项目实战3-添加分页功能
index.html
<html xmlns:th="http://www.thymeleaf.org" xmlns:width="http://www.w3.org/1999/xhtml" xmlns:>
<head>
<meta charset="utf-8">
<link rel="stylesheet" href="css/index.css">
<script language="JavaScript" src="js/index.js"></script>
</head>
<body>
<div id="div_container">
<div id="div_fruit_list">
<p class="center f30">欢迎使用水果库存后台管理系统</p>
<div style="border: 0px solid red;width:60%; margin-left: 20%; text-align: right">
<a th:href="@{/add.html}" style="border: 0px solid blue;margin-bottom: 4px;">添加新库存记录</a>
</div>
<table id="tbl_fruit">
<tr>
<th class="w20">名称</th>
<th class="w20">单价</th>
<th class="w20">库存</th>
<th>操作</th>
</tr>
<tr th:if="${#lists.isEmpty(session.fruitList)}">
<td colspan="4">对不起,库存为空!</td>
</tr>
<tr th:unless="${#lists.isEmpty(session.fruitList)}" th:each="fruit : ${session.fruitList}">
<!--<td><a th:text="${fruit.fname}" th:href="@{'/edit.do?fid='+${fruit.fid}}">苹果</a></td>-->
<td><a th:text="${fruit.fname}" th:href="@{/edit.do(fid=${fruit.fid})}">苹果</a></td>
<td th:text="${fruit.price}">5</td>
<td th:text="${fruit.fcount}">20</td>
<!--<td><img src="imgs/del.jpg" class="delImg" th:onclick="'delFruit('+${fruit.fid}+')'"/></td>-->
<td><img src="imgs/del.jpg" class="delImg" th:onclick="|delFruit(${fruit.fid})|"/></td>
</tr>
</table>
<div style="width:60%; margin-left: 20%;border: 0px solid red;padding-top: 4px;" class="center">
<input type="button" value="首 页" class="btn" th:onclick="page(1)" th:disabled="${session.pageNo==1}"/>
<input type="button" value="上一页" class="btn" th:onclick="|page(${session.pageNo-1})|" th:disabled="${session.pageNo==1}"/>
<input type="button" value="下一页" class="btn" th:onclick="|page(${session.pageNo+1})|" th:disabled="${session.pageNo==session.pageCount}"/>
<input type="button" value="尾 页" class="btn" th:onclick="|page(${session.pageCount})|" th:disabled="${session.pageNo==session.pageCount}"/>
</div>
</div>
</div>
</body>
</html>
index.css
*{
color: threeddarkshadow;
}
a{
text-decoration-line: none;
}
body{
margin:0;
padding:0;
background-color:#808080;
}
div{
position:relative;
float:left;
}
#div_container{
width:80%;
height:100%;
border:0px solid blue;
margin-left:10%;
float:left;
background-color: honeydew;
border-radius:8px;
}
#div_fruit_list{
width:100%;
border:0px solid red;
}
#tbl_fruit{
width:60%;
line-height:28px;
margin-top:16px;
margin-left:20%;
}
#tbl_fruit , #tbl_fruit tr , #tbl_fruit th , #tbl_fruit td{
border:1px solid gray;
border-collapse:collapse;
text-align:center;
font-size:16px;
font-family:"黑体";
font-weight:lighter;
