#pragma once
#include<iostream>
using namespace std;
template<class T>
class MyArray {
public:
MyArray(int Capacity) {
this->Capacity = Capacity;
this->Size = 0;
this->pAddress = new T[this->Capacity];
}
//尾插法
void PushBack(const T& val) {
//判断容量是否等于大小
if (this->Capacity == this->Size)
return;
this->pAddress[this->Size] = val;
this->Size++;
}
//尾删法
void PopBack() {
//让用户访问不到最后一个元素即可。逻辑删除
if (this->Size == 0)
return;
this->Size--;
}
//通过下标方式访问数组中的元素
T& operator[](int index) {
return this->pAddress[index];
}
//返回数组的容量
int getCapacity() {
return this->Capacity;
}
//返回数组的数据元素个数
int getSize() {
return this->Size;
}
~MyArray() {
if (this->pAddress != NULL) {
delete[] this->pAddress;
this->pAddress = NULL;
this->Capacity = 0;
this->Size = 0;
}
}
MyArray(const MyArray& arr) {
this->Capacity = arr.Capacity;
this->Size = arr.Size;
this->pAddress = new T[arr.Capacity];
for(int i=0;i<this->Size;i++)
this->pAddress[i] = arr.pAddress[i];
}
MyArray& operator=(const MyArray& arr) {
//先判断原来堆区是否有数据,如果有,先释放
if (this->pAddress != NULL) {
delete[]this->pAddress;
this->pAddress = NULL;
this->Capacity = 0;
this->Size = 0;
}
this->Capacity = arr.Capacity;
this->Size = arr.Size;
this->pAddress = new T[arr->Capacity];
for (int i = 0; i < this->Size; i++)
this->pAddress[i] = arr.pAddress[i];
return *this;
}
private:
T* pAddress; //指针指向堆区开辟的真实数组
int Capacity; //数组容量
int Size; //数组元素个数
};
类模板案例-数组类封装
最新推荐文章于 2024-09-09 12:31:15 发布
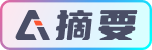