某班期末考试科目为数学(MT)、英语(EN)和物理(PH),有最多不超过30人参加考试。考试后要求:
(1)计算每个学生的总分和平均分;
(2)按总分成绩由高到低排出成绩的名次;
(3)打印出名次表,表格内包括学生编号、各科分数、总分和平均分;
(4)任意输入一个学号,能够查找出该学生在班级中的排名及其考试分数。
#include<iostream>
using namespace std;
void bubble(double a[], int);
int main()
{
string stu_names[]{ "01","02","03","04","05" };
string course_names[]{ "数学","英语","物理" };
const int Row = 5;
const int Col = 3;
double scores[Row][Col];
//输入1~30号学生的成绩
for (int i = 0; i < Row; i++)
{
for (int j = 0; j < Col; j++)
{
cout << stu_names[i] << "的" << course_names[j] << "成绩:";
cin >> scores[i][j];
}
}
//求学生的总分与平均分
const int a = 5, b = 5;
double zongfen[a];
double zongfen1[a];//多复制一个总分的数组,因为使用冒泡排序之后数组中的元素顺序已经改变
double pinjunfen[b];
for (int i = 0; i < Row; i++)
{
double sum = 0;
double ave = 0;
for (int j = 0; j < Col; j++)
{
sum += scores[i][j];
ave = sum / 3;
}
zongfen[i] = sum;
zongfen1[i] = sum;
pinjunfen[i] = ave;
}
//因为本人电脑‘/t’无法显示(不知道为什么,所以用空格代替
cout << "学生编号" << " " << "数学" <<" " << "英语" <<" " << "物理" << " " << "总分" <<" " << "平均分" << endl;
for (int i = 0; i < Row; i++)
{
cout << stu_names[i] << " ";
for (int j = 0; j < Col; j++)
{
cout << scores[i][j] << " ";
}
cout << zongfen[i] << " " << pinjunfen[i] << endl;
}
//给总分排序
bubble(zongfen, Row);
//输入学号查询
for (int d = 0; d < 30; d++)//多加一个循环可以起到一直查找的作用
{
string c;
cout << "请输入想要查询的学生的成绩:";
cin >> c;
//查询学生各科分数与总分
for (int i = 0; i < Row; i++)
{
if (stu_names[i] == c)
{
cout << "总分:" << zongfen1[i];
for (int j = 0; j < Row; j++)
{
if (zongfen1[i] == zongfen[j])
cout << "排名:第" << j + 1 << " " << endl;
}
}
}
}
return 0;
}
//冒泡排序
void bubble(double a[], int size)
{
double temp =0;//临时变量
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size - 1; j++)
{
if (a[j] < a[j + 1])
{
temp = a[j];
a[j] = a[j + 1];
a[j + 1] = temp;
}
}
}
}
**打印结果**
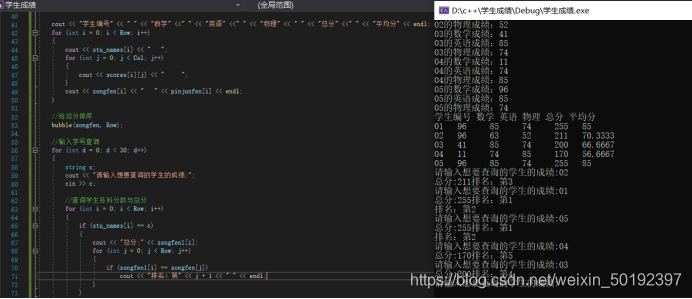
初学者,望指正