Mybatis-Plus代码生成器
实验脚本:
-- ----------------------------
-- Table structure for parent_list
-- ----------------------------
DROP TABLE IF EXISTS `parent_list`;
CREATE TABLE `parent_list`
(
`p_id` int(11) NOT NULL AUTO_INCREMENT,
`parent_id` int(11) DEFAULT NULL COMMENT '父id',
`name` varchar(255) DEFAULT NULL COMMENT '名称',
`is_enable` tinyint(4) DEFAULT NULL COMMENT '是否启用',
`create_at` datetime(3) DEFAULT NULL COMMENT '创建时间',
PRIMARY KEY (`p_id`) USING BTREE
) ENGINE = InnoDB
AUTO_INCREMENT = 0
DEFAULT CHARSET = utf8
ROW_FORMAT = DYNAMIC;
-- ----------------------------
-- Records of parent_list
-- ----------------------------
INSERT INTO `parent_list`
VALUES (1, 0, 'aa', 1, now());
INSERT INTO `parent_list`
VALUES (2, 1, 'bb', 1, now());
INSERT INTO `parent_list`
VALUES (3, 1, 'cc', 1, now());
INSERT INTO `parent_list`
VALUES (4, 2, 'dd', 1, now());
生成代码:
package com.example.mybatis_generation.utils;
import com.baomidou.mybatisplus.annotation.DbType;
import com.baomidou.mybatisplus.annotation.FieldFill;
import com.baomidou.mybatisplus.generator.AutoGenerator;
import com.baomidou.mybatisplus.generator.config.*;
import com.baomidou.mybatisplus.generator.config.converts.MySqlTypeConvert;
import com.baomidou.mybatisplus.generator.config.po.TableFill;
import com.baomidou.mybatisplus.generator.config.rules.DbColumnType;
import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy;
import java.util.ArrayList;
import java.util.List;
/**
* 配置参考 : https://baomidou.com/pages/d357af
*
* @author: liangtl
*/
public class GlobalConfigs {
/**
* 数据库地址
*/
private static final String DB_URL = "jdbc:mysql://localhost:3306/test?useUnicode=true&useSSL=false&characterEncoding=utf8&useTimezone=true&serverTimezone=Asia/Shanghai&rewriteBatchedStatements=true&useServerPrepStmts=true&allowMultiQueries=true";
/**
* db 账号
*/
private static final String USERNAME = "****";
/**
* db 密码
*/
private static final String PASSWORD = "****";
/**
* db 驱动
*/
private static final String DRIVER_NAME = "com.mysql.cj.jdbc.Driver";
/**
* 存放路径,包路径
*/
private static final String PACKAGE = "com.example.mybatis_generation";
/**
* 需要生成的数据库表名,数组,可多个
*/
private static final String[] TABLE_NAME = {"parent_list"};
public static void main(String[] args) {
GlobalConfig config = new GlobalConfig();
String path = System.getProperty("user.dir");
config.setActiveRecord(true)
// 设置作者信息
.setAuthor("liangtl")
// 设置输出目录位置
.setOutputDir(path + "\\springboot-mybatis-plus-generator\\src\\main\\java\\")
// 是否在xml中生成ResultMap
.setBaseResultMap(true)
// 是否在xml中生成所有列名
.setBaseColumnList(true)
// 是否覆盖原来的代码
.setFileOverride(true);
//****************************** 数据源配置 ***************************************
DataSourceConfig dataSourceConfig = new DataSourceConfig();
dataSourceConfig.setDbType(DbType.MYSQL)
// url
.setUrl(DB_URL)
// 登录用户名
.setUsername(USERNAME)
// 登录密码
.setPassword(PASSWORD)
// 数据库驱动
.setDriverName(DRIVER_NAME)
// 自定义数据库表字段类型转换
.setTypeConvert(new MySqlTypeConvert() {
@Override
public DbColumnType processTypeConvert(GlobalConfig globalConfig, String fieldType) {
System.out.println("转换类型:" + fieldType);
// tinyint转换成Boolean
if (fieldType.toLowerCase().contains("tinyint")) {
return DbColumnType.INTEGER;
}
// datetime转换为Date
if (fieldType.toLowerCase().contains("datetime")) {
return DbColumnType.DATE;
}
return (DbColumnType) super.processTypeConvert(globalConfig, fieldType);
}
});
//****************************** 策略配置 ******************************************************
// 自定义需要填充的字段 数据库中的字段
List<TableFill> tableFillList = new ArrayList<>();
tableFillList.add(new TableFill("gmt_modified", FieldFill.INSERT_UPDATE));
tableFillList.add(new TableFill("modifier_id", FieldFill.INSERT_UPDATE));
tableFillList.add(new TableFill("creator_id", FieldFill.INSERT));
tableFillList.add(new TableFill("gmt_create", FieldFill.INSERT));
tableFillList.add(new TableFill("available_flag", FieldFill.INSERT));
tableFillList.add(new TableFill("deleted_flag", FieldFill.INSERT));
tableFillList.add(new TableFill("sync_flag", FieldFill.INSERT));
StrategyConfig strategyConfig = new StrategyConfig();
// 策略配置
strategyConfig
// 全局大写命名是否开启
.setCapitalMode(true)
// 实体类是否为Lombok模型
.setEntityLombokModel(false)
// 表明生成策略:下划线转驼峰
.setNaming(NamingStrategy.underline_to_camel)
// 自动填充设置
.setTableFillList(tableFillList)
// 需要生成的表
.setInclude(TABLE_NAME);
// 集成注入设置
new AutoGenerator().setGlobalConfig(config)
// 注入数据源配置
.setDataSource(dataSourceConfig)
// 注入策略配置
.setStrategy(strategyConfig)
// 包名信息
.setPackageInfo(
new PackageConfig()
// 提取公共父级包名
.setParent(PACKAGE)
// controller层
.setController("controller")
// 实体层
.setEntity("entity")
// dao层
.setMapper("dao")
// xml
.setXml("dao/mapper")
)
// 自定义模板
.setTemplate(
new TemplateConfig()
.setServiceImpl("templates/serviceImpl.java")
)
.execute();
}
}
生成目录结构:
// 集成注入设置
new AutoGenerator().setGlobalConfig(config)
// 注入数据源配置
.setDataSource(dataSourceConfig)
// 注入策略配置
.setStrategy(strategyConfig)
// 包名信息
.setPackageInfo(
new PackageConfig()
// 提取公共父级包名
.setParent(PACKAGE)
// controller层
.setController(“controller”)
// 实体层
.setEntity(“entity”)
// dao层
.setMapper(“dao”)
// xml
.setXml(“dao/mapper”)
)
// 自定义模板
.setTemplate(
new TemplateConfig()
.setServiceImpl(“templates/serviceImpl.java”)
)
.execute();
}
}
生成目录结构:
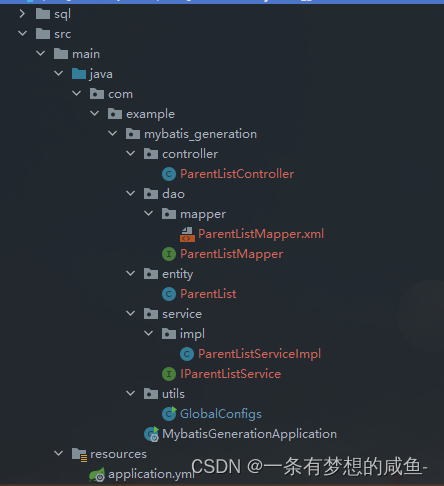