编程导航算法通关村第 1关 | 链表的操作
单链表
链表的定义
public class ListNode {
public int val;
public ListNode next;
ListNode(int x) {
val = x;
next = null;
}
}
初始化
public static ListNode init(ListNode head) {
int i = 2;
ListNode temp = head;
while (i <= 10) {
ListNode listNode = new ListNode(i);
temp.next = listNode;
temp = temp.next;
i++;
}
return head;
}
链表的遍历
public static void print(ListNode head) {
ListNode temp = head;
while (temp != null) {
System.out.println(temp.val);
temp = temp.next;
}
}
获取链表的长度
public static int getLength(ListNode head) {
ListNode temp = head;
int length = 0;
while (temp != null) {
temp = temp.next;
length++;
}
return length;
}
链表的插入
- 在链表的插入中,我们需要考虑三种情况
- 头结点前插入:直接将newNode的next指向head,然后将head指向为第一个节点(newNode)
newNode.next = head;
head = newNode;
return head;
ListNode temp = head;
while (temp.next != null) {
temp = temp.next;
}
temp.next = newNode;
return head;
- 中间插入:需要将指针指向待插入位置的前一个节点
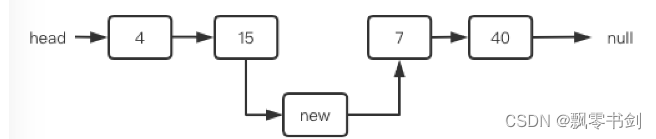
ListNode temp = head;
int i = 1;
while (i < posttion - 1) {
temp = temp.next;
i++;
}
newNode.next = temp.next;
temp.next = newNode;
return head;
public static ListNode insert(ListNode head, ListNode newNode, int posttion) {
if (head == null) {
return null;
}
if (newNode == null) {
return null;
}
int length = getLength(head);
if (posttion <= 0 || posttion > length) {
return null;
}
if (posttion == 1) {
newNode.next = head;
head = newNode;
return head;
}
if (posttion == length) {
ListNode temp = head;
while (temp.next != null) {
temp = temp.next;
}
temp.next = newNode;
return head;
}
ListNode temp = head;
int i = 1;
while (i < posttion - 1) {
temp = temp.next;
i++;
}
newNode.next = temp.next;
temp.next = newNode;
return head;
}
链表的节点的删除
public static ListNode delete(ListNode head, int posttion) {
if (head == null) {
return null;
}
if (posttion <= 0 || posttion > getLength(head)) {
return null;
}
if (posttion == 1) {
head = head.next;
return head;
}
if (posttion == getLength(head)) {
ListNode temp = head;
while (temp.next.next != null) {
temp = temp.next;
}
temp.next = null;
return head;
}
ListNode temp = head;
int i = 1;
while (i < posttion - 1) {
temp = temp.next;
i++;
}
temp.next = temp.next.next;
return head;
}
双向链表
节点的定义
package com.lmx.project.first.bronze;
class DoubleNode {
public int data;
public DoubleNode next;
public DoubleNode prev;
public DoubleNode(int data) {
this.data = data;
}
public void displayNode() {
System.out.print("{" + data + "} ");
}
}
双向链表的定义
private DoubleNode first;
private DoubleNode last;
public DoublyLinkList() {
first = null;
last = first;
}
节点的打印
* 正序打印
*/
public void printOrder() {
DoubleNode temp = first;
while (temp != null) {
temp.displayNode();
temp = temp.next;
}
}
public void printRevereOrder() {
DoubleNode temp = last;
while (temp != null) {
temp.displayNode();
temp = temp.prev;
}
}
获取长度
public int getLength() {
int length = 0;
DoubleNode temp = first;
while (temp != null) {
length++;
temp = temp.next;
}
return length;
}
头部插入元素
public void insertFirst(int data) {
DoubleNode newNode = new DoubleNode(data);
if (first == null) {
first = newNode;
last = first;
return;
}
newNode.next = first;
first.prev = newNode;
first = newNode;
}
尾部插入元素
public void insertLast(int data) {
DoubleNode newNode = new DoubleNode(data);
if (first == null) {
first = newNode;
last = first;
return;
}
newNode.prev = last;
last.next = newNode;
last = newNode;
}
- 中间插入元素
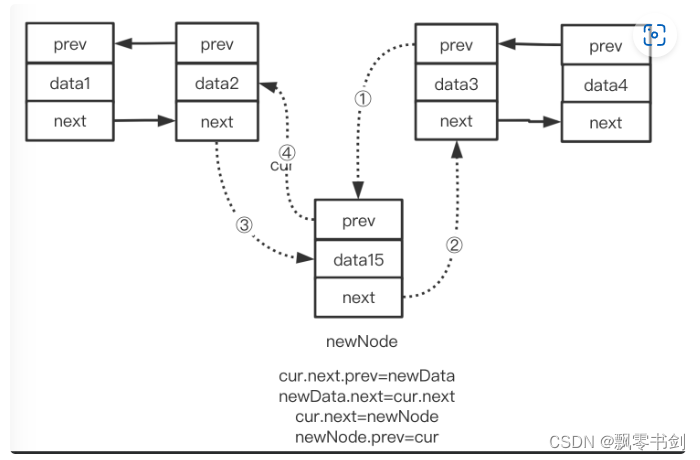
public void insert(int data, int position) {
int length = getLength();
if (position <= 0 || position > length) {
return;
}
if (position == 1) {
insertFirst(data);
return;
}
if (position == length + 1) {
insertLast(data);
return;
}
DoubleNode newNode = new DoubleNode(data);
DoubleNode temp = first;
int i = 1;
while (i < position - 1) {
temp = temp.next;
i++;
}
temp.next.prev = newNode;
newNode.next = temp.next;
temp.next = newNode;
newNode.prev = temp;
}
链表的删除
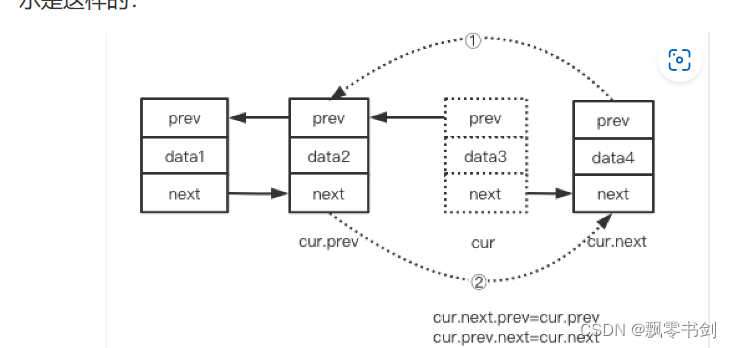
public DoubleNode delete(int key) {
DoubleNode temp = first;
while (temp != null && temp.data != key) {
temp = temp.next;
}
if (temp == null) {
return temp;
}
if (temp == first) {
first = first.next;
first.prev = null;
} else if (temp == last) {
last.prev.next = null;
} else {
temp.next.prev = temp.prev;
temp.prev.next = temp.next;
temp.next = null;
temp.prev = null;
}
return temp;
}