#include"iostream"
#include"map"
#include"algorithm"
#include"string"
using namespace std ;
// 什么是map容器?
// map中所有的元素都是pair
// 什么是pair?
// pair是一个成对出现的数据。
// 如下程序:
void pairs()
{
// 创建一个数对
pair<string,double> p1(string("clucy"),120);
// 输出数对
cout<<p1.first<<p1.second<<endl;
// 修改pair
p1.first="Blocla";
p1.second=150;
cout<<p1.first<<p1.second<<endl;
}
// 但是在map中,pair的第一个值为键值,第二个值为实值。
// 所有的元素都会根据元素的键值自动排序。
// 本质:
// 底层是由带哈希的红黑树实现。
// 优点:
// 可以根据key值快速的找到value的值。
// map和multimap的区别
// map只能允许一个value值,而mutimap相反。
// 生成数据到map
void genMap(multimap<string,double> &mp)
{
for(int i=0;i<10;i++)
{
mp.insert(make_pair("a",i+1));
}
}
// 打印map
void printMap(multimap<string,double> &mp)
{
for(multimap<string,double>::iterator it = mp.begin();it!=mp.end();it++)
{
cout<<(*it).first<<(*it).second<<endl;
}
}
// map的构造
void constructor()
{
// 默认构造
multimap<string,double> mp;
// 生成数据
genMap(mp);
// 打印数据
printMap(mp);
// 拷贝构造
multimap<string,double> mp2(mp);
printMap(mp2);
// 赋值
multimap<string,double> mp3= mp;
printMap(mp3);
}
// 大小交换
void mapSizeAndSwapMap()
{
multimap<string,double> mp;
genMap(mp);
printMap(mp);
cout<<"The size is ::"<<mp.size()<<endl;
// 判断map是否为空
if(mp.empty())
{
cout<<"This map is not empty"<<endl;
}
else
{
cout<<"This map is empty"<<endl;
}
// 交换两个map的值
multimap<string,double> mp2;
mp2.insert(make_pair("b1,",23));
mp2.insert(make_pair("b2,",42));
mp2.insert(make_pair("b3,",34));
//
mp.swap(mp2);
printMap(mp);
printMap(mp2);
}
// map的插入和删除
void mapInsertAndDelete()
{
multimap<string,double> mp;
// 插入元素
mp.insert(make_pair("Zanpian",234.2));
mp.insert(make_pair("Zanpian",234.2));
mp.insert(make_pair("Zanpian",234.2));
printMap(mp);
// 迭代器的方式删除
mp.erase(mp.begin());
// 范围方式删除
mp.erase(mp.begin(),mp.end());
// 删除key值为:Zanpian,这种方法只能安装key值的方式删除元素
mp.erase("Zanpian");
printMap(mp);
// 清除map
mp.clear();
printMap(mp);
}
// map的查找和统计
void mapFindAndCount()
{
multimap<string,double> mp;
genMap(mp);
printMap(mp);
// 查找map的key值为a的元素
multimap<string,double>::iterator it = mp.find("a");
// 输出
cout<<"the result is ::";
cout<<it->first<<it->second<<endl;
// 统计key为a的个数
cout<<mp.count("a")<<endl;
}
// 自定义数据类型的排序规则仿函数
class Cat{
string name;
public:
int age;
public:
Cat(string name,int age)
{
this->name = name;
this->age = age;
}
void showInfo()
{
cout<<this->name<<this->age<<endl;
}
};
// 定义排序规则
class mapSort{
public:
bool operator()(Cat &c1,Cat &c2)
{
return c1.age>c2.age;
}
};
void mapClassSort()
{
multimap<int,Cat> mp;
mp.insert(make_pair(1,Cat("Nany",23)));
mp.insert(make_pair(1,Cat("Nany",2)));
mp.insert(make_pair(1,Cat("Nany",1)));
mp.insert(make_pair(1,Cat("Nany",3)));
// cout<<mp.begin()->first;
// mp.begin()->second.showInfo();
// 打印输出
for(multimap<int,Cat>::iterator it = mp.begin();it!=mp.end();it++)
{
cout<<(*it).first<<endl;
(*it).second.showInfo();
}
}
int main()
{
mapClassSort();
return 0;
}
C++ Map容器的使用和概念
最新推荐文章于 2023-04-24 17:25:50 发布
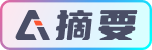