*index]=root->val;
*index+=1;
_preorderTraversal(root->left,array,index);
_preorderTraversal(root->right,array,index);
}
int* preorderTraversal(struct TreeNode* root, int* returnSize){
if(root==NULL)
{
*returnSize=0;
return NULL;
}
int size=getTreeSize(root);
int *array=(int*)malloc(sizeof(int)*size);
*returnSize=size;
int index=0;
_preorderTraversal(root,array,&index);
return array;
}
四 、二叉树中序遍历:
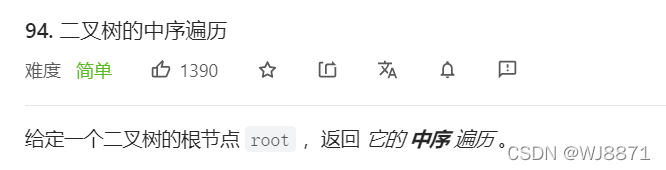
int getTreeSize(struct TreeNode* root)
{
if(root==NULL)
{
return 0;
}
return getTreeSize(root->left)+getTreeSize(root->right)+1;
}
void _inorderTraversal(struct TreeNode*root,int array[],int* index)
{
if(root==NULL)
{
return ;
}
_inorderTraversal(root->left,array,index);
array[*index]=root->val;
*index+=1;
_inorderTraversal(root->right,array,index);
}
int* inorderTraversal(struct TreeNode* root, int* returnSize){
if(root==NULL)
{
*returnSize=0;
return NULL;
}
int size=getTreeSize(root);
*returnSize=size;
int *array=(int*)malloc(sizeof(int)*size);
int index=0;
_inorderTraversal(root,array,&index);
return array;
}
五、二叉树后序遍历:
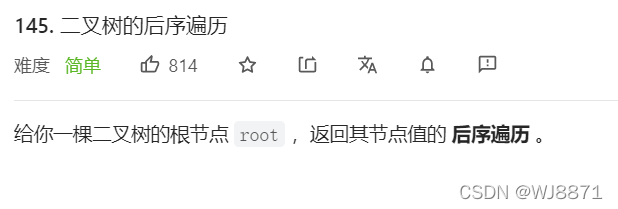
int getTreeSize(struct TreeNode*root)
{
if(root==NULL)
{
return 0;
}
return getTreeSize(root->left)+getTreeSize(root->right)+1;
}
void _postorderTraversal(struct TreeNode*root,int array[],int* index)
{
if(root==NULL)
{
return;
}
_postorderTraversal(root->left,array,index);
_postorderTraversal(root->right,array,index);
array[*index]=root->val;
*index+=1;
}
int* postorderTraversal(struct TreeNode* root, int* returnSize){
if(root==NULL)
{
*returnSize=0;
return NULL;
}
int size=getTreeSize(root);
int *array=(int*)malloc(sizeof(int)*size);
*returnSize=size;
int index=0;
_postorderTraversal(root,array,&index);
return array;
}
六、另一颗树的子树:
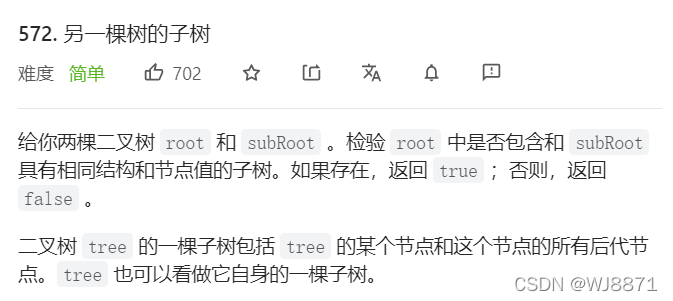
//判断是否是相同的树
bool isSameTree(struct TreeNode*p,struct TreeNode*q)
{
if(p==NULL&&q==NULL)
{
return true;
}
if(p==NULL||q==NULL)
{
return false;
}
return p->val==q->val&& isSameTree(p->left,q->left) &&isSameTree(p->right,q->right);
}
bool isSubtree(struct TreeNode* root, struct TreeNode* subRoot){
//都为空,自己是自己的子树
if(root==NULL&&subRoot==NULL)
{
return true;
}
//所有不为空的树都不是空树的子树
if(root==NULL)
{
return false;
}
//所有空树都是某树的子树
if(subRoot==NULL)
{
return true;
}
//两树相同的情况
if(root->val==subRoot->val&& isSameTree(root,subRoot))
{
return true;
}
//其它情况
return isSubtree(root->left,subRoot)||isSubtree(root->right,subRoot);
}
七、平衡二叉树:
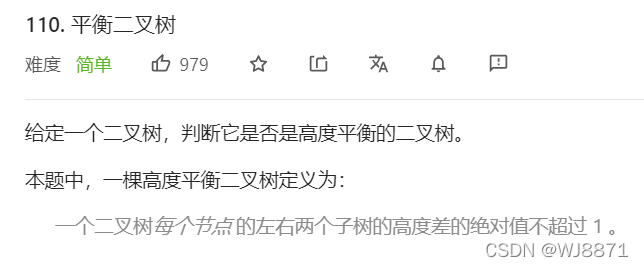
int height(struct TreeNode* root) {
if (root == NULL) {
return 0;
} else {
return fmax(height(root->left), height(root->right)) + 1;
}
}
bool isBalanced(struct TreeNode* root) {
if (root == NULL) {
return true;
} else {
return fabs(height(root->left) - height(root->right)) <= 1 && isBalanced(root->left) && isBalanced(root->right);
}
}
八、二叉树的构建及遍历:
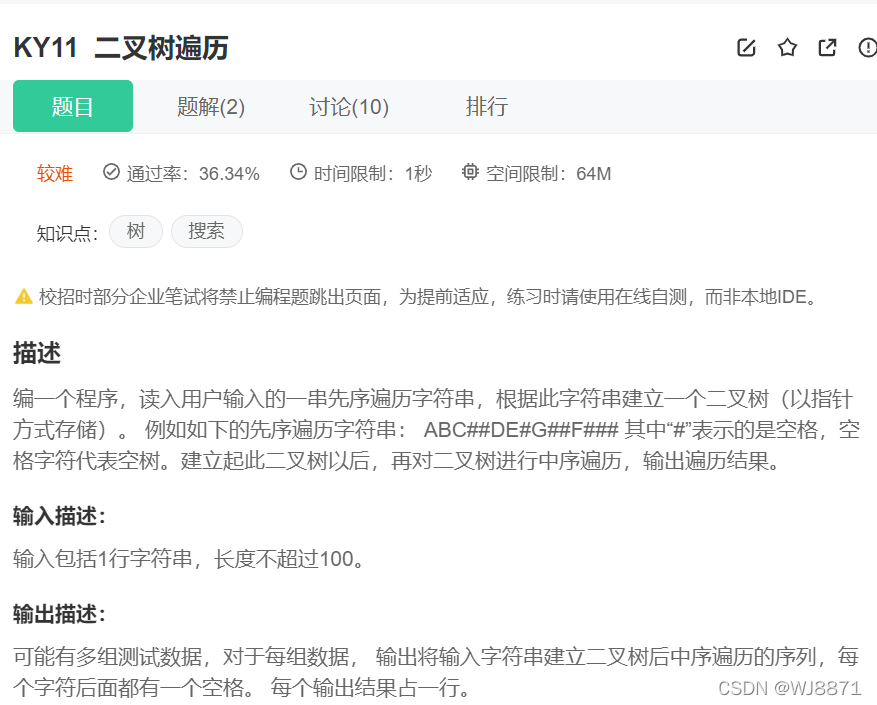
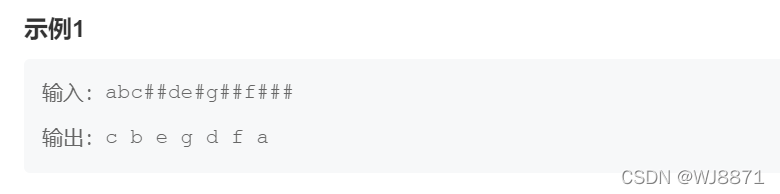
#include<stdio.h>
#include<stdlib.h>
typedef struct BinTreeNode
{
char val;
struct BinTreeNode* left;
struct BinTreeNode* right;
}BTNode;
BTNode* BinTreeCreate(char* str,int* i)
{
if(str[*i]=='#')
{
(*i)++;
return NULL;
}
BTNode* root=malloc(sizeof(BTNode));
root->val=str[*i];
(*i)++;
root->left=BinTreeCreate(str,i);
root->right=BinTreeCreate(str,i);
return root;
}
void InOrder(BTNode* root)
{
if(!root)
return;
InOrder(root->left);
printf("%c ",root->val);
InOrder(root->right);
}
int main()
{
char str[100]={0};
while(~scanf("%s",str))
{
int i=0;
BTNode* root=BinTreeCreate(str,&i);
InOrder(root);
}
return 0;
}