IOC
IOC本质
- IOC(控制反转),是一种设计思想,DI(依赖注入)是实现IOC的一种方法。没有IOC的程序中,使用面向对象编程,对象的创建以及对象间的依赖关系完全在程序中,对象的创建由程序自己控制。使用IOC的程序中,控制反转后将对象的创建转移给第三方,即获得依赖对象的方式反转了。
- 控制:控制对象的创建,传统 程序的对象是由程序本身控制创建的,使用Spring后,对象是由Spring来创建的。
- 反转:程序本身不创建对象,而是被动的接收对象。
- 依赖注入:利用set方法来进行注入。
- 依赖:bean对象的创建依赖于容器
- 注入:bean对象的中的所有属性,由容器来注入。
创建一个 Maven 项目
解决静态资源导出问题
<build>
<resources>
<resource>
<directory>src/main/resources</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>true</filtering>
</resource>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.properties</include>
<include>**/*.xml</include>
</includes>
<filtering>true</filtering>
</resource>
</resources>
</build>
引入依赖
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.12.RELEASE</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.12</version>
</dependency>
</dependencies>
XML配置实现
编写实体类
package com.xuan.pojo;
public class User {
private Integer userId;
private String userName;
public User(Integer userId, String userName) {
this.userId = userId;
this.userName = userName;
}
public Integer getUserId() {
return userId;
}
public void setUserId(Integer userId) {
this.userId = userId;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
@Override
public String toString() {
return "User{" +
"userId=" + userId +
", userName='" + userName + '\'' +
'}';
}
}
package com.xuan.pojo;
public class Teacher {
private Integer teacherId;
private String teacherName;
public Integer getTeacherId() {
return teacherId;
}
public void setTeacherId(Integer teacherId) {
this.teacherId = teacherId;
}
public String getTeacherName() {
return teacherName;
}
public void setTeacherName(String teacherName) {
this.teacherName = teacherName;
}
@Override
public String toString() {
return "Teacher{" +
"teacherId=" + teacherId +
", teacherName='" + teacherName + '\'' +
'}';
}
}
package com.xuan.pojo;
import java.util.*;
public class Student {
private Integer studentId;
private String studentName;
private Teacher teacher;
private String[] strings;
private List<String> list;
private Map<String, Object> map;
private Set<String> set;
private String wife;
private Properties properties;
public Integer getStudentId() {
return studentId;
}
public void setStudentId(Integer studentId) {
this.studentId = studentId;
}
public String getStudentName() {
return studentName;
}
public void setStudentName(String studentName) {
this.studentName = studentName;
}
public Teacher getTeacher() {
return teacher;
}
public void setTeacher(Teacher teacher) {
this.teacher = teacher;
}
public String[] getStrings() {
return strings;
}
public void setStrings(String[] strings) {
this.strings = strings;
}
public List<String> getList() {
return list;
}
public void setList(List<String> list) {
this.list = list;
}
public Map<String, Object> getMap() {
return map;
}
public void setMap(Map<String, Object> map) {
this.map = map;
}
public Set<String> getSet() {
return set;
}
public void setSet(Set<String> set) {
this.set = set;
}
public String getWife() {
return wife;
}
public void setWife(String wife) {
this.wife = wife;
}
public Properties getProperties() {
return properties;
}
public void setProperties(Properties properties) {
this.properties = properties;
}
@Override
public String toString() {
return "Student{" +
"studentId=" + studentId +
", studentName='" + studentName + '\'' +
", teacher=" + teacher +
", strings=" + Arrays.toString(strings) +
", list=" + list +
", map=" + map +
", set=" + set +
", wife='" + wife + '\'' +
", properties=" + properties +
'}';
}
}
编写配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="teacher" class="com.xuan.pojo.Teacher">
