题目
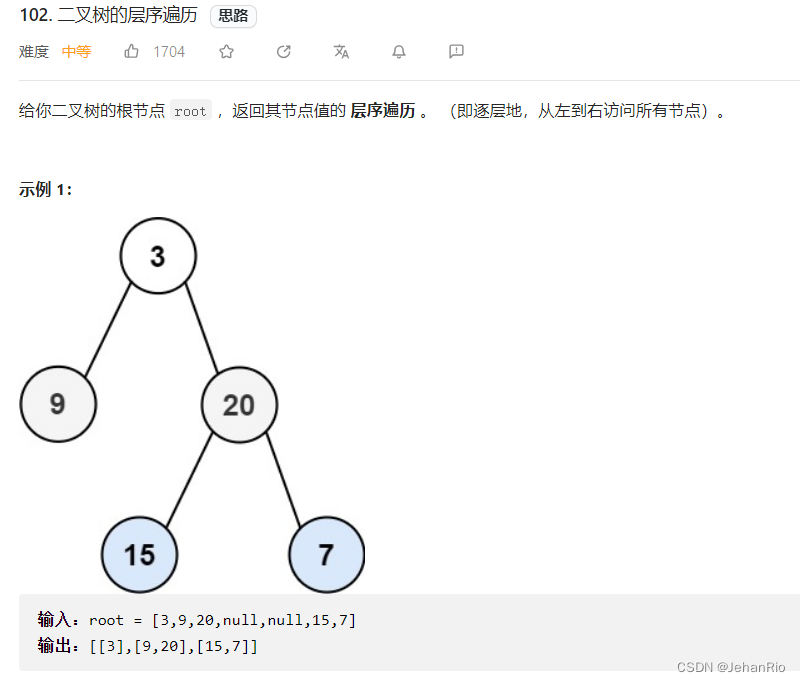
Leetcode 102. 二叉树的层序遍历
代码(首刷自解)
class Solution {
public:
vector<vector<int>> levelOrder(TreeNode* root) {
vector<vector<int>> ans;
if(!root)
return ans;
deque<TreeNode*> q;
q.push_back(root);
while(!q.empty()) {
vector<int> tmp;
int sz = q.size();
for(int i = 0; i < sz; i++) {
auto node = q.front();
q.pop_front();
tmp.push_back(node->val);
if(node->left) q.push_back(node->left);
if(node->right) q.push_back(node->right);
}
ans.push_back(tmp);
}
return ans;
}
};
代码(8.14 二刷自解)
class Solution {
public:
vector<vector<int> > levelOrder(TreeNode* root) {
queue<TreeNode*> q;
vector<vector<int>> res;
if(!root)
return res;
q.push(root);
while(!q.empty()) {
int sz = q.size();
vector<int> tmp;
for(int i = 0; i < sz; i++) {
auto front = q.front();
q.pop();
tmp.push_back(front->val);
if(front->left) q.push(front->left);
if(front->right) q.push(front->right);
}
res.push_back(tmp);
}
return res;
}
};
func levelOrder(root *TreeNode) [][]int {
q := []*TreeNode{}
res := [][]int{}
if root == nil {
return res
}
q = append(q, root)
for len(q) != 0 {
sz := len(q)
tmp := []int{}
for i := 0; i < sz; i++ {
front := q[0]
q = q[1:]
tmp = append(tmp, front.Val)
if front.Left != nil {
q = append(q, front.Left)
}
if front.Right != nil {
q = append(q, front.Right)
}
}
res = append(res, tmp)
}
return res
}
代码(9.5 三刷自解)
class Solution {
public:
vector<vector<int>> levelOrder(TreeNode* root) {
vector<vector<int>> res;
if(!root)
return res;
queue<TreeNode*> q;
q.push(root);
while(!q.empty()) {
int sz = q.size();
vector<int> tmp;
for(int i = 0; i < sz; i++) {
auto front = q.front();
if(front->left) q.push(front->left);
if(front->right) q.push(front->right);
tmp.emplace_back(front->val);
q.pop();
}
res.emplace_back(tmp);
}
return res;
}
};