目录
构造注入
//实体类参数
private AnotherBean anotherBean1;
private char sex;
<!-- 无参构造注入-->
<bean id="anotherBean" class="org.zpf.demo.AnotherBean"></bean>
<bean id="bean" class="org.zpf.demo.Bean" >
<!-- name:对应实体类中的参数
ref:对应实体类中依赖的其他类BeanId,依赖类也必须注入依赖
value:给对应的参数赋值
-->
<property name="anotherBean1" ref="anotherBean"></property>
<!-- 也可注入时在创建内部Bean 此注入和上述注入都可以使用-->
<property name="anotherBean1">
<bean class="org.zpf.demo.AnotherBean"></bean>
</property>
<property name="sex" value="男"></property>
</bean>
Set注入
//实体类参数
private AnotherBean anotherBean;
private String name;
<bean id="bean" class="org.zpf.demo.Bean">
<!-- set构造注入-->
<!--
index:第几个参数,从0开始
name :注入依赖的参数名
type :注入依赖的路径
ref :对应实体类中依赖的其他类BeanId,依赖类也必须注入依赖
-->
<bean id="anotherBean" class="org.zpf.demo.AnotherBean"></bean>
<constructor-arg index="0" name="anotherBean" type="org.zpf.demo.AnotherBean" ref="anotherBean"></constructor-arg>
<constructor-arg index="1" name="name" type="java.lang.String" value="zpf">
</constructor-arg>
</bean>
集合类Bean注入
List集合
//实体类参数
private List<String> list;
private List<AnotherBean> anotherBeansList;
<!-- List -->
<property name="list">
<list>
<value>aaa</value>
<value>bbb</value>
<value>ccc</value>
</list>
</property>
<bean id="anotherBean" class="org.zpf.demo.AnotherBean"></bean>
<property name="anotherBeansList">
<list>
<ref bean="anotherBean"></ref>
<ref bean="anotherBean"></ref>
</list>
</property>
Set集合
//实体类参数
private Set<String> set;
private Set<AnotherBean> anotherBeansSet;
<!-- Set -->
<bean id="bean" class="org.zpf.demo.Bean" >
<property name="set">
<set>
<value>aaa</value>
<value>aaa</value>
<value>aaa</value>
</set>
</property>
<bean id="anotherBean" class="org.zpf.demo.AnotherBean"></bean>
<property name="anotherBeansSet">
<set>
<ref bean="anotherBean"></ref>
<ref bean="anotherBean"></ref>
</set>
</property>
</bean>
Map集合
//实体类参数
private Map<String,String > map;
private Map<AnotherBean,AnotherBean> anotherBeansMap;
<!-- Map -->
<bean id="bean" class="org.zpf.demo.Bean" >
<!-- Map -->
<property name="map">
<map>
<entry key="a" value="b"></entry>
<entry key="c" value="d"></entry>
</map>
</property>
