目录
文件操作
文件创建
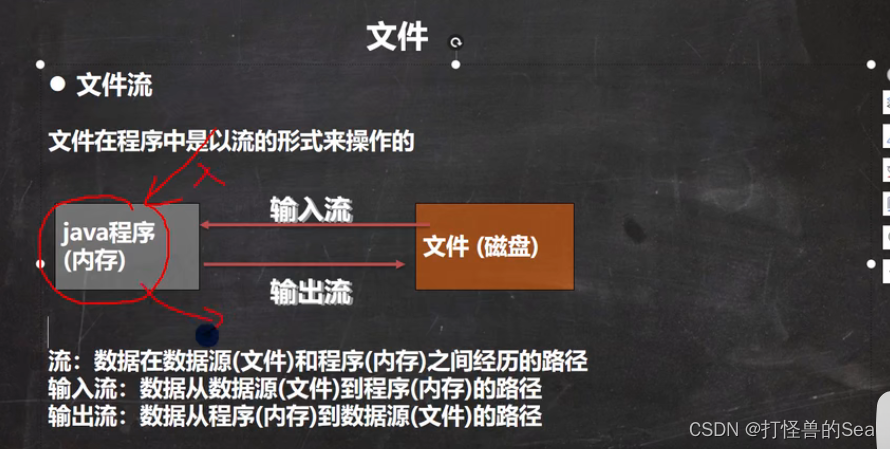
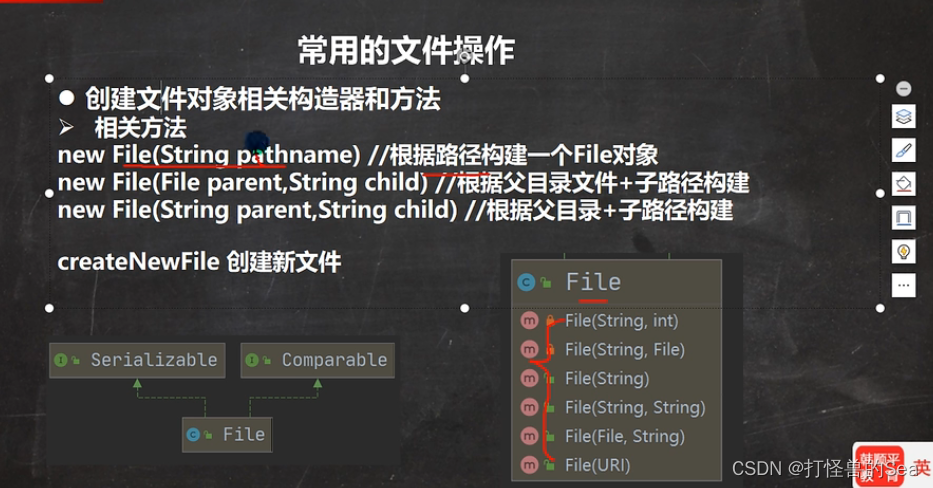
package com.edu.file;
import org.junit.jupiter.api.Test;
import java.io.File;
import java.io.IOException;
public class Demo01 {
public static void main(String[] args) {
}
@Test
//方式1
public void create01(){
String filePath = "D:\\new1.txt";
File file = new File(filePath);
try {
file.createNewFile();
} catch (IOException e) {
throw new RuntimeException(e);
}
System.out.println("创建成功");
}
@Test
//方式二
public void create02(){
File file = new File("d:\\"); //父
String fileName = "new2.txt";//子
File file1 = new File(file,fileName);
try {
file1.createNewFile(); //真正创建
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Test
//方式三
public void crete03(){
String parentPath = "d:\\";
String sonPath = "new3.txt";
File file = new File(parentPath,sonPath);
try {
file.createNewFile();
} catch (IOException e) {
throw new RuntimeException(e);
}
System.out.println("创建成功");
}
}
获取文件信息
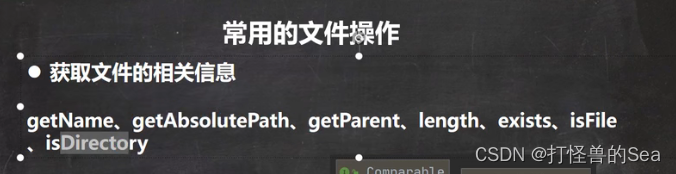
//获取文件信息
public void info(){
//先创建文件对象
File file = new File("d:\\new3.txt");
//调用相应方法得到对应信息
System.out.println("文件名字:"+file.getName());
System.out.println("文件绝对路径"+file.getAbsolutePath());
System.out.println("文件父目录"+file.getParent());
System.out.println("文件大小"+file.length());
System.out.println("文件是否存在"+file.exists());
System.out.println("是不是一个文件"+file.isFile());
System.out.println("是不是一个目录"+file.isDirectory());
}
文件目录操作
package com.edu.file;
import org.junit.jupiter.api.Test;
import java.io.File;
public class Demo03 {
public static void main(String[] args) {
m1();
m2();
}
//删除文件
public static void m1(){
String filePath = "d:\\new3.txt";
File file = new File(filePath);
if (file.exists()){
if(file.delete()){
System.out.println("成功");
}else {
System.out.println("失败");
}
}else {
System.out.println("文件不存在");
}
}
//删除目录 目录在java编程中也是一种文件的存在
@Test
public static void m2(){
String filePath = "d:\\demo02";
File file = new File(filePath);
if (file.exists()){
if(file.delete()){
System.out.println("成功");
}else {
System.out.println("失败");
}
