1.文件编程的概念(文件IO)
文件编程其实就是操作文件,对文件进行一系列的操作,比如文件的打开、关闭、读写等操作。在Windows操作系统下可以直接使用鼠标来进行这些文件的操作,也就是点点...,但是Windows下也可以使用cmd指令来操作;而在Linux操作系统下就需要去调用API访问内核,从而进行对文件的操作。
2.文件的打开与创建
打开文件open()函数
2.1函数需要包含头文件:
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
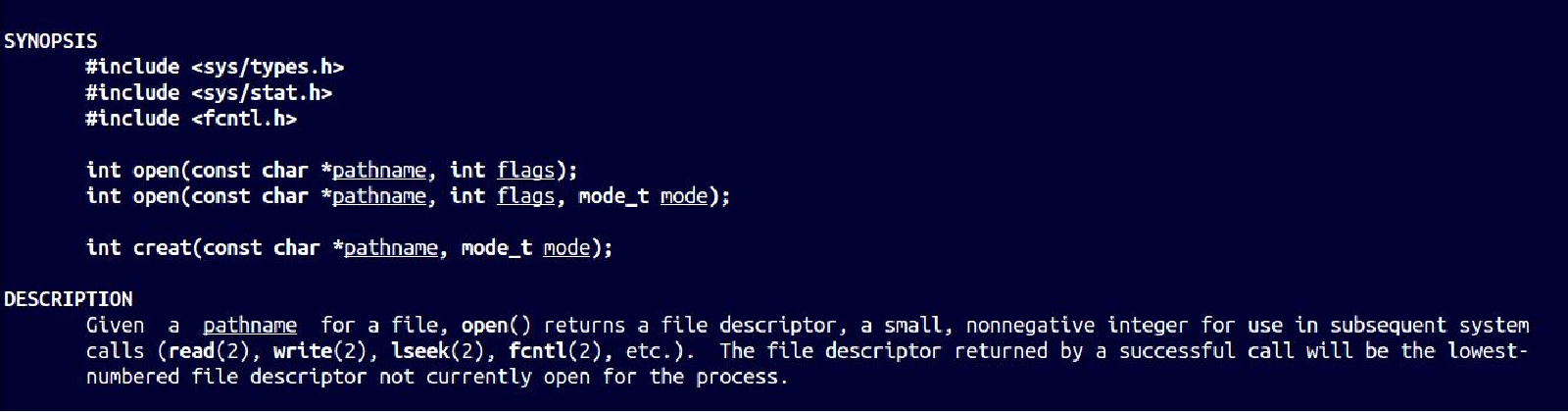
2.2参数说明

下面flags参数可以自行测试,我将以 O_CREAT为例,其他几个也是大同小异
Pathname:
要打开的文件名(含路径,缺省为当前路径)
Flags:
O_RDONLY 只读打开 O_WRONLY 只写打开 O_RDWR 可读可写打开
当我们附带了权限后,打开的文件就只能按照这种权限来操作。
以上这三个常数中应当只指定一 个。下列常数是可选择的:
O_CREAT 若文件不存在则创建它。使用此选项时,需要同时说明第三个参数mode,用其说明该 新文件的存取许可权限。
O_EXCL 如果同时指定了OCREAT,而文件已经存在,则出错。
O_APPEND 每次写时都加到文件的尾端。
O_TRUNC 属性去打开文件时,如果这个文件中本来是有内容的,而且为只读或只写成功打开, 则将其长度截短为0。
Mode:
一定是在flags中使用了O_CREAT标志,mode记录待创建的文件的访问权限
通过man手册 查看open函数的指令 :man 2 open即可
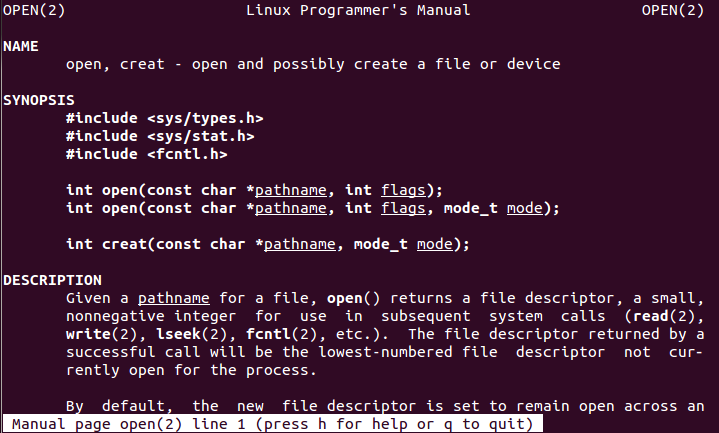
2.3 此段代码 打开文件file1,是需要文件已经存在的情况下;
下一段代码将演示当文件不存在时需先创建文件后方能打开。