<bean id="anotherBean" class="org.zpf.demo.AnotherBean"></bean>
<property name="anotherBeansMap">
<map>
<entry key-ref="anotherBean" value-ref="anotherBean"></entry>
</map>
</property>
</bean>
Properties集合
//实体类参数
private Properties properties;
<!-- Properties-->
<bean id="bean" class="org.zpf.demo.Bean" >
<property name="properties">
<props>
<prop key="abc">aabbcc</prop>
</props>
</property>
</bean>
作用域: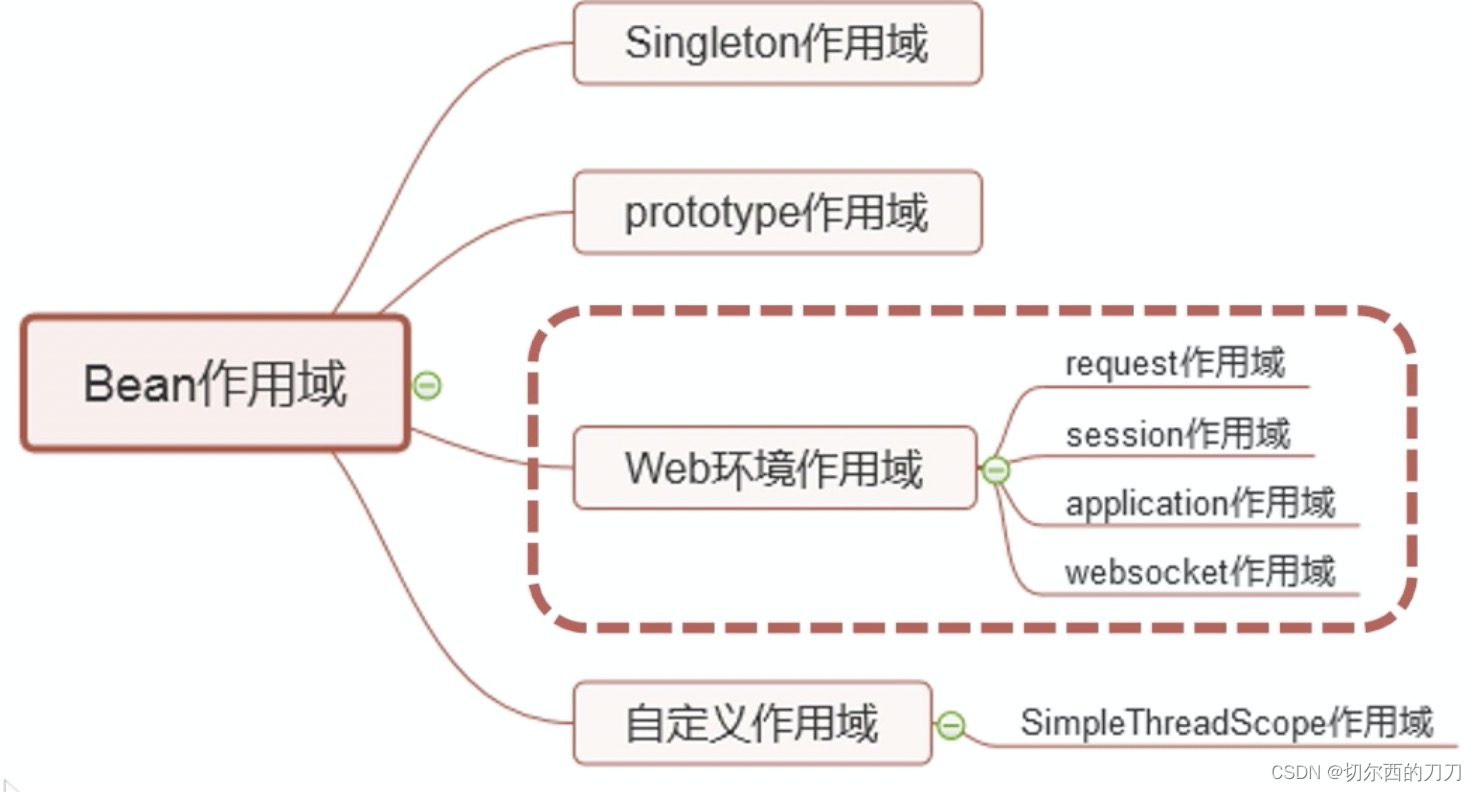
singleton(单例)作用域
prototype(多例)作用域
在Spring中,Bean,要么是单例要么是多例,默认单例
在Spring中,bean的Scope常被定义的两种模式:prototype(多例)和singleton(单例)
singleton(单例):只有一个共享的实例存在,singleton类型的bean定义从容器启动到第一次被请求而实例化开始,只要容器不销毁或退出,该类型的bean的单一实例就会一直存活,典型单例模式,简单理解:在一次上下文配置中之创建一次实例。
prototype(多例):对这个bean的每次请求都会创建一个新的bean实例,类似于new。
好处:
单例,是因为没必要每个请求都新建一个对象,这样做浪费CPU又浪费内存;
多例,是为了防止并发问题;即一个请求改变了对象的状态,此时对象又处理另一个请求,而之前请求对对象状态的改变导致了对象对另一个请求做了错误的处理;
<!--单例-->
<bean id="anotherBean" class="org.zpf.demo.AnotherBean" scope="singleton"></bean>
<!--多例-->
<bean id="anotherBean" class="org.zpf.demo.AnotherBean" scope="prototype"></bean>
<!--Bean实例依赖别的Bean实例
anotherBean为单例模式,上下文配置中之创建一次,属于共享实例
bean也为单例模式,上下文配置中之创建一次,属于共享实例
-->
<bean id="anotherBean" class="org.zpf.demo.AnotherBean" scope="singleton"></bean>
<bean id="bean" class="org.zpf.demo.Bean" scope="singleton">
<property name="anotherBean" ref="anotherBean"></property>
<property name="stringBean" value="aaa"></property>
</bean>
<!--Bean实例依赖别的Bean实例
anotherBean为多例模式,每次创建上下文配置都会new一个新的实例
bean也为单例模式,上下文配置中之创建一次,属于共享实例
-->
<bean id="anotherBean" class="org.zpf.demo.AnotherBean" scope="prototype"></bean>
<bean id="bean" class="org.zpf.demo.Bean" scope="singleton">
<property name="anotherBean" ref="anotherBean"></property>
<property name="stringBean" value="aaa"></property>
</bean>
<!--Bean实例依赖别的Bean实例
anotherBean为单例模式,上下文配置中之创建一次,属于共享实例
bean也为多例模式,每次创建上下文配置都会new一个新的实例
-->
<bean id="anotherBean" class="org.zpf.demo.AnotherBean" scope="singleton"></bean>
<bean id="bean" class="org.zpf.demo.Bean" scope="prototype">
<property name="anotherBean" ref="anotherBean"></property>
<property name="stringBean" value="aaa"></property>
</bean>
<!--Bean实例依赖别的Bean实例
