我们利用java制作一个简单的打斗类小游戏,首先要明白其中需要建造几个类型。然后分别定义类的属性,在这其中会不断地衍射出许多方法,对面向对象是一个不错的检验,接下来我们来看简单的代码提示。
(1)、设置一个英雄类;
在这里插入代码片package Newgame;
public class Hero {
private String name;
private int hp;
private int attacker;
private int defender;
private int exp;
private boolean isLive;
private int age;
private char gender;
private String weapon;
private int maxLife;
public int getMaxLife() {
return maxLife;
}
public void setMaxLife(int maxLife) {
this.maxLife = maxLife;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getHp() {
return hp;
}
public void setHp(int hp) {
this.hp = hp;
}
public int getAttacker() {
return attacker;
}
public void setAttacker(int attacker) {
this.attacker = attacker;
}
public int getDefender() {
return defender;
}
public void setDefender(int defender) {
this.defender = defender;
}
public int getExp() {
return exp;
}
public void setExp(int exp) {
this.exp = exp;
}
public boolean isLive() {
return isLive;
}
public void setLive(boolean isLive) {
this.isLive = isLive;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public char getGender() {
return gender;
}
public void setGender(char gender) {
this.gender = gender;
}
public String getWeapon() {
return weapon;
}
public void setWeapon(String weapon) {
this.weapon = weapon;
}
public Hero() {
}
public Hero(String name, int age, char gender,String weapon) {
this.name = name;
this.maxLife = Constant.INILIVE;
this.hp = maxLife;
this.attacker = Constant.INIATT;
this.defender = Constant.INIDEF;
this.exp = Constant.INTEXP;
this.isLive = true;
this.age = age;
this.gender = gender;
this.weapon = weapon;
}
//战斗方法
public void fight(Monster monster) {
System.out.println("超越世界一流的英雄:" + this.getName() + "拿着" + this.weapon + "砍向了怪物:" + monster.getName());
// 判断怪物是否死亡
if (!monster.isLive()) {
System.out.println("黑暗终将被消灭");
return;
}
// 判断英雄是否死亡
if (!this.isLive()) {
System.out.println("如果你还想拯救世界,那就复活你的英雄吧");
return;
}
monster.hurt(this);
monster.show();
}
//英雄状态
public void status() {
System.out.println("当前英雄是:" + this.getName() + "性别:" + this.getGender() + "、血量为:" + this.getHp() + "、攻击力为:"
+ this.getAttacker() + "防御值为:" + this.getDefender());
}
//英雄受伤
public void hurt(Monster monster) {
int loseLife = Constant.TYPEATTR * monster.getType() + monster.getAttacker() - this.getDefender();
if(loseLife <= 0) {
this.setHp(this.getHp() - Constant.LOSELIFE);
}else {
this.setHp(this.getHp() - loseLife);
}
// 判断是否英雄死亡
if (this.getHp() <= 0) {
this.dead();
}
this.status();
this.fight(monster);
}
private void dead() {
System.err.println("黑暗终将来临,我神龙一族不会就这样放弃光明,我的子孙会一直的战斗下去,迎接到新的光明");
this.setLive(false);
}
}
(2)、设置怪物类
```java
package Newgame;
public class Monster {
// 怪物名称
private String name;
// 怪物血量属性
