1. 案例说明:
- 在界面上以表格的形式,显示一些书籍的数据;
- 点击+或者-可以增加或减少书籍数量(如果为1,那么不能继续-);
- 点击移除按钮,可以将书籍移除;
- 在底部显示书籍的总价格;
- 用方法统一显示价格¥;
- 当所有的书籍移除完毕时,显示:购物车为空~。
2. 效果图:
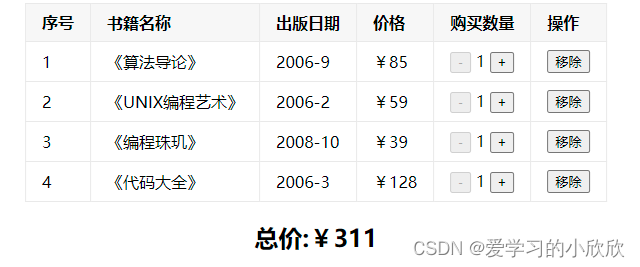
3. 开发过程
3.1 在界面上以表格的形式,显示一些书籍的数据;
<body>
<div id="app"></div>
<template id="shopping">
<table>
<thead>
<th>序号</th>
<th>书籍名称</th>
<th>出版日期</th>
<th>价格</th>
<th>购买数量</th>
<th>操作</th>
</thead>
<tbody>
<tr v-for="(item,index) in books">
<td>{{index+1}}</td>
<td>{{item.name}}</td>
<td>{{item.date}}</td>
<td>{{item.price}}</td>
<td>
<button>-</button>
<span class="count">{{item.count}}</span>
<button>+</button>
</td>
<td>
<button>移除</button>
</td>
</tr>
</tbody>
</table>
<h2>总价:</h2>
</template>
<script src="../js/vue.js"></script>
<script src="./index.js"></script>
</body>
Vue.createApp({
template: '#shopping',
data: function () {
return {
books: [
{
id: 1,
name: '《算法导论》',
date: '2006-9',
price: 85.00,
count: 1
},
{
id: 2,
name: '《UNIX编程艺术》',
date: '2006-2',
price: 59.00,
count: 1
},
{
id: 3,
name: '《编程珠玑》',
date: '2008-10',
price: 39.00,
count: 1
},
{
id: 4,
name: '《代码大全》',
date: '2006-3',
price: 128.00,
count: 1
},
]
}
},
computed: {
},
methods: {
}
}).mount("#app");
table {
border: 1px solid #e9e9e9;
border-collapse: collapse;
border-spacing: 0;
margin-left: 50%;
transform: translateX(-50%);
}
th,td{
padding: 8px 16px;
border: 1px solid #e9e9e9;
text-align: left;
}
th{
background-color: #f7f7f7;
color: 5c6b77;
font-weight: 600;
}
.count{
margin: 0px 5px;
}
h2{
text-align: center;
}
3.2 点击+或者-可以增加或减少书籍数量(如果为1,那么不能继续-);
<td>
<button :disabled="item.count<2" @click="sub(index)">-</button>
<span class="count">{{item.count}}</span>
<button @click="add(index)">+</button>
</td>
methods: {
sub(index) {
this.books[index].count--;
},
add(index) {
this.books[index].count++;
}
}
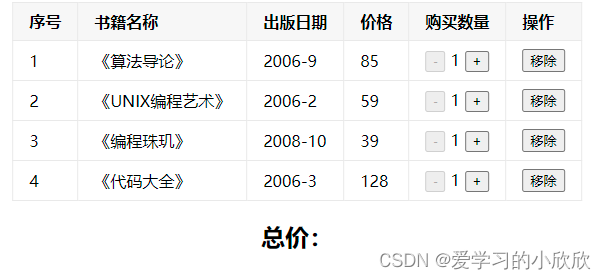
3.3 点击移除按钮,可以将书籍移除;
<button @click="remove(index)">移除</button>
methods: {
sub(index) {
this.books[index].count--;
},
add(index) {
this.books[index].count++;
},
remove(index) {
// 从index开始,切割1位
this.books.splice(index, 1)
}
}
3.4 在底部显示书籍的总价格;
<h2>总价:{{total}}</h2>
computed: {
total() {
let sum = 0;
for (let book of this.books) {
sum += book.price * book.count;
}
return sum;
}
},
-
3.5 用方法统一显示价格¥;
-
methods: {
sub(index) {
this.books[index].count--;
},
add(index) {
this.books[index].count++;
},
remove(index) {
// 从index开始,切割1位
this.books.splice(index, 1)
},
formatPrice(price) {
return "¥" + price;
}
}
//表格价格
<td>{{formatPrice(item.price)}}</td>
//总价
<h2>总价:{{formatPrice(total)}}</h2>
-
-
3.6 当所有的书籍移除完毕时,显示:购物车为空~。
<template v-if="books.length>0">
<table>
<thead>
<th>序号</th>
<th>书籍名称</th>
<th>出版日期</th>
<th>价格</th>
<th>购买数量</th>
<th>操作</th>
</thead>
<tbody>
<tr v-for="(item,index) in books">
<td>{{index+1}}</td>
<td>{{item.name}}</td>
<td>{{item.date}}</td>
<td>{{formatPrice(item.price)}}</td>
<td>
<button :disabled="item.count<2" @click="sub(index)">-</button>
<span class="count">{{item.count}}</span>
<button @click="add(index)">+</button>
</td>
<td>
<button @click="remove(index)">移除</button>
</td>
</tr>
</tbody>
</table>
<h2>总价:{{formatPrice(total)}}</h2>
</template>
<template v-else>
<h2>购物车为空~</h2>
</template>