轮播图基础
- 巩固完JavaScriptDOM自己尝试写一下轮播图
小功能
- 上一页、下一页
- 自动轮播
- 鼠标悬停止轮播
- 点击小方框轮播
- 无缝轮播
涉及知识点
- JS面向对象Class
- JSDOM操作
- this指向
- 事件委托
- 事件监听
- 动画封装
- 定时器
- 节流阀
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>轮播图</title>
</head>
<body>
<style type="text/css">
* {
padding: 0;
margin: 0;
box-sizing: border-box;
}
#slideshow {
margin: 60px 60px 0 60px;
width: 1000px;
position: relative;
}
li {
list-style: none;
}
.continer {
width: 1000px;
overflow: hidden;
}
.continer ul {
width: 1000px;
display: flex;
}
.continer ul li {
width: 100%;
height: 100%;
}
.continer ul li img {
width: 1000px;
height: 640px;
cursor: pointer;
}
.labels {
position: absolute;
top: 90%;
left: 50%;
transform: translate(-50%, -50%);
}
.labels ul {
display: flex;
}
.labels ul li {
width: 30px;
display: flex;
justify-content: center;
align-items: center;
}
.labels ul li span {
display: inline-block;
width: 15px;
height: 15px;
background: #fff;
}
.active {
background: red !important;
width: 30px;
}
.arrows {
width: 100%;
height: 60px;
display: flex;
justify-content: space-between;
align-items: center;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
.circl {
width: 60px;
height: 60px;
border-radius: 50%;
background: rgb(0, 0, 0, .2);
position: relative;
overflow: hidden;
cursor: pointer;
}
.circl .square {
width: 60px;
height: 60px;
border-left: 3px solid #999999;
border-bottom: 3px solid #999999;
position: absolute;
top: 50%;
left: 100%;
transform: translate(-50%, -50%) rotate(45deg);
}
.circl .square.right {
top: 50%;
left: 0%;
border-top: 3px solid #999999;
transform: translate(-50%, -50%) rotate(135deg);
}
</style>
<div id="slideshow">
<div class="continer">
<ul>
</ul>
</div>
<div class="labels">
<ul>
</ul>
</div>
<div class="arrows">
<div class="circl left">
<div class="square ">
</div>
</div>
<div class="circl right">
<div class="square right">
</div>
</div>
</div>
</div>
<script type="text/javascript">
let imgArr = ["./img/1.jpg", "./img/2.jpg", "./img/3.jpg", "./img/4.jpg"]
let that;
class Slideshow {
constructor(name) {
that = this
this.continer = document.querySelector('.continer')
this.labels = document.querySelector('.labels')
this.arrowsLeft = document.querySelector('.left')
this.arrowsRight = document.querySelector('.right')
this.init()
this.getAll()
this.currentCarouselIndex = 0
this.carouselTimer = null
this.autoCarousel()
this.flag = true
}
getAll() {
this.lis = this.labels.querySelectorAll('li')
this.imgs = this.continer.querySelectorAll('ul li img')
this.lis[0].children[0].className += ' active'
this.labels.children[0].addEventListener('click', that.hadellabelClick)
}
init() {
that = this
let str = [];
let labelStr = []
imgArr.forEach((item, index) => {
str.push(`<li><img data-index=${index} src="${item}" ></li>`)
labelStr.push(`<li data-index=${index}><span></span></li>`)
})
that.continer.children[0].innerHTML = str.join('')
that.labels.children[0].innerHTML = labelStr.join('')
that.continer.children[0].appendChild(that.continer.children[0].children[0].cloneNode(true))
this.continer.children[0].addEventListener('mouseover', this.handelMouseenter)
this.continer.children[0].addEventListener('mouseout', this.handelMouseleave)
this.arrowsLeft.addEventListener('click', this.handelArrowsLef)
this.arrowsRight.addEventListener('click', this.handelArrowsRight)
this.arrowsLeft.parentNode.addEventListener('mouseover', this.handelMouseenter)
}
hadellabelClick(e) {
that.flag = false
clearInterval(that.carouselTimer)