<property name="teacherId" value="1"/>
<property name="teacherName" value="唐僧"/>
</bean>
<bean id="student" class="com.xuan.pojo.Student">
<property name="studentId" value="2"/>
<property name="studentName" value="孙悟空"/>
<property name="teacher" ref="teacher"/>
<property name="strings">
<array>
<value>数组1</value>
<value>数组2</value>
</array>
</property>
<property name="list">
<list>
<value>list1</value>
<value>list2</value>
</list>
</property>
<property name="map">
<map>
<entry key="map1" value="map1"/>
<entry key="map2" value="map2"/>
</map>
</property>
<property name="set">
<set>
<value>set1</value>
<value>set2</value>
</set>
</property>
<property name="wife">
<null/>
</property>
<property name="properties">
<props>
<prop key="properties1">properties1</prop>
<prop key="properties2">properties2</prop>
</props>
</property>
</bean>
<bean id="user" class="com.xuan.pojo.User">
<constructor-arg name="userId" value="1"/>
<constructor-arg name="userName" value="观音菩萨"/>
</bean>
</beans>
测试
package com.xuan;
import com.xuan.pojo.Student;
import com.xuan.pojo.Teacher;
import com.xuan.pojo.User;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MyTest {
@Test
public void test01(){
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
Teacher teacher = context.getBean("teacher", Teacher.class);
Student student = context.getBean("student", Student.class);
System.out.println(teacher);
System.out.println(student);
}
@Test
public void test02(){
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
User user = context.getBean("user", User.class);
System.out.println(user);
}
}
最终项目包结构
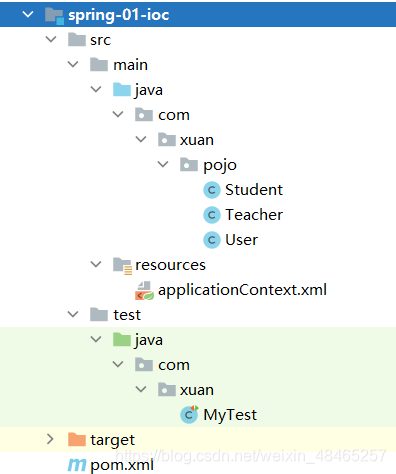
Bean的作用域
- 单例模式(Spring默认机制)
- 原型模式:每次从容器中get的时候,都会产生一个新对象。
注解实现
编写配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd">
<context:component-scan base-package="com.xuan"/>
<context:annotation-config/>
</beans>
编写实体类
package com.xuan.pojo;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class User {
@Value("1")
private Integer userId;
@Value("孙悟空")
private String userName;
public Integer getUserId() {
return userId;
}
public void setUserId(Integer userId) {
this.userId = userId;
}
public String getUserName() {
return userName;
}
public void setUserName(String userName) {
this.userName = userName;
}
@Override
public String toString() {
return "User{" +
"userId=" + userId +
", userName='" + userName + '\'' +
'}';
}
}
编写dao层
package com.xuan.dao;
import com.xuan.pojo.User;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Repository;
@Repository
public class UserDao {
@Autowired
private User user;
public void hello(){
System.out.println("==="+user.getUserId()+"==="+user.getUserName()+"===");
}
}
编写service层
package com.xuan.service;
import com.xuan.dao.UserDao;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class UserService {
@Autowired
private UserDao userDao;
public void hello(){
userDao.hello();
}
}
编写controller层
package com.xuan.controller;
import com.xuan.service.UserService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
@Controller
public class UserController {
@Autowired
private UserService userService;
public void hello(){
userService.hello();
}
}
测试
package com.xuan.controller;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class TestUserController {
@Test
public void testHello(){
ApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
UserController userController = context.getBean("userController", UserController.class);
userController.hello();
}
}
最终项目包结构
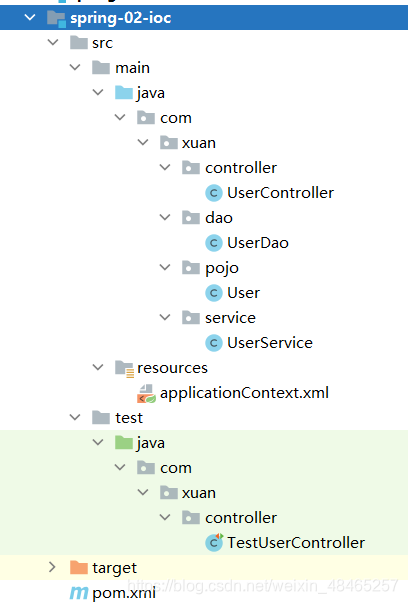