}else {
System.out.println("文件不存在");
}
}
//判断目录是否存在,不存在则创建
@Test
public void m3(){
String filePath = "d:\\demo02\\a";
File file = new File(filePath);
if (file.exists()){
System.out.println("已经存在");
}else {
if (file.mkdirs()){
System.out.println("创建成功");
}else {
System.out.println("失败");
}
}
}
}
IO流原理和流的分类
流的分类
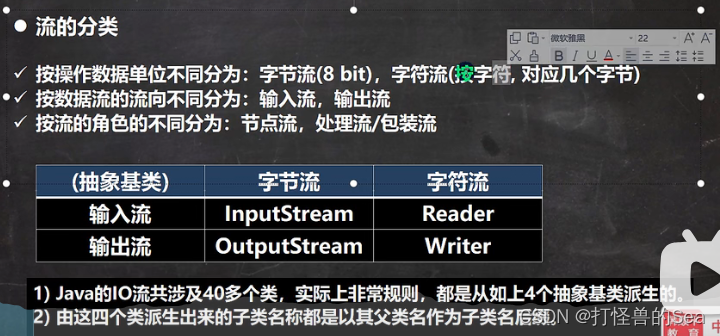
流和文件的关系: 流是文件传输的载体
字节流
FileInputStream
package com.edu.inputstream;
import org.junit.jupiter.api.Test;
import java.io.FileInputStream;
import java.io.IOException;
/*
FileInputStream,文件---->程序
只能读取字节,效率较低
*/
public class Demo01 {
public static void main(String[] args) {
try {
readFile01();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
//效率低
public static void readFile01() throws IOException {
String filePath = "d:\\hello.txt";
FileInputStream fileInputStream = null;
int readDate = 0;
fileInputStream = new FileInputStream(filePath);
try {
//返回-1表示读取完毕
//不能读取中文
while ((readDate=fileInputStream.read())!=-1){
System.out.print((char)readDate);
}
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
fileInputStream.close();
}
}
@Test
//使用read(byte[] b) 提高效率
public void readFile02() throws IOException {
String filePath = "d:\\hello.txt";
FileInputStream fileInputStream = null;
int readLen = 0;
//字节数组,一次读取8个
byte[] buf = new byte[8];
fileInputStream = new FileInputStream(filePath);
try {
//返回-1表示读取完毕
//读取正常则返回实际读取的字节数
while ((readLen=fileInputStream.read(buf))!=-1){
System.out.print(new String(buf,0,readLen));
}
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
fileInputStream.close();
}
}
}
FileOutputStream
package com.edu.outputstream;
import org.junit.jupiter.api.Test;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
public class Demo01 {
public static void main(String[] args) {
}
/*
数据写到文件中,如果文件不存在则创建文件
*/
@Test
public void writeFile(){
String filePath = "d:\\hello.txt";
File file = new File(filePath);
FileOutputStream fileOutputStream = null;
try {
//ture表示追加 false表示创建新文件或覆盖
fileOutputStream = new FileOutputStream(filePath,true);
//写入一个字符
fileOutputStream.write('a');
