6-1 递增的整数序列链表的插入
本题要求实现一个函数,在递增的整数序列链表(带头结点)中插入一个新整数,并保持该序列的有序性。
函数接口定义:
List Insert( List L, ElementType X );
其中List结构定义如下:
typedefstructNode *PtrToNode;structNode { ElementType Data; /* 存储结点数据 */ PtrToNode Next; /* 指向下一个结点的指针 */};
typedef PtrToNode List; /* 定义单链表类型 */
L是给定的带头结点的单链表,其结点存储的数据是递增有序的;函数Insert要将X插入L,并保持该序列的有序性,返回插入后的链表头指针。
裁判测试程序样例:
#include<stdio.h>#include<stdlib.h>typedefint ElementType;
typedefstructNode *PtrToNode;structNode { ElementType Data;
PtrToNode Next;
};
typedef PtrToNode List;
List Read(); /* 细节在此不表 */voidPrint( List L ); /* 细节在此不表 */List Insert( List L, ElementType X );
intmain(){
List L;
ElementType X;
L = Read();
scanf("%d", &X);
L = Insert(L, X);
Print(L);
return0;
}
/* 你的代码将被嵌在这里 */
输入样例:
5
1 2 4 5 6
3
输出样例:
1 2 3 4 5 6
List Insert( List L, ElementType X ){
List t=(List)malloc(sizeof(struct Node));
t->Data=X;
t->Next=NULL;
List head=L;
while(L->Next!=NULL&&L->Next->Data<=X){
L=L->Next;
}
t->Next=L->Next;
L->Next=t;
return head;
}
6-61 求二叉树高度
本题要求给定二叉树的高度。
函数接口定义:
int GetHeight( BinTree BT );
其中BinTree结构定义如下:
typedef struct TNode *Position;
typedef Position BinTree;
struct TNode{
ElementType Data;
BinTree Left;
BinTree Right;
};
要求函数返回给定二叉树BT的高度值。
裁判测试程序样例:
#include <stdio.h>
#include <stdlib.h>
typedef char ElementType;
typedef struct TNode *Position;
typedef Position BinTree;
struct TNode{
ElementType Data;
BinTree Left;
BinTree Right;
};
BinTree CreatBinTree(); /* 实现细节忽略 */
int GetHeight( BinTree BT );
int main()
{
BinTree BT = CreatBinTree();
printf("%d\n", GetHeight(BT));
return 0;
}
/* 你的代码将被嵌在这里 */
输出样例(对于图中给出的树):
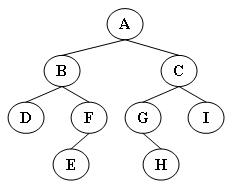
4
int GetHeight( BinTree BT )
{
int HL,HR,MaxH;
if(BT)
{
HL=GetHeight(BT->Left);
HR=GetHeight(BT->Right);
MaxH=HL>HR? HL:HR;
return (MaxH+1);
}
else return 0;
}
6-2 先序输出叶结点
本题要求按照先序遍历的顺序输出给定二叉树的叶结点。
函数接口定义:
void PreorderPrintLeaves( BinTree BT );
其中BinTree结构定义如下:
typedef struct TNode *Position;
typedef Position BinTree;
struct TNode{
ElementType Data;
BinTree Left;
BinTree Right;
};
函数PreorderPrintLeaves应按照先序遍历的顺序输出给定二叉树BT的叶结点,格式为一个空格跟着一个字符。
裁判测试程序样例:
#include <stdio.h>
#include <stdlib.h>
typedef char ElementType;
typedef struct TNode *Position;
typedef Position BinTree;
struct TNode{
ElementType Data;
BinTree Left;
BinTree Right;
};
BinTree CreatBinTree(); /* 实现细节忽略 */
void PreorderPrintLeaves( BinTree BT );
int main()
{
BinTree BT = CreatBinTree();
printf("Leaf nodes are:");
PreorderPrintLeaves(BT);
printf("\n");
return 0;
}
/* 你的代码将被嵌在这里 */
输出样例(对于图中给出的树):
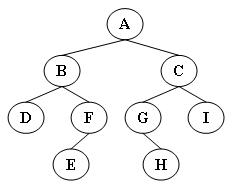
Leaf nodes are: D E H I
void PreorderPrintLeaves( BinTree BT ){
if(BT==NULL){
printf("");
}
else{
if(BT->Left==NULL&&BT->Right==NULL){
printf(" %c",BT->Data);
}
PreorderPrintLeaves( BT->Left );
PreorderPrintLeaves( BT->Right );
}
}
6-3 中序输出度为1的结点
本题要求实现一个函数,按照中序遍历的顺序输出给定二叉树中度为1的结点。
函数接口定义:
void InorderPrintNodes( BiTree T);
T是二叉树树根指针,InorderPrintNodes按照中序遍历的顺序输出给定二叉树T中度为1的结点,格式为一个空格跟着一个字符。
其中BiTree结构定义如下:
typedef struct BiTNode
{
ElemType data;
struct BiTNode *lchild,*rchild;
}BiTNode,*BiTree;
裁判测试程序样例:
#include <stdio.h>
#include <stdlib.h>
typedef char ElemType;
typedef struct BiTNode
{
ElemType data;
struct BiTNode *lchild,*rchild;
}BiTNode,*BiTree;
BiTree Create();/* 细节在此不表 */
void InorderPrintNodes( BiTree T);
int main()
{
BiTree T = Create();
printf("Nodes are:");
InorderPrintNodes(T);
return 0;
}
/* 你的代码将被嵌在这里 */
输入样例:
输入为由字母和'#'组成的字符串,代表二叉树的扩展先序序列。例如对于如下二叉树,输入数据:
ACG#H###BEF###D##
输出样例(对于图中给出的树):
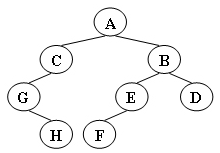