文章目录
- 一、准备工作
- 1. 注册微信小程序,并开通订阅消息功能。
- 2. 获取小程序的AppID和AppSecret。
- 3. 在微信小程序管理后台,设置提醒模板,并获取模板ID。
- 4. 小程序端需要获取用户订阅允许提醒的权限
- (1)引导用户触发订阅
- (2)编写订阅消息的JavaScript代码
- (3)用户授权订阅
- (4)后台发送订阅消息
- (5)注意事项
- 二、实现步骤
- 1. 获取Access Token
- 2. 用户订阅消息
- 3. 发送订阅消息
- 三、总结
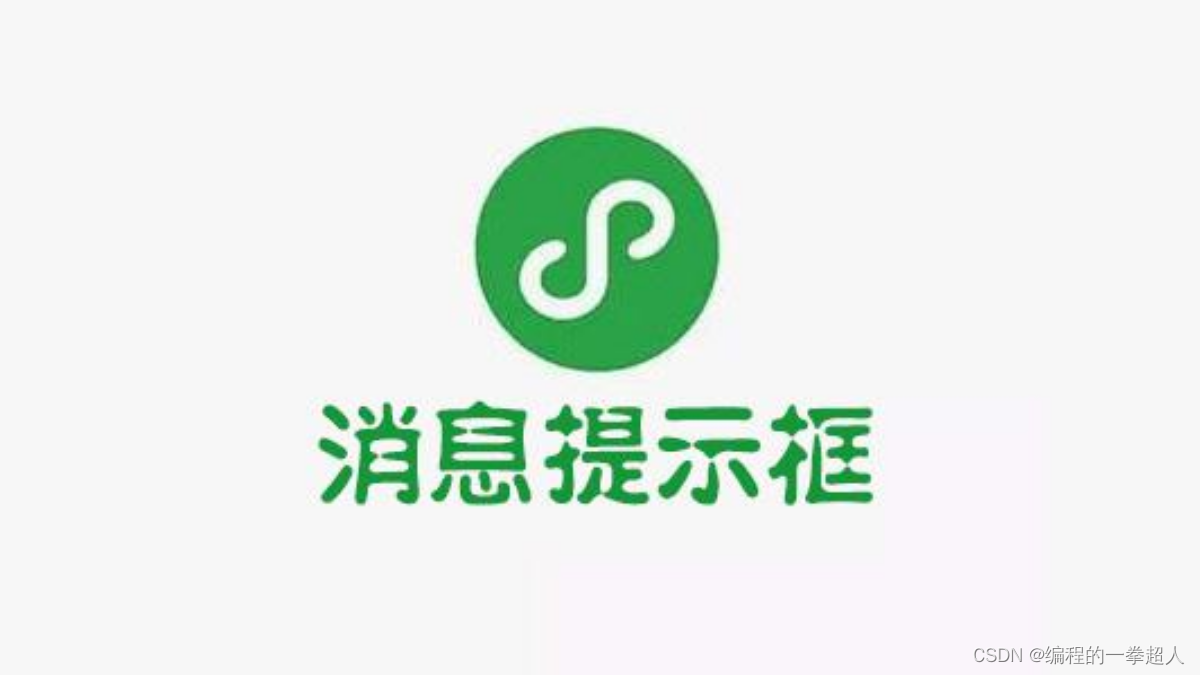
微信小程序订阅消息提醒功能,允许开发者向用户提供一种主动推送消息的机制。通过该功能,用户可以订阅感兴趣的内容,一旦有更新,开发者就可以通过微信平台向用户发送消息提醒。本文将介绍如何使用Java实现微信小程序订阅消息提醒,并对接微信小程序提醒模板。
一、准备工作
1. 注册微信小程序,并开通订阅消息功能。
2. 获取小程序的AppID和AppSecret。
3. 在微信小程序管理后台,设置提醒模板,并获取模板ID。
4. 小程序端需要获取用户订阅允许提醒的权限
在小程序中,要获取用户订阅允许提醒的权限,需要遵循以下步骤:
- 模板配置:首先,在微信公众平台后台配置好订阅消息模板,并获取模板ID。
- 小程序代码编写:在小程序中编写调用订阅消息API的代码。
- 用户触发订阅:通过用户点击某个按钮或完成某个操作来触发订阅消息的请求。
- 用户授权:用户会看到一个弹窗,询问是否允许订阅该消息。
- 后台发送订阅消息:一旦用户同意订阅,后台就可以根据模板ID和用户OpenID发送订阅消息。
下面是具体的实现步骤:
(1)引导用户触发订阅
在小程序中,通常会在用户完成某个操作(如查看详情、下单等)后,提供一个按钮引导用户订阅相关内容。
<button open-type="订阅消息" bindtap="subscribeMessage">订阅消息</button>
(2)编写订阅消息的JavaScript代码
在页面的js
文件中,编写subscribeMessage
函数,用于调用微信的wx.requestSubscribeMessage
接口。
Page({
subscribeMessage() {
wx.requestSubscribeMessage({
tmplIds: ['你的模板ID'],
success(res) {
if (res.errMsg === 'requestSubscribeMessage:ok') {
// 用户同意订阅,可以在这里处理后续逻辑,如发送订阅消息等
if (res['你的模板ID'] === 'accept') {
// 用户同意订阅该模板ID的消息
// 可以在这里调用服务器接口,将用户的订阅信息保存到数据库
}
}
},
fail(err) {
// 订阅失败的处理
console.log(err);
}
});
}
});
(3)用户授权订阅
当用户点击“订阅消息”按钮时,会弹出授权窗口,询问用户是否允许订阅该消息。
(4)后台发送订阅消息
一旦用户同意订阅,后台服务器就可以根据用户的OpenID和模板ID发送订阅消息。这通常会在用户完成某个操作后触发,例如下单成功、预约成功等。
(5)注意事项
- 订阅消息模板的内容和格式需要提前在微信公众平台配置好,且需要审核通过后才能使用。
- 用户同意订阅后,开发者可以在7天内向用户发送一条订阅消息,超过这个时间期限,需要用户重新授权。
- 订阅消息的使用需要遵守微信小程序的相关政策和规定,不得用于发送广告、营销等内容。
通过以上步骤,小程序端可以获取用户订阅允许提醒的权限,并在合适的时机向用户发送订阅消息。
二、实现步骤
1. 获取Access Token
首先,需要通过AppID和AppSecret获取微信小程序的Access Token。Access Token是微信小程序API调用的凭证,具有时效性,需要定时更新。
public static String getAccessToken() {
String url = "https://api.weixin.qq.com/cgi-bin/token?grant_type=client_credential&appid=APPID&secret=APPSECRET";
url = url.replace("APPID", yourAppId).replace("APPSECRET", yourAppSecret);
String result = HttpUtil.get(url);
JSONObject jsonObject = JSON.parseObject(result);
return jsonObject.getString("access_token");
}
2. 用户订阅消息
在小程序中,当用户同意订阅消息时,需要调用微信API进行订阅。
public static void subscribeMessage(String openId, String templateId) {
String accessToken = getAccessToken();
String url = "https://api.weixin.qq.com/cgi-bin/message/subscribe/send?access_token=" + accessToken;
JSONObject data = new JSONObject();
data.put("touser", openId);
data.put("template_id", templateId);
data.put("page", "index");
JSONObject miniprogram = new JSONObject();
miniprogram.put("appid", yourAppId);
data.put("miniprogram", miniprogram);
// 设置消息内容
JSONObject thing1 = new JSONObject();
thing1.put("value", "thing1");
JSONObject thing2 = new JSONObject();
thing2.put("value", "thing2");
JSONObject thing3 = new JSONObject();
thing3.put("value", "thing3");
JSONObject thing4 = new JSONObject();
thing4.put("value", "thing4");
JSONObject thing5 = new JSONObject();
thing5.put("value", "thing5");
JSONObject thing6 = new JSONObject();
thing6.put("value", "thing6");
JSONObject thing7 = new JSONObject();
thing7.put("value", "thing7");
JSONObject thing8 = new JSONObject();
thing8.put("value", "thing8");
JSONObject thing9 = new JSONObject();
thing9.put("value", "thing9");
JSONObject thing10 = new JSONObject();
thing10.put("value", "thing10");
data.put("data", new JSONObject().fluentPut("thing1", thing1)
.fluentPut("thing2", thing2)
.fluentPut("thing3", thing3)
.fluentPut("thing4", thing4)
.fluentPut("thing5", thing5)
.fluentPut("thing6", thing6)
.fluentPut("thing7", thing7)
.fluentPut("thing8", thing8)
.fluentPut("thing9", thing9)
.fluentPut("thing10", thing10));
String result = HttpUtil.post(url, data.toJSONString());
System.out.println("订阅消息结果:" + result);
}
3. 发送订阅消息
当需要向用户发送订阅消息时,可以调用以下方法:
public static void sendSubscribeMessage(String openId, String templateId) {
String accessToken = getAccessToken();
String url = "https://api.weixin.qq.com/cgi-bin/message/subscribe/send?access_token=" + accessToken;
JSONObject data = new JSONObject();
data.put("touser", openId);
data.put("template_id", templateId);
data.put("page", "index");
// 设置消息内容
JSONObject thing1 = new JSONObject();
thing1.put("value", "更新内容1");
JSONObject thing2 = new JSONObject();
thing2.put("value", "更新内容2");
data.put("data", new JSONObject().fluentPut("thing1", thing1).fluentPut("thing2", thing2));
String result = HttpUtil.post(url, data.toJSONString());
System.out.println("发送订阅消息结果:" + result);
}
三、总结
通过以上步骤,我们可以实现Java微信小程序订阅消息提醒,并对接微信小程序提醒模板。在实际开发中,需要根据业务需求调整消息内容和发送时机。同时,要注意遵守微信小程序平台的相关规定,确保订阅消息功能的合规使用。