private int hp;
// 怪物攻击属性
private int attacker;
// 怪物防御属性
private int defender;
// 武器属性
private String weapon;
// 怪物状态属性
private boolean isLive;
// 怪物类型
private int type;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getHp() {
return hp;
}
public void setHp(int hp) {
this.hp = hp;
}
public int getAttacker() {
return attacker;
}
public void setAttacker(int attacker) {
this.attacker = attacker;
}
public int getDefender() {
return defender;
}
public void setDefender(int defender) {
this.defender = defender;
}
public String getWeapon() {
return weapon;
}
public void setWeapon(String weapon) {
this.weapon = weapon;
}
public boolean isLive() {
return isLive;
}
public void setLive(boolean isLive) {
this.isLive = isLive;
}
public int getType() {
return type;
}
public void setType(int type) {
this.type = type;
}
public Monster() {
}
public Monster(String name, int hp, int attacker, int defender,String weapon,int type) {
this.name = name;
this.hp = hp;
this.attacker = attacker;
this.defender = defender;
this.weapon = weapon;
this.type = type;
this.isLive = true;
}
//怪物受伤方法
public void hurt(Hero hero) {
int loseLife = hero.getAttacker() - this.getDefender();
if(loseLife <= 0) {
this.setHp(this.getHp() - Constant.LOSELIFE);
}else {
this.setHp(getHp() - loseLife);
}
// 怪物是否死亡
if(this.getHp() <= 0) {
// 怪物死亡
this.dead();
}
}
private void dead() {
System.out.println("小b玩意,既然弄死你大爷我,我会回来报仇的");
this.setLive(false);
}
// 怪物信息展示
public void show() {
System.out.println("当前的怪物是:" + this.getName() + "、当前的血量为:" + this.getHp()
+ "攻击力:" + this.getAttacker() + "防御力:"
+ this.getDefender() + "是否活着:" + this.isLive);
}
// 怪物打英雄的方法
public void kill(Hero hero) {
System.out.println("和我抖,不自量力");
if (!hero.isLive()) {
System.out.println("英雄落幕,告别时代了,我在没有对手了");
return;
}
if (!this.isLive()) {
System.out.println("我最终没能战胜黑暗,我不服!!!");
return;
}
hero.hurt(this);
}
}
(3)、设置一个工具类
package Newgame;
public interface Constant {
public static final int INILIVE = 100;
public static final int INIATT = 3;
public static final int INIDEF = 2;
public static final int INTEXP = 1;
public static final int LOSELIFE = 2;
public static final int TYPEATTR = 3;
public static final int INITAILE = 10;
public static final int DODGE = 10;
public static final int MAXEXP = 50;
}
(4)、设置一个测试类
package Newgame;
public class TextGame {
public static void main(String[] args) {
Hero h1 = new Hero("辛巴",18,'男',"神龙🗡");
Monster m1 = new Monster("树妖",100,2,1,"藤曼缠身",2);
h1.fight(m1);
h1.fight(m1);
m1.kill(h1);
h1.fight(m1);
h1.fight(m1);
m1.kill(h1);
h1.fight(m1);
m1.kill(h1);
h1.status();
m1.kill(h1);
}
}
(5)、看我们的运行结果
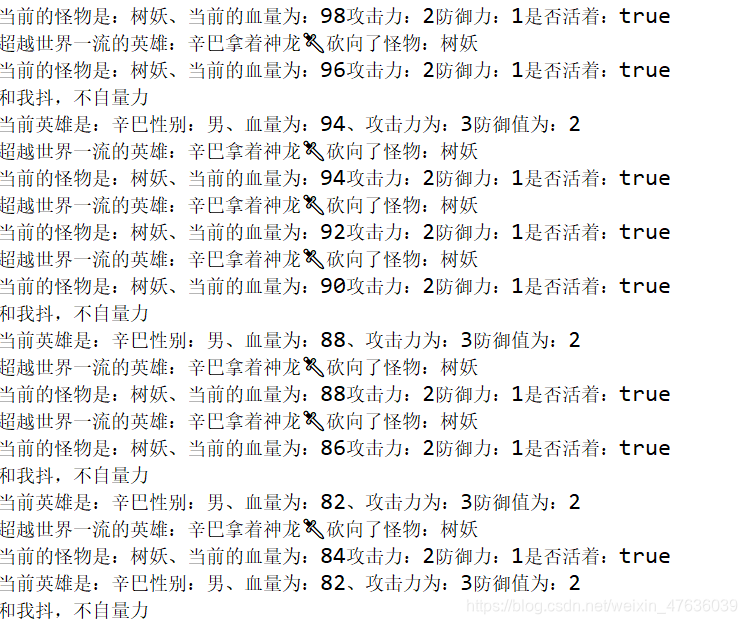
一个利用java制作的小游戏就制作完成了,中间还有些不足之处,可以考虑添加英雄等级提升的方法,有感兴趣的小伙伴可以自己尝试一下~