206. 反转链表

难度 2
题解
var reverseList = function(head) {
let pre = null, cur = head, temp = null;
while(cur){
temp = cur.next;
cur.next = pre;
pre = cur;
cur = temp
}
return pre;
};
思路
3. 无重复字符的最长子串

难度 2
题解1 set
var lengthOfLongestSubstring = function(s) {
let len = s.length;
let res = 0;
for(let i = 0;i<s.length;i++){
let set = new Set(), count = 0;
let j = i;
while(!set.has(s[j])&&j<s.length){
set.add(s[j]);
count++;
j++;
}
res = Math.max(res, count);
}
return res;
};
题解2 滑动窗口
var lengthOfLongestSubstring = function(s) {
let set = new Set();
let left = 0, right = left, max = 0;
while(left<s.length){
if(left!=0) set.delete(s[left-1]);
while(right<s.length&&!set.has(s[right])){
set.add(s[right]);
right++;
}
left++;
max = Math.max(max, right-left+1);
}
return max;
};
思路
滑动窗口比较有意思, 每次把左侧的去除
考虑 advda 3, 和 abb 2
146. LRU缓存机制

难度 2
题解 map
var LRUCache = function(capacity) {
this.map = new Map();
this.capacity = capacity;
};
LRUCache.prototype.get = function(key) {
if(this.map.has(key)){
let value = this.map.get(key);
this.map.delete(key)
this.map.set(key, value);
return value;
}
return -1;
};
LRUCache.prototype.put = function(key, value) {
if(this.map.has(key)){
this.map.delete(key)
}
this.map.set(key,value);
if(this.map.size>this.capacity){
this.map.delete(this.map.keys().next().value)
}
};
思路
215. 数组中的第K个最大元素
难度
题解1 快排
题解2 堆
思路
25. K 个一组翻转链表
难度
题解
思路
15. 三数之和
难度
题解
思路
补充题4. 手撕快速排序

难度 2
题解 快排
var sortArray = function(nums) {
if(nums.length<2) return nums;
let mid = Math.floor(nums.length * Math.random())
const cur = nums[mid];
const left = nums.filter((x,i)=> x<=cur && i!==mid);
const right = nums.filter(x=> x>cur);
return [...sortArray(left), cur, ...sortArray(right)]
};
思路
53. 最大子序和
给你一个整数数组 nums
,请你找出一个具有最大和的连续子数组(子数组最少包含一个元素),返回其最大和。
子数组 是数组中的一个连续部分。
难度 1
题解 贪心(或者是动规)
var maxSubArray = function(nums) {
let sum = nums[0], max = sum;
for(let i = 1;i <nums.length;i++){
sum = Math.max(nums[i],nums[i]+sum);
max = Math.max(max,sum);
}
return max;
};
思路
21. 合并两个有序链表
将两个升序链表合并为一个新的 升序 链表并返回。新链表是通过拼接给定的两个链表的所有节点组成的。
难度 1
题解
var mergeTwoLists = function(list1, list2) {
if(!list1) return list2;
if(!list2) return list1;
const newList = new ListNode(0);
let p = newList;
while(list1&&list2){
if(list1.val<=list2.val){
p.next = list1;
list1 = list1.next;
}else{
p.next = list2;
list2 = list2.next;
}
p = p.next;
}
if(!list1&&list2){
p.next =list2;
}
if(!list2&&list1){
p.next =list1;
}
return newList.next;
};
思路
1. 两数之和
给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出 和为目标值 target 的那 两个 整数,并返回它们的数组下标。
你可以假设每种输入只会对应一个答案。但是,数组中同一个元素在答案里不能重复出现。
你可以按任意顺序返回答案
难度 1
题解 map
var twoSum = function(nums, target) {
let len = nums.length;
const map = new Map();
for(let i = 0;i<len;i++) {
if(map.has(target-nums[i])){
return [i,map.get(target-nums[i])]
}
map.set(nums[i],i);
}
};
思路
102. 二叉树的层序遍历
给你二叉树的根节点 root
,返回其节点值的 层序遍历 。 (即逐层地,从左到右访问所有节点)。
难度 2.5
题解 队列
var levelOrder = function(root) {
let res = [], queue = [root];
while(queue.length&&root){
let len = queue.length;
let curLevel = [];
while(len--){
let node = queue.shift();
curLevel.push(node.val);
node.left&&queue.push(node.left);
node.right&&queue.push(node.right);
}
res.push(curLevel);
}
return res;
};
思路
有个关键点是要判断 root 是否存在
33. 搜索旋转排序数组
难度
题解
思路
121. 买卖股票的最佳时机
给定一个数组 prices ,它的第 i 个元素 prices[i] 表示一支给定股票第 i 天的价格。
你只能选择 某一天 买入这只股票,并选择在 未来的某一个不同的日子 卖出该股票。设计一个算法来计算你所能获取的最大利润。
返回你可以从这笔交易中获取的最大利润。如果你不能获取任何利润,返回 0 。
难度 2
题解1 动规
var maxProfit = function(prices) {
const len = prices.length;
const dp = new Array(len).fill([0,0]);
dp[0]= [-prices[0],0]
for(let i = 1;i<len;i++){
dp[i]= [
Math.max(dp[i-1][0],-prices[i]),
Math.max(dp[i-1][1],prices[i]+dp[i-1][0])
]
}
return dp[prices.length-1][1];
};
题解2 贪心
var maxProfit = function(prices) {
let min = prices[0], res = -min;
for(let i = 0;i<prices.length;i++){
min = Math.min(min,prices[i]);
res = Math.max(res,prices[i]-min);
}
return res<0?0:res;
};
思路
贪心探寻维持最低数
141. 环形链表

