一.封装返回值
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
/**
* @author jitwxs
* @date 2023年01月05日 17:18
*/
@Data
@NoArgsConstructor
@AllArgsConstructor
public class ResultUtil {
private Integer code;
private String mess;
public static ResultUtil ok(){ return new ResultUtil(200, null); };
public static ResultUtil fail(String errorMess){ return new ResultUtil( 0, errorMess); };
}
二.处理系统异常
1.1处理参数缺失异常
@ControllerAdvice 注解即可拦截项目中抛出的异常。
在方法上通过 @ExceptionHandler 注解来指定具体的异常,然后在方法中处理该异常信息,最后将结果通过统一的 json 结构体返回给调用者。
加了 @RequestParam 注解,如果缺少参数,会抛出 org.springframework.web.bind.MissingServletRequestParameterException 异常。
import my2.utils.ResultUtil;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpStatus;
import org.springframework.validation.BindException;
import org.springframework.web.bind.MissingServletRequestParameterException;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.ResponseStatus;
@ControllerAdvice
@ResponseBody
public class GlobalExceptionHandler {
private static final Logger logger = LoggerFactory.getLogger(GlobalExceptionHandler.class);
/**
* RequestParam 缺少请求参数异常
* @param ex HttpMessageNotReadableException
* @return
*/
@ExceptionHandler(MissingServletRequestParameterException.class)
@ResponseStatus(value = HttpStatus.BAD_REQUEST)
public ResultUtil handleHttpMessageNotReadableException(MissingServletRequestParameterException ex) {
logger.error("缺少请求参数,{}", ex.getMessage());
return new ResultUtil(400, "RequestParam 缺少必要的请求参数");
}
}
1.2测试处理参数缺失异常
@GetMapping("test")
public void test(@RequestParam String s,@RequestParam int age) {
logger.info("s:{}",s);
logger.info("age:{}",age);
}
测试结果:
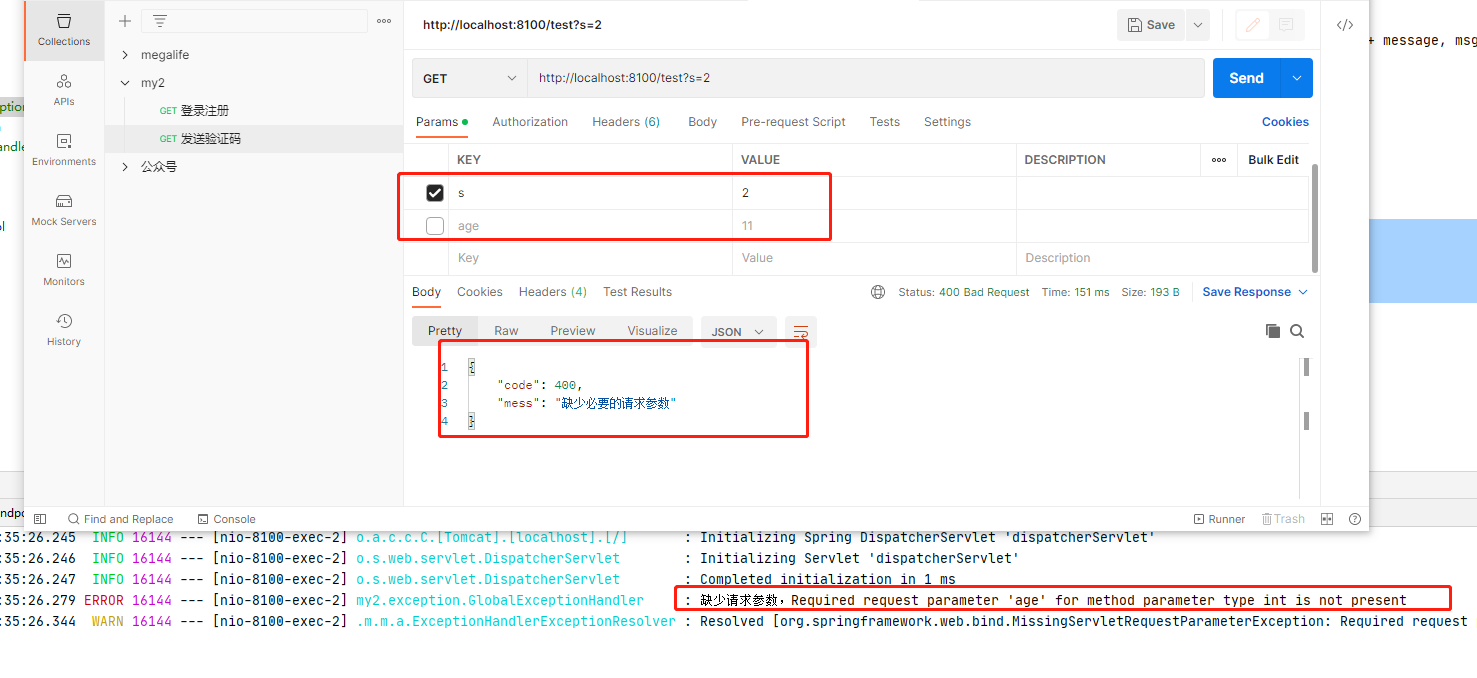
2.1处理空指针异常
/**
* 空指针异常
* @param ex NullPointerException
* @return
*/
@ExceptionHandler(NullPointerException.class)
@ResponseStatus(value = HttpStatus.INTERNAL_SERVER_ERROR)
public ResultUtil handleTypeMismatchException(NullPointerException ex) {
logger.error("空指针异常,{}", ex.getMessage());
return new ResultUtil(500, "空指针异常了");
}
2.2测试处理空指针异常
@GetMapping("test")
public void test(@RequestParam String s,@RequestParam int age) {
logger.info("s:{}",s);
logger.info("age:{}",age);
String s1 = null;
s1.toString();
}
测试结果:
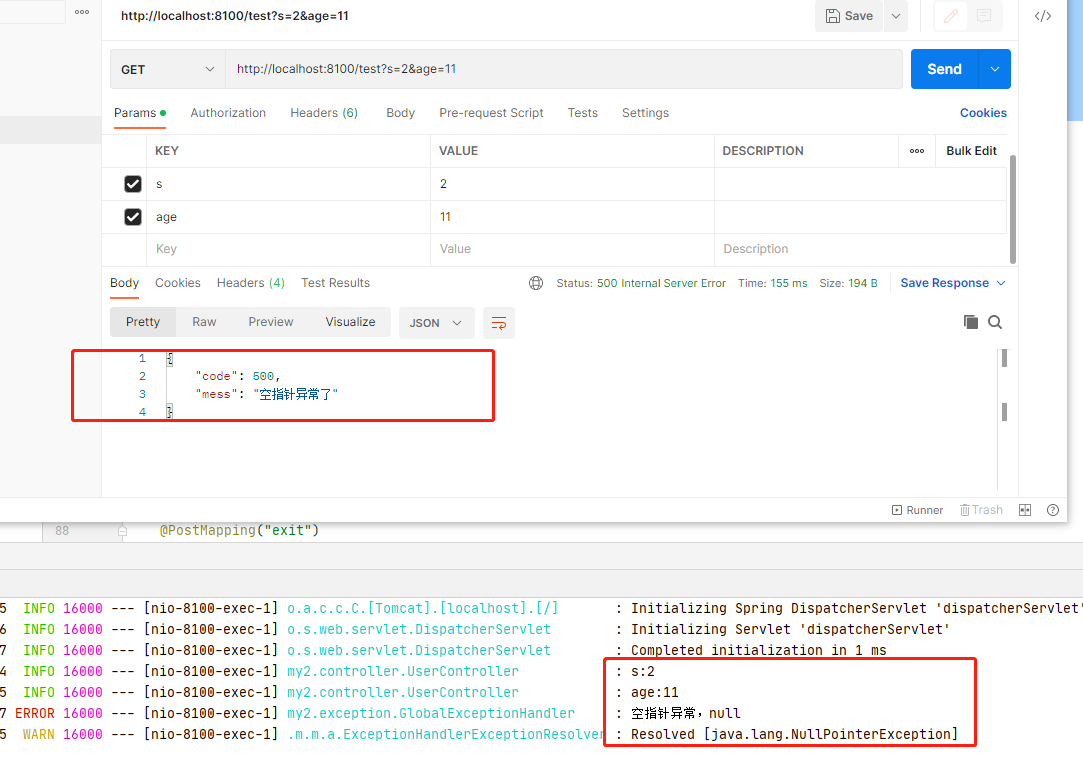
3.1系统未知异常
/**
* 系统异常 预期以外异常
* @param ex
* @return
*/
@ExceptionHandler(Exception.class)
@ResponseStatus(value = HttpStatus.INTERNAL_SERVER_ERROR)
public ResultUtil handleUnexpectedServer(Exception ex) {
logger.error("系统异常:", ex);
return new ResultUtil(500, "系统发生异常,请联系管理员");
}
3.2测试系统未知异常
@GetMapping("test")
public void test(@RequestParam String s,@RequestParam int age) {
logger.info("s:{}",s);
logger.info("age:{}",age);
int n = 0/0;
}
测试结果:
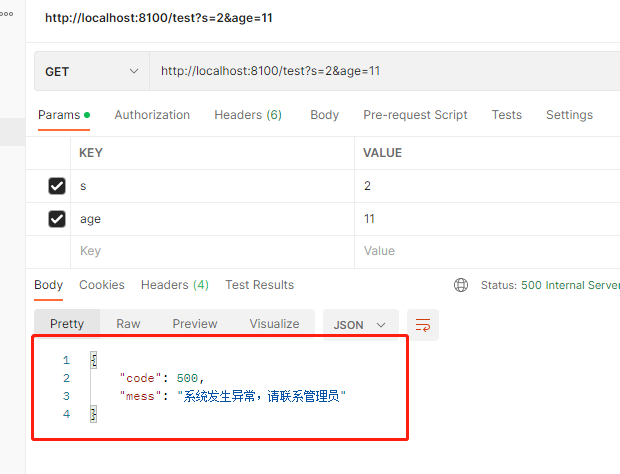
三.处理自定义异常
定义异常信息
public enum BusinessMsgEnum {
/** 参数异常 */
PARMETER_EXCEPTION("102", "参数异常!"),
/** 等待超时 */
SERVICE_TIME_OUT("103", "服务调用超时!"),
/** 参数过大 */
PARMETER_BIG_EXCEPTION("102", "输入的图片数量不能超过50张!"),
/** 500 : 发生异常 */
UNEXPECTED_EXCEPTION("500", "系统发生异常,请联系管理员!");
/**
* 消息码
*/
private String code;
/**
* 消息内容
*/
private String msg;
private BusinessMsgEnum(String code, String msg) {
this.code = code;
this.msg = msg;
}
public String code() {
return code;
}
public String msg() {
return msg;
}
}
public class BusinessErrorException extends RuntimeException {
private static final long serialVersionUID = -7480022450501760611L;
/**
* 异常码
*/
private String code;
/**
* 异常提示信息
*/
private String message;
public BusinessErrorException(BusinessMsgEnum businessMsgEnum) {
this.code = businessMsgEnum.code();
this.message = businessMsgEnum.msg();
}
public String getCode() {
return code;
}
public void setCode(String code) {
this.code = code;
}
@Override
public String getMessage() {
return message;
}
public void setMessage(String message) {
this.message = message;
}
}
拦截自定义异常
/**
* 拦截业务异常,返回业务异常信息
* @param ex
* @return
*/
@ExceptionHandler(BusinessErrorException.class)
@ResponseStatus(value = HttpStatus.INTERNAL_SERVER_ERROR)
public ResultUtil handleBusinessError(BusinessErrorException ex) {
Integer code = Integer.valueOf(ex.getCode());
String message = ex.getMessage();
return new ResultUtil(code, message);
}
测试
@GetMapping("test")
public void test(@RequestParam String s,@RequestParam int age) {
logger.info("s:{}",s);
logger.info("age:{}",age);
throw new BusinessErrorException(BusinessMsgEnum.SERVICE_TIME_OUT);
}
测试结果:
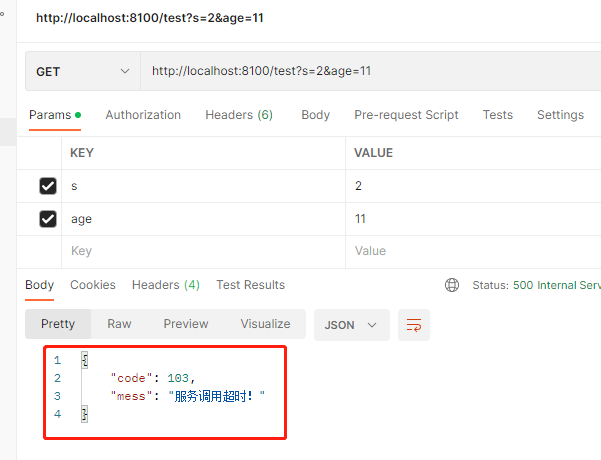