anotherBean为多例模式,每次创建上下文配置都会new一个新的实例
bean也为多例模式,每次创建上下文配置都会new一个新的实例
-->
<bean id="anotherBean" class="org.zpf.demo.AnotherBean" scope="prototype"></bean>
<bean id="bean" class="org.zpf.demo.Bean" scope="prototype">
<property name="anotherBean" ref="anotherBean"></property>
<property name="stringBean" value="aaa"></property>
</bean>
web环境下的作用域
1.request
表示该针对每一次HTTP请求都会创建新的bean实例,同时该bean仅在当前HTTP request内有效。
使用web相关作用域,首先要在初始化web的web.xml中做如下配置:如果你使用的是Servlet 2.4及以上的web容器,那么你仅需要在web应用的XML声明文件web.xml中增加下述ContextListener即可:
<web-app> ... <listener> <listener-class>org.springframework.web.context.request.RequestContextListener</listener-class> </listener> ... </web-app>如果是Servlet2.4以前的web容器,那么你要使用一个javax.servlet.Filter的实现:
<web-app> .. <filter> <filter-name>requestContextFilter</filter-name> <filter-class>org.springframework.web.filter.RequestContextFilter</filter-class> </filter> <filter-mapping> <filter-name>requestContextFilter</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> ... </web-app>接着既可以配置bean的作用域:
<bean id="role" class="spring.chapter2.maryGame.Role" scope="request"/>2. session
在同一session中只会创建一个实例。而对不同的Session请求则会创建新的实例,该bean实例仅在当前Session内有效。配置实例:和request配置实例的前提一样,配置好web启动文件就可以如下配置:
<bean id="role" class="spring.chapter2.maryGame.Role" scope="session"/>5. global session
global session作用域类似于标准的HTTP Session作用域,不过它仅仅在基于portlet的web应用中才有意义。Portlet规范定义了全局Session的概念,它被所有构成某个 portlet web应用的各种不同的portlet所共享。在global session作用域中定义的bean被限定于全局portlet Session的生命周期范围内。如果你在web中使用global session作用域来标识bean,那么web会自动当成session类型来使用。配置实例:和request配置实例的前提一样,配置好web启动文件就可以如下配置:
<bean id="role" class="spring.chapter2.maryGame.Role" scope="global session"/>
懒加载
解释:spring容器会在创建容器时提前初始化作用域为Singleton作用域的Bean,如果该Bean被标注为lazy-init=“true”,则该Bean只有在被需要的时候才会创建
注意:只有作用域:scope=‘singleton’ 可以设置懒加载
配置:lazy-init="true" 开启懒加载
<bean id="anotherBean" class="org.zpf.demo.AnotherBean" scope="singleton" lazy-init="true"></bean>
为什么其他作用域不需要懒加载:因为其他作用域没法判断什么时候会被需要,而如果是单例只存在一个实例,那就可以考虑使用懒加载
如果上下文配置.xml中有很多Bean都需要开启懒加载可以在 .xml中<beans/>中配置
<beans default-lazy-init="true">
</beans>
使用场景:
某个Bean在整个程序运行间被使用的概率非常小,可以考虑设置为懒加载。
优点:节约资源,缺点:可能会导致某个操作相应稍慢一点