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
//此段代码 打开文件file1,是需要文件已经存在的情况下;
//下一段代码将演示当文件不存在时需先创建文件后方能打开。
int main()
{
int fd;
fd = open("./file1",O_RDWR);
printf("fd=%d\n",fd);
return 0;
}
打开成功,返回一个文件操作符这个数大于0,失败返回-1;
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
int main()
{
int fd;
fd = open("./file1",O_RDWR);
if(fd < 0)
{
printf("open file1 fail!\n");
fd = open("./file1",O_RDWR|O_CREAT,0600);
//0600 表示设置文件访问权限的初始值 -rw---可读可写 (解释如下)
if(fd > 0)
{
printf("creat file1 succeed\n");
}
}
return 0;
}
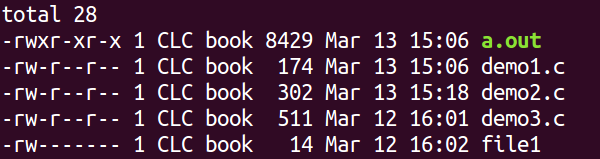
0600表示分配给文件的权限
一共四位数,第一位数表示gid/uid一般不用,剩下三位分别表示owner,group,other的权限
每个数可以转换为三位二进制数,分别表示rwx(读,写,执行)权限,为1表示有权限,0无权限
如6是上面第二个数,可以表示为二进制数110,表示owner有读,写权限,无执行权限
创建文件creat()函数
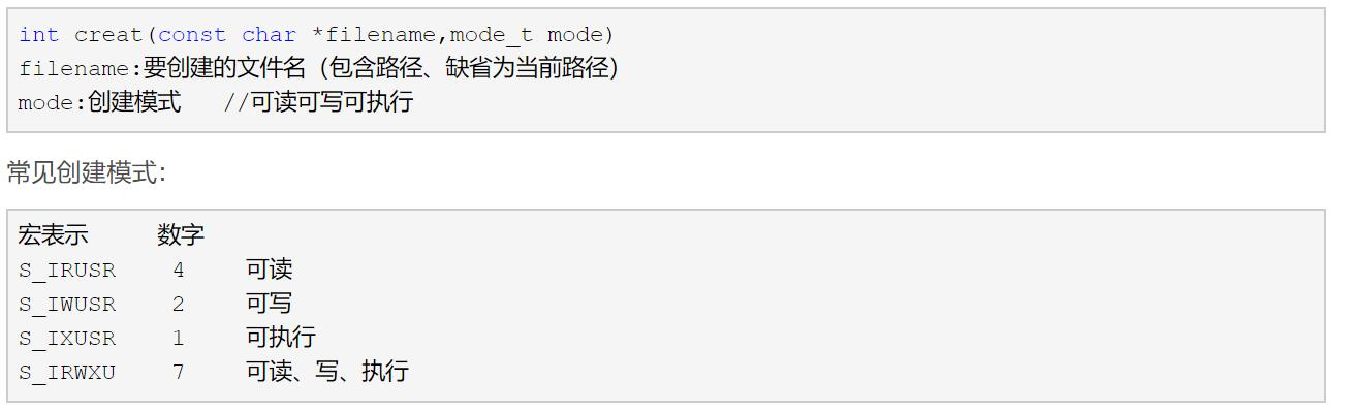
3.文件的读写操作
写文件write() 函数
3.1函数需要包含头文件:#include <unistd.h>
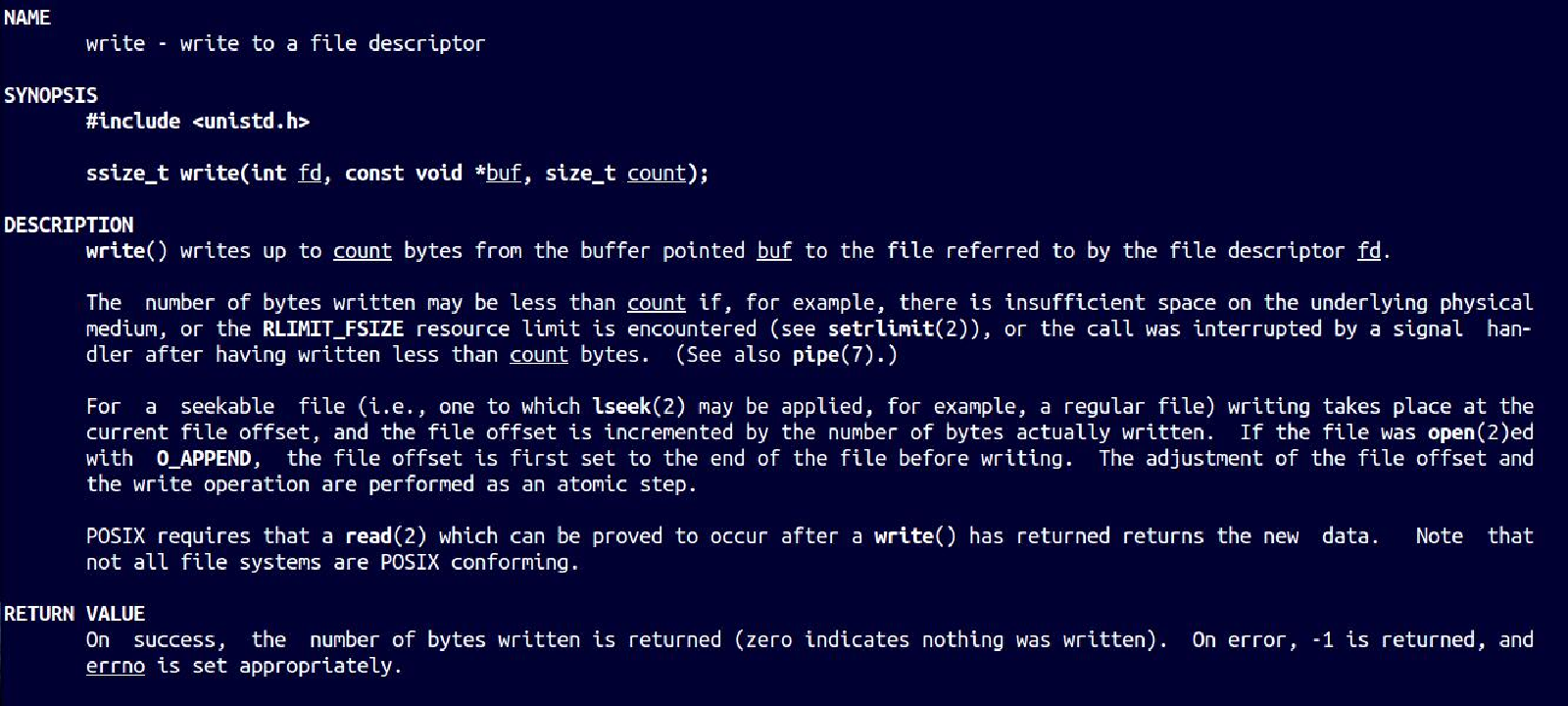