const index = e.target.getAttribute('data-index') ? e.target.getAttribute('data-index') : e.target
.parentNode.getAttribute('data-index')
that.cancelActiveClass()
that.labels.children[0].children[index].children[0]?.classList.add('active')
that.currentCarouselIndex = index
that.hadelOffset(that.currentCarouselIndex)
}
cancelActiveClass() {
Array.from(that.labels.children[0].children).forEach(item => {
item.children[0].classList?.remove('active')
})
}
hadelOffset(index) {
clearInterval(that.carouselTimer)
const ul = this.continer.children[0]
if (index >= imgArr.length) {
that.cancelActiveClass()
that.labels.children[0].children[0].children[0]?.classList.add('active')
animate(ul, -ul.offsetWidth * imgArr.length, 10, 30, function() {
ul.style.marginLeft = 0
that.currentCarouselIndex = 0
that.flag = true
})
} else if (index <= 0) {
that.cancelActiveClass()
that.labels.children[0].children[0].children[0]?.classList.add('active')
animate(ul, -ul.offsetWidth * index, 10, 30, function() {
ul.style.marginLeft = -(ul.children.length - 1) * ul.offsetWidth + 'px'
that.currentCarouselIndex = ul.children.length - 1
that.flag = true
})
} else {
animate(ul, -ul.offsetWidth * that.currentCarouselIndex, 10, 30, function() {
that.autoCarousel(that.currentCarouselIndex)
that.flag = true
})
that.cancelActiveClass()
that.labels.children[0].children[that.currentCarouselIndex].children[0]?.classList.add('active')
}
}
autoCarousel(currentCarouselIndex) {
const ul = this.continer.children[0]
that.currentCarouselIndex = currentCarouselIndex ? currentCarouselIndex : 0
if (that.carouselTimer) clearInterval(that.carouselTimer)
that.carouselTimer = setInterval(() => {
that.currentCarouselIndex > imgArr.length ? that.currentCarouselIndex = 0 : that
.currentCarouselIndex++
if (that.currentCarouselIndex >= imgArr.length) {
that.cancelActiveClass()
that.labels.children[0].children[0].children[0]?.classList.add('active')
animate(ul, -ul.offsetWidth * imgArr.length, 10, 30, function() {
ul.style.marginLeft = 0
that.currentCarouselIndex = 0
})
} else {
animate(ul, -ul.offsetWidth * that.currentCarouselIndex, 10, 30)
that.cancelActiveClass()
that.labels.children[0].children[that.currentCarouselIndex].children[0]?.classList.add(
'active')
}
}, 2000)
}
stopCarousel() {
if (that.carouselTimer)
clearInterval(that.carouselTimer)
}
handelMouseenter() {
that.stopCarousel()
that.arrowsLeft.style.display = 'block'
that.arrowsRight.style.display = 'block'
}
handelMouseleave() {
that.autoCarousel(that.currentCarouselIndex)
that.arrowsLeft.style.display = 'none'
that.arrowsRight.style.display = 'none'
}
handelArrowsLef() {
if (that.flag) {
that.flag = false
that.currentCarouselIndex <= 0 ? that.currentCarouselIndex = 0 : that.currentCarouselIndex--
that.hadelOffset(that.currentCarouselIndex)
}
}
handelArrowsRight() {
if (that.flag) {
that.flag = false
that.currentCarouselIndex > imgArr.length ? that.currentCarouselIndex = 0 : that
.currentCarouselIndex++
that.hadelOffset(that.currentCarouselIndex)
}
}
}
new Slideshow('#slideshow')
function animate(obj, target, spped = 10, time, callback) {
clearInterval(obj.timer)
obj.timer = setInterval(() => {
let step = (target - obj.offsetLeft) / spped
step = step > 0 ? Math.ceil(step) : Math.floor(step)
if (obj.offsetLeft == target) {
clearInterval(obj.timer)
callback?.()
}
obj.style.marginLeft = obj.offsetLeft + step + 'px'
}, time)
}
</script>
</body>
</html>
``
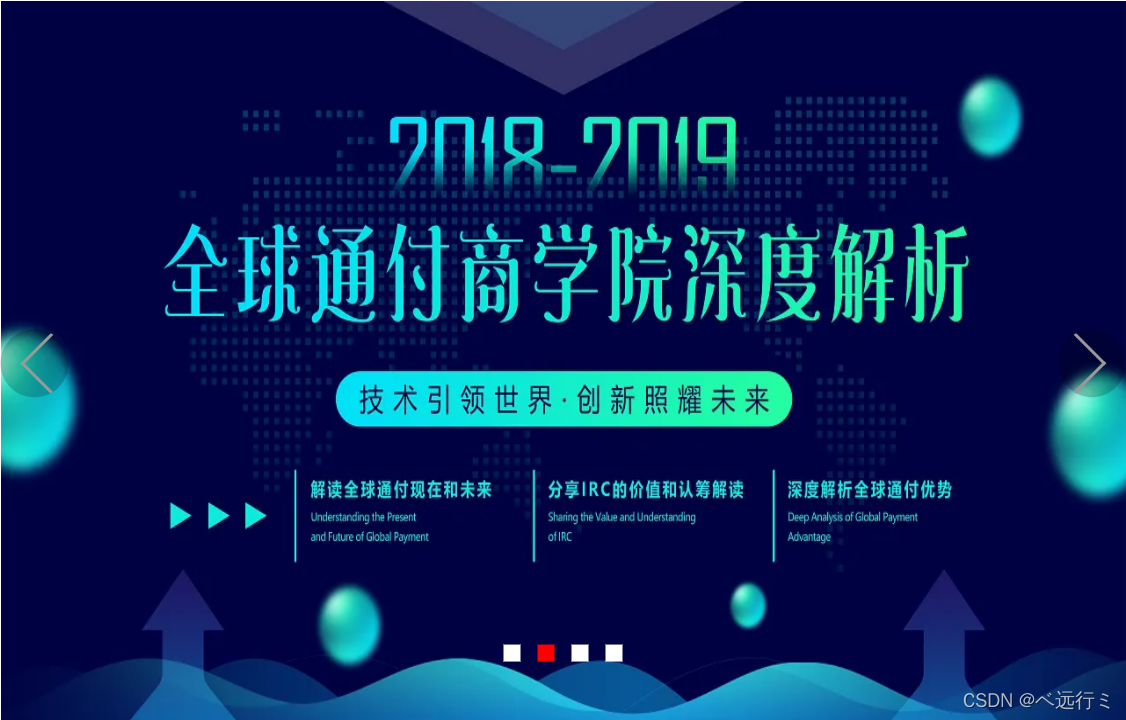