Android基础知识(二)
UI的控件使用
控件的用法基本相似,给控件定义一个id,在指定控件的宽度和高度,然后再加入一些控件特有的属性。
碎片(Fragment)
一种可以嵌入在活动中的Ui片段,是一种迷你型的活动,它能让程序更加充分合理的利用大屏幕空间。在平板应用广泛。
静态加载Fragment实现流程:
静态加载Fragment的流程
1、定义碎片的Fragment的xml布局文件
2、自定义Fragment类,需继承Fragment类或其子类,同时实现onCreateView()方法在方法中,通过inflater.inflate加载布局文件,接着返回view即可
3、在需要加载Fragment的Activity对应布局文件中的name属性需要为全限定类名即包名,fragment
4、Activity调用setContentView()加载布局文件即可。
LayoutInflater inflater是加载一个布局文件。
findViewById则是从布局文件中查找一个控件。
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
//第二个参数表示一个容器
//第三个参数保存当前的状态,在活动的生命周期中,只要离开了可见阶段,活动很可能就会被进程终止,这种机制能保存当时的状态
View view=inflater.inflate(R.layout.right_fragment,container,false);
return view;
}
简单试验一下:
先键左右碎片的class类和布局
1.left_fragment.xml布局
<LinearLayout xmlns:android=“http://schemas.android.com/apk/res/android”
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:text="Button"
/>
2.right_fragment.xml布局
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:textSize="20sp"
android:text="This is right fragment"
/>
3.LeftFragment public class LeftFragment extends Fragment { @Override public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) { View view = inflater.inflate(R.layout.left_fragment,container,false); return view; } } 4.RightFragment public class RightFragment extends Fragment {
@Override
public View onCreateView( LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view=inflater.inflate(R.layout.right_fragment,container,false);
//第二个参数表示一个容器
//第三个参数保存当前的状态,在活动的生命周期中,只要离开了可见阶段,活动很可能就会被进程终止,这种机制能保存当时的状态
return view;
}
}
5.最后写活动布局Activity_suipian1.xml
<fragment
android:id="@+id/left_fragment"
android:name="com.example.pingban.LeftFragment"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="2" />
<fragment
android:id="@+id/right_fragment"
android:name="com.example.pingban.RightFragment"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1" />
结果如下图: 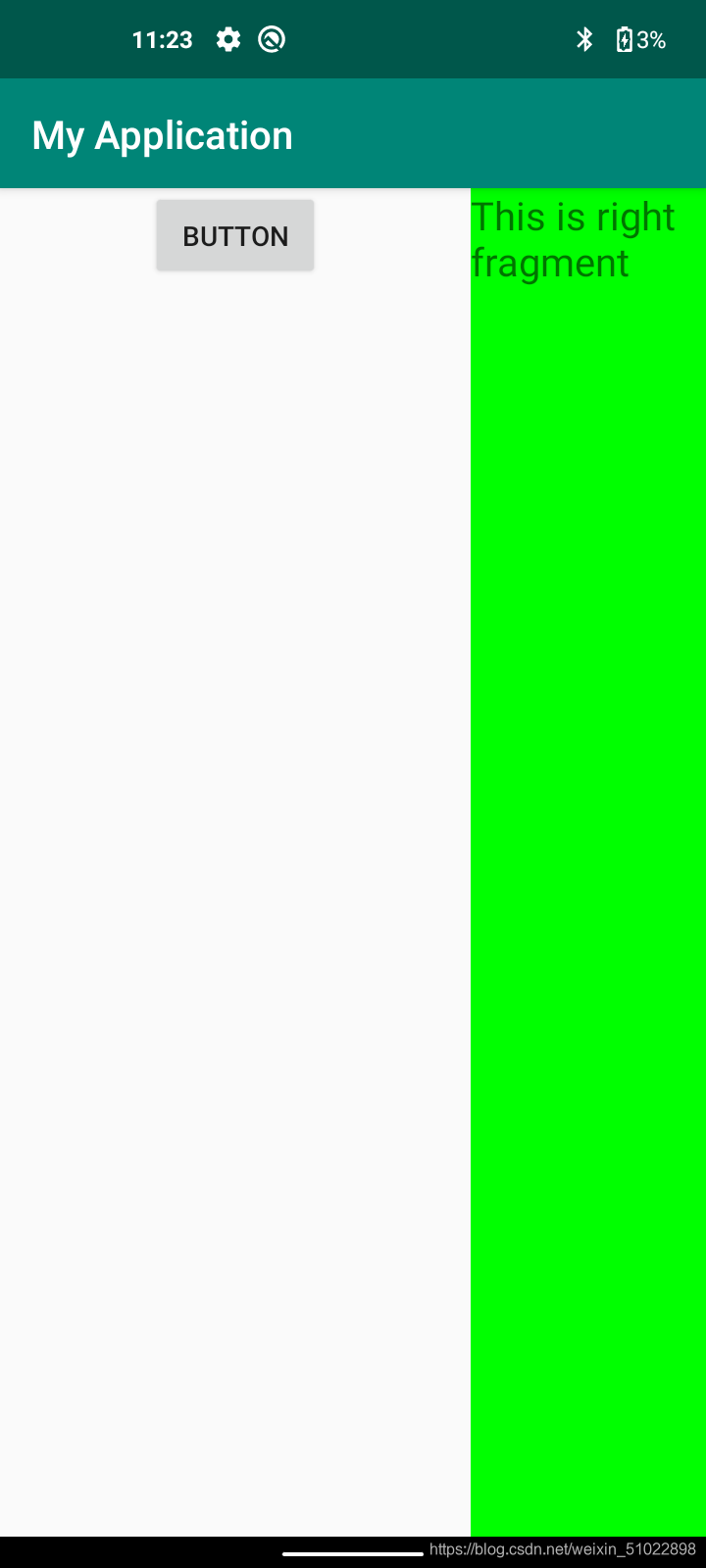
动态添加碎片
我们在静态的基础上修改
分五部
1.创建待添加的碎片实列。
2.获取FragmentManager,在活动中可以直接通过调getSupportFragmentManager()方法的到
3.开启一个事务,通过调用beginTransaction方法开启
4.像容器内添加或者替换碎片,一般使用replace()方法实现,需要传入容器的id和待添加的碎片的实列。
5.提交事务调用commit()方法来完成。
在静态的基础上点一下左边的按钮替换右边的布局
在静态的基础上添加AnotherRightFragment类
public class AnotherRightFragment extends Fragment {
@Override
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
View view=inflater.inflate(R.layout.another_right_fragment,container,false);
//第二个参数表示一个容器
//第三个参数保存当前的状态,在活动的生命周期中,只要离开了可见阶段,活动很可能就会被进程终止,这种机制能保存当时的状态
return view;
}
}
和xml布局
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal"
android:textSize="20sp"
