无缝切换轮播图
一、效果图(完整效果参见源码)
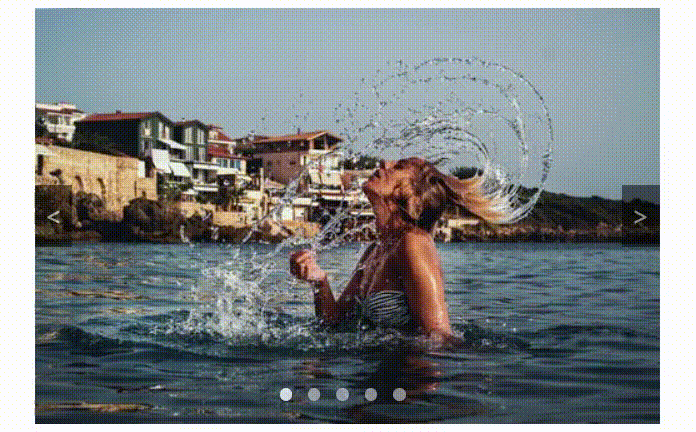
二、源码
(一)HTML文档(index.html)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>无缝切换轮播图(JS运动框架的运用)</title>
<link rel="stylesheet" href="css/index.css">
</head>
<body>
<div class="wrapper">
<ul class="imgBox">
<li><img src="images/01.jpeg" alt=""></li>
<li><img src="images/02.jpeg" alt=""></li>
<li><img src="images/03.jpeg" alt=""></li>
<li><img src="images/04.jpeg" alt=""></li>
<li><img src="images/05.jpeg" alt=""></li>
<li><img src="images/01.jpeg" alt=""></li>
</ul>
<div class="btn btnLeft"><</div>
<div class="btn btnRight">></div>
<div class="circleBox">
<span class="active"></span>
<span></span>
<span></span>
<span></span>
<span></span>
</div>
</div>
<script src="js/tools.js"></script>
<script src="js/index.js"></script>
</body>
</html>
(二)CSS文档(index.css)
* {
padding: 0px;
margin: 0px;
list-style: none;
}
.wrapper {
width: 500px;
height: 333px;
margin: 60px auto 0px;
background: #333;
position: relative;
overflow: hidden;
}
.wrapper .imgBox {
width: 3000px;
height: 333px;
position: absolute;
left: 0px;
top: 0px;
}
.wrapper .imgBox li {
float: left;
width: 500px;
height: 333px;
}
.wrapper .imgBox li img {
width: 100%;
height: 100%;
}
.wrapper .btn {
width: 30px;
height: 50px;
position: absolute;
top: 50%;
margin-top: -25px;
font-size: 20px;
color: #fff;
background: #000;
text-align: center;
line-height: 50px;
cursor: pointer;
opacity: 0.5;
}
.wrapper:hover .btn {
opacity: 0.8;
}
.wrapper .btnLeft {
left: 0px;
}
.wrapper .btnRight {
right: 0px;
}
.wrapper .circleBox {
position: absolute;
left: 50%;
bottom: 15px;
margin-left: -54px;
}
.wrapper .circleBox span {
display: inline-block;
width: 10px;
height: 10px;
margin-right: 8px;
background: #fff;
opacity: 0.5;
cursor: pointer;
border-radius: 50%;
text-align: center;
}
.wrapper .circleBox span.active {
opacity: 0.8;
}
(三)JS文档(index.js)
var imgBox = document.getElementsByClassName('imgBox')[0],
moveWidth = imgBox.children[0].offsetWidth,
timer = null,
imgNum = imgBox.children.length - 1;
var btnLeft = document.getElementsByClassName('btnLeft')[0],
btnRight = document.getElementsByClassName('btnRight')[0],
oSpanArray = document.getElementsByClassName('circleBox')[0].getElementsByTagName('span');
var lock = true;
var index = 0;
btnLeft.onclick = function () {
autoMove('rightToLeft');
}
btnRight.onclick = function () {
autoMove('leftToRight')
}
for(var i = 0; i < oSpanArray.length; i++) {
(function (myIndex) {
oSpanArray[i].onclick = function () {
lock = false;
clearTimeout(timer);
index = myIndex;
changeIndex(index);
startMove(imgBox, {left : -index * moveWidth}, function () {
lock = true;
timer = setTimeout(autoMove, 1500);
});
}
})(i);
}
function autoMove(direction) {
if(lock) {
lock = false;
clearTimeout(timer);
if(!direction || direction == 'leftToRight') {
index++;
if(index == imgNum) {
index = 0;
}
changeIndex(index);
startMove(imgBox, {left : imgBox.offsetLeft - moveWidth}, function () {
if(imgBox.offsetLeft == -imgNum * moveWidth) {
imgBox.style.left = '0px';
}
timer = setTimeout(autoMove, 1500);
lock = true;
});
}else if(direction == 'rightToLeft') {
if(imgBox.offsetLeft == 0) {
imgBox.style.left = (-imgNum * moveWidth) +'px';
index = imgNum;
}
index--;
changeIndex(index);
startMove(imgBox, {left : imgBox.offsetLeft + moveWidth}, function () {
timer = setTimeout(autoMove, 1500);
lock = true;
});
}
}
}
function changeIndex(_index) {
for(var i = 0; i < oSpanArray.length; i++) {
oSpanArray[i].className = '';
}
oSpanArray[_index].className = 'active';
}
timer = setTimeout(autoMove, 1500);
(四)JS文档(tools.js)
function getStyle (dom, attr) {
if (window.getComputedStyle) {
return window.getComputedStyle(dom, null)[attr];
}else {
return dom.currentStyle[attr];
}
}
function startMove(dom, attrObj, callback) {
clearInterval(dom.timer);
var iSpeed = null, iCur = null;
dom.timer = setInterval(function () {
var bStop = true;
for(var attr in attrObj) {
if(attr == 'opacity') {
iCur = parseFloat( getStyle(dom, attr) ) * 100;
}else {
iCur = parseInt( getStyle(dom, attr) );
}
iSpeed = (attrObj[attr] - iCur) / 7;
iSpeed = iSpeed > 0 ? Math.ceil(iSpeed) : Math.floor(iSpeed);
if(attr == 'opacity') {
dom.style.opacity = (iCur + iSpeed) / 100;
}else {
dom.style[attr] = iCur + iSpeed + 'px';
}
if(iCur != attrObj[attr]) {
bStop = false;
}
}
if(bStop) {
clearInterval(dom.timer);
typeof callback == 'function' && callback();
}
}, 30);
}