}
.w20{
width:20%;
}
.delImg{
width:24px;
height:24px;
}
.btn{
border:1px solid lightgray;
width:80px;
height:24px;
}
.center{
text-align: center;
}
.f30{
font-size: 30px;
}
IndexServlet 类
//Servlet从3.0版本开始支持注解的方式进行注册
@WebServlet("/index")
public class IndexServlet extends ViewBaseServlet{
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
Integer pageNo = 1;
String pageNoStr = request.getParameter("pageNo");
if (StringUtil.isNotEmpty(pageNoStr)){
pageNo = Integer.parseInt(pageNoStr);
}
HttpSession session = request.getSession();
session.setAttribute("pageNo",pageNo);
FruitDAO fruitDAO = new FruitDaoImpl();
List<Fruit> fruitList = fruitDAO.getFruitList(pageNo);
session.setAttribute("fruitList",fruitList);
//总记录条数
int fruitCount = fruitDAO.getFruitCount();
//总页数
int pageCount = (fruitCount+5-1)/5;
/*
总记录条数 总页数
1 1
5 1
6 2
10 2
11 3
fruitCount (fruitCount+5-1)/5
*/
session.setAttribute("pageCount",pageCount);
//此处的视图名称是index
//那么thymeleaf会将这个 逻辑视图 名称对应到 物理视图 名称上去
// //逻辑视图名称:index
//物理视图名称: viw-prefix + 逻辑视图名称 +view-suffix
//所以真实的视图名称是: / index .html
super.processTemplate("index",request,response);
}
}
FruitDAO 类
public interface FruitDAO {
//获取指定页码上的库存列表信息,每页显示5条
List<Fruit> getFruitList(Integer pageNo);
//根据fid获取特定的水果库存信息
Fruit getFruitByFid(Integer fid);
//修改指定的库存记录
void updateFruit(Fruit fruit);
//根据fid删除指定的库存记录
void delFruit(Integer fid);
//添加新库存记录
void addFruit(Fruit fruit);
//查询库存总记录条数
int getFruitCount();
}
FruitDaoImpl 类
public class FruitDaoImpl extends BaseDAO<Fruit> implements FruitDAO {
@Override
public List<Fruit> getFruitList(Integer pageNo) {
return super.executeQuery("select * from t_fruit limit ?,5",(pageNo-1)*5);
}
@Override
public Fruit getFruitByFid(Integer fid) {
return super.load("select * from t_fruit where fid = ?",fid);
}
@Override
public void updateFruit(Fruit fruit) {
String sql = "update t_fruit set fname = ?,price = ?,fcount = ?,remark = ? where fid = ?";
super.executeUpdate(sql,fruit.getFname(),fruit.getPrice(),fruit.getFcount(),fruit.getRemark(),fruit.getFid());
}
@Override
public void delFruit(Integer fid) {
super.executeUpdate("delete from t_fruit where fid = ?",fid);
}
@Override
public void addFruit(Fruit fruit) {
String sql = "insert into t_fruit values(0,?,?,?,?)";
super.executeUpdate(sql, fruit.getFname(), fruit.getPrice(), fruit.getFcount(), fruit.getRemark());
}
@Override
public int getFruitCount() {
//count()方法返回的是Long类型
return ((Long) super.executeComplexQuery("select count(*) from t_fruit")[0]).intValue();
}
}
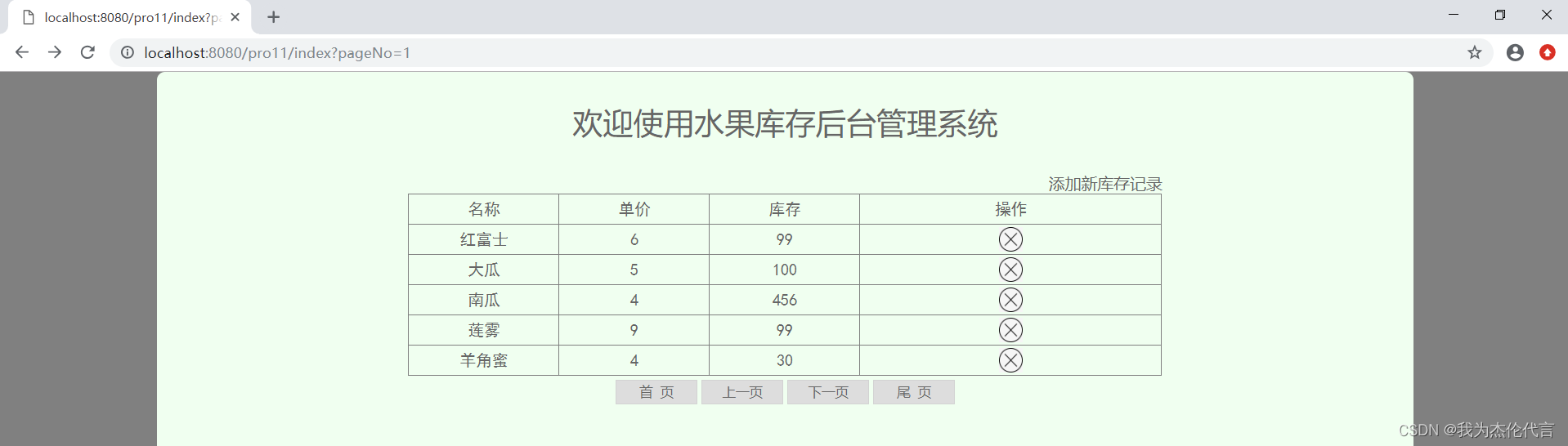