思维导图
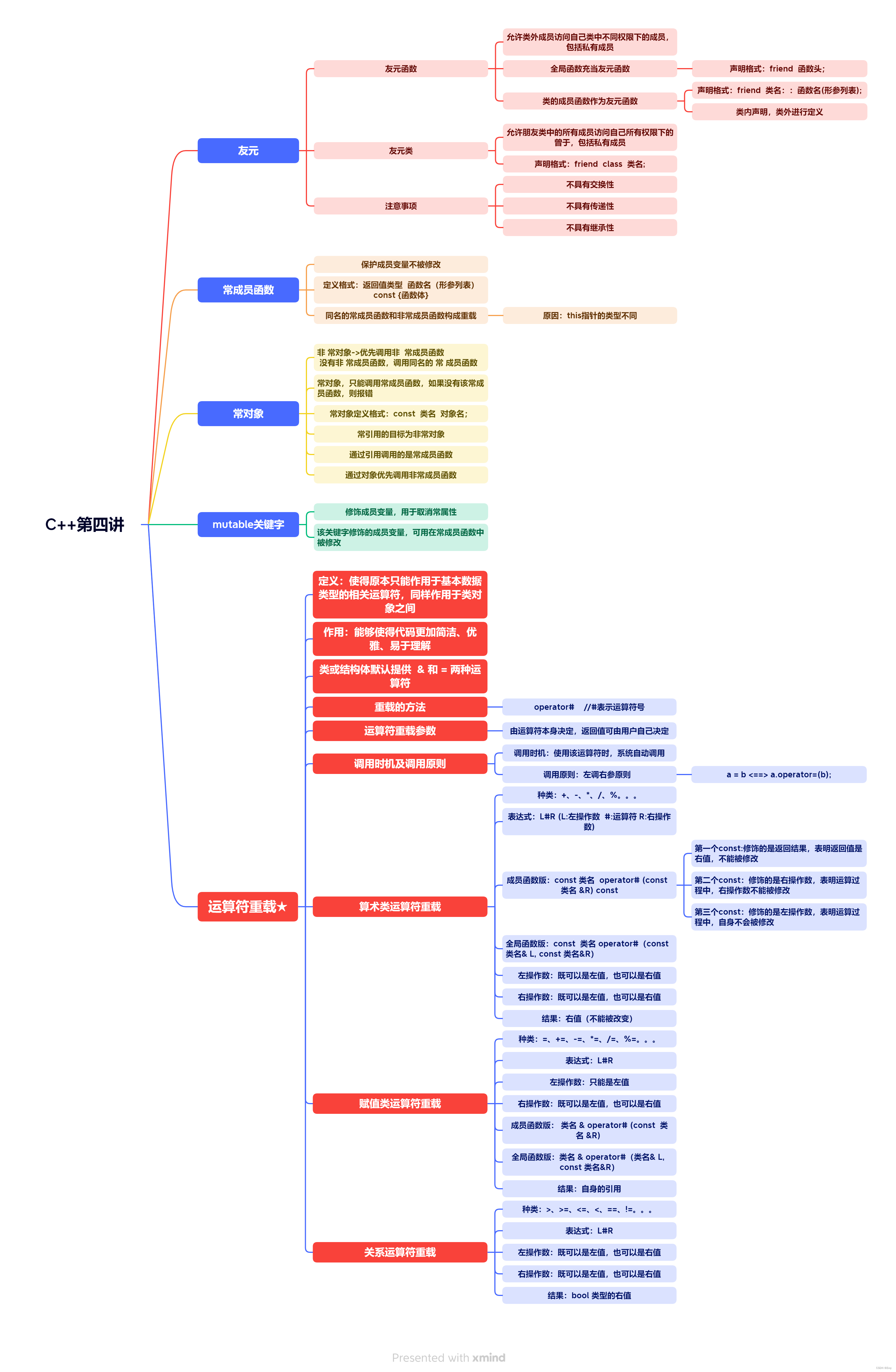
仿照string类,实现myString类
/*
---------------------------------
@author:YoungZorn
created on 2023/7/19 19:20.
---------------------------------
*/
#include<iostream>
#include<cstring>
using namespace std;
class myString
{
private:
char *str; //记录c风格的字符串
int size; //记录字符串的实际长度
public:
//无参构造
myString()
{
size = 10;
str = new char[size]; //构造出一个长度为10的字符串
strcpy(str,""); //初始化myString对象str为空串
}
//有参构造
myString(const char *s) //string s("hello world")
{
size = strlen(s);
str = new char[size+1]; //末尾添加 '\0'
strcpy(str, s); //--->str = "hello world"
}
//拷贝构造
myString(const myString & other):str(new char(*other.str)),size(other.size){}
//析构函数
~myString(){delete str;}
//拷贝赋值函数
myString& operator=(const myString& other){
if(this != &other){
delete str; //释放原有的内存
this->size = other.size;
this->str = new char(*(other.str));
}
return *this;
}
//判空函数
bool isEmpty(){
if(strcmp(str,"") == 0){
return true;
}
return false;
}
//size函数
int strSize(){
if (isEmpty()){
return -1;
}
return strlen(str);
}
//c_str函数
const char *str_c_str()const{
return str; //返回一个C风格的字符串
}
//at函数
char &at(int pos){
if (isEmpty()){
const int &ref = -1;
return (char &)ref;
}
return str[pos]; //返回字符串下标的字符
}
//加号运算符重载 (+)
const myString operator+ (const myString & R) const{
int newSize = size + R.size; //新字符串的长度
char *newStr = new char[newSize+1]; //分配新字符串内存
strcpy(newStr, this->str);
strcat(newStr,R.str);
return myString(newStr);
}
//加等于运算符重载 (+=)
const myString operator+= (const myString & R){
size += R.size; //更新size
strcat(this->str,R.str); //将R.str追加到str上
return myString(this->str);
}
//关系运算符重载 (>)
bool operator> (const myString & R) const {
int flag = strcmp(this->str,R.str);
if (flag > 0)
return true;
return false;
}
};
int main(){
myString string1 = "hello";
bool flag = string1.isEmpty();
cout<<flag<<endl;
cout<<string1.strSize()<<endl;
cout<<"at[0]:"<<string1.at(0)<<endl;
myString s1 = "qwe";
myString s2 = "asd";
cout<<(s1>s2)<<endl; //判断 s1 和 s2 的大小
s1 += s2;
cout<<"s1 += s2 = "<<s1.str_c_str()<<endl; // +=
cout<<s1.str_c_str()<<endl;
myString s3 = "aaa";
myString s4 = "bbb";
myString s5 = s3 + s4;
cout<<"s3 + s4 = "<<s5.str_c_str()<< endl; // +
return 0;
}