1.window窗体
数据格式(纯文本.txt,.json文件)
读取某个格式的文件
数据格式成类
数据渲染
json参考笔记
https://www.runoob.com/json/json-eval.html
2.json概念
是一种轻量级数据交互格式。
轻量级说明此种数据格式在网络传输的时候所占的带宽少,传输效率高。
和xml相比较是比较轻量的。
xml是一种老旧的数据交换格式。
json是目前市场最流行的一种数据交换格式。很多语言都支持它,如:js,java,c#等。
3.json格式文件特点:
以键值对(名值对)的形式存储数据。如:"name":"value"。称name为键,value为值,也可称name为属性名称,value为属性值。
每一个键值对必须以英文逗号分割,但最后一个键值对除外。
属性值(键值)如果是字符串必须是双引号包裹,不能使用单引号。而属性名称(键)必须使用双引号包裹。
属性值(键值)如果是数字、布尔不需要使用双引号包裹。
属性名称是区分大小写。
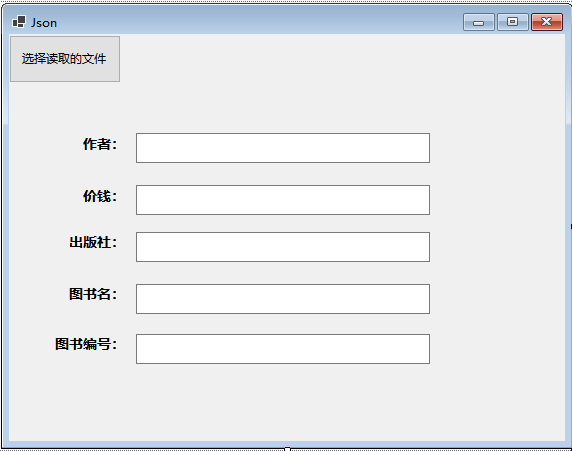
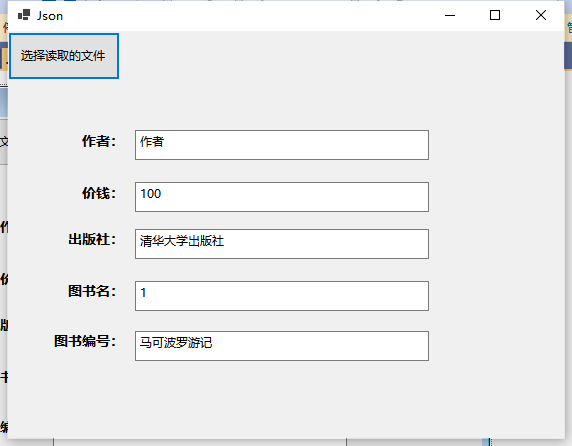
要读取的JSON文件:book.json
{
"Author": "作者",
"price": 100,
"publisher": "清华大学出版社",
"bookId": 1,
"bookName": "马可波罗游记"
}
book对应的类
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Day13_W
{
public class book
{
public int bookId { get; set; }
public string? bookName { get; set; }
public string? Author { get; set; }
public int price { get; set; }
public string? publisher { get; set; }
}
}
完整窗体代码:
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
using static System.Windows.Forms.VisualStyles.VisualStyleElement;
namespace Day13_W
{
public partial class Json : Form
{
public Json()
{
InitializeComponent();
#region 设置文本框大小
textBox1.AutoSize = false;
textBox2.AutoSize = false;
textBox3.AutoSize = false;
textBox4.AutoSize = false;
textBox5.AutoSize = false;
textBox1.Height = 30;
textBox2.Height = 30;
textBox3.Height = 30;
textBox4.Height = 30;
textBox5.Height = 30;
#endregion
}
private void button1_Click(object sender, EventArgs e)
{
openFileDialog1.ShowDialog(this);
if (!string.IsNullOrEmpty(openFileDialog1.FileName))
{
string str = rf(openFileDialog1.FileName);
//JsonConvert.DeserializeObject<book>(str)将json数据str反序列化成为book类型
book? b = JsonConvert.DeserializeObject<book>(str);
if (b != null)
{
textBox1.Text = b.Author;
textBox2.Text = b.price.ToString();
textBox3.Text = b.publisher;
textBox4.Text = b.bookId.ToString();
textBox5.Text = b.bookName;
}
}
}
private static string rf(string fileName)
{
StreamReader sr = new StreamReader(fileName, Encoding.Default);
string content = sr.ReadToEnd();
sr.Close();
return content;
}
}
}