//写入字符串
fileOutputStream.write("hello".getBytes());
//指定位置
String str = "hello";
fileOutputStream.write(str.getBytes(),0,str.length());
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
try {
fileOutputStream.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
}
FileCopy
package com.edu.outputstream;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
public class Demo02 {
public static void main(String[] args) {
String regionFilePath = "c:\\1.jpg";
String targetFilePath = "d:\\1.jpg";
FileInputStream fileInputStream = null;
FileOutputStream fileOutputStream = null;
byte[] buf = new byte[1024];
int readLen = 0;
try {
fileInputStream = new FileInputStream(regionFilePath);
fileOutputStream = new FileOutputStream(targetFilePath,true);
while ((readLen=fileInputStream.read(buf))!=-1){
fileOutputStream.write(buf,0,readLen);//必须是这个方法
}
} catch (IOException e) {
throw new RuntimeException(e);
} finally {
if (fileInputStream!=null){
try {
fileInputStream.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
if(fileOutputStream!=null){
try {
fileOutputStream.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
}
}
字符流
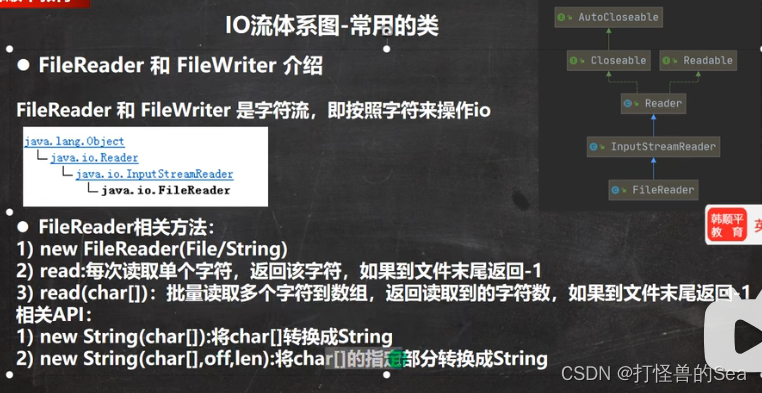
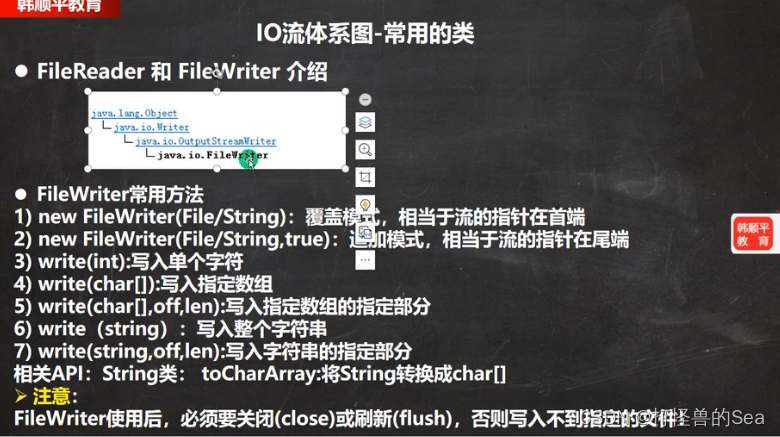
close和flush的区别:close会刷新缓冲区域并关闭输出流对象,flush刷新缓冲区域,不关闭。如果使用close的话,则无法继续再输出数据到目标文件了。
节点流和处理流
BufferedReader
public class Demo01 {
public static void main(String[] args) throws Exception{
String filePath = "d:\\x.txt";
//创建
BufferedReader bufferedReader = new BufferedReader(new FileReader(filePath));
//读取
String line = null;
while ((line=bufferedReader.readLine())!=null){
System.out.println(line);
}
//关闭
bufferedReader.close();
}
}
BufferedWritter
public class Demo02 {
public static void main(String[] args) throws Exception {
String filePath = "d:\\x.txt";
BufferedWriter bufferWriter = new BufferedWriter(new FileWriter(filePath,true));
bufferWriter.write("123");
bufferWriter.write("\r\n");
bufferWriter.write("hello java大神");
bufferWriter.write("hello java大牛");
bufferWriter.write("\r");
bufferWriter.write("123");
bufferWriter.close();
}
}