难度 1
题解1 set
var hasCycle = function(head) {
const set = new Set();
while(head){
if(set.has(head)){
return true;
}else{
set.add(head);
head = head.next;
}
}
return false;
};
题解2 双指针
var hasCycle = function(head) {
if(!head) return false;
let l = head, r = head.next;
while(r&&r.next){
if(l==r) return true;
l = l.next;
r = r.next.next;
}
return false;
};
思路
200. 岛屿数量
难度
题解
思路
20. 有效的括号
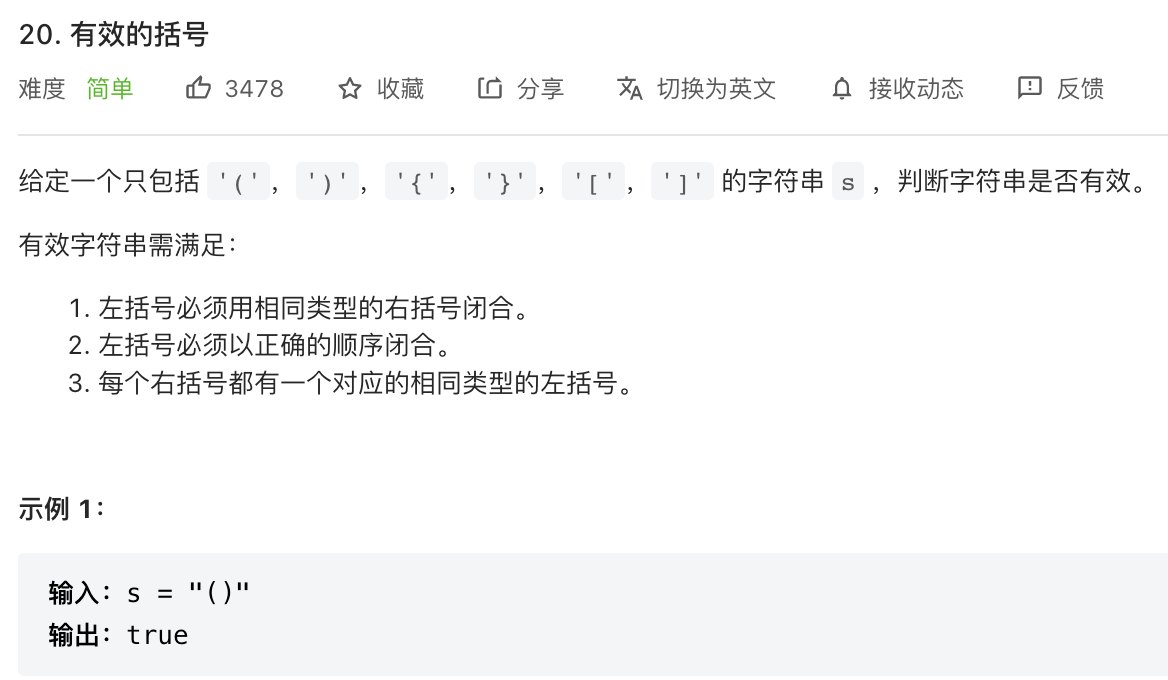
难度 2
题解 map
var isValid = function(s) {
const map = new Map([
['(',')'],
['{','}'],
['[',']']
])
const arr = [];
for(let i = 0;i<s.length;i++){
if(map.has(s[i])){
arr.push(s[i]);
}else if(!map.has(s[i])&&map.get(arr.pop())!=s[i]){
return false;
}
}
return arr.length==0?true:false;
};
思路
103. 二叉树的锯齿形层次遍历
难度 2
题解 层序
var zigzagLevelOrder = function(root) {
let res = [], path = [root], k = 1;
while(path.length&&root){
let curLevel = [];
let len = path.length;
while(len--){
let node = path.shift();
if(k%2){
curLevel.push(node.val);
}else{
curLevel.unshift(node.val)
}
node.left && path.push(node.left);
node.right && path.push(node.right);
}
res.push(curLevel);
k++;
}
return res;
};
思路
和层序类似, 但重点在
5. 最长回文子串
难度
题解
思路
236. 二叉树的最近公共祖先
难度
题解
思路
88. 合并两个有序数组

难度 1
题解 api
var merge = function(nums1, m, nums2, n) {
nums1.splice(m,n,...nums2)
return nums1.sort((a,b)=>a-b);
};