android:text="This is another right fragment"
/>
然后修改主活动 public class ActivitySuipian1 extends AppCompatActivity implements View.OnClickListener{
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_suipian1);
Button button=(Button) findViewById(R.id.button);
button.setOnClickListener(this);
replaceFragment(new RightFragment());
}
@Override
public void onClick(View view) {
switch (view.getId()){
case R.id.button:
replaceFragment(new AnotherRightFragment());
break;
default:
break;
}
}
private void replaceFragment(Fragment fragment){
FragmentManager fragmentManager=getSupportFragmentManager();
FragmentTransaction transaction=fragmentManager.beginTransaction();
transaction.replace(R.id.right_layout,fragment);
transaction.commit();
} }
然后要修改主活动布局,不然会重叠如图:
<fragment
android:id="@+id/left_fragment"
android:name="com.example.pingban.LeftFragment"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1" />
<!--<fragment
android:id="@+id/right_fragment"
android:name="com.example.pingban.RightFragment"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1" />
–>
<!--最简单的一种布局所有控件会摆放在布局的坐上角,这里仅需要在布局里放一个碎片,不需要任何定位适合此布局-->
不理解为什么在这放这个布局,还有布局中可以套布局吗?
<FrameLayout
android:id="@+id/right_layout"
android:layout_width="0dp"
android:layout_height="match_parent"
android:layout_weight="1"/>
碎片的管理和事务
管理:Activity管理Fragment主要依靠FragmentManager可以dindFragmentById()获取指定的fragment也可以调用popBackStack()方法弹出后台Fragment也可以调用addToBackStack(null)加入栈或者监听后台栈的变换:addOnBackStackChangeListener
事务:如果是增删替换Fragment的话,则需要借助FragmentTransaction对象;同时添加Fragment的操作,记得操作完成后在使用commit()方法提交事务哦!
碎片和活动之间如何通信的
碎片都是嵌入在活动中显示的,但是他们都各自存在一个独立的类中,他们之间没有明显的方式来直接进行通信。
1.活动中调用碎片的方法:FragmentManager提供了一个类似于findViewById()的方法专门用于从布局文件中获取碎片的实列。调用FragmentManage的方法,可以在活动中得到相应的实列,轻松调用碎片里的方法。
RightFRagment rightFragment=(RightFragment) getFragmentManager().findFragmentById(R.id.right_fragment);
2.碎片中调活动的方法:在每个碎片中都可以通过调用getActivity()方法来得到和当前碎片相关联的活动实列。
MainActivity activity = (MainActivity) getActivity();
3.碎片和碎片之间的通信
在一个碎片中可以得到与它相关链的活动,然后再通过这个活动获取另一个碎片的实列。
碎片和活动交互
在碎片中模拟返回栈
按下back键后程序会直接推出,无妨返回点击按钮之钱的状态,模仿返回栈效果
加入一个方法即可,该方法可以接收一个名字用于描述返回栈的状态,一般传入null即可
private void replaceFragment(Fragment fragment){
FragmentManager fragmentManager=getSupportFragmentManager();
FragmentTransaction transaction=fragmentManager.beginTransaction();
transaction.replace(R.id.right_layout,fragment);
transaction.addToBackStack(null);//该方法将事务添加到返回栈中,
transaction.commit();
}
碎片的生命周期
碎片的几个子类
子类用的不多
一般都是重写Fragment,inflate加载布局完成相应业务了,子类用的不多。
对话框:DialogFragment
列表:ListFragment
选项设置:PreferenceFragment
WebView界面:WebViewFragment