文件拷贝
public class Demo03 {
public static void main(String[] args) throws IOException {
String filePath = "d:\\x.txt";
String targetPath = "d:\\copy.txt";
BufferedReader bufferedReader = new BufferedReader(new FileReader(filePath));
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(targetPath));
String str = null;
while ((str=bufferedReader.readLine())!=null){
bufferedWriter.write(str);
bufferedWriter.newLine();
}
bufferedReader.close();
bufferedWriter.close();
}
}
处理流
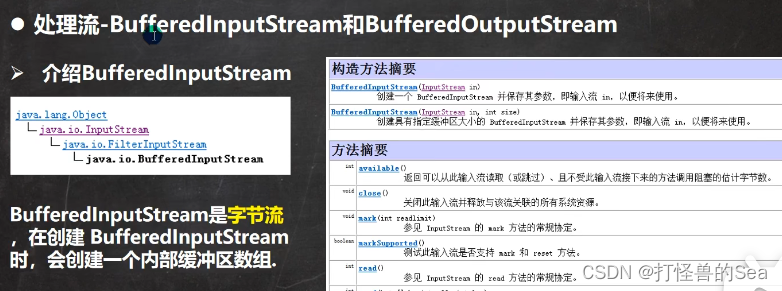
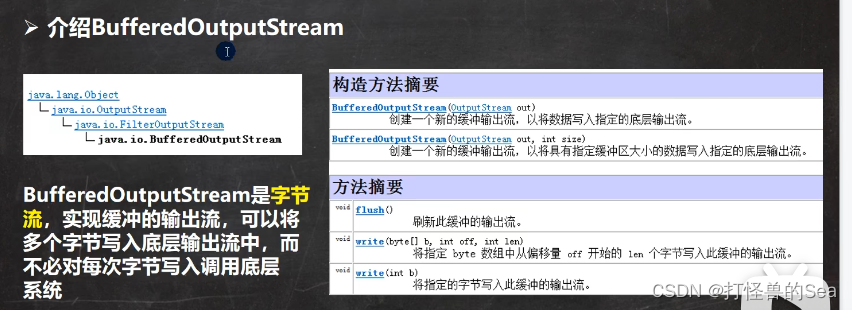
图片或者音视频复制
字节流可以处理二进制文件,也可以处理文本文件
public class Demo02 {
public static void main(String[] args) throws IOException {
String filePath = "c:\\1.jpg";
String targetPath = "d:\\1.jpg";
BufferedInputStream bufferedInputStream = new BufferedInputStream(new FileInputStream(filePath));
BufferedOutputStream bufferedOutputStream = new BufferedOutputStream(new FileOutputStream(targetPath));
byte[] buf = new byte[1024];
int readlen = 0;
while ((readlen=bufferedInputStream.read(buf))!=-1){
bufferedOutputStream.write(buf,0,readlen);
}
bufferedInputStream.close();
bufferedOutputStream.close();
}
}
对象处理流
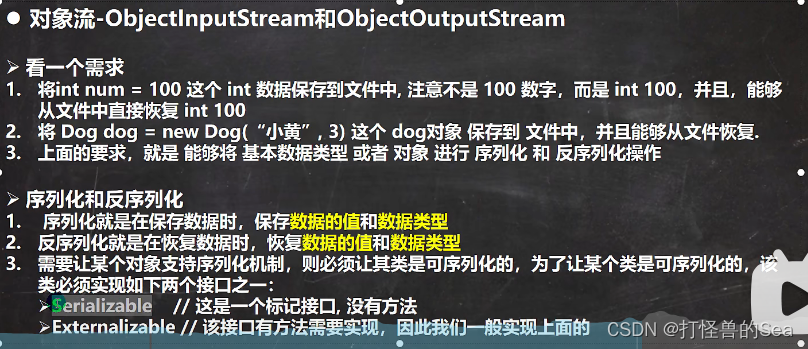
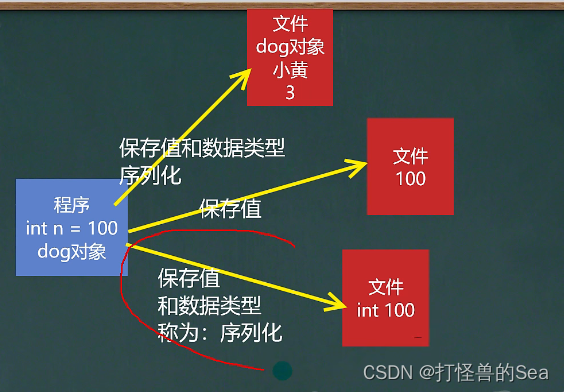
ObjectOutputStream
package com.edu.outputstream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.io.Serializable;
/*
*/
public class Demo03 {
public static void main(String[] args) throws IOException {
String filePath = "d:\\data.txt"; //序列化后保存的文本格式是按照他的格式保存
ObjectOutputStream oos = new ObjectOutputStream(new FileOutputStream(filePath));
//序列化数据到文件中
oos.write(100); //int-->Integer实现了序列化接口
oos.writeBoolean(true);
oos.writeChar('a');
oos.writeDouble(9.5);
oos.writeUTF("你哈哈哈哈哈"); //String
//保存dog对象
Dog dog = new Dog(100,"大黄");
oos.writeObject(dog); //要实现Serializable接口
oos.close();
System.out.println("存储完毕");
}
}
class Dog implements Serializable {
private int age;
private String name;
public Dog(int age, String name) {
this.age = age;
this.name = name;
}
}
ObjectInputStream
package com.edu.outputstream;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
public class Demo04 {
public static void main(String[] args) throws IOException, ClassNotFoundException {
String filePath = "d:\\data.txt";
ObjectInputStream ois = new ObjectInputStream(new FileInputStream(filePath));
//读取 存放顺序和读取顺序要一致
System.out.println(ois.readInt());
System.out.println(ois.readBoolean());
System.out.println(ois.readChar());
System.out.println(ois.readDouble());
System.out.println(ois.readUTF());
Object dog = ois.readObject();
System.out.println(dog.getClass());
System.out.println(dog);
//细节
//Dog dog2 = (Dog) dog //类不见了,需要拿回来
// 因为包也序列化进去了包要保持一致,
//或者Dog公有化
ois.close();
}
}
标准输入输出流
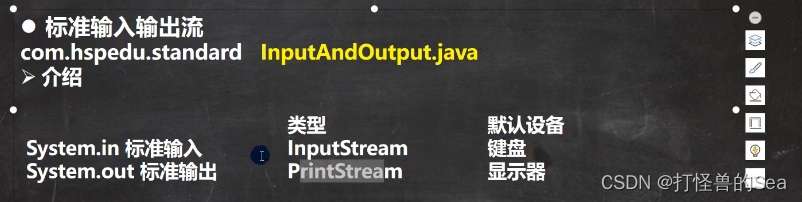
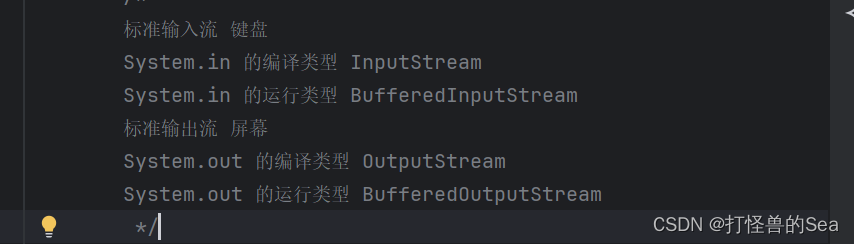
转换流
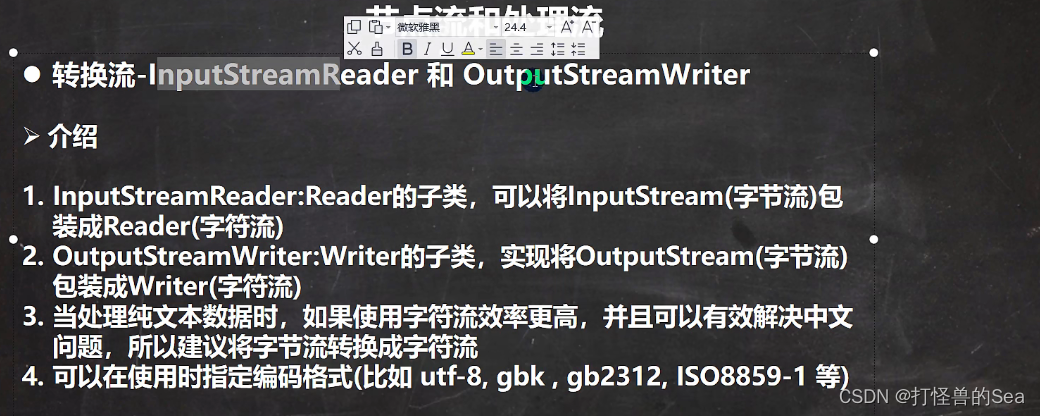
public class Demo02 {
public static void main(String[] args) throws Exception {
/*
编码默认是utf-8读取
文件改了编码的会乱码--->引出了转换流
*/
String str = null;
String filePath = "d:\\1.txt";
BufferedReader bufferedReader = new BufferedReader(new FileReader(filePath));
while ((str=bufferedReader.readLine())!=null){
System.out.println(str);
}
bufferedReader.close();
}
}
InputStreamReader
public class Demo03 {
public static void main(String[] args) throws Exception{
String filePath = "d:\\1.txt";
//解读
//1、把FileInputStream 转换成 InputStreamReader
//2、指定编码gbk
InputStreamReader inputStreamReader = new InputStreamReader(new FileInputStream(filePath),"gbk");
//3、把InputStreamReader传入BufferedReader
BufferedReader bufferedReader = new BufferedReader(inputStreamReader);
String str = null;
while ((str=bufferedReader.readLine())!=null){
System.out.println(str);
}
bufferedReader.close();
}
}
OutputStreamReader
public class Demo04 {
public static void main(String[] args) throws Exception {
String filePath = "d:\\1.txt";
BufferedWriter bufferedWriter = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(filePath,true),"gbk"));
bufferedWriter.write("1231");
bufferedWriter.flush();
bufferedWriter.close();
}
}
打印流
PrintStream
public class Demo05 {
public static void main(String[] args) throws IOException {
PrintStream out = System.out;
//默认情况下可以输出到显示器
out.println("John");
out.write("默认情况下可以输出到显示器".getBytes());
//可以修改输出的位置
System.setOut(new PrintStream("d:\\f1.txt"));
System.out.println("1231231");
out.close();
}
}
PrintWriter
public class Demo06 {
public static void main(String[] args) throws IOException {
PrintWriter printWriter = new PrintWriter(System.out);
printWriter.println("Hello,小孩");
PrintWriter printWriter1 = new PrintWriter(new FileWriter("d:\\f2.txt",true));
printWriter1.println("北京你好");
//要关闭,没有关闭进不去
printWriter.close();
printWriter1.close();
}
}
Properties
public class Demo01 {
public static void main(String[] args) throws IOException {
//传统读取文件,并得到ip user和pwd
BufferedReader bufferReader = new BufferedReader(new FileReader("D:\\Java\\JavaSE\\IO\\src\\mysql_properties"));
String str = null;
while ((str=bufferReader.readLine())!=null){
String[] strings = str.split("=");
System.out.println(strings[0]+"值是:"+strings[1]);
}
try {
bufferReader.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
}
public class Demo02 {
public static void main(String[] args) throws IOException {
//使用Properties完成文件的读取
//1、创建对象
Properties properties = new Properties();
//2、加载指定文件
properties.load(new FileReader("D:\\Java\\JavaSE\\IO\\src\\mysql_properties"));
//3、把k-v显示到控制台
properties.list(System.out);
//4、更加键获取值
String user = properties.getProperty("user");
System.out.println(user);
//使用Properties添加k-v到文件中
//1、创建对象
Properties properties1 = new Properties();
//2、创建k-v
properties1.setProperty("charSet","utf8");
properties1.setProperty("root","小虎");
//3、将k-v存储到文件中
//FileWriter 存的还是字符,FileOutputStream存的是unicode编码
//comment是注释
properties1.store(new FileWriter("D:\\Java\\JavaSE\\IO\\src\\mysql_properties",true),null);
properties1.store(new FileOutputStream("D:\\Java\\JavaSE\\IO\\src\\mysql_properties"),null);
System.out.println("成功");
//使用Properties修改文件
//文件没有key就是创建
//文件有key就是修改
properties1.setProperty("user","小2");
properties1.store(new FileOutputStream("D:\\Java\\JavaSE\\IO\\src\\mysql_properties"),null);
System.out.println("成功");
}
}