3.2参数说明
#include <unistd.h>
ssize_t write(int fd, const void *buf, size_t count);
int fd:文件描述符(open的返回值,是一个索引);
const void*buf:缓冲区(位置);
size_t count:写入文件的大小;
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
//创建并写入内容
int main()
{
int fd;
char *buf = "ni hen shuai!";
fd = open("./file1",O_RDWR);
if(fd < 0)
{
printf("open file1 fail!\n");
fd = open("./file1",O_RDWR|O_CREAT,0600);
if(fd > 0)
{
printf("creat file1 succeed\n");
}
}
printf("open succeed:file1 = %d\n",fd);
//ssize_t write(int fd, const void *buf, size_t count);
write(fd,buf,strlen(buf));//此处为什么不能使用sizeof()的原因,可自行查阅
close(fd);
return 0;
}
3.3读文件read()函数
函数需包含头文件:#include <unistd.h>
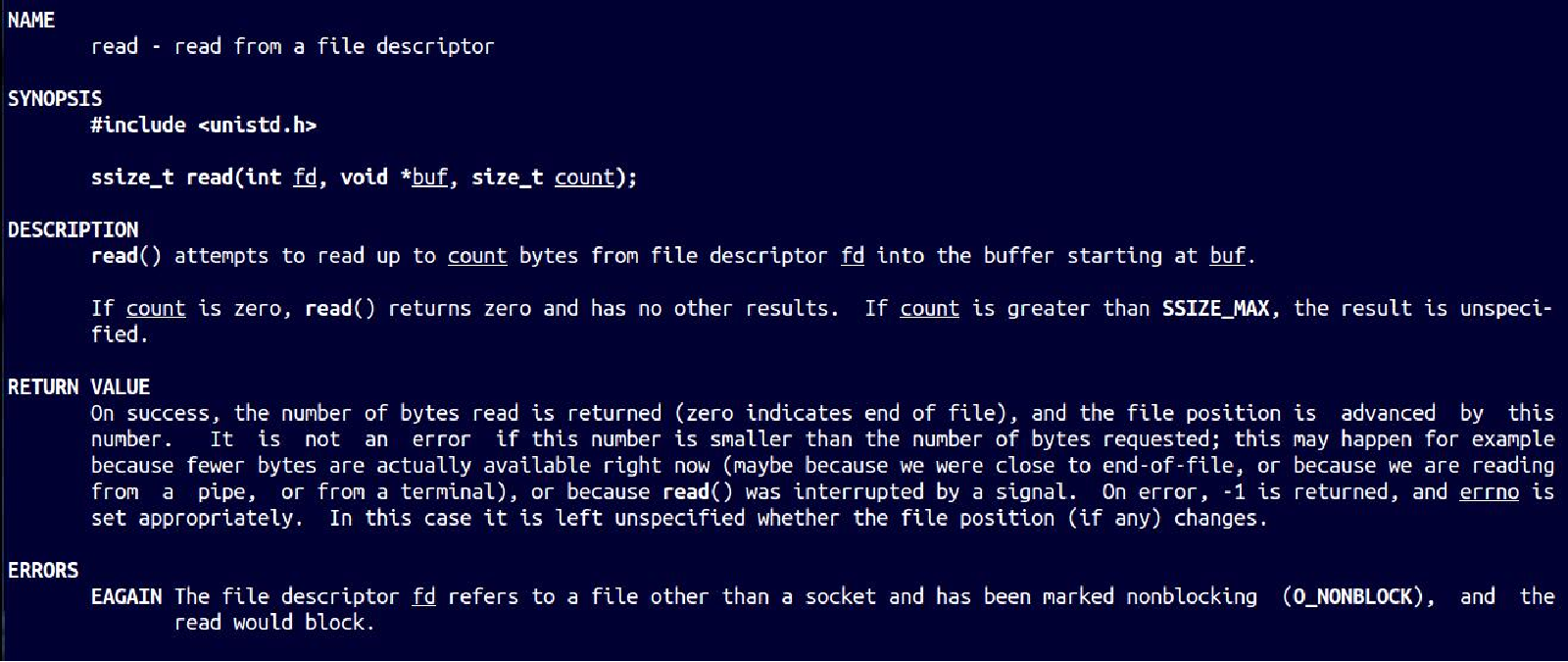
参数说明
#include <unistd.h>
ssize_t read(int fd, void *buf, size_t count);
int fd:文件描述符;
void *buf:用buf来接收要读取的字符串内容
size_t count:读取的内容;
读取成功 返回字节数;
读取失败 返回-1
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <stdio.h>
#include <unistd.h>
#include <string.h>
#include <stdlib.h>
int main()
{
int fd;
char *buf = "ni hen shuai!";
fd = open("./file1",O_RDWR);
if(fd < 0)
{
printf("open file1 fail!\n");
fd = open("./file1",O_RDWR|O_CREAT,0600);
if(fd > 0)
{
printf("creat file1 succeed\n");
}
}
printf("open succeed:file1 = %d\n",fd);
//ssize_t write(int fd, const void *buf, size_t count);
int n_write = write(fd,buf,strlen(buf));
if(n_write != -1)
{
printf("write %d byte to file1\n",n_write);
}
close(fd);
fd = open("./file1",O_RDWR);
char *readBuf;
readBuf = (char *)malloc(sizeof(char) *n_write+1);
//ssize_t read(int fd, void *buf, size_t count);
int n_read = read(fd, readBuf, n_write);
printf("read %d, context:%s\n",n_read,readBuf);
close(fd);
return 0;
}
运行效果:


剩下的将在下一篇等你
光标定位 lseek()函数
关闭 文件close